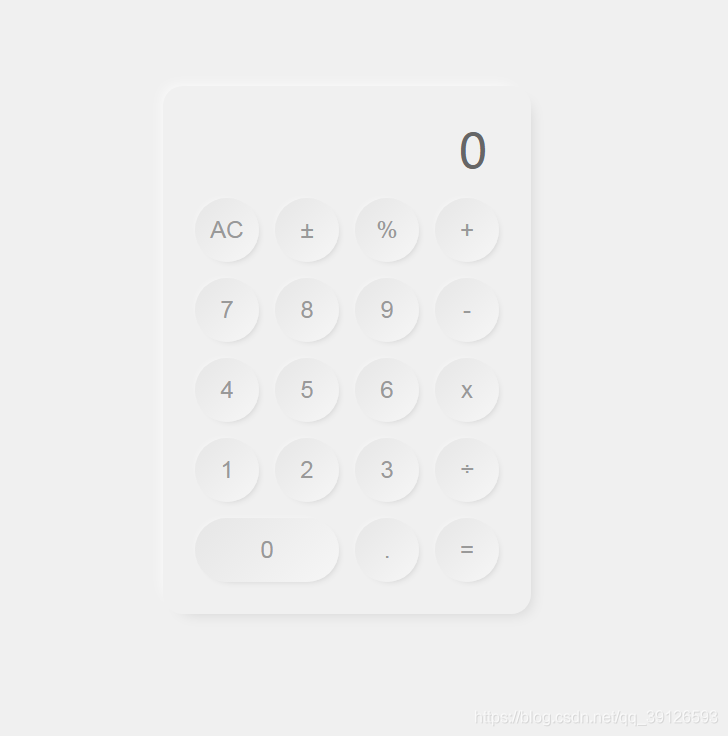
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>calculator</title>
<!-- WebApp全屏模式 -->
<meta name="viewport" content="width=device-width,initial-scale=1.0, minimum-scale=1.0, maximum-scale=1.0, user-scalable=no,minimal-ui">
<!-- 屏蔽ico404 -->
<link rel="shortcut icon" href="#" />
<script src="https://cdn.bootcdn.net/ajax/libs/vue/2.6.12/vue.min.js"></script>
<style type="text/css">
body {
height: 100vh;
margin: 0;
padding: 0;
background: rgba(240, 240, 240, 1);
display: flex;
justify-content: center;
align-items: center;
}
.calculator {
--button-with: 80px;
--button-height: 80px;
display: grid;
grid-template-areas:
"result result result result"
"number-ac number-plus-minus number-percent number-plus"
"number-7 number-8 number-9 number-minus"
"number-4 number-5 number-6 number-multiply"
"number-1 number-2 number-3 number-divide"
"number-0 number-0 number-dot number-equal";
grid-template-columns: repeat(4, var(--button-with));
grid-template-rows: repeat(6, var(--button-height));
box-shadow: -8px -8px 16px -10px rgba(255, 255, 255, 1),
8px 8px 16px -10px rgba(0, 0, 0, 0.15);
padding: 24px;
border-radius: 20px;
}
.calculator button {
margin: 8px;
border: 0;
display: block;
outline: none;
border-radius: calc(var(--button-height) / 2);
font-size: 24px;
font-weight: normal;
color: #999;
background: linear-gradient(135deg, rgba(230, 230, 230, 1) 0%, rgba(246, 246, 246, 1) 100%);
box-shadow: -4px -4px 10px -8px rgba(255, 255, 255, 1), 4px 4px 10px -8px rgba(0, 0, 0, .3);
}
.calculator button:active {
color: rgba(0, 0, 0, .3);
background: rgba(240, 240, 240, 1);
box-shadow: -4px -4px 10px -8px rgba(255, 255, 255, 1) inset, 4px 4px 10px -8px rgba(0, 0, 0, .3) inset;
}
.result {
margin: 0 20px;
text-align: right;
line-height: var(--button-height);
font-size: 48px;
color: #666;
white-space: nowrap;
overflow-y: hidden;
overflow-x: auto;
}
.fail {
color: #f73535;
}
</style>
</head>
<body>
<div id="app">
<div class="calculator">
<div class="result" :class="{fail:equation==='Infinity'}" style="grid-area:result" ref="result">
{{equation==='Infinity'?'不能除以0':equation}}
</div>
<button @click="buttonHandle(item.key)" v-for="(item,index) in buttonSymbol" :key="index" :style="{'grid-area':item.name}">{{item.key}}</button>
<button @click="buttonHandle(index)" v-for="(item,index) in 10" :key="index" :style="{'grid-area':'number-'+index}">{{index}}</button>
</div>
</div>
</body>
<script type="text/javascript">
new Vue({
el: "#app",
data() {
return {
buttonSymbol: [{
name: 'number-ac',
key: 'AC',
},
{
name: 'number-plus-minus',
key: '±',
},
{
name: 'number-percent',
key: '%',
},
{
name: 'number-multiply',
key: 'x',
},
{
name: 'number-divide',
key: '÷',
},
{
name: 'number-plus',
key: '+',
},
{
name: 'number-minus',
key: '-',
},
{
name: 'number-dot',
key: '.',
},
{
name: 'number-equal',
key: '=',
},
],
equation: '0',
isDecimalAdded: false,
isOperatorAdded: false,
isStarted: false
};
},
watch: {
equation() {
this.scrollChange();
}
},
methods: {
isOperator(character) {
return this.buttonSymbol.map(item => item.key).indexOf(character) > -1
},
buttonHandle(character) {
if (this.equation === 'Infinity') {
this.equation = '0'
}
if (this.isOperator(character)) {
if (character === "AC") {
this.equation = '0'
this.isDecimalAdded = false
this.isOperatorAdded = false
return
} else if (character === "±") {
if (/^\d+$/.test(this.equation) || /^\-[1-9][0-9]*$/.test(this.equation)) {
this.equation = (parseFloat(this.equation) * -1).toString()
}
return
} else if (character === "=") {
if (/^\d+$/.test(this.equation.charAt(this.equation.length - 1))) {
let result = this.equation.replace(/x/g, "*").replace(/÷/g, "/")
this.equation = parseFloat(eval(result).toFixed(9)).toString()
this.isOperatorAdded = false
}
return
} else if (character === ".") {
if (this.isDecimalAdded) return
this.isDecimalAdded = true
this.equation += '' + character
}
else {
if (this.isOperatorAdded) {
this.equation = this.equation.slice(0, this.equation.length - 1) + character
return
}
if (this.equation === '0' && character === '-') {
this.equation = '-'
}else{
this.equation += '' + character
}
this.isDecimalAdded = false
this.isOperatorAdded = true
}
} else {
if (this.equation === '0') {
if (character === 0) {
this.equation = '0';
} else {
this.equation = '' + character
}
} else {
this.equation += '' + character
}
this.isOperatorAdded = false
}
},
scrollChange() {
this.$nextTick(() => {
this.$refs.result.scrollLeft = this.$refs.result.scrollWidth;
})
}
},
});
</script>
</html>