今天总结一下redis和spring集合的总结一下
开始添加一下maven的jar包
经过很多次的测试这个版本spring和redis的兼容性很好
(其他的版本很多有兼容性的问题)
<!--Redis start -->
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-redis</artifactId>
<version>1.6.1.RELEASE</version>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>2.7.3</version>
</dependency>
<!--Redis end -->
redis.properties基本信息的配置
# Redis settings
redis.host=47.94.**.**
redis.port=6379
redis.password=******
redis.maxIdle=300
redis.maxWait=1000
redis.testOnBorrow=true
redis.timeout=300
接下来就是applicationContext.XML的配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx" xmlns:jdbc="http://www.springframework.org/schema/jdbc"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc-3.0.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.0.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<context:annotation-config />
<context:component-scan base-package="com.fuwei.service" />
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource" init-method="init" destroy-method="close">
<!-- 基本属性 url、user、password -->
<property name="url" value="jdbc:mysql://localhost:3306/task_four?useUnicode=true&characterEncoding=utf-8" />
<property name="username" value="root" />
<property name="password" value="666666"/>
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<!-- 配置初始化大小、最小、最大 -->
<property name="initialSize" value="3" />
<property name="minIdle" value="3" />
<property name="maxActive" value="20" />
<!-- 配置获取连接等待超时的时间 -->
<property name="maxWait" value="60000" />
<!-- 配置间隔多久才进行一次检测,检测需要关闭的空闲连接,单位是毫秒 -->
<property name="timeBetweenEvictionRunsMillis" value="60000" />
<!-- 配置一个连接在池中最小生存的时间,单位是毫秒 -->
<property name="minEvictableIdleTimeMillis" value="300000" />
<property name="validationQuery" value="SELECT 1" />
<property name="testWhileIdle" value="true" />
<property name="testOnBorrow" value="false" />
<property name="testOnReturn" value="false" />
<!-- 打开PSCache,并且指定每个连接上PSCache的大小 -->
<property name="poolPreparedStatements" value="true" />
<property name="maxPoolPreparedStatementPerConnectionSize" value="20" />
</bean>
<!-- jedis 配置 -->
<bean id="poolConfig" class="redis.clients.jedis.JedisPoolConfig" >
<property name="maxIdle" value="${redis.maxIdle}" />
<property name="maxWaitMillis" value="${redis.maxWait}" />
<property name="testOnBorrow" value="${redis.testOnBorrow}" />
</bean >
<context:property-placeholder location="classpath:redis.properties" />
<!-- redis服务器中心 -->
<bean id="connectionFactory" class="org.springframework.data.redis.connection.jedis.JedisConnectionFactory" >
<property name="poolConfig" ref="poolConfig" />
<property name="port" value="${redis.port}" />
<property name="hostName" value="${redis.host}" />
<property name="password" value="${redis.password}" />
<property name="timeout" value="${redis.timeout}" ></property>
</bean >
<bean id="redisTemplate" class="org.springframework.data.redis.core.RedisTemplate" >
<property name="connectionFactory" ref="connectionFactory" />
<property name="keySerializer" >
<bean class="org.springframework.data.redis.serializer.StringRedisSerializer" />
</property>
<property name="valueSerializer" >
<bean class="org.springframework.data.redis.serializer.JdkSerializationRedisSerializer" />
</property>
</bean >
<!-- cache配置 -->
<bean id="redisUtil" class="com.fuwei.util.RedisUtil" >
<property name="redisTemplate" ref="redisTemplate" />
</bean >
<!-- spring自己的缓存管理器,这里定义了缓存位置名称 ,即注解中的value -->
<!-- <bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource"> -->
<!-- <property name="driverClassName"> -->
<!-- <value>com.mysql.jdbc.Driver</value> -->
<!-- </property> -->
<!-- <property name="url"> -->
<!-- <value>jdbc:mysql://localhost:3306/fuwei?characterEncoding=UTF-8</value> -->
<!-- </property> -->
<!-- <property name="username"> -->
<!-- <value>root</value> -->
<!-- </property> -->
<!-- <property name="password"> -->
<!-- <value>admin</value> -->
<!-- </property> -->
<!-- </bean> -->
<bean id="sqlSession" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="typeAliasesPackage" value="com.fuwei.pojo" />
<property name="dataSource" ref="dataSource"/>
<property name="mapperLocations" value="classpath:com/fuwei/mapper/*.xml"/>
</bean>
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.fuwei.mapper"/>
</bean>
</beans>
接下来就是RedisUtil类的编写
package com.fuwei.util;
import org.apache.log4j.Logger;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import java.io.Serializable;
import java.util.List;
import java.util.Set;
import java.util.concurrent.TimeUnit;
/**
* Redis工具类
*/
public class RedisUtil {
private RedisTemplate<Serializable, List> redisTemplate;
/**
* 批量删除对应的value
* @param keys
*/
public void remove(final String... keys) {
for (String key : keys) {
remove(key);
}
}
/**
* 批量删除key
* @param pattern
*/
public void removePattern(final String pattern) {
Set<Serializable> keys = redisTemplate.keys(pattern);
if (keys.size() > 0)
redisTemplate.delete(keys);
}
/**
* 删除对应的value
* @param key
*/
public void remove(final String key) {
if (exists(key)) {
redisTemplate.delete(key);
}
}
/**
* 判断缓存中是否有对应的value
* @param key
* @return
*/
public boolean exists(final String key) {
return redisTemplate.hasKey(key);
}
/**
* 读取缓存
* @param key
* @return
*/
public Object get(final String key) {
Object result = null;
ValueOperations<Serializable, List> operations = redisTemplate
.opsForValue();
result = operations.get(key);
return result;
}
/**
* 写入缓存
* @param key
* @param value
* @return
*/
public boolean set(final String key, List value) {
boolean result = false;
try {
ValueOperations<Serializable, List> operations = redisTemplate
.opsForValue();
operations.set(key, value);
result = true;
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
/**
* 写入缓存
* @param key
* @param value
* @return
*/
public boolean set(final String key, List value, Long expireTime) {
boolean result = false;
try {
ValueOperations<Serializable, List> operations = redisTemplate
.opsForValue();
operations.set(key, value);
redisTemplate.expire(key, expireTime, TimeUnit.SECONDS);
result = true;
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
public void setRedisTemplate(
RedisTemplate<Serializable, List> redisTemplate) {
this.redisTemplate = redisTemplate;
}
}
接下来就是查询的连接层的调用类
package com.fuwei.service.impl;
import com.fuwei.mapper.StudentMapper;
import com.fuwei.pojo.Student;
import com.fuwei.service.StudentService;
import com.fuwei.util.MemcachedUtil;
import com.fuwei.util.RedisUtil;
import com.google.gson.Gson;
import org.apache.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.Cache;
import org.springframework.stereotype.Service;
import org.springframework.test.context.ContextConfiguration;
import java.io.UnsupportedEncodingException;
import java.util.List;
@Service
/*@ContextConfiguration("classpath:memcachedContext.xml")*/ //memcached的缓存
@ContextConfiguration("classpath:applicationContext.xml") //redis的缓存
public class StudentServiceImpl implements StudentService {
private Logger logger=Logger.getLogger(StudentServiceImpl.class);
@Autowired
StudentMapper studentMapper;
@Autowired
RedisUtil redisUtil;
/*public List<Student> list(){
*//*List<Student> ls=studentMapper.list();
MemcachedUtil.put("Student", ls, 60);
Object obj = MemcachedUtil.get("Student".toString() );
System.out.println(obj);*//*
return studentMapper.list();
}*/
public List<Student> list1() {
List<Student> students;
if(redisUtil.exists("student2")){
students= (List<Student>) redisUtil.get("student2");
http://logger.info("从缓存里面取移动端"+students+"-----+----");
System.out.println("从缓存里面取移动端"+students+"-----+----");
return students;
}else {
students=studentMapper.list();
redisUtil.set("student2",students);
System.out.println("存储在缓存"+students+"123-----+----");
return students;
}
}
@Override
public int count(int state) {
return studentMapper.count(state);
}
}
redis的spring的流程基本就是这个样子
还有前面要测试你的Redis的端口的开放
简单的java的测试的类的编写
package com.fuwei.test;
import org.junit.Test;
import redis.clients.jedis.Jedis;
public class RedisStringJava {
@Test
public void it() {
//连接本地的 Redis 服务
Jedis jedis = new Jedis("localhost",6379);
jedis.auth("666666");
System.out.println("连接成功");
//设置 redis 字符串数据
jedis.set("A", "好的");
// 获取存储的数据并输出
System.out.println("redis 存储的字符串为: "+ jedis.getrange("student2",27,1000));
System.out.println("redis 存储的字符串为: "+ jedis.ping());
}
}
http://blog.csdn.net/fly43108622/article/details/52972433(参考文档)
还有关闭一次redis它就会被保护起来(这个是测试的时候遇到的问题)
-DENIED Redis is running in protected modebecause protected mode is enabled, no bind address was specified, noauthentication password is requested to clients. (这个问题的出现)
在路径
/usr/redis-4.0.8# 下面
127.0.0.1:6379> config set protected-mode "no" (设置不保护)
还有重新设置一下登录的密码(redis)
47.94.14.145:6379> config set requirepass 666666 (666666是密码)
登录redis
47.94.14.145:6379> auth 666666
查看全部的keys
47.94.14.145:6379> keys *
查看具体的那个key的值
47.94.14.145:6379> get student2
查找展示
47.94.14.145:6379> get student2
java.util.ArrayListxНơIsizexpwsrcom.fuwei.pojo.StudentW¦/:¡-ƑIiddescribetLjava/lang/String;Lnameq~passwordq~Lpictureq~Lstateq~xpt[修真院: 散修师弟爱好多,艾斯比很大声能达到把时间的不能杀菌灯吧.t 吴彦祖t123t
242424.pngt1sq~tLjava:安徽省大V氨基酸的啊是的八九十蛋宝宝加不上撒娇大.t 谢霆锋t123tbad.pngt2sq~tMphp:吧圣诞节啊就好撒比大家都就家斯巴达卡视角打开不卡.t美女t111t weman.pngt1sq~tKjava:可视电话福克斯比较烦看是否开始看独守空房了思考.t付伟t111good.pngt1x
这个redis与spring的整合总结完成了
还有前面的乱码的问题也一同解决啦
//filter编码设置
<filter>
<filter-name>CharacterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>Encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharacterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
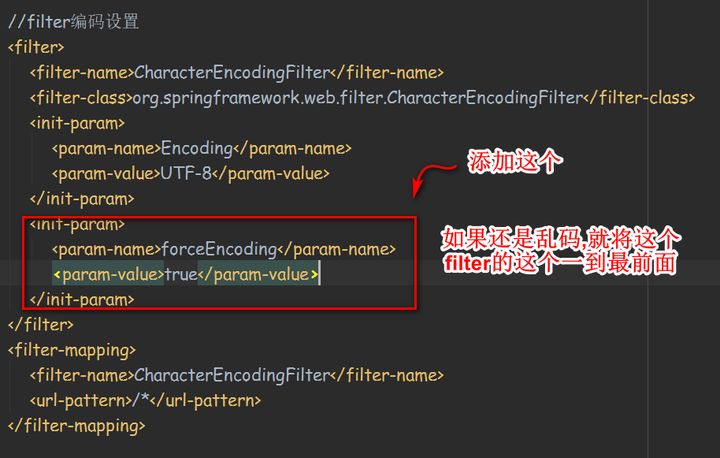