还是第一次调用此类型接口,还是基于soap模式,因此比较麻烦,不像http接口那样,可以使用第三方工具
调用代码如下:
package com.donlim.fms.common.utils;
import lombok.extern.slf4j.Slf4j;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.w3c.dom.Document;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.sql.Date;
import java.text.SimpleDateFormat;
/**
* @ClassName MessageSync
* @Description 消息同步调用函数
* <p>
* Version 1.0
**/
@Slf4j
public class MessageSync {
private static Logger logger = LoggerFactory.getLogger(MessageSync.class);
private static String destinationApp = "EIP";
private static String sourceApp = "FMS";
private static String systemSort = "A12";
private static String systemName = "FMS";
private static String ipAddress = "****";
private static String BO = "待办通知信息同步";
public static int ESBMailBase(String mailID, String billMan, String billUrl,
String mailSubject, String mailBody, int type, String createMan, String mailType
) {
int flag = 0;
try {
String operateType = "";
if (type == 0) {
operateType = "ADD";
} else if (type == 1) {
operateType = "UPDATE";
} else if (type == 2) {
operateType = "DELETE";
}
Date date = new Date(System.currentTimeMillis());
String createDate = dateToStr(date);
//第一步:创建服务地址
URL url = new URL("http://**********:7080/WS/DONLIM/ES/AGENCYNOTICEINFOSYNC?wsdl");
//第二步:打开一个通向服务地址的连接
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
//第三步:设置参数
//3.1发送方式设置:POST必须大写
connection.setRequestMethod("POST");
//3.2设置数据格式:content-type
connection.setRequestProperty("content-type", "text/xml;charset=utf-8");
//3.3设置输入输出,因为默认新创建的connection没有读写权限,
connection.setDoInput(true);
connection.setDoOutput(true);
//第四步:组织SOAP数据,发送请求
String soapXML = getMessageSyncXml(mailID, billMan, billUrl, mailSubject, mailBody, operateType, createDate, createMan, mailType);
// 将信息以流的方式发送出去
OutputStream os = connection.getOutputStream();
os.write(soapXML.getBytes("UTF-8"));
//第五步:接收服务端响应,打印
int responseCode = connection.getResponseCode();
System.err.println(responseCode);
if (200 == responseCode) {
// 获取当前连接请求返回的数据流
InputStream is = connection.getInputStream();
InputStreamReader isr = new InputStreamReader(is);
BufferedReader br = new BufferedReader(isr);
StringBuilder sb = new StringBuilder();
String temp = null;
while (null != (temp = br.readLine())) {
sb.append(temp);
}
//打印结果
System.err.println(sb.toString());
if (sb.toString().contains("<RCODE>Y</RCODE><ESBCODE>S</ESBCODE>")){
flag = 1;
}
// if (sb.toString().length() == 402 || sb.toString().length() == 406 || sb.toString().length() == 407) {
// flag = 1;
// }
is.close();
isr.close();
br.close();
}
os.close();
} catch (Exception e) {
log.debug("发送消息失败{}",e.getMessage());
}
return flag;
}
public static String getMessageSyncXml(String mailID, String billMan, String billUrl,
String mailSubject, String mailBody, String type, String createDate, String createMan, String mailType) {
StringBuilder str = new StringBuilder("<?xml version=\"1.0\" encoding=\"UTF-8\" standalone=\"yes\" ?>");
str.append("<soapenv:Envelope xmlns:soapenv='http://schemas.xmlsoap.org/soap/envelope/'");
str.append(" xmlns:don='http://www.example.org/DONLIM_ES_AGENCYNOTICEINFOSYNC_086/'>");
str.append("<soapenv:Header/>");
str.append("<soapenv:Body>");
str.append("<don:DONLIM_ES_AGENCYNOTICEINFOSYNC_086>");
str.append("<SvcHdr>");
str.append("<SOURCEID>").append(sourceApp).append("</SOURCEID>");
str.append("<DESTINATIONID>").append(destinationApp).append("</DESTINATIONID>");
str.append("<TYPE>").append(type).append("</TYPE>");
str.append("<IPADDRESS>").append(ipAddress).append("</IPADDRESS>");
str.append("<BO>1</BO>");
str.append("</SvcHdr>");
str.append("<AppBody>");
str.append("<AddNotice>");
str.append("<Account>").append(billMan).append("</Account>");
str.append("<CreateDate>").append(createDate).append("</CreateDate>");
str.append("<CreateMan>").append(createMan).append("</CreateMan>");
str.append("<MailBody>").append(mailBody).append("</MailBody>");
str.append("<MailID>").append(mailID).append("</MailID>");
str.append("<MailSubject>").append(mailSubject).append("</MailSubject>");
str.append("<MailType>").append(mailType).append("</MailType>");
str.append("<SystemName>").append(systemName).append("</SystemName>");
str.append("<SystemSort>").append(systemSort).append("</SystemSort>");
str.append("<Url>").append(billUrl).append("</Url>");
str.append("</AddNotice>");
str.append("</AppBody>");
str.append("</don:DONLIM_ES_AGENCYNOTICEINFOSYNC_086>");
str.append("</soapenv:Body>");
str.append("</soapenv:Envelope>");
return str.toString();
}
public static String dateToStr(Date dateDate) {
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss");
String dateString = formatter.format(dateDate);
return dateString;
}
}
返回结果说明:<RCODE>Y</RCODE><ESBCODE>S</ESBCODE>
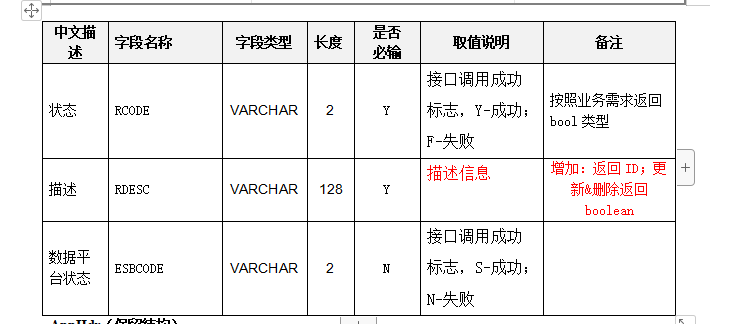
调用代码:
MessageSync.ESBMailBase("FMS" + number, approve_id,
"192.1**.*.**:***", "FMS待审单,单号:" + number + ",请登录到FMS系统处理", "",
0, "", "待办");