queryselector
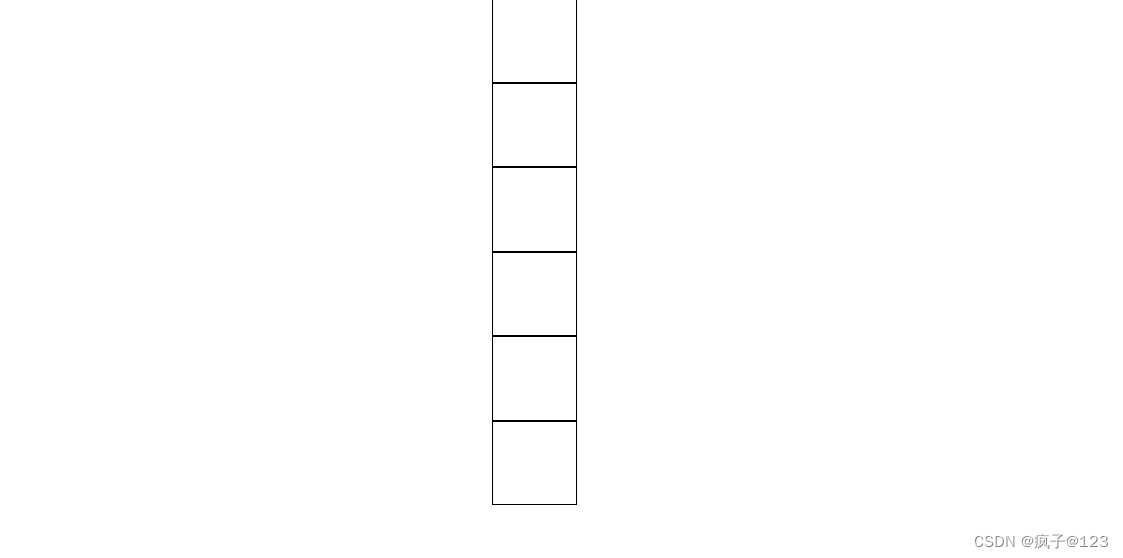
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
*{
margin: 0;
padding: 0;
}
.item{
margin-left: 300px;
width: 50px;
height: 50px;
border: 1px solid;
}
</style>
</head>
<body>
<div class="item"></div>
<div class="item"></div>
<div class="item"></div>
<div class="item"></div>
<div class="item"></div>
</body>
<script type="text/javascript">
window.onload=function(){
var itemNodes = document.querySelectorAll(".item");
console.log(itemNodes.length)
document.body.innerHTML+="<div class='item'></div>";
console.log(itemNodes.length)
for(var i=0;i<itemNodes.length;i++){
itemNodes[i].style.background="pink";
}
}
</script>
</html>
移动端事件基础
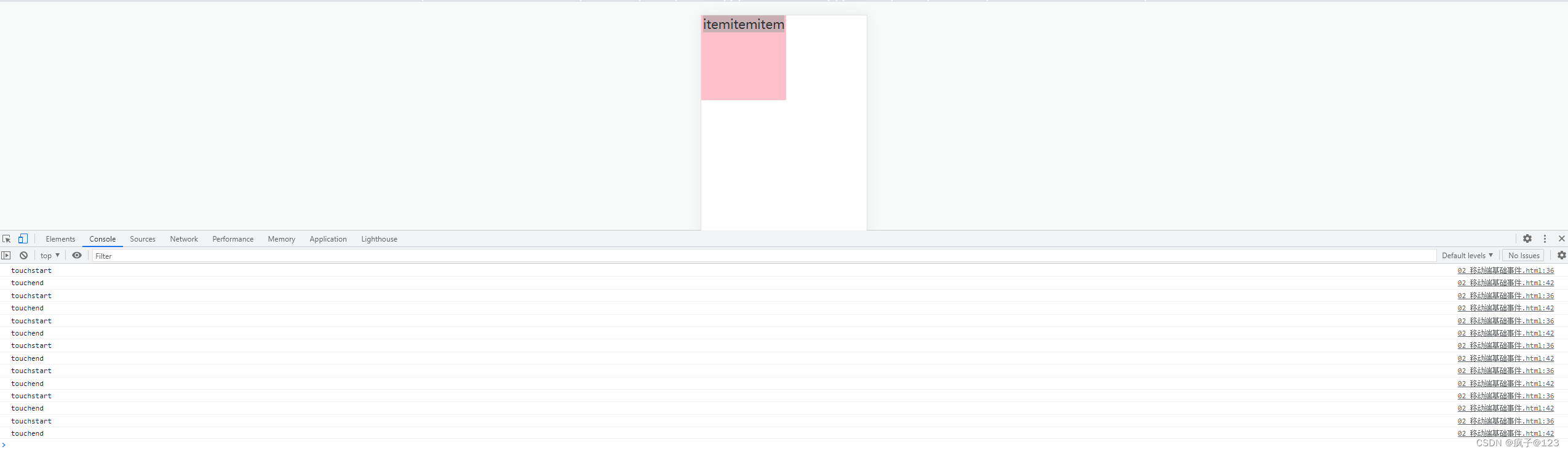
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,user-scalable=no" />
<title></title>
<style type="text/css">
*{
margin: 0;
padding: 0;
}
.item{
width: 200px;
height: 200px;
background: pink;
font-size: 30px;
text-align: center;
}
</style>
</head>
<body>
<div class="item">itemitemitem</div>
</body>
<script type="text/javascript">
window.onload=function(){
var item = document.querySelector(".item");
item.addEventListener("touchstart",function(){
console.log("touchstart")
})
item.addEventListener("touchmove",function(){
console.log("touchmove")
})
item.addEventListener("touchend",function(){
console.log("touchend")
})
}
</script>
</html>
移动端入门应用
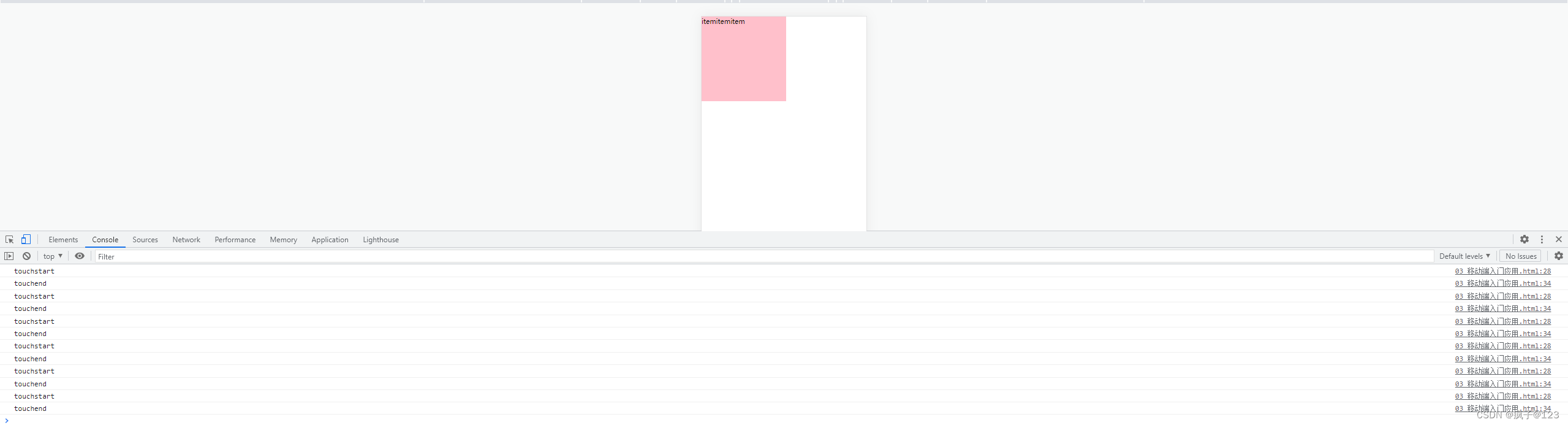
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,user-scalable=no" />
<title></title>
<style type="text/css">
*{
margin: 0;
padding: 0;
}
.item{
width: 200px;
height: 200px;
background: pink;
overflow: auto;
}
</style>
</head>
<body>
<div class="item">itemitemitem</div>
</body>
<script type="text/javascript">
window.onload=function(){
var item = document.querySelector(".item");
item.addEventListener("touchstart",function(){
console.log("touchstart")
})
item.addEventListener("touchmove",function(){
console.log("touchmove")
})
item.addEventListener("touchend",function(){
console.log("touchend")
})
}
</script>
</html>
全面静止事件的默认行为
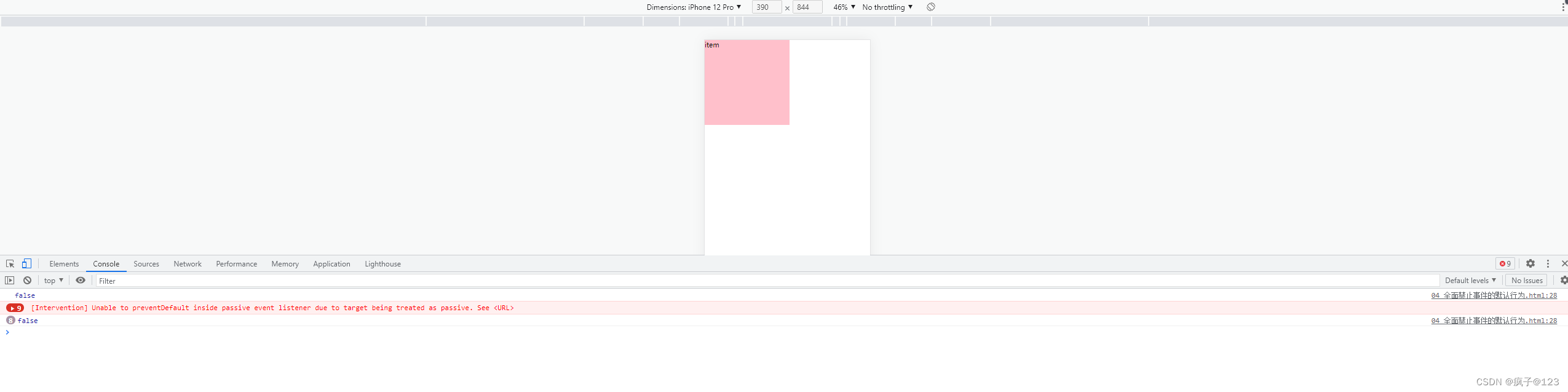
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,user-scalable=no" />
<title></title>
<style type="text/css">
*{
margin: 0;
padding: 0;
}
.item{
width: 200px;
height: 200px;
background: pink;
overflow: auto;
}
</style>
</head>
<body>
<div class="item">item</div>
</body>
<script type="text/javascript">
window.onload=function(){
document.addEventListener("touchstart",function(ev){
ev=ev||event;
var item = document.querySelector(".item");
console.log(ev.cancelable);
ev.preventDefault();
})
}
</script>
</html>
自定义右键菜单
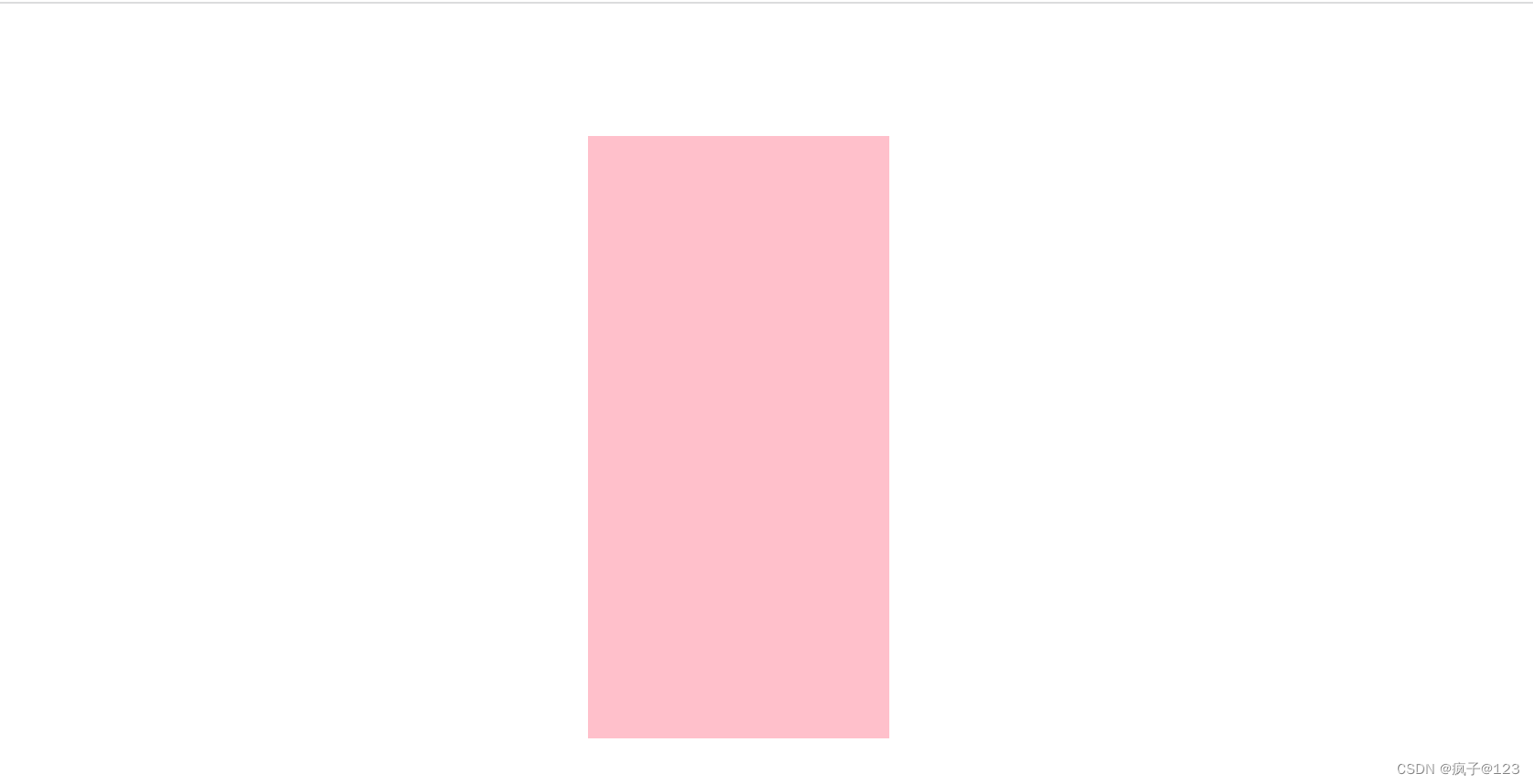
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,user-scalable=no" />
<title></title>
<style type="text/css">
*{
margin: 0;
padding: 0;
}
#wrap{
position: absolute;
width: 200px;
height: 400px;
background: pink;
display: none;
}
</style>
</head>
<body>
<div id="wrap">
</div>
</body>
<script type="text/javascript">
window.onload=function(){
document.oncontextmenu=function(ev){
ev = ev || event;
var x = ev.clientX;
var y = ev.clientY;
var wrap = document.querySelector("#wrap");
wrap.style.display="block";
wrap.style.left = x+"px";
wrap.style.top = y+"px";
return false;
}
}
document.onclick=function(){
var wrap = document.querySelector("#wrap");
wrap.style.display="none"
}
</script>
</html>