文章目录
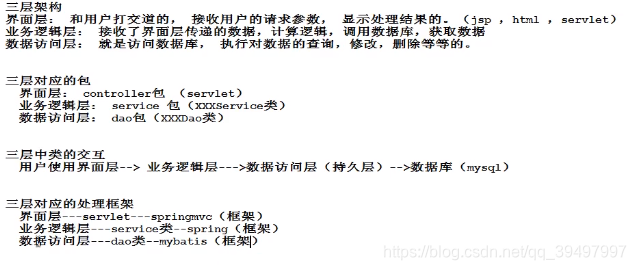
1 MVC (DAO-Service-Controller)
1.1 简介 Model View Controller
经典MVC模式中,M是指业务模型,V是指用户界面,C则是控制器,使用MVC的目的是将M和V的实现代码分离,从而使同一个程序可以使用不同的表现形式。其中,View的定义比较清晰,就是用户界面。
- 流程:在controller访问model获取数据,通过view渲染页面。
1.2 View(视图)
V即View视图是指用户看到并与之交互的界面。比如由html元素组成的网页界面,或者软件的客户端界面。MVC的好处之一在于它能为应用程序处理很多不同的视图。在视图中其实没有真正的处理发生,它只是作为一种输出数据并允许用户操作的方式。
1.3 Model(处理业务)
M即model模型是指模型表示业务规则。在MVC的三个部件中,模型拥有最多的处理任务。被模型返回的数据是中立的,模型与数据格式无关,这样一个模型能为多个视图提供数据,由于应用于模型的代码只需写一次就可以被多个视图重用,所以减少了代码的重复性。
DAO(Data Access Object)是一个数据访问接口,数据访问:顾名思义就是与数据库打交道。夹在业务逻辑与数据库资源中间。
Service层:业务层 控制业务
1.4 Controller(接收客户端请求)
C即controller控制器是指控制器接受用户的输入并调用模型和视图去完成用户的需求,控制器本身不输出任何东西和做任何处理。它只是接收请求并决定调用哪个模型构件去处理请求,然后再确定用哪个视图来显示返回的数据。
2 省市联动
2.1 entity
vo(封装返回数据类型)
Response
package com.tony.pc.entity.vo;
import com.tony.constant.ResponseCode;
/**
* 每个接口返回的数据结构都是统一的
*/
public class Response {
private Integer code = ResponseCode.SUCCESS;//自定义的响应码
private String msg = "成功";//响应信息
private Object data;//携带数据
public Integer getCode() {
return code;
}
public void setCode(Integer code) {
this.code = code;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
public Object getData() {
return data;
}
public void setData(Object data) {
this.data = data;
}
}
实体类
City
package com.tony.pc.entity;
public class City {
private Integer id;//城市Id
private String name;//城市名称
private String descp;//城市描述
private Integer pid;//省份Id
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescp() {
return descp;
}
public void setDescp(String descp) {
this.descp = descp;
}
public Integer getPid() {
return pid;
}
public void setPid(Integer pid) {
this.pid = pid;
}
@Override
public String toString() {
return "City{" +
"id=" + id +
", name='" + name + '\'' +
", descp='" + descp + '\'' +
", pid=" + pid +
'}';
}
}
Province
package com.tony.pc.entity;
public class Province {
private Integer id;//封装省份id
private String name;//封装省份名称
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Province{" +
"id=" + id +
", name='" + name + '\'' +
'}';
}
}
2.2 常量(响应码)
package com.tony.constant;
public interface ResponseCode {
Integer SUCCESS = 6666;//成功
Integer ERROR = 8888;//失败
}
2.3 utils
QueryRunnerUtil
package com.tony.pc.utils;
import com.alibaba.druid.pool.DruidDataSource;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import org.apache.commons.dbutils.QueryRunner;
import javax.sql.DataSource;
import java.util.Properties;
public class QueryRunnerUtil {
private static QueryRunner queryRunner = null;
//私有化构造方法
private QueryRunnerUtil(){}
//饿汉模式
static {
try {
Properties prop = new Properties();
prop.load(QueryRunnerUtil.class.getClassLoader().getResourceAsStream("db.properties"));
DataSource druidDataSource = (DruidDataSource) DruidDataSourceFactory.createDataSource(prop);
queryRunner = new QueryRunner(druidDataSource);
} catch (Exception e) {
e.printStackTrace();
}
}
public static QueryRunner getQueryRunner(){
return queryRunner;
}
}
db.properties
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/tony?serverTimezone=Asia/Shanghai
username=root
password=123456
initialSize=5
2.4 Dao
CityDao
package com.tony.pc.dao;
import com.tony.pc.entity.City;
import com.tony.pc.utils.QueryRunnerUtil;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import java.sql.SQLException;
import java.util.List;
public class CityDao {
public List<City> selectCityListByProvinceId(Integer provinceId){
QueryRunner queryRunner = QueryRunnerUtil.getQueryRunner();
String sql = "select c.* from city c inner join province p on c.pid=p.id where p.id=?";
List<City> cityList = null;
try {
cityList = (List<City>) queryRunner.query(sql, provinceId, new BeanListHandler(City.class));
} catch (SQLException throwables) {
throwables.printStackTrace();
}
return cityList;
}
}
ProvinceDao
package com.tony.pc.dao;
import com.tony.pc.entity.Province;
import com.tony.pc.utils.QueryRunnerUtil;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import java.sql.SQLException;
import java.util.List;
public class ProvinceDao {
public List<Province> selectQueryList(){
QueryRunner queryRunner = QueryRunnerUtil.getQueryRunner();
try {
String sql = "select * from province;";
List<Province> provinceList = (List<Province>) queryRunner.query(sql, new BeanListHandler(Province.class));
return provinceList;
} catch (SQLException throwables) {
throwables.printStackTrace();
}
return null;
}
}
2.5 Service
CityService
package com.tony.pc.service;
import com.tony.pc.dao.CityDao;
import com.tony.pc.entity.City;
import com.tony.pc.entity.vo.Response;
import java.util.List;
public class CityService {
private CityDao cityDao = new CityDao();
public Response queryCityListByProvinceId(Integer provinceId) {
List<City> cityList = cityDao.selectCityListByProvinceId(provinceId);
Response response = new Response();
response.setData(cityList);
return response;
}
}
ProvinceService
package com.tony.pc.service;
import com.alibaba.fastjson.JSON;
import com.tony.pc.dao.ProvinceDao;
import com.tony.pc.entity.Province;
import com.tony.pc.entity.vo.Response;
import java.util.List;
public class ProvinceService {
private ProvinceDao provinceDao = new ProvinceDao();
/**
* 查询省份列表
* @return 返回省份列表
*/
public Response queryProvinceList() {
List<Province> provinceList = provinceDao.selectQueryList();
//创建响应对象
Response response = new Response();
response.setData(provinceList);
return response;
}
}
2.6 Controller
CityListController
package com.tony.pc.controller;
import com.alibaba.fastjson.JSON;
import com.tony.pc.entity.vo.Response;
import com.tony.pc.service.CityService;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class CityListController extends HttpServlet {
private CityService cityService = new CityService();
@Override
protected void doGet(HttpServletRequest httpRequest, HttpServletResponse httpResponse) throws ServletException, IOException {
//获取客户端传来的pid
Integer pid = Integer.parseInt(httpRequest.getParameter("pid"));
httpResponse.setContentType("application/json;charset=utf-8");
Response response = cityService.queryCityListByProvinceId(pid);
String JsonStr = JSON.toJSONString(response);
httpResponse.getWriter().write(JsonStr);
}
}
ProvinceListController
package com.tony.pc.controller;
/**
* 获取省份列表的controller
*
* 只是用来接收客户端的请求,不负责处理具体的业务逻辑
*/
import com.alibaba.fastjson.JSON;
import com.tony.constant.ResponseCode;
import com.tony.pc.entity.Province;
import com.tony.pc.entity.vo.Response;
import com.tony.pc.service.ProvinceService;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.List;
public class ProvinceListController extends HttpServlet {
private ProvinceService provinceService = new ProvinceService();
@Override
protected void doGet(HttpServletRequest httpRequest, HttpServletResponse httpResponse) throws ServletException, IOException {
httpResponse.setContentType("application/json;charset=utf8");
Response response = provinceService.queryProvinceList();
String jsonStr = JSON.toJSONString(response);
httpResponse.getWriter().write(jsonStr);
}
}
3 Web
3.1 web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<servlet>
<servlet-name>controller</servlet-name>
<servlet-class>com.tony.pc.controller.ProvinceListController</servlet-class>
</servlet>
<servlet>
<servlet-name>cityListController</servlet-name>
<servlet-class>com.tony.pc.controller.CityListController</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>controller</servlet-name>
<url-pattern>/listProvince</url-pattern>
</servlet-mapping>
<servlet-mapping>
<servlet-name>cityListController</servlet-name>
<url-pattern>/listCity</url-pattern>
</servlet-mapping>
</web-app>
3.2 ops1.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>省市联动</title>
<style>
.box {
width: 400px;
height: 26px;
margin: 0 auto;
display: flex;
align-items: center;
justify-content: center;
margin-top: 100px;
}
.box select {
height: 100%;
width: 80px;
}
.box select:nth-child(2) {
margin: 0 2px;
}
.btn {
height: 100%;
width: 140px;
}
.box-descp {
width: 400px;
height: 26px;
margin: 40px auto;
display: flex;
align-items: center;
justify-content: center;
}
</style>
<script type="text/javascript" src="jquery-1.9.1.min.js"></script>
</head>
<body>
<div class="box">
<select class="province">
</select>
<select class="city">
</select>
<button class="btn">点我查询城市详情</button>
</div>
<div class="box-descp">
</div>
<script>
//dao controller service
$(function () {
//1.填充省份数据
$.ajax({
url: "/listProvince",
type: "GET",
dataType: "json",
success: function (resp) {
//清空
$(".province").empty();
//console.log(resp)
if (resp.code === 6666) {
for (index in resp.data) {
//console.log(resp.data[index].name)
let provinceName = resp.data[index].name;
let provinceId = resp.data[index].id;
$(".province").append("<option value='" + provinceId + "'>" + provinceName + "</option>>")
}
}
/*console.log($(".province").val())
console.log($(".city").val())*/
// 使用默认选择的省份id查询城市列表
getCityListBtPid($(".province").val())
},
error: function (msg) {
console.log("失败")
}
});
//2.根据选中省份查询城市列表
$(".province").change(function () {
//选中立马置空城市
$(".city").empty();
getCityListBtPid(this.value)
});
//3.点击查询城市详情信息
$(".btn").click(function (){
$(".box-descp").empty();
//获取当前省份id
let provinceId = $(".province").val();
//获取当前城市id
let cityId = $(".city").val();
//根据省份id查到city信息
$.ajax({
url: "/listCity?pid=" + provinceId,
success: function (resp) {
if (resp.code === 6666) {
for (index in resp.data) {
if(resp.data[index].id==cityId){
let descp1 = resp.data[index].descp
$(".box-descp").append(`<span>${descp1}</span>`)
}
}
}
}
});
});
});
function getCityListBtPid(provinceId) {
/*//获取省份Id
let provinceId = this.value;*/
//根据省份Id查询所有城市
$.ajax({
url: "listCity?pid=" + provinceId,
success: function (resp) {
if (resp.code === 6666) {
//console.log(resp.data)
for (index in resp.data) {
//console.log(resp.data[index].name)
let cityName = resp.data[index].name;
let cityId = resp.data[index].id;
$(".city").append(`<option value="${cityId}">${cityName}</option>`)
}
}
}
});
}
</script>
</body>
</html>