导读
1.多态简介
2.向上造型与向下造型
3.instanceof的运用
多态简介
多态允许不同类的对象对相同的信息作出不同的响应。
一般java所指的多态是运行时多态
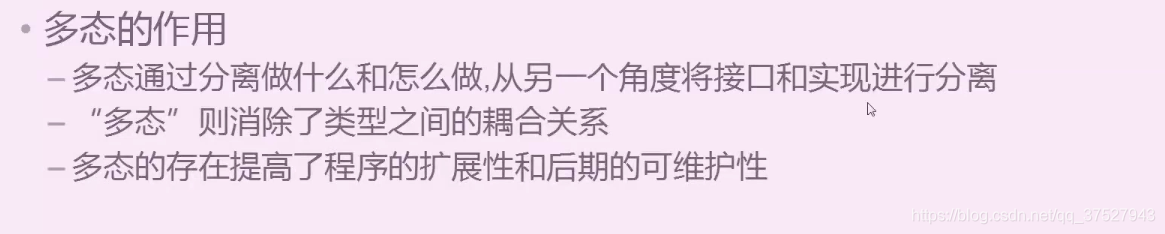
向上造型与向下造型
Animal父类
package com.hala.animal;
public class Animal {
protected String name;
private int math;
private String species;
//父类构造方法不能被继承,不能被重写
public Animal(){
}
public Animal(String name,int math){
this.setName(name);
this.setMath(math);
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getMath() {
return math;
}
public void setMath(int math) {
this.math = math;
}
public String getSpecies() {
return species;
}
public void setSpecies(String species) {
this.species = species;
}
//吃东西
public void eat(){
System.out.println("动物可以吃东西。");
}
}
Cat子类
package com.hala.animal;
public class Cat extends Animal {
//子类可以继承父类所有的非私有成员,没有选择性
private double weight;
public Cat(){
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
//跑动方法
public void run(){
System.out.println("小猫正在跑。");
}
@Override
public void eat() {
// TODO Auto-generated method stub
super.eat();
System.out.println("小猫会吃鱼");
}
}
Dog子类
package com.hala.animal;
public class Dog extends Animal {
private String sex;
public Dog(){
}
public Dog(String name,int math){
super(name,math);
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
//睡觉方法
public void sleep(){
System.out.println("小狗正在睡觉。");
}
//这里是注解,表示重写父类的方法
@Override
public void eat() {
// 继承父类eat方法的格式,要记住!
super.eat();
System.out.println("小狗会吃肉");
}
}
测试类
import com.hala.animal.Animal;
import com.hala.animal.Cat;
import com.hala.animal.Dog;
public class Test {
public static void main(String[] args) {
Animal one =new Animal();
/*
* 以下两行称为向上造型又叫自动转型,隐式转型
* 1.父类引用指向子类实例
* 2.可以调用继承父类的方法,以及子类重写父类的方法,但是不能调用子类特有的方法
*/
Animal two=new Cat();
Animal three=new Dog();
one.eat();
two.eat();
three.eat();
System.out.println("+++++++++++++++++++++++++++");
/*
* 以下几行是向下造型,又叫强制转换
* 1.子类引用指向父类对象,此处要进行强制转换,这样可以调用子类所有方法
* 2.转换是有节操的:要看这块空间在根上存的是什么类型的,如two在根上就是
* Cat类型的,它就不能强转换成Dog类型的
*/
Cat four=(Cat)two;
four.eat();
four.run();
}
}
输出结果
动物可以吃东西。
动物可以吃东西。
小猫会吃鱼
动物可以吃东西。
小狗会吃肉
+++++++++++++++++++++++++++
动物可以吃东西。
小猫会吃鱼
小猫正在跑。
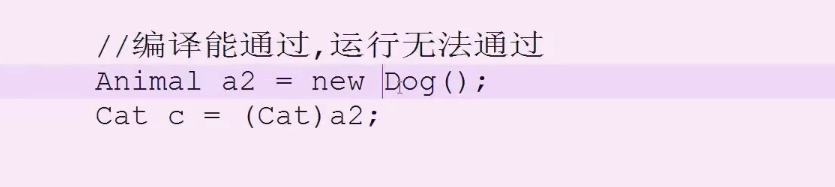
instanceof的运用
package com.hala.test;
import com.hala.animal.Animal;
import com.hala.animal.Cat;
import com.hala.animal.Dog;
public class Test {
public static void main(String[] args) {
Animal two=new Cat();
/*
* instanceof的作用
* 用来检验一个对象是否符合一个类的特征,返回值为boolean
* 可以匹配本类或者父类
*/
if(two instanceof Cat){
Cat four=(Cat)two;
four.eat();
four.run();
System.out.println("我是Cat~~");
}
if(two instanceof Dog){
Dog four=(Dog)two;
four.eat();
four.sleep();
System.out.println("我是Dog~~");
}
if(two instanceof Animal){
Animal four=(Animal)two;
four.eat();
//注意这里实际上调用的还是Cat类的eat
System.out.println("我是Animal~~");
}
if(two instanceof Object){
Object four=(Object)two;
System.out.println("我是Object~~");
}
}
}
输出结果
动物可以吃东西。
小猫会吃鱼
小猫正在跑。
我是Cat~~
动物可以吃东西。
小猫会吃鱼
我是Animal~~
我是Object~~