import java.util.ArrayList;
import java.util.Scanner;
public class Employees {
static ArrayList<Employee> list = new ArrayList<Employee>();
static Scanner input = new Scanner(System.in);
public static void main(String[] args) {
System.out.println("欢迎使用职工薪水管理系统\n");
while(true){
System.out.println("-------------------------------------------------------------------------------------------------------------------");
System.out.println("请选择:\n1.添加员工 2.查询员工信息 3.显示所有员工信息 4.修改员工薪水 5.删除员工 6.员工薪水排序 7.统计平均工资,最高、低工资");
System.out.print("请输入序号:");
int choice = input.nextInt();
switch(choice){
case 1:
addEmployee();
break;
case 2:
selectEmployee();
break;
case 3:
showAllEmployee();
break;
case 4:
updateEmployeeMoney();
break;
case 5:
delEmployee();
break;
case 6:
MoneySort();
break;
case 7:
other();
break;
default:
System.out.println("\n没有这个选项,请重新选择!!!!!");
}
}
}
public static void addEmployee(){
System.out.println("\n--------------添加员工-------------");
String id;
String name;
double salary;
while(true){
boolean flag = false;
System.out.print("请输入员工号:");
id = input.next();
for(int i=0;i<list.size();i++){
Employee emp = list.get(i);
if(emp.getId().equals(id)){
flag = true;
} else {
break;
}
}
if(flag){
System.out.println("该员工号已存在,请重新输入!!!!!");
} else {
break;
}
}
System.out.print("请输入员工姓名:");
name = input.next();
System.out.print("请输入员工工资:");
salary = input.nextDouble();
Employee emp = new Employee(id, name, salary);
boolean bool = list.add(emp);
if(bool==true){
System.out.println("恭喜,添加成功!");
} else {
System.out.println("员工数据添加失败!");
}
}
public static void selectEmployee(){
System.out.println("\n--------------查询员工信息-------------");
System.out.print("请输入要查询的员工号:");
boolean flag = false;
String checkId = input.next();
for(int i=0;i<list.size();i++){
Employee emp = list.get(i);
if(emp.getId().equals(checkId)){
System.out.println("查询成功!");
System.out.println("员工号 员工姓名 薪资");
System.out.println(emp.toString());
flag = true;
}
}
if(flag == false){
System.out.println("该员工不存在!");
}
}
public static void showAllEmployee(){
if(list.size()==0){
System.out.println("无员工信息");
return;
}
System.out.println("员工号 员工姓名 薪资");
for(Employee emp:list){
System.out.println(emp.toString());
}
}
public static void updateEmployeeMoney(){
System.out.println("\n--------------员工薪资修改-------------");
System.out.print("请输入要修改薪资的员工号:");
String id = input.next();
boolean flag = false;
int index = 0;
double newSalary;
for(int i=0;i<list.size();i++){
Employee emp = list.get(i);
if(emp.getId().equals(id)){
flag = true;
index = i;
}
}
if(flag == true){
System.out.print("请输入你要修改的薪资:");
newSalary = input.nextDouble();
Employee emp = list.get(index);
emp.setSalary(newSalary);
System.out.println("修改成功!!!");
} else {
System.out.println("没有此员工!!!");
}
}
public static void delEmployee(){
System.out.println("\n--------------删除员工信息-------------");
System.out.print("请输入要删除员工的员工号:");
String id = input.next();
boolean flag = false;
for(int i=0;i<list.size();i++){
Employee emp = list.get(i);
if(emp.getId().equals(id)){
list.remove(i);
flag = true;
}
}
if(flag == true){
System.out.println("删除成功!!!");
} else {
System.out.println("未找到此员工,删除失败!!!");
}
}
public static void MoneySort(){
System.out.println("\n--------------员工薪资排序-------------");
System.out.println("请选择:1.薪资从高到低 2.薪资从低到高");
System.out.print("请输入:");
int choice = input.nextInt();
switch(choice){
case 1:
Desc();
break;
case 2:
Asc();
break;
}
}
public static void Asc(){
Employee temp;
System.out.println("薪资从低到高排序结果是:");
System.out.println("员工号 员工姓名 薪资");
for(int i=0;i<list.size()-1;i++){
for(int j=0;j<list.size()-1-i;j++){
if(list.get(j).getSalary()>list.get(j+1).getSalary()){
temp = list.get(j);
list.set(j, list.get(j+1));
list.set(j+1, temp);
}
}
}
for(int i =0;i<list.size();i++){
System.out.println(list.get(i));
}
}
public static void Desc(){
Employee temp;
System.out.println("薪资从高到低排序结果是:");
System.out.println("员工号 员工姓名 薪资");
for(int i=0;i<list.size()-1;i++){
for(int j=0;j<list.size()-1-i;j++){
if(list.get(j).getSalary()<list.get(j+1).getSalary()){
temp = list.get(j);
list.set(j, list.get(j+1));
list.set(j+1, temp);
}
}
}
for(int i =0;i<list.size();i++){
System.out.println(list.get(i));
}
}
public static void other(){
System.out.println("\n--------------员工平均、最高、最低工资-------------");
double sum = 0;
double avg;
double max = list.get(0).getSalary();
double min = list.get(0).getSalary();
for(int i=0;i<list.size();i++){
Employee emp = list.get(i);
sum += emp.getSalary();
if(list.get(i).getSalary() > max){
max = list.get(i).getSalary();
}
if(list.get(i).getSalary()<min){
min = list.get(i).getSalary();
}
}
avg = sum/list.size();
showAllEmployee();
System.out.println("员工平均工资:"+avg+", 最高工资"+max+", 最低工资"+min);
}
}
class Employee{
private String id;
private String name;
private double salary;
public Employee() {
super();
}
public Employee(String id, String name, double salary) {
super();
this.id = id;
this.name = name;
this.salary = salary;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
@Override
public String toString() {
return id + "\t" + name + "\t" + salary ;
}
}
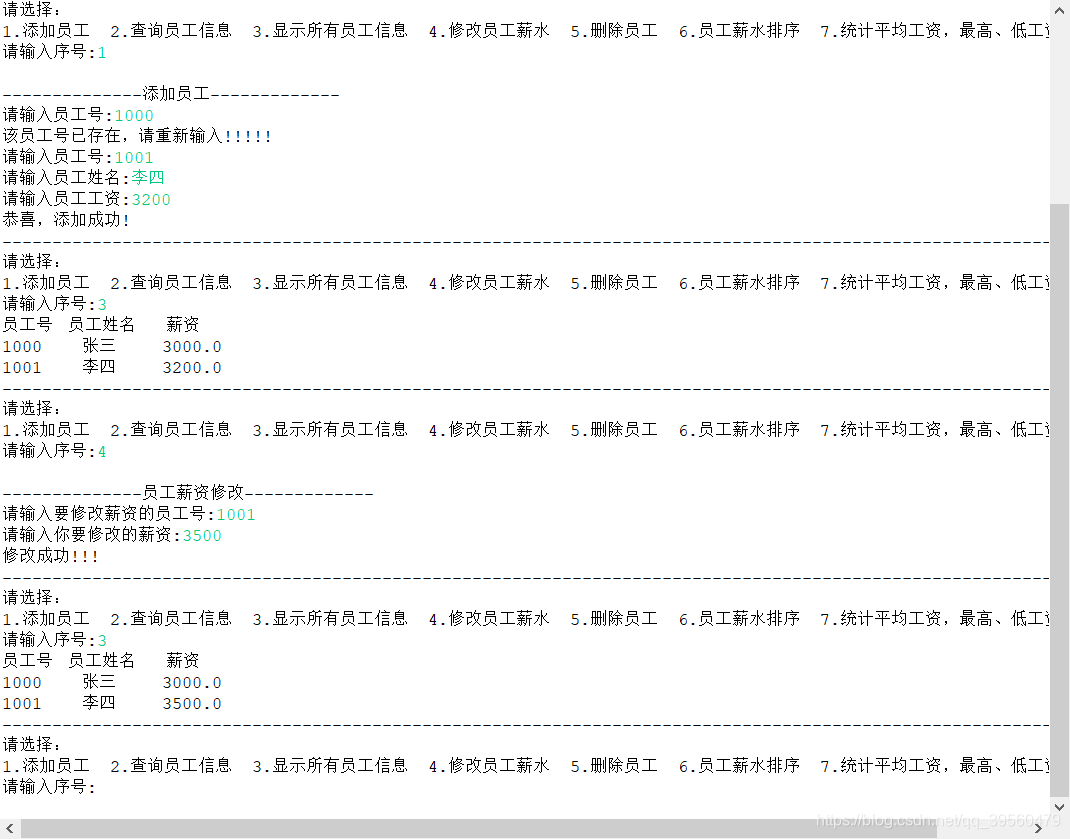