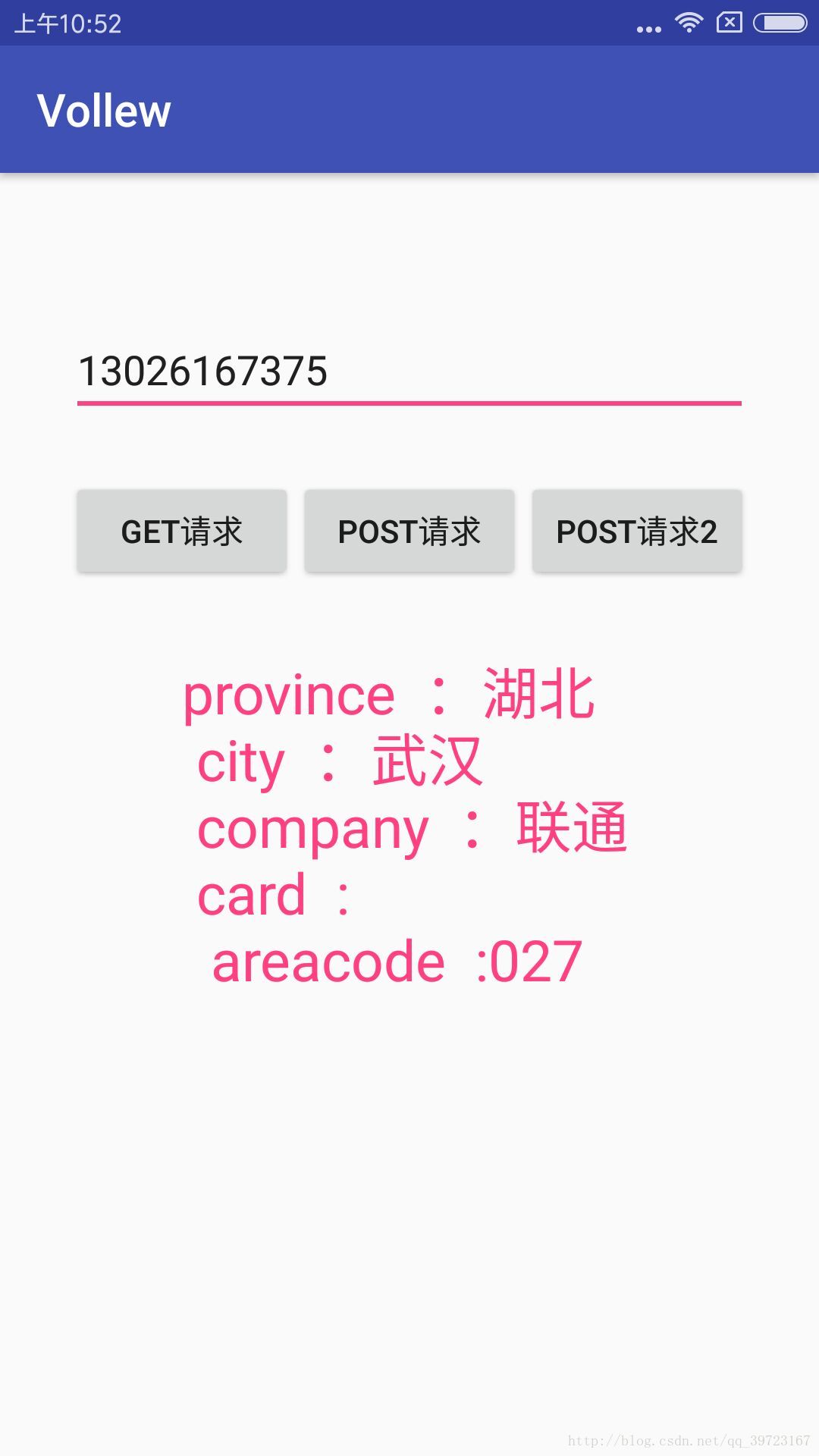
1创建请求队列 MyApplication
import android.app.Application;
import android.view.animation.Animation;
import com.android.volley.RequestQueue;
import com.android.volley.toolbox.JsonObjectRequest;
import com.android.volley.toolbox.Volley;
/**
* Created by BX on 2017/8/7.
*/
public class MyApplication extends Application {
public static RequestQueue queues;
@Override
public void onCreate() {
super.onCreate();
queues= Volley.newRequestQueue(getApplicationContext());
}
public static RequestQueue getHttpQueuse(){
return queues;
}
}
2,把MyApplication注册到项目清单中…加入网络权限
android:name=".MyApplication"
<uses-permission android:name="android.permission.INTERNET"></uses-permission>
3,布局main-xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.bx.vollew.MainActivity">
<EditText
android:id="@+id/edit1"
android:layout_width="300dp"
android:hint="输入手机号"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="64dp" />
<Button
android:id="@+id/but1"
android:layout_width="100dp"
android:text="get请求"
android:layout_height="wrap_content"
android:layout_below="@+id/edit1"
android:layout_alignLeft="@+id/edit1"
android:layout_alignStart="@+id/edit1"
android:layout_marginTop="24dp" />
<Button
android:id="@+id/but2"
android:layout_width="100dp"
android:text="POST请求"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/but3"
android:layout_alignBottom="@+id/but3"
android:layout_toRightOf="@+id/but1"
android:layout_toEndOf="@+id/but1" />
<Button
android:id="@+id/but3"
android:layout_width="100dp"
android:text="POST请求2"
android:layout_height="wrap_content"
android:layout_above="@+id/textview"
android:layout_alignRight="@+id/edit1"
android:layout_alignEnd="@+id/edit1" />
<TextView
android:id="@+id/textview"
android:layout_width="200dp"
android:textSize="25dp"
android:textColor="@color/colorAccent"
android:layout_height="200dp"
android:layout_marginTop="30dp"
android:layout_below="@+id/but1"
android:layout_centerHorizontal="true" />
</RelativeLayout>
3main 中的代码
package com.example.bx.vollew
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.util.Log
import android.view.View
import android.widget.Button
import android.widget.EditText
import android.widget.TextView
import android.widget.Toast
import com.android.volley.AuthFailureError
import com.android.volley.Request
import com.android.volley.Response
import com.android.volley.VolleyError
import com.android.volley.toolbox.JsonObjectRequest
import com.android.volley.toolbox.StringRequest
import com.google.gson.Gson
import org.json.JSONException
import org.json.JSONObject
import java.net.URI
import java.util.HashMap
import java.util.Map
public class MainActivity extends AppCompatActivity {
private EditText phone
private Button button,but2,but3
private TextView textView
private String num=""
private static final String TAG = "MainActivity"
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
//初始化控件
phone= (EditText) findViewById(R.id.edit1)
but2= (Button) findViewById(R.id.but2)
but3= (Button) findViewById(R.id.but3)
button= (Button) findViewById(R.id.but1)
textView= (TextView) findViewById(R.id.textview)
// get的点击事件,
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
num= phone.getText().toString()
volley_Get()
}
})
//post 的请求事件
but2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
volley_Post()
}
})
// but3.setOnClickListener(new View.OnClickListener() {
// @Override
// public void onClick(View view) {
// volley_Post1()
// }
//
//
// })
}
private void volley_Get() {
//申请的接口
String url="http://apis.juhe.cn/mobile/get?phone="+num+"&key=69eae34c7c30b963a0ec2eeefc05a37d"
StringRequest request=new StringRequest(Request.Method.GET, url,new Response.Listener<String>() {
@Override
//get请求成功是显示的数据
public void onResponse(String response) {
Log.e("TAG",response)
Toast.makeText(MainActivity.this,response,Toast.LENGTH_LONG).show()
// get请求的数据是 JSON 的数据 用Gson 吧数据解析出来
Gson gson=new Gson()
Resultcode resultcode=gson.fromJson(response,Resultcode.class)
//解析的数据在textView 中显示
textView.setText("province :" + resultcode.getResult().getProvince()+ "\n city :"
+ resultcode.getResult().getCity() + "\n company :"
+ resultcode.getResult().getCompany() + "\n card :" + resultcode.getResult().getCard()
+"\n areacode :" +resultcode.getResult().getAreacode())
}
//请求失败显示的数据
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
Log.e("TAG",error.getMessage())
Toast.makeText(MainActivity.this,error.toString(),Toast.LENGTH_LONG).show()
}
})
request.setTag("abcGet")
MyApplication.getHttpQueuse().add(request)
}
private void volley_Post() {
String url="http://apis.juhe.cn/mobile/get?"
StringRequest request=new StringRequest(Request.Method.POST, url, new Response.Listener<String>() {
@Override
public void onResponse(String response) {
Log.e("TAG",response)
Toast.makeText(MainActivity.this,response,Toast.LENGTH_LONG).show()
Gson gson=new Gson()
Resultcode resultcode=gson.fromJson(response,Resultcode.class)
textView.setText("province :" + resultcode.getResult().getProvince()+ "\n city :"
+ resultcode.getResult().getCity() + "\n company :"
+ resultcode.getResult().getCompany() + "\n card :" + resultcode.getResult().getCard()
+"\n areacode :" +resultcode.getResult().getAreacode())
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
Toast.makeText(MainActivity.this,error.toString(),Toast.LENGTH_LONG).show()
}
}){
@Override
//post请求方法 在后面加一个getParams方法
protected Map<String, String> getParams() throws AuthFailureError {
num= phone.getText().toString()
Map<String,String> map=new HashMap<>()
map.put("phone",num)
map.put("key","69eae34c7c30b963a0ec2eeefc05a37d")
return map
}
}
request.setTag("abcPost")
MyApplication.getHttpQueuse().add(request)
}
//这个post请求方法 key的请求错误 已注解
// private void volley_Post1() {
//
// num= phone.getText().toString()
// String url="http://apis.juhe.cn/mobile/get"
// Map<String,String> map=new HashMap<>()
// map.put("phone","13026167375")
// map.put("key","69eae34c7c30b963a0ec2eeefc05a37d")
// JSONObject obj=new JSONObject(map)
// JsonObjectRequest jsonObjectRequest=new JsonObjectRequest(Request.Method.POST, url, obj, new Response.Listener<JSONObject>() {
// @Override
// public void onResponse(JSONObject response) {
//
// Toast.makeText(MainActivity.this,response.toString(),Toast.LENGTH_LONG).show()
//
// }
// }, new Response.ErrorListener() {
// @Override
// public void onErrorResponse(VolleyError error) {
// Toast.makeText(MainActivity.this,error.toString(),Toast.LENGTH_LONG).show()
// }
// })
// jsonObjectRequest.setTag("abcPost")
// MyApplication.getHttpQueuse().add(jsonObjectRequest)
// }
}