常用图示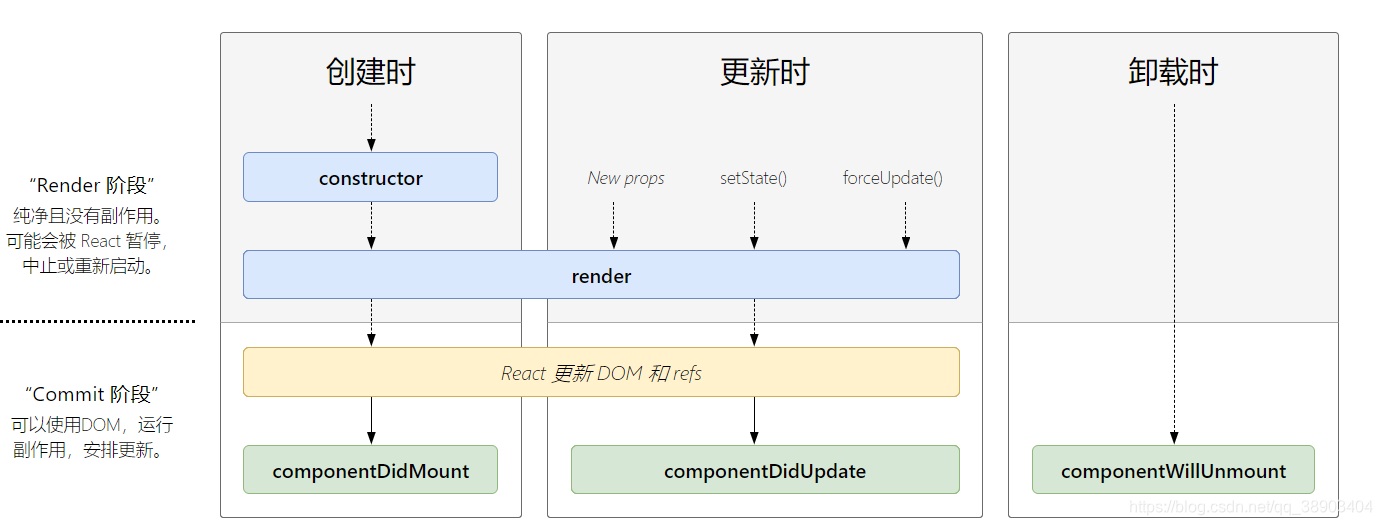
完整图示
React
组件的生命周期可以分为3种状态。
- 创建时
- 更新时
- 卸载时
import React, { Component } from 'react'
/*
生命周期函数
*/
export default class App extends Component {
state = {
num: 0
}
constructor(props) {
super(props)
console.log("1.进入到构造函数中");
/*
a. super(props) 调用父类的构造方法
b. 初始化 state this.state={}
c. 修正this指向 this.handleClick = this.handleClick.bind(this)
*/
}
static getDerivedStateFromProps() {
// a props和state的同步
// b 再调用render方法之前调用,并且在初始化挂载及后续更新时都会调用
// 返回的是更新的state ,返回null 表示不更新任何内容
console.log("2. 进入到 getDerivedStateFromProps");
return null
}
handelClick = () => {
this.setState({ num: this.state.num + 1 })
}
render() {
/*
1. 返回dom react元素
2. 不能在这里 修改状态!!! 会造成死循环
3. class组件中必须实现的方法
*/
console.log("3. 进入到Render函数中...");
return (
<div>
<h1>声明周期函数</h1>
<h2>{this.state.num}</h2>
<button onClick={this.handelClick}>add</button>
</div>
)
}
componentDidMount() {
/*
在组件挂载后立即调用
1. 数据请求
2. 注册事件
3. 监听器
*/
console.log("4. 组件挂载后立即调用 componentDidMount");
}
componentDidUpdate() {
console.log("5. 数据更新后,立即调用,componentDidUpdate");
/*
不建议在这里修改state
在这里修改state时,必须放在一个条件语句里,否则会造成死循环
当`props`和`state`发生改变时会触发 shouldComponentUpdate,返回`true` 则表示允许执行 `render` ,返回 `false`这表示 不允许运行`render`
*/
}
componentWillUnmount() {
console.log("6. 在组件卸载及销毁之前调用");
/*
在这里可以执行必要的清除操作
1. 清除定时器 timer
2. 取消网络请求
3. 清除监听器
*/
}
}
页面创建时
页面第一次加载时,会触发:constructor、getDerivedStateFromProps、Render、componentDidMount
当state改变时
当state改变时,会触发:getDerivedStateFromProps、Render、componentDidUpdate