自己从事前端开发5年了,对于原生js目前已经消除不少恐惧,这也跟一直在做项目,一直在使用有很大的关系,但是,我觉得很大程度上是先学了jquery的原因,之前看《锋利的jquery》一书,造就了我当前的js水平,最开始学也是17/18年的时候吧,目前时间充裕了,又重新回顾了一下jquery,发现还是有一定收获的。
先将我整理的jquery事件的知识点发出来,方便大家自己以后学习,好记性不如赖笔头!
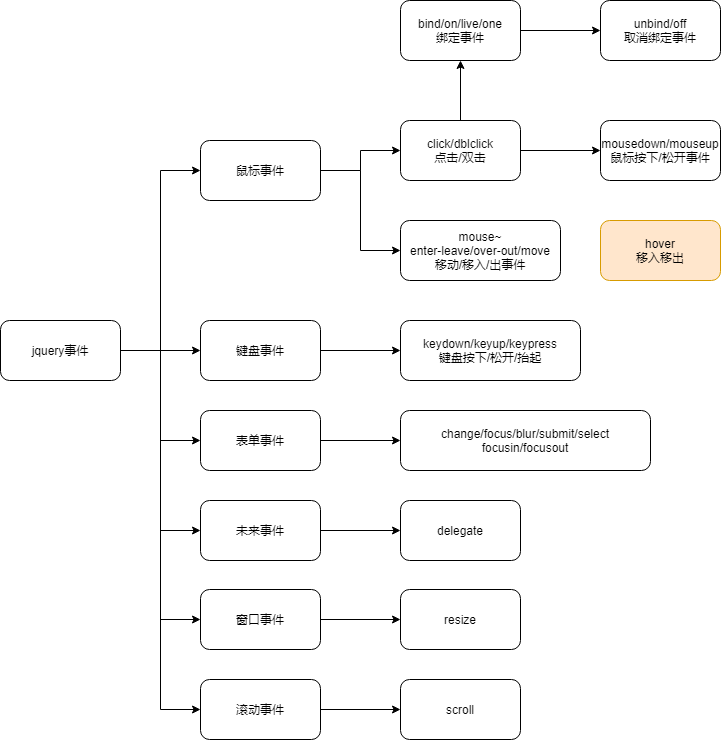
1.绑定事件:on/bind,live在1.9版本移除
$(".animate").bind("click",function(){ //等同于$(".animate").click()...
$(".box").animate({width:"100%"},3000)
})
$(".stop").on("click",function(){ //单击事件
$(".box").stop()
})
$(".hide").live("click",function(){ //live在1.9版后移除,不推荐
$(".box").hide(500,function(){ //函数回调callback
alert("隐藏了")
})
})
2.取消绑定事件:off/unbind
$(".offs").click(function(){
$(".stop").off("click") //取消动画停止按钮的绑定事件
})
$(".unbind").click(function(){
$(".stop").unbind() //解除所有box的事件句柄
})
3.点击事件:click/dblclick
$(".offs").click(function(){ // 点击事件
$(".stop").off("click")
})
$(".dbclick").dblclick(function(){ //双击事件
alert("双击了一下")
})
4.输入框input事件 change,foucs,blur,select,submit - from
$("#change").change(function(){ //监听输入框的值变化
alert("输入框改变了")
})
$("#change").select(function(){ //监听输入框内容被选择
alert("已选择")
})
$("#change").focus(function(){ //监听输入框被激活
alert("哇,激活了")
})
$("#change").blur(function(){ //监听输入框失去焦点时
alert("输入框失去了焦点")
})
//键盘事件:keydown - 键按下的过程 keypress - 键被按下 keyup - 键被松开
$("#change").keydown(function(event){ //监听输入时,键盘按下
console.log(event.which) //event.which 返回某个按键按下
})
//submit,表单提交事件
$("form").submit(function(){
alert("submit事件只能绑定到form身上")
})
5.鼠标事件:hover...
//hover鼠标移入移出事件,相当于mouseenter和mouseleaver
$(".stopgo").hover(function(){
alert("123")
},function(){
alert("456")
})
//mouseenter 鼠标移入事件
$(".mover").mouseenter(function(){
alert("abc")
})
//mouseleave 鼠标移出事件
$(".mover").mouseleave(function(){
alert("def")
})
//mouseover类似于mouseenter,移入事件,子元素也会触发
$(".mover360").mouseover(function(){
alert("AAA")
})
//mouseout类似于mouseleave,移出事件,子元素也会触发
$(".mover360").mouseout(function(){
alert("bbb")
})
//mousemove 鼠标移动事件
$(".contain").mousemove(function(evevt){
//event.pageX,event.pageY鼠标指针所在位置 $(this).children("span").text("x:"+event.pageX+",y:"+event.pageY)
console.log(event.type) //返回事件类型
//console.log(event.relatedTarget.nodeName) //event.relatedTarget属性返回当鼠标移动时哪个元素进入或退出
})
6.鼠标左键点击分解事件:mouseup - 释放鼠标左键 mousedown - 按下鼠标左键
$("body").mousedown(function(e){
console.log("按下了鼠标左键")
if(e.which == 3){
alert("鼠标点击了右键");
}else if(e.which == 2){
alert("鼠标点击了中键");
}else if(e.which == 1){
alert("鼠标点击了左键");
}
})
$("body").mouseup(function(){
console.log("释放了鼠标左键")
})
7.鼠标右键点击,可以用来网页禁止右键,或者自定义右键
//触发鼠标右键
$("body").contextmenu(function() {
alert( "处理程序.contextmenu()被调用。" );
});
8.阻止冒泡:event.stopPropagation(),阻止元素默认行为:event.preventDefault()
$(".stopgo").on("click",function(event){
event.preventDefault() //阻止a默认跳转行为
})
$(".box2 p").bind("click",function(event){
event.stopPropagation()
})
9.委托-模拟点击/经过事件方法:delegate(),undelegate 移除委托事件
$("#box").delegate("p","click",function(){ //用于动态加入代码的时候,点击代码的元素,可以用委托事件
alert("未来元素事件");
})
//undelegate 移除委托事件
$("body").click(function(){
$("#box p").undelegate();
})
10.one 事件仅生效一次
$(".scale").one("click",function(){
$(this).css("font-size","20px")
})
11.trigger 模拟事件触发
$(".scale").one("click",function(){
$("#box1 p").trigger("click") //模拟p元素被点击,事实上我没有并没点击,但是通过trigger事件确实触发了
})
12.窗口监听:resize,滚动事件scroll
//窗口大小改变时触发
var i = j = 0
$(window).resize(function(){
i++
console.log("窗口变化了"+ i +"次")
})
//页面滚动,元素 内部滚动时触发
$(window).scroll(function(){
j++
console.log("页面滚动了"+ j +"次")
})