#include<cstdio>
#include<cstring>
#include<map>
using namespace std;
typedef long long ll;
typedef unsigned long long ull;
const int N=35;
const char WORD[51][101]={
"","PROGRAM","CONST","VAR","INTEGER","LONG","PROCEDURE","IF","THEN","WHILE","DO","READ","WRITE","BEGIN","END","ODD",
"+","-","*","/","=","<>","<","<=",">",">=",",",".",";",":",":=","(",")","","","#"
};
char s[10010];
map <ull,int> mp;
int cnt_var,len;
const ull seed=13131;
ull gethash(int l,int r){
ull re=0;
for (int i=l;i<=r;i++){
re=re*seed+s[i]-'0';
}
return re;
}
void print(int pos,int l=0,int r=0){
if (pos==34){
printf("<34,");
for (int i=l;i<=r;i++) printf("%c",s[i]);
printf(">\n");
}
else printf("<%d,%s>\n",pos,WORD[pos]);
}
void Divide_Expression(){
int l=1;
for (int i=1;i<=len;i++){
if (s[i]=='+'){
if (i-1>0) print(34,l,i-1);
print(16);
l=i+1;
}else if (s[i]=='-'){
if (i-1>0) print(34,l,i-1);
print(17);
l=i+1;
}else if (s[i]=='*'){
if (i-1>0) print(34,l,i-1);
print(18);
l=i+1;
}else if (s[i]=='/'){
if (i-1>0) print(34,l,i-1);
print(19);
l=i+1;
}else if (s[i]=='='){
if (i-1>0) print(34,l,i-1);
print(20);
l=i+1;
}else if (s[i]=='<'){
if (i-1>0) print(34,l,i-1);
if (s[i+1]=='>') print(21),i++;
else if (s[i+1]=='=') print(23),i++;
else print(22);
l=i+1;
}else if (s[i]=='>'){
if (i-1>0) print(34,l,i-1);
if (s[i+1]=='=') print(25),i++;
else print(24);
l=i+1;
}else if (s[i]=='.'){
if (i-1>0) print(34,l,i-1);
print(26);
l=i+1;
}else if (s[i]==','){
if (i-1>0) print(34,l,i-1);
print(27);
l=i+1;
}else if (s[i]==';'){
if (i-1>0) print(34,l,i-1);
print(28);
l=i+1;
}else if (s[i]==':'){
if (i-1>0) print(34,l,i-1);
if (s[i+1]=='=') print(30),i++;
else print(29);
l=i+1;
}else if (s[i]=='('){
if (i-1>0) print(34,l,i-1);
print(31);
l=i+1;
}else if (s[i]==')'){
if (i-1>0) print(34,l,i-1);
print(32);
l=i+1;
}else if (s[i]=='#'){
if (i-1>0) print(34,l,i-1);
print(35);
l=i+1;
}else if (i==len){
print(34,l,len);
}
}
}
void Check_Keyword(){
len=strlen(s+1);
int tag=0;
if(s[1]=='P') // 1-PROGRAM or 6-PROCEDURE
{
tag=1;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==7) {
print(1); return;
}
tag=6;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==9){
print(6); return;
}else {
Divide_Expression(); return;//print(34); continue;
}
}
if (s[1]=='C') // 2-CONST
{
tag=2;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==5){
print(2); return;
}else {
Divide_Expression(); return;
}
}
if (s[1]=='V'){ // 3-VAR
tag=3;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==3){
print(3); return;
}
else {
Divide_Expression(); return;
}
}
if (s[1]=='I'){ // 4-INTEGER or 7-IF
tag=4;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==7) {
print(4); return;
}
tag=7;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==2){
print(7); return;
}else {
Divide_Expression(); return;//print(34); continue;
}
}
if (s[1]=='L'){ // 5-LONG
tag=5;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==4){
print(5); return;
}
else {
Divide_Expression(); return;
}
}
if (s[1]=='T') {// 8-THEN
tag=8;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==4){
print(8); return;
}
else {
Divide_Expression(); return;
}
}
if (s[1]=='W'){ // 9-WHILE or 12-WRITE
tag=9;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==5) {
print(9); return;
}
tag=12;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==5){
print(12); return;
}else {
Divide_Expression(); return;//print(34); continue;
}
}
if (s[1]=='D'){ // 10-DO
if (len==2&&s[2]=='O'){
print(10); return;
}else {
Divide_Expression(); return;
}
}
if (s[1]=='R'){ // 11-READ
if (len==4&&s[2]=='E'&&s[3]=='A'&&s[4]=='D'){
print(11); return;
}else {
Divide_Expression(); return;
}
}
if (s[1]=='B') { // 13-BEGIN
tag=13;
for (int i=1;i<=len;i++){
if (s[i]!=WORD[tag][i-1]){
tag=-1; break;
}
}
if (tag!=-1&&len==5){
print(13); return;
}
else {
Divide_Expression(); return;
}
}
if (s[1]=='E'){ // 14-END
if (len==3&&s[2]=='N'&&s[3]=='D'){
print(14); return;
}else {
Divide_Expression(); return;
}
}
if (s[1]=='O'){ // 15-ODD
if (len==3&&s[2]=='D'&&s[3]=='D'){
print(15); return;
}else {
Divide_Expression(); return;
}
}
Divide_Expression();
}
int main(){
//freopen("read.txt","r",stdin);
//freopen("out.txt","w",stdout);
while (~scanf("%s",s+1)){
Check_Keyword();
}
}
编译原理-词法分析器
最新推荐文章于 2024-08-12 16:55:46 发布
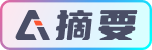