1.poco库的安装和编译见上篇:
https://blog.csdn.net/qq_40167046/article/details/109119763
2.QT的poco配置
.pro文件,添加poco的配置
## POCO配置 ##
# poco的头文件等都在这个目录下,到include一级即可,不需要再下去到 /poco/
# 其实不需要加这句,因为该路径是默认添加的
INCLUDEPATH += /usr/local/include
# 配置1,动态库
CONFIG(debug, debug|release){
LIBS += -L/usr/local/lib \
-lPocoCryptod \
-lPocoDatad \
-lPocoDataSQLited \
-lPocoEncodingsd \
-lPocoFoundationd \
-lPocoJSONd \
-lPocoNetd \
-lPocoRedisd \
-lPocoUtild \
-lPocoXMLd \
-lPocoZipd
}
CONFIG(release, debug|release){
LIBS += -L/usr/local/lib \
-lPocoCrypto \
-lPocoData \
-lPocoDataSQLite \
-lPocoEncodings \
-lPocoFoundation \
-lPocoJSON \
-lPocoNet \
-lPocoRedis \
-lPocoUtil \
-lPocoXML \
-lPocoZip
}
# 配置2,静态库
#LIBS += /usr/local/lib/libPocoJSONd.a \
# /usr/local/lib/libPocoNetd.a \
# /usr/local/lib/libPocoFoundationd.a
#LIBS += /usr/local/lib/libPocoFoundation.a \
# /usr/local/lib/libPocoJSON.a \
# /usr/local/lib/libPocoNet.a
## POCO配置结束 ##
3.#include头文件
// poco,http解析json所需的头文件
#include "Poco/Net/HTMLForm.h"
#include "Poco/URI.h"
#include "Poco/Net/HTTPClientSession.h"
#include "Poco/Net/HTTPRequest.h"
#include "Poco/Net/HTTPResponse.h"
#include "Poco/StreamCopier.h"
#include "Poco/Net/NetException.h"
#include "Poco/JSON/Object.h"
#include "Poco/JSON/Parser.h"
#include "Poco/Dynamic/Var.h"
#include "Poco/ASCIIEncoding.h"
#include "Poco/UTF8Encoding.h"
#include "Poco/UTF16Encoding.h"
#include "Poco/TextConverter.h"
4.http发送请求及接受返回信息,并解析json
static bool getdata(const std::string &pointId,
const std::string &time1,
const std::string &time2,
const int &time_interval,
std::vector<double> &result,
std::vector<std::string> &time_sign,
const std::string &get_data_url)
{
if(get_data_url.empty()) //地址没有初始化
{
std::cout<< "model_status_url haven't been initialized right.";
return false;
}
Poco::URI url(get_data_url); // 第一步
Poco::Net::HTTPClientSession session(url.getHost(), url.getPort()); // 第二步
std::string path(url.getPath());
Poco::Net::HTTPRequest request(//Poco::Net::HTTPRequest::HTTP_POST,
Poco::Net::HTTPRequest::HTTP_GET,
path,
Poco::Net::HTTPMessage::HTTP_1_1); // 第三步
//请求头中内容
//request.add("Authorization1","123456789");
//请求数据
Poco::Net::HTMLForm htmlform;// 第四步
//htmlform.setEncoding(Poco::Net::HTMLForm::ENCODING_MULTIPART);//使用"multipart/form-data"编码
htmlform.set("pointIdList",pointId);
htmlform.set("starttime",time1);
htmlform.set("endtime",time2); // 第五步
htmlform.prepareSubmit(request); // 第六步
//发送请求
try{
std::ostream &output=session.sendRequest(request);
if(output.fail()==true){
//LOG(error) << "Sendrequest stream failed,reset now.";
session.reset();
}
htmlform.write(output); // 第七步
}
catch(Poco::Exception &e){
session.reset();
std::cout << "error00" << std::endl;
return false;
}
//得到返回信息
Poco::Net::HTTPResponse response;
try{
std::istream &stream = session.receiveResponse(response);
std::string temp_str;
std::ostringstream os;
os<<stream.rdbuf();
temp_str=os.str();//返回的是一个临时变量,不能直接用指针去接收
// 解析接收到json串
Poco::JSON::Parser parser;
Poco::Dynamic::Var json_result = parser.parse(temp_str);
Poco::JSON::Object::Ptr request_object1 = json_result.extract<Poco::JSON::Object::Ptr>(); // json对象
if(!request_object1->has("data")){
std::cout << "error0" << std::endl;
std::cout << "get point data failed:"<<pointId<<". In response,no key word "<<MyHTTPModelStatusKey::DATA.c_str();
return false;
}
Poco::JSON::Array::Ptr temp_point_array_ptr = request_object1->getArray("data"); // 该对象下的json数组
Poco::JSON::Array::Ptr point_array_ptr = temp_point_array_ptr->getArray(0); // json数组下的数组对象
int data_num = point_array_ptr->size();//从数据库得到的数据数量
int needed_data_num = result.size();//需要的数据数量
std::cout << needed_data_num<<std::endl;
//测试用,除以60是因为模拟的数据库中,数据间隔为60秒
int interval_temp = time_interval / 60;
if(interval_temp < 1)
interval_temp =1;
std::cout << interval_temp <<std::endl;
std::cout << "get data:"<<pointId<<","<<time1<<","<<time2<<" list size:"<<data_num<<std::endl;
std::string pointId;
Poco::JSON::Object::Ptr point_ptr;
//result.clear();
for(int i=0,j=0;i<data_num && j<needed_data_num;++i) //多加一个限制条件,为了确保所有测点获得的数据量相同
{
if(i%interval_temp == 0){
point_ptr = point_array_ptr->get(i).extract<Poco::JSON::Object::Ptr>(); // 循环提取json对象
time_sign[j] = point_ptr->get("time").toString();
result[j] = std::stod(point_ptr->get("value").toString());
pointId = point_ptr->get("pointId").toString();
std::cout << pointId;
++j;
}
}
std::cout<<"succeed in getting data.point:"<<pointId;
}
catch(Poco::Exception &e){
std::cout << ". receiveresponse:"<<e.what();
session.reset();
result.clear();//出错,返回空数组
time_sign.clear();
std::cout << "error1" << std::endl;
return false;
}
catch(...){
std::cout << "error2" << std::endl;
return false;
}
return true;
}
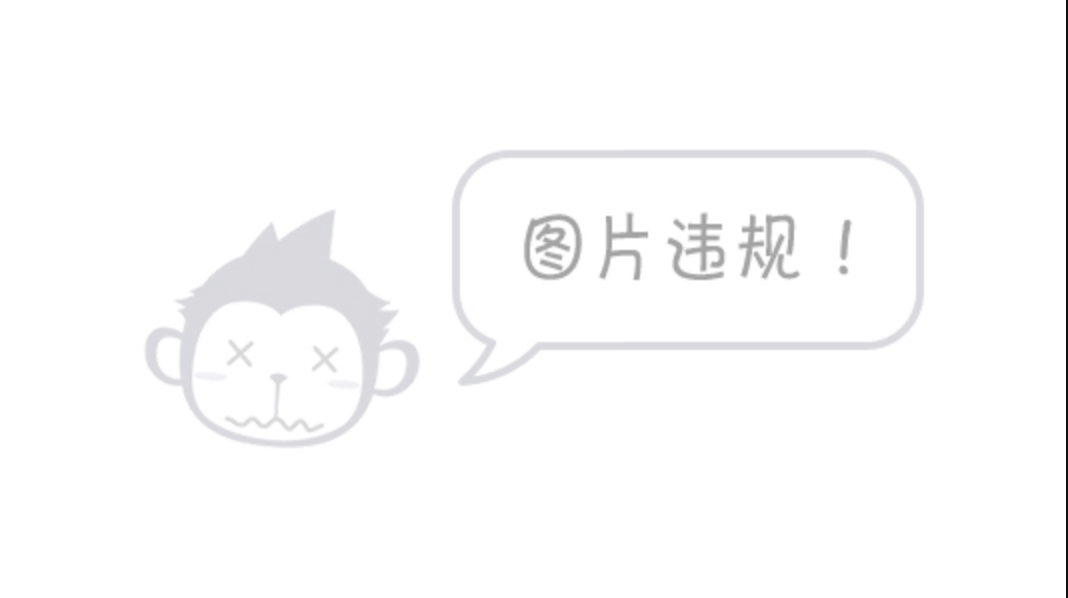
5.调用及实现
int main()
{
const std::string pointId = "PDSFD_1000_01_DJ_01_DT5d:test";
const std::string time1 = "2020-10-16 08:50:08";
const std::string time2 = "2020-10-16 12:50:08";
std::vector<double> result(10);
std::vector<std::string> time_sign(10);
const std::string get_data_url = "http://192.168.1.180:7999/";
const int time_interval=10;
bool res = getdata(pointId,time1,time2,time_interval,result,time_sign,get_data_url);
if (result[0] > 0)
{
std::cout << "yes" << res;
}
else
{
std::cout << "no" << res;
}
return 0;
}