1、父组件参数传递到子组件(父=>子)
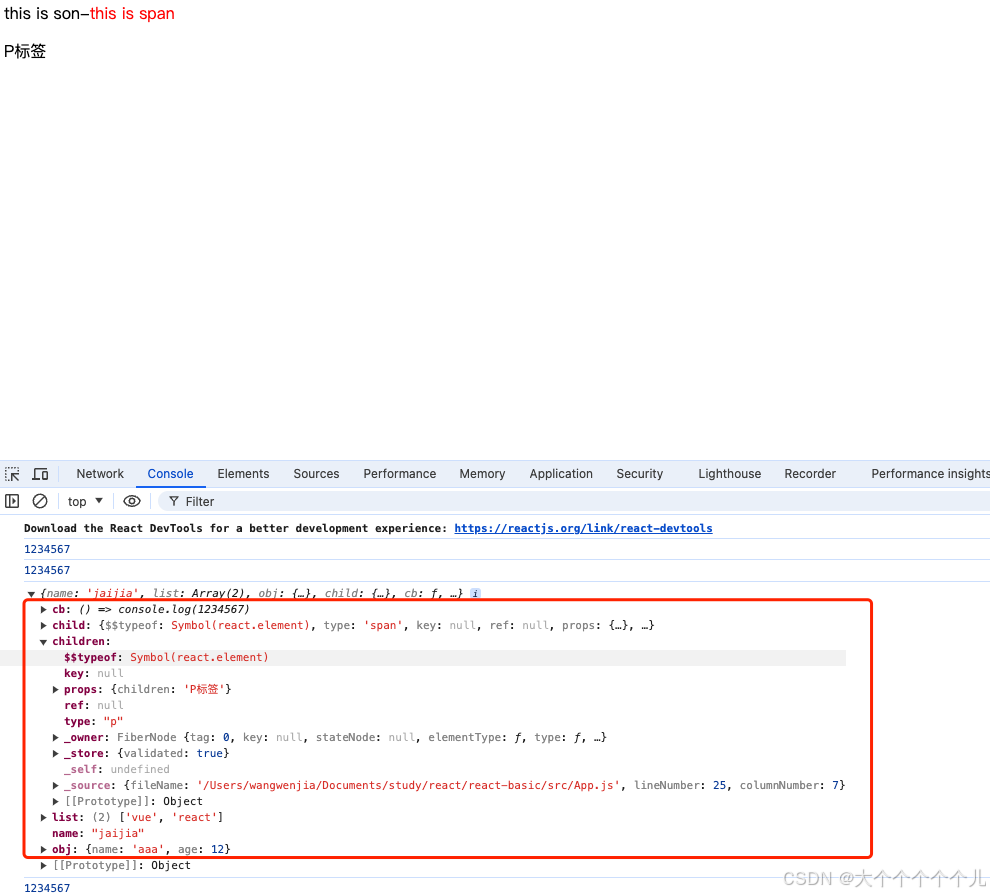
function Son(props){
console.log(props);
// props.name = 'hello';//props传递过来的属性是只读的,想修改的话去父组件修改;(简单理解:谁的东西谁修改)
return (
<div>
this is son{props.cb()}-{props.child}
<div>
{props.children}
</div>
</div>
)
}
function App(){
let name = 'jaijia'
return (
<Son
name={name}
list={['vue','react']}
obj={{name:'aaa',age:12}}
cb={()=>console.log(1234567)}
child={<span style={{color:'red'}}>this is span</span>}
>
<p>P标签</p>
</Son>
)
}
export default App
2、子组件参数传递到父组件(子=>父)
import { useState } from "react"
function Son({onGetMsg}){
const sonMsg = '我是子组件的消息'
return (
<div>
<button onClick={()=>{onGetMsg(sonMsg)}}>send</button>
</div>
)
}
// 在子组件中调用父组件中的函数并传递参数
function App(){
const [fatherMsg,setFatherMsg] = useState('')
const getMsg = (msg)=>{
console.log(msg);
setFatherMsg(msg)
}
return (
<div>
<Son onGetMsg={getMsg} />
{fatherMsg}
</div>
)
}
export default App
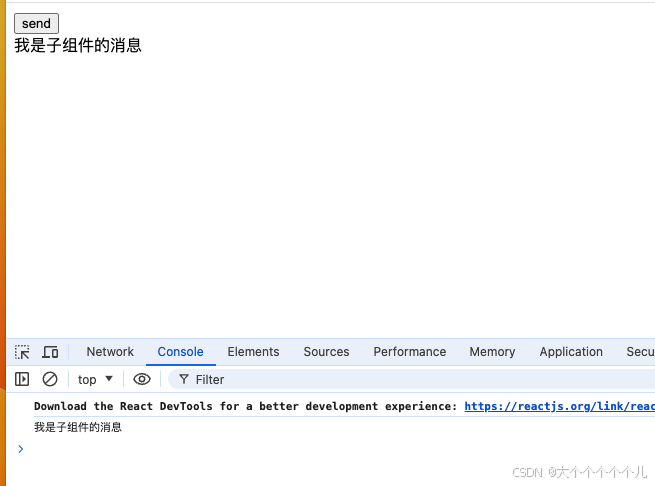