一.使用MVC开发的朋友都有一个苦恼,那就是三层结构大量重复代码比较鸡肋,写之无味,不写不行.最近也是从网上找了一些资料,自己又简单加工下,完善了该工具.
1.1 支持sqlserver 2012 2014 2016 2017等
1.2 代码目录结构如下
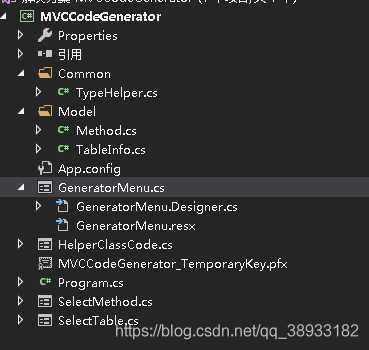
1.3 使用前,修改默认本地库 登录名,密码
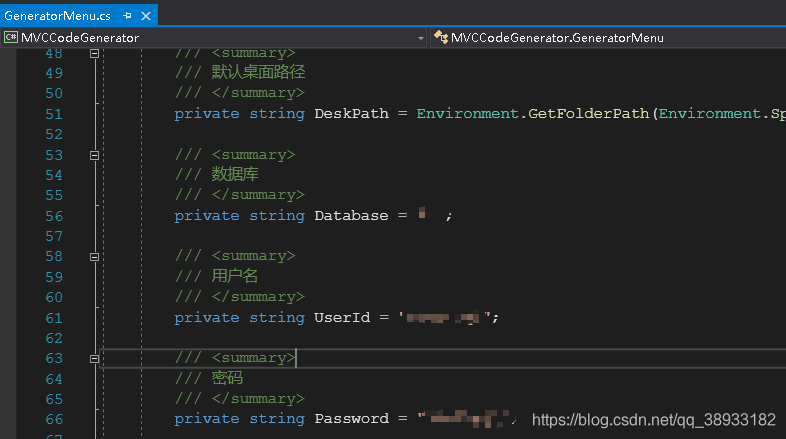
/// <summary>
/// 数据库
/// </summary>
private string Database = ".";
/// <summary>
/// 用户名
/// </summary>
private string UserId = "你想要登录数据库的登录名";
/// <summary>
/// 密码
/// </summary>
private string Password = "登录密码";
二.每个团队开发使用的框架都不一样,我这里写了一个小Demo,根据该Demo框架,生成对应的三层代码.这里把原始 ModelBase , DalBase 和 BllBase 代码贴出
2.1EntityModel , 对字段应用了反射,过滤器等相关知识作参考
/// <summary>
/// 实体类基类
/// </summary>
public class EntityModel
{
private const BindingFlags BindingFlag = BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.Instance;
//将类型与该类型所有的可写且未被忽略属性之间建立映射
private static Dictionary<Type, Dictionary<string, PropertyInfo>> propertyMappings = new Dictionary<Type, Dictionary<string, PropertyInfo>>();
//存储Nullable<T>与T的对应关系
private static Dictionary<Type, Type> genericTypeMappings = new Dictionary<Type, Type>();
static EntityModel()
{
genericTypeMappings.Add(typeof(Byte?), typeof(Byte));
genericTypeMappings.Add(typeof(SByte?), typeof(SByte));
genericTypeMappings.Add(typeof(Char?), typeof(Char));
genericTypeMappings.Add(typeof(Boolean?), typeof(Boolean));
genericTypeMappings.Add(typeof(Guid?), typeof(Guid));
genericTypeMappings.Add(typeof(Int16), typeof(Int16));
genericTypeMappings.Add(typeof(UInt16), typeof(UInt16));
genericTypeMappings.Add(typeof(Int32), typeof(Int32));
genericTypeMappings.Add(typeof(UInt32), typeof(UInt32));
genericTypeMappings.Add(typeof(Int64), typeof(Int64));
genericTypeMappings.Add(typeof(UInt64), typeof(UInt64));
genericTypeMappings.Add(typeof(Single), typeof(Single));
genericTypeMappings.Add(typeof(Double), typeof(Double));
genericTypeMappings.Add(typeof(Decimal), typeof(Decimal));
genericTypeMappings.Add(typeof(DateTime), typeof(DateTime));
genericTypeMappings.Add(typeof(TimeSpan), typeof(TimeSpan));
genericTypeMappings.Add(typeof(Enum), typeof(Enum));
}
/// <summary>
/// 将DataTable中的所有数据转换成List>T<集合
/// </summary>
/// <typeparam name="T">DataTable中每条数据可以转换的数据类型</typeparam>
/// <param name="dataTable">包含有可以转换成数据类型T的数据集合</param>
/// <returns></returns>
public static List<T> GetEntities<T>(DataTable dataTable) where T : new()
{
if (dataTable == null)
{
throw new ArgumentNullException("dataTable");
}
//如果T的类型满足以下条件:字符串、ValueType或者是Nullable<ValueType>
if (typeof(T) == typeof(string) || typeof(T).IsValueType)
{
return GetSimpleEntities<T>(dataTable);
}
else
{
return GetComplexEntities<T>(dataTable);
}
}
/// <summary>
/// 将DbDataReader中的所有数据转换成List>T<集合
/// </summary>
/// <typeparam name="T">DbDataReader中每条数据可以转换的数据类型</typeparam>
/// <param name="dataTable">包含有可以转换成数据类型T的DbDataReader实例</param>
/// <returns></returns>
public static List<T> GetEntities<T>(DbDataReader reader) where T : new()
{
List<T> list = new List<T>();
if (reader == null)
{
throw new ArgumentNullException("reader");
}
//如果T的类型满足以下条件:字符串、ValueType或者是Nullable<ValueType>
if (typeof(T) == typeof(string) || typeof(T).IsValueType)
{
return GetSimpleEntities<T>(reader);
}
else
{
return GetComplexEntities<T>(reader);
}
}
/// <summary>
/// 从DataTable中将每一行的第一列转换成T类型的数据
/// </summary>
/// <typeparam name="T">要转换的目标数据类型</typeparam>
/// <param name="dataTable">包含有可以转换成数据类型T的数据集合</param>
/// <returns></returns>
private static List<T> GetSimpleEntities<T>(DataTable dataTable) where T : new()
{
List<T> list = new List<T>();
foreach (DataRow row in dataTable.Rows)
{
list.Add((T)GetValueFromObject(row[0], typeof(T)));
}
return list;
}
/// <summary>
/// 将指定的 Object 的值转换为指定类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <param name="targetType">要转换的目标数据类型</param>
/// <returns></returns>
private static object GetValueFromObject(object value, Type targetType)
{
if (targetType == typeof(string))//如果要将value转换成string类型
{
return GetString(value);
}
else if (targetType.IsGenericType)//如果目标类型是泛型
{
return GetGenericValueFromObject(value, targetType);
}
else//如果是基本数据类型(包括数值类型、枚举和Guid)
{
return GetNonGenericValueFromObject(value, targetType);
}
}
/// <summary>
/// 从DataTable中读取复杂数据类型集合
/// </summary>
/// <typeparam name="T">要转换的目标数据类型</typeparam>
/// <param name="dataTable">包含有可以转换成数据类型T的数据集合</param>
/// <returns></returns>
private static List<T> GetComplexEntities<T>(DataTable dataTable) where T : new()
{
if (!propertyMappings.ContainsKey(typeof(T)))
{
GenerateTypePropertyMapping(typeof(T));
}
List<T> list = new List<T>();
Dictionary<string, PropertyInfo> properties = propertyMappings[typeof(T)];
//Dictionary<string, int> propertyColumnOrdinalMapping = GetPropertyColumnIndexMapping(dataTable.Columns, properties);
T t;
foreach (DataRow row in dataTable.Rows)
{
t = new T();
foreach (KeyValuePair<string, PropertyInfo> item in properties)
{
//int ordinal = -1;
//if (propertyColumnOrdinalMapping.TryGetValue(item.Key, out ordinal))
//{
// item.Value.SetValue(t, GetValueFromObject(row[ordinal], item.Value.PropertyType), null);
//}
item.Value.SetValue(t, GetValueFromObject(row[item.Key], item.Value.PropertyType), null);
}
list.Add(t);
}
return list;
}
/// <summary>
/// 从DbDataReader的实例中读取复杂的数据类型
/// </summary>
/// <typeparam name="T">要转换的目标类</typeparam>
/// <param name="reader">DbDataReader的实例</param>
/// <returns></returns>
private static List<T> GetComplexEntities<T>(DbDataReader reader) where T : new()
{
if (!propertyMappings.ContainsKey(typeof(T)))//检查当前是否已经有该类与类的可写属性之间的映射
{
GenerateTypePropertyMapping(typeof(T));
}
List<T> list = new List<T>();
Dictionary<string, PropertyInfo> properties = propertyMappings[typeof(T)];
//Dictionary<string, int> propertyColumnOrdinalMapping = GetPropertyColumnIndexMapping(reader, properties);
T t;
while (reader.Read())
{
t = new T();
foreach (KeyValuePair<string, PropertyInfo> item in properties)
{
//int ordinal = -1;
//if (propertyColumnOrdinalMapping.TryGetValue(item.Key, out ordinal))
//{
// item.Value.SetValue(t, GetValueFromObject(reader[ordinal], item.Value.PropertyType), null);
//}
item.Value.SetValue(t, GetValueFromObject(reader[item.Key], item.Value.PropertyType), null);
}
list.Add(t);
}
return list;
}
/// <summary>
/// 从DbDataReader的实例中读取简单数据类型(String,ValueType)
/// </summary>
/// <typeparam name="T">目标数据类型</typeparam>
/// <param name="reader">DbDataReader的实例</param>
/// <returns></returns>
private static List<T> GetSimpleEntities<T>(DbDataReader reader)
{
List<T> list = new List<T>();
while (reader.Read())
{
list.Add((T)GetValueFromObject(reader[0], typeof(T)));
}
return list;
}
/// <summary>
/// 将Object转换成字符串类型
/// </summary>
/// <param name="value">object类型的实例</param>
/// <returns></returns>
private static object GetString(object value)
{
return Convert.ToString(value);
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <param name="targetType"></param>
/// <returns></returns>
private static object GetEnum(object value, Type targetType)
{
return Enum.Parse(targetType, value.ToString());
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetBoolean(object value)
{
if (value is Boolean)
{
return value;
}
else
{
byte byteValue = (byte)GetByte(value);
if (byteValue == 0)
{
return false;
}
else
{
return true;
}
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetByte(object value)
{
if (value is Byte)
{
return value;
}
else
{
return byte.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetSByte(object value)
{
if (value is SByte)
{
return value;
}
else
{
return SByte.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetChar(object value)
{
if (value is Char)
{
return value;
}
else
{
return Char.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetGuid(object value)
{
if (value is Guid)
{
return value;
}
else
{
return new Guid(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetInt16(object value)
{
if (value is Int16)
{
return value;
}
else
{
return Int16.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetUInt16(object value)
{
if (value is UInt16)
{
return value;
}
else
{
return UInt16.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetInt32(object value)
{
if (value is Int32)
{
return value;
}
else
{
return Int32.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetUInt32(object value)
{
if (value is UInt32)
{
return value;
}
else
{
return UInt32.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetInt64(object value)
{
if (value is Int64)
{
return value;
}
else
{
return Int64.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetUInt64(object value)
{
if (value is UInt64)
{
return value;
}
else
{
return UInt64.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetSingle(object value)
{
if (value is Single)
{
return value;
}
else
{
return Single.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetDouble(object value)
{
if (value is Double)
{
return value;
}
else
{
return Double.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetDecimal(object value)
{
if (value is Decimal)
{
return value;
}
else
{
return Decimal.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetDateTime(object value)
{
if (value is DateTime)
{
return value;
}
else
{
return DateTime.Parse(value.ToString());
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定枚举类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <returns></returns>
private static object GetTimeSpan(object value)
{
if (value is TimeSpan)
{
return value;
}
else
{
return TimeSpan.Parse(value.ToString());
}
}
/// <summary>
/// 将Object类型数据转换成对应的可空数值类型表示
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <param name="targetType">可空数值类型</param>
/// <returns></returns>
private static object GetGenericValueFromObject(object value, Type targetType)
{
if (value == DBNull.Value)
{
return null;
}
else
{
//获取可空数值类型对应的基本数值类型,如int?->int,long?->long
Type nonGenericType = genericTypeMappings[targetType];
return GetNonGenericValueFromObject(value, nonGenericType);
}
}
/// <summary>
/// 将指定的 Object 的值转换为指定类型的值。
/// </summary>
/// <param name="value">实现 IConvertible 接口的 Object,或者为 null</param>
/// <param name="targetType">目标对象的类型</param>
/// <returns></returns>
private static object GetNonGenericValueFromObject(object value, Type targetType)
{
if (targetType.IsEnum)//因为
{
return GetEnum(value, targetType);
}
else
{
switch (targetType.Name)
{
case "Byte": return GetByte(value);
case "SByte": return GetSByte(value);
case "Char": return GetChar(value);
case "Boolean": return GetBoolean(value);
case "Guid": return GetGuid(value);
case "Int16": return GetInt16(value);
case "UInt16": return GetUInt16(value);
case "Int32": return GetInt32(value);
case "UInt32": return GetUInt32(value);
case "Int64": return GetInt64(value);
case "UInt64": return GetUInt64(value);
case "Single": return GetSingle(value);
case "Double": return GetDouble(value);
case "Decimal": return GetDecimal(value);
case "DateTime": return GetDateTime(value);
case "TimeSpan": return GetTimeSpan(value);
default: return null;
}
}
}
/// <summary>
/// 获取该类型中属性与数据库字段的对应关系映射
/// </summary>
/// <param name="type"></param>
private static void GenerateTypePropertyMapping(Type type)
{
if (type != null)
{
PropertyInfo[] properties = type.GetProperties(BindingFlag);
Dictionary<string, PropertyInfo> propertyColumnMapping = new Dictionary<string, PropertyInfo>(properties.Length);
string description = string.Empty;
Attribute[] attibutes = null;
string columnName = string.Empty;
bool ignorable = false;
foreach (PropertyInfo p in properties)
{
ignorable = false;
columnName = string.Empty;
attibutes = Attribute.GetCustomAttributes(p);
foreach (Attribute attribute in attibutes)
{
//检查是否设置了ColumnName属性
if (attribute.GetType() == typeof(ColumnNameAttribute))
{
columnName = ((ColumnNameAttribute)attribute).ColumnName;
ignorable = ((ColumnNameAttribute)attribute).Ignorable;
break;
}
}
//如果该属性是可读并且未被忽略的,则有可能在实例化该属性对应的类时用得上
if (p.CanWrite && !ignorable)
{
//如果没有设置ColumnName属性,则直接将该属性名作为数据库字段的映射
if (string.IsNullOrEmpty(columnName))
{
columnName = p.Name;
}
propertyColumnMapping.Add(columnName, p);
}
}
propertyMappings.Add(type, propertyColumnMapping);
}
}
//private static Dictionary<string, int> GetPropertyColumnIndexMapping(DataColumnCollection dataSource, Dictionary<string, PropertyInfo> properties)
//{
// Stopwatch watch = new Stopwatch();
// watch.Start();
// Dictionary<string,int> propertyColumnIndexMapping=new Dictionary<string,int>(dataSource.Count);
// foreach(KeyValuePair<string,PropertyInfo> item in properties)
// {
// for (int i = 0; i < dataSource.Count; i++)
// {
// if (item.Key.Equals(dataSource[i].ColumnName, StringComparison.InvariantCultureIgnoreCase))
// {
// propertyColumnIndexMapping.Add(item.Key, i);
// break;
// }
// }
// }
// watch.Stop();
// Debug.WriteLine("Elapsed:" + watch.ElapsedMilliseconds);
// return propertyColumnIndexMapping;
//}
//private static Dictionary<string, int> GetPropertyColumnIndexMapping(DbDataReader dataSource, Dictionary<string, PropertyInfo> properties)
//{
// Dictionary<string, int> propertyColumnIndexMapping = new Dictionary<string, int>(dataSource.FieldCount);
// foreach (KeyValuePair<string, PropertyInfo> item in properties)
// {
// for (int i = 0; i < dataSource.FieldCount; i++)
// {
// if (item.Key.Equals(dataSource.GetName(i), StringComparison.InvariantCultureIgnoreCase))
// {
// propertyColumnIndexMapping.Add(item.Key, i);
// continue;
// }
// }
// }
// return propertyColumnIndexMapping;
//}
}
/// <summary>
/// 自定义属性,用于指示如何从DataTable或者DbDataReader中读取类的属性值
/// </summary>
public class ColumnNameAttribute : Attribute
{
/// <summary>
/// 类属性对应的列名
/// </summary>
public string ColumnName { get; set; }
/// <summary>
/// 指示在从DataTable或者DbDataReader中读取类的属性时是否可以忽略这个属性
/// </summary>
public bool Ignorable { get; set; }
/// <summary>
/// 构造函数
/// </summary>
/// <param name="columnName">类属性对应的列名</param>
public ColumnNameAttribute(string columnName)
{
ColumnName = columnName;
Ignorable = false;
}
/// <summary>
/// 构造函数
/// </summary>
/// <param name="ignorable">指示在从DataTable或者DbDataReader中读取类的属性时是否可以忽略这个属性</param>
public ColumnNameAttribute(bool ignorable)
{
Ignorable = ignorable;
}
/// <summary>
/// 构造函数
/// </summary>
/// <param name="columnName">类属性对应的列名</param>
/// <param name="ignorable">指示在从DataTable或者DbDataReader中读取类的属性时是否可以忽略这个属性</param>
public ColumnNameAttribute(string columnName, bool ignorable)
{
ColumnName = columnName;
Ignorable = ignorable;
}
}
/// <summary>
/// 主键属性
/// </summary>
public class KeyFlagAttribute : Attribute
{
private bool _bIsKey;
public bool IsKey { get { return _bIsKey; } }
public KeyFlagAttribute(bool bIsKey)
{
_bIsKey = bIsKey;
}
}
2.2 EntityDal部分
/// <summary>
/// 操作数据库的方法
/// 约定:
/// 1)对象属性必须有public string TableName
/// 2)属性名与字段名一样
/// 3)主键属性需标记自定义特性KeyFlagAttribute,表示此属性是数据表中的主键
/// 4)数据表中每个表必须设有主键
/// </summary>
public class EntityDal
{
#region 属性
/// <summary>
/// 构造
/// </summary>
public EntityDal()
{
}
/// <summary>
/// 构造
/// </summary>
/// <param name="conn"></param>
public EntityDal(string conn)
{
this.connectionString = conn;
}
/// <summary>
/// 构造
/// </summary>
/// <param name="conn"></param>
public EntityDal(SqlConnection conn)
{
this.sqlConnection = conn;
}
/// <summary>
/// 数据库连接字符串
/// </summary>
private string connectionString
{
get;
set;
}
/// <summary>
/// 数据库连接对象
/// </summary>
private SqlConnection sqlConnection
{
get;
set;
}
#endregion
#region 数据库增、删、改、查
/// <summary>
/// 增
/// </summary>
/// <param name="obj">要存入数据库的对象</param>
public int Insert(object obj)
{
int n = 0;
using (SqlConnection conn = new SqlConnection(connectionString))
{
conn.Open();
StringBuilder sql = new StringBuilder();
StringBuilder sqlend = new StringBuilder();
//获取对象的属性数组
PropertyInfo[] pro = obj.GetType().GetProperties();
//主键属性数组
List<PropertyInfo> idlist = GetIdProperty(pro);
//要更新的数据表
string table = FindPropertyInfoValue(obj, "TableName").ToString();
//执行的sql语句
string sqltext = string.Empty;
//INSERT INTO table_name (列1, 列2,...) VALUES (值1, 值2,....)
sql.Append("INSERT INTO " + table + "(");
sqlend.Append(" VALUES (");
foreach (PropertyInfo item in pro)
{
//拼接sql语句主体,插入数据时,主键不能显示插入值
if (item.Name == "TableName" || idlist.Any(w => w.Name == item.Name))
{
continue;
}
else
{
string columnValue = item.GetValue(obj, null) + "";
if (string.IsNullOrEmpty(columnValue))
{//去掉空属性
continue;
}
if (item.PropertyType == typeof(DateTime))
{//时间属性初始化时未赋值会变为默认最小值
DateTime dt;
DateTime.TryParse(columnValue, out dt);
if (dt <= SqlDateTime.MinValue.Value)
continue;
}
sql.Append(" " + item.Name + ",");
sqlend.Append(" '" + columnValue + "',");
}
}
string start = sql.ToString();
start = start.Substring(0, start.Length - 1) + ")";
string end = sqlend.ToString();
end = end.Substring(0, end.Length - 1) + ")";
sqltext = start + end;
using (SqlCommand cmd = new SqlCommand(sqltext, conn))
{
n = cmd.ExecuteNonQuery();
}
}
return n;
}
/// <summary>
/// 改
/// </summary>
/// <param name="obj"></param>
/// <returns></returns>
public int Update(object obj)
{
int n = 0;
using (SqlConnection conn = new SqlConnection(connectionString))
{
conn.Open();
StringBuilder sql = new StringBuilder();
StringBuilder sqlend = new StringBuilder();
//获取对象的属性数组
PropertyInfo[] pro = obj.GetType().GetProperties();
//主键属性数组
List<PropertyInfo> idlist = GetIdProperty(pro);
//要更新的数据表
string table = FindPropertyInfoValue(obj, "TableName").ToString();
//执行的sql语句
string sqltext = string.Empty;
//UPDATE 表名称 SET 列名称 = 新值 WHERE 列名称 = 某值 and 列2=某值
sql.Append("UPDATE " + table + " set");
sqlend.Append("WHERE");
//拼接sql语句主体
foreach (PropertyInfo item in pro)
{
if (item.Name == "TableName")
{
continue;
}
else
{
sql.Append(" " + item.Name + "= " + item.GetValue(obj, null));
}
}
//根据主键增加定位条件
foreach (PropertyInfo item in idlist)
{
sqlend.Append(" " + item.Name + "= '" + item.GetValue(obj, null) + ", and ");
}
string start = sql.ToString();
start = start.Substring(0, start.Length - 1) + " ";
string end = sqlend.ToString();
end = end.Substring(0, end.Length - 5) + " ";
sqltext = start + end;
using (SqlCommand cmd = new SqlCommand(sqltext, conn))
{
n = cmd.ExecuteNonQuery();
}
}
return n;
}
/// <summary>
/// 查
/// </summary>
/// <param name="pdata">要填充的对象</param>
/// <returns>赋值后的对象</returns>
public object Select(object pdata)
{
PropertyInfo[] pro = pdata.GetType().GetProperties();//获取传来的对象的属性
List<PropertyInfo> idlist = GetIdProperty(pro);
using (SqlConnection conn = new SqlConnection(connectionString))
{
conn.Open();
StringBuilder sql = new StringBuilder();
StringBuilder sqlend = new StringBuilder();
string table = FindPropertyInfoValue(pdata, "TableName").ToString();
string sqltext = string.Empty;
sql.AppendFormat("select * from {0} with(nolock) where", table);
foreach (PropertyInfo item in idlist)
{
if (item.Name == "TableName")
{
continue;
}
else
{
sql.Append(" " + item.Name + " = '" + item.GetValue(pdata, null) + "'");
}
}
sqltext = sql.ToString();
using (SqlCommand cmd = new SqlCommand(sqltext, conn))
{
SqlDataReader dr = cmd.ExecuteReader(CommandBehavior.SingleRow);
dr.Read();
foreach (PropertyInfo item in pro)
{
if (item.Name == "TableName")
{
continue;
}
else
{
if (string.IsNullOrEmpty(dr[item.Name].ToString()))
{
continue;
}
item.SetValue(pdata, dr[item.Name], null);
}
}
}
}
return pdata;
}
/// <summary>
/// 删
/// </summary>
/// <param name="pdata"></param>
/// <returns></returns>
public int Delete(object pdata)
{
int n = 0;//影响的行数
PropertyInfo[] pro = pdata.GetType().GetProperties();//获取传来的对象的属性
List<PropertyInfo> idlist = GetIdProperty(pro);//找到主键属性
using (SqlConnection conn = new SqlConnection(connectionString))
{
conn.Open();
StringBuilder sql = new StringBuilder();
StringBuilder sqlend = new StringBuilder();
string table = FindPropertyInfoValue(pdata, "TableName").ToString();
string sqltext = string.Empty;
sql.AppendFormat("delete from {0} where", table);
foreach (PropertyInfo item in idlist)
{
sql.Append(" " + item.Name + " = '" + item.GetValue(pdata, null) + "'");
}
sqltext = sql.ToString();
using (SqlCommand cmd = new SqlCommand(sqltext, conn))
{
n = cmd.ExecuteNonQuery();
}
}
return n;
}
//public static List<T> GetPagedList<T>(T obj, int pPageIndex, int pPageSize, string pOrderBy, string pSortExpression, out int pRecordCount)
//{
// if (pPageIndex <= 1)
// pPageIndex = 1;
// List<T> list = new List<T>();
// Query q = Repair.CreateQuery();
// pRecordCount = q.GetRecordCount();
// q.PageIndex = pPageIndex;
// q.PageSize = pPageSize;
// q.ORDER_BY(pSortExpression, pOrderBy.ToString());
// RepairCollection collection = new RepairCollection();
// collection.LoadAndCloseReader(q.ExecuteReader());
// foreach (Repair repair in collection)
// {
// T repairInfo = new T();
// LoadFromDAL(T, repair);
// list.Add(repairInfo);
// }
// return list;
//}
#endregion
#region 辅助方法
/// <summary>
/// 查找返回属性数组中指定名称的属性
/// </summary>
/// <param name="pros"></param>
/// <param name="proName"></param>
/// <returns></returns>
private object FindPropertyInfoValue(object pdata, string proName)
{
PropertyInfo pro = pdata.GetType().GetProperty(proName);
return pro.GetValue(pdata, null);
}
/// <summary>
/// 返回对应名称的属性
/// </summary>
/// <param name="pros"></param>
/// <param name="proName"></param>
/// <returns></returns>
private PropertyInfo FindPropertyInfo(PropertyInfo[] pros, string proName)
{
foreach (PropertyInfo item in pros)
{
if (item.Name == proName)
{
return item;
}
}
return null;
}
/// <summary>
/// 返回主键属性
/// add cjk
/// </summary>
/// <param name="pros">属性数组</param>
/// <returns>主键属性</returns>
private List<PropertyInfo> GetIdProperty(PropertyInfo[] pros)
{
List<PropertyInfo> keyIds = new List<PropertyInfo>();
foreach (PropertyInfo item in pros)
{
//KeyFlagAttribute flag = item.GetCustomAttributes(typeof(KeyFlagAttribute), false)[0] as KeyFlagAttribute;
//if (flag.IsKey)
//{
// return item;
//}
object[] attrs = item.GetCustomAttributes(false);
foreach (var id in attrs)
{
if (id.GetType().Name == "KeyFlagAttribute")
{
keyIds.Add(item);
}
}
}
return keyIds;
}
#endregion
}
/// <summary>
/// 主键属性
/// </summary>
public class KeyFlagAttribute : Attribute
{
private bool _bIsKey;
public bool IsKey { get { return _bIsKey; } }
public KeyFlagAttribute(bool bIsKey)
{
_bIsKey = bIsKey;
}
}
2.3 EntityBll部分
public class EntityBll<T>
{
private string _conn;
/// <summary>
/// 连接数据库字符
/// </summary>
protected string conn
{
get
{
if (string.IsNullOrEmpty(_conn))
{
return ConnectionSql.ConnectionString_CN_DB;
}
else
{
return _conn;
}
}
set
{
_conn = value;
}
}
#region 基本的增删改查
/// <summary>
/// 增
/// </summary>
/// <param name="t"></param>
/// <returns></returns>
public bool Insert(T t)
{
if (new DAL.Base.EntityDal(conn).Insert(t) > 0)
{
return true;
}
else
{
return false;
}
}
/// <summary>
/// 删
/// </summary>
/// <param name="t"></param>
/// <returns></returns>
public bool Delete(T t)
{
if (new DAL.Base.EntityDal(conn).Delete(t) > 0)
{
return true;
}
else
{
return false;
}
}
/// <summary>
/// 改
/// </summary>
/// <param name="t"></param>
/// <returns></returns>
public bool Update(T t)
{
if (new DAL.Base.EntityDal(conn).Update(t) > 0)
{
return true;
}
else
{
return false;
}
}
/// <summary>
/// 查
/// </summary>
/// <param name="t"></param>
/// <returns></returns>
public T Select(T t)
{
return (T)new DAL.Base.EntityDal(conn).Select(t);
}
#endregion
}
三.下面提供三层代码生成器下载地址