复数类重载
#include<iostream>
using namespace std;
class CComplex
{
public:
CComplex (int r=0, int i=0)
:_real(r)
, _vir(i)
{
}
CComplex operator+(const CComplex& com)
{
return CComplex(this->_real + com._real, this->_vir + com._vir);
}
CComplex& operator++()
{
_real += 1;
_vir += 1;
return *this;
}
CComplex operator++(int)
{
return CComplex(_real++, _vir++);
}
void operator+=(const CComplex& src)
{
_real += src._real;
_vir += src._vir;
}
void show()
{
cout << "this" << this << endl;
cout << "real:" << _real << endl;
cout << "vir:" << _vir << endl;
}
private:
int _real;
int _vir;
friend CComplex operator+ (const CComplex& lhs, const CComplex& rhs);
friend ostream& operator<<(ostream& out, const CComplex& src);3
friend istream& operator>>(istream &in, CComplex &srx);
};
CComplex operator+ (const CComplex &lhs,const CComplex &rhs)
{
return CComplex(lhs._real + rhs._real, lhs._vir + rhs._vir);
}
ostream& operator<<(ostream& out, const CComplex& src)
{
out << "_real" << src._real << "_vir" << src._vir << endl;
return out;
}
istream& operator>>(istream &in, CComplex &srx)
{
in >> src._real>>src._vir;
return in;
}
int main()
{
CComplex comp1(10, 10);
CComplex comp2(20, 20);
CComplex comp3 = comp1 + comp2;
comp3.show();
CComplex comp4 = comp1 + 20;
comp4.show();
CComplex comp5 = 30 + comp2;
comp5.show();
comp5 = comp1++;
comp5.show();
comp1.show();
comp5 = ++comp1;
comp5.show();
comp1.show();
comp1 += comp2;
comp1.show();
return 0;
}
template<typename T>
void show(T a)
{
cout << a << endl;
}
模拟C++String类代码
#include<iostream>
#include<string>
using namespace std;
class String
{
public:
String(const char* p = nullptr)
{
if (p != nullptr)
{
_pstr = new char[strlen(p) + 1];
strcpy(_pstr, p);
}
else
{
_pstr = new char[1];
*_pstr = '\0';
}
}
~String()
{
delete[] _pstr;
_pstr = nullptr;
}
String(const String& src)
{
_pstr = new char[strlen(src._pstr) + 1];
strcpy(_pstr, src._pstr);
}
String& operator=(const String& src)
{
if (this == &src)
{
return *this;
}
delete[] _pstr;
_pstr = new char[strlen(src._pstr) + 1];
strcpy(_pstr, src._pstr);
return *this;
}
bool operator>(const String& str) const
{
return strcmp(_pstr, str._pstr) > 0;
}
bool operator<(const String& str) const
{
return strcmp(_pstr, str._pstr) < 0;
}
bool operator==(const String& str) const
{
return strcmp(_pstr, str._pstr) == 0;
}
int length()const
{
return strlen(_pstr);
}
char& operator[](int index)
{
return _pstr[index];
}
const char& operator[](int index)const
{
return _pstr[index];
}
const char* c_str()const
{
return _pstr;
}
private:
char* _pstr;
friend String operator+(const String& lhs, const String& rhs);
friend ostream& operator<<(ostream& out, const String& str);
};
String operator+(const String& lhs, const String& rhs)
{
String tmp;
tmp._pstr = new char[strlen(lhs._pstr) + strlen(rhs._pstr) + 1];
strcpy(tmp._pstr, lhs._pstr);
strcat(tmp._pstr, rhs._pstr);
return tmp;
}
ostream& operator<<(ostream& out, const String& str)
{
out << str._pstr;
return out;
}
int main()
{
String str1;
String str2 = "aaa";
String str3 = "bbb";
String str4 = str2 + str3;
String str5 = str2 + "ccc";
String str6 = "ddd" + str2;
cout << "str6:" << str6 << endl;
if (str5 > str6)
{
cout << str5 << " > " << str6 << endl;
}
else
{
cout << str5 << " < " << str6 << endl;
}
int len = str6.length();
for (int i = 0; i < len; ++i)
{
cout << str6[i] << " ";
}
char buf[1024] = { 0 };
strcpy(buf, str6.c_str());
return 0;
}
对象迭代器iterator
String
#include<iostream>
#include<string>
using namespace std;
class String
{
public:
String(const char* p = nullptr)
{
if (p != nullptr)
{
_pstr = new char[strlen(p) + 1];
strcpy(_pstr, p);
}
else
{
_pstr = new char[1];
*_pstr = '\0';
}
}
~String()
{
delete[] _pstr;
_pstr = nullptr;
}
String(const String& src)
{
_pstr = new char[strlen(src._pstr) + 1];
strcpy(_pstr, src._pstr);
}
String& operator=(const String& src)
{
if (this == &src)
{
return *this;
}
delete[] _pstr;
_pstr = new char[strlen(src._pstr) + 1];
strcpy(_pstr, src._pstr);
return *this;
}
bool operator>(const String& str) const
{
return strcmp(_pstr, str._pstr) > 0;
}
bool operator<(const String& str) const
{
return strcmp(_pstr, str._pstr) < 0;
}
bool operator==(const String& str) const
{
return strcmp(_pstr, str._pstr) == 0;
}
int length()const
{
return strlen(_pstr);
}
char& operator[](int index)
{
return _pstr[index];
}
const char& operator[](int index)const
{
return _pstr[index];
}
const char* c_str()const
{
return _pstr;
}
class iterator
{
public:
iterator(char* p = nullptr)
:_p(p)
{
}
bool operator!=(const iterator& it)
{
return _p != it._p;
}
void operator++()
{
++_p;
}
char& operator*()
{
return *_p;
}
private:
char* _p;
};
iterator begin()
{
return iterator(_pstr);
}
iterator end()
{
return iterator(_pstr+length());
}
private:
char* _pstr;
friend String operator+(const String& lhs, const String& rhs);
friend ostream& operator<<(ostream& out, const String& str);
};
String operator+(const String& lhs, const String& rhs)
{
String tmp;
tmp._pstr = new char[strlen(lhs._pstr) + strlen(rhs._pstr) + 1];
strcpy(tmp._pstr, lhs._pstr);
strcat(tmp._pstr, rhs._pstr);
return tmp;
}
ostream& operator<<(ostream& out, const String& str)
{
out << str._pstr;
return out;
}
int main()
{
string str1 = "hellow world!";
auto it = str1.begin();
for (; it != str1.end(); ++it)
{
cout << *it << " ";
}
cout << endl;
cout << str1 << endl;
for (char ch : str1)
{
cout << ch;
}
return 0;
}
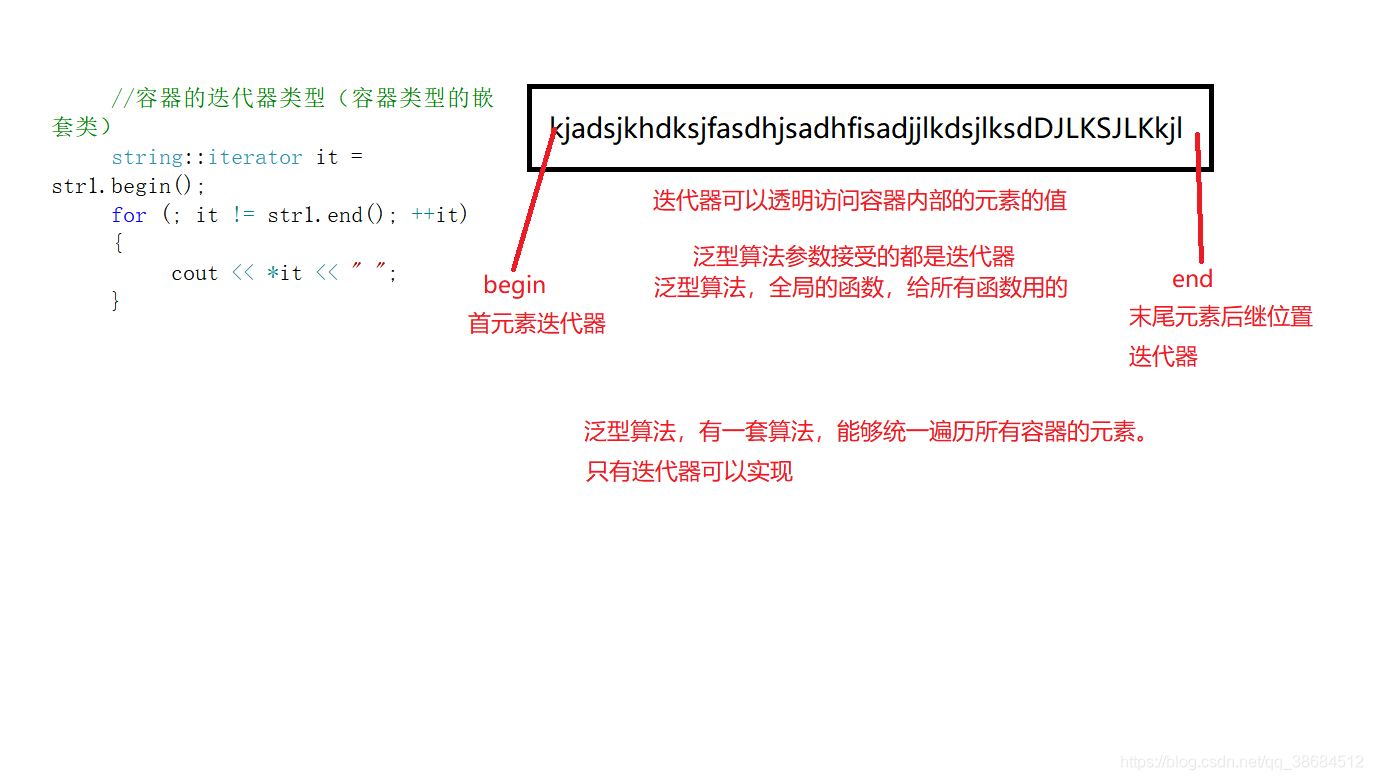
vector
#include<iostream>
using namespace std;
template<typename T>
class Allocator
{
public:
Allocator() {}
~Allocator() {}
T* allocate(size_t size)
{
return (T*)malloc(sizeof(T) * size);
}
void deallocate(void* p)
{
free(p);
}
void construct(T* p, const T& val)
{
new(p)T(val);
}
void destory(T* p)
{
p->~T();
}
private:
};
template<typename T,typename Alloc = Allocator<T>>
class vector
{
public:
vector(T size = 10, const Alloc& alloc = Allocator<T>())
:m_alloctor(alloc)
{
m_first = m_alloctor.allocate(10);
m_last = m_first;
m_endl = m_first + size;
}
~vector()
{
for (T* p = m_first; p != m_last; ++p)
{
m_alloctor.destory(p);
}
m_alloctor.deallocate(m_first);
m_first = m_last = m_endl = nullptr;
}
vector(const vector<T>& val)
{
int len = val.m_last - val.m_first;
int size = val.m_endl - val.m_first;
m_first = m_alloctor.allocate(size);
for (int i = 0; i < size; i)
{
m_alloctor.construct(m_first + i, val.m_first + i);
}
m_last = m_first + len;
m_endl = m_first + size;
}
vector<T>& operator=(const vector<T>& val)
{
if (this == &val)
{
return *this;
}
for (T* p = m_last; p != m_first; --p)
{
m_alloctor.destroy(p);
}
m_alloctor.deallocate(m_first);
int len = val.m_last - val.m_first;
int size = val.m_endl - val.m_first;
m_first = m_alloctor.allocate(size);
for (int i = 0; i < size; ++i)
{
m_alloctor.construct(m_first + i, val.m_first + i);
}
m_last = m_first + len;
m_endl = m_first + size;
}
void push_back(const T& val)
{
if (full())
{
expand();
}
m_alloctor.construct(m_last, val);
cout << "Push back:" << m_last << endl;
m_last++;
}
void pop_back()
{
if (empty())
{
return;
}
m_last--;
m_alloctor.destroy(m_last);
}
T back() const
{
return *(m_last - 1);
}
bool full() const { return m_last == m_endl; }
bool empty() const { return m_last == m_first; }
T& operator[](int index)
{
if (index < 0 || index >= (m_last-m_first))
{
throw"OutOfRangeException";
}
return m_first[index];
}
class iterator
{
public:
iterator(T* ptr = nullptr)
:_ptr(ptr)
{
}
bool operator!=(const iterator& it)
{
return _ptr != it._ptr;
}
void operator++()
{
_ptr++;
}
T& operator*()
{
return *_ptr;
}
const T& operator*()const
{
return *_ptr;
}
private:
T* _ptr;
};
iterator begin() { return iterator(m_first); }
iterator end() { return iterator(m_last); }
private:
T* m_first;
T* m_last;
T* m_endl;
Alloc m_alloctor;
void expand()
{
int size = m_endl - m_first;
T* ptmp = m_alloctor.allocate(2 * size);
for (int i = 0; i < size; ++i)
{
m_alloctor.construct(ptmp + i, m_first[i]);
}
for (T* p = m_first; p != m_last; ++p)
{
m_alloctor.destory(p);
}
m_alloctor.deallocate(m_first);
m_first = ptmp;
m_last = m_first + size;
m_endl = m_first + 2 * size;
}
};
int main()
{
vector<int> vec;
for (int i = 0; i < 20; ++i)
{
vec.push_back(rand() % 100 + 1);
}
vector<int>::iterator it = vec.begin();
for (; it != vec.end(); ++it)
{
cout << *it << endl;
}
for (auto a : vec)
{
cout << a<< endl;
}
for (int i = 0; i < 20; ++i)
{
cout << vec[i] << endl;
}
cout << endl;
return 0;
}
迭代器失效问题
#include<iostream>
#include<vector>
using namespace std;
int main()
{
vector<int> vec;
for (int i = 0; i < 20; ++i)
{
vec.push_back(rand() % 100 + 1);
}
for (int v : vec)
{
cout << v<<" ";
}
cout << endl;
auto it = vec.begin();
for (; it != vec.end();)
{
if (*it % 2 == 0)
{
it = vec.erase(it);
}
else
{
++it;
}
}
for (int v : vec)
{
cout << v<<" ";
}
cout << endl;
}
在vector中寻找迭代器失效的原理