监控的意义
- 监控服务状态是否宕机
- 监控服务运行指标(内存、虚拟机、线程、请求等)
- 监控日志
- 管理服务(服务下线)
- 监控的实施方式
- 显示监控信息的服务器:用于获取服务信息,并显示对应的信息
- 运行的服务:启动时主动上报,告知监控服务器自己需要收到监控
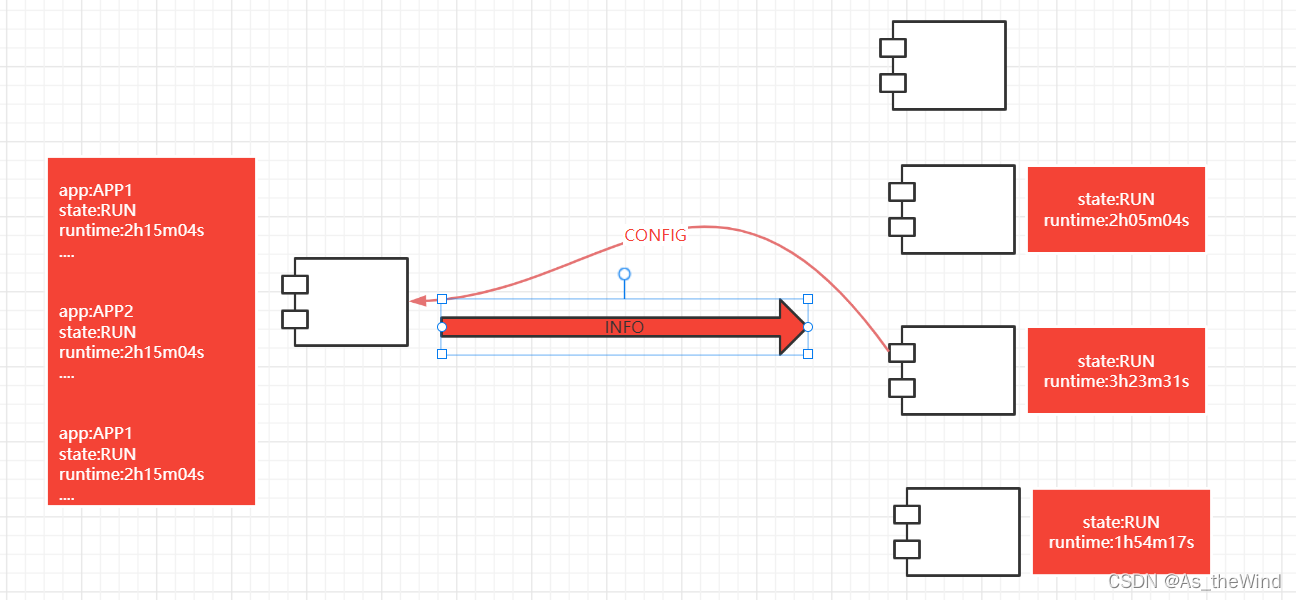
可视化监控平台
- SpringBoot Admin,开源社区项目,用于管理和监控springboot应用程序。客户端注册到服务器后,通过HTTP请求方式,服务端定期从客户端获取对应的信息,并通过UI界面展示对应信息。
- Admin服务端(与spring-boot-starter-parent,version版本一致)
<dependency>
<groupId>de.codecentric</groupId>
<artifactId>spring-boot-admin-starter-server</artifactId>
<version>2.6.6</version>
</dependency>
- Admin客户端(与spring-boot-starter-parent,version版本一致)
<dependency>
<groupId>de.codecentric</groupId>
<artifactId>spring-boot-admin-starter-client</artifactId>
<version>2.6.6</version>
</dependency>
server:
port: 8080
@SpringBootApplication
@EnableAdminServer
public class SpringBoot25AdminServerApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBoot25AdminServerApplication.class, args);
}
}
server:
port: 80
spring:
boot:
admin:
client:
url: http://localhost:8080
management:
endpoint:
health:
show-details: always
endpoints:
web:
exposure:
include: '*'
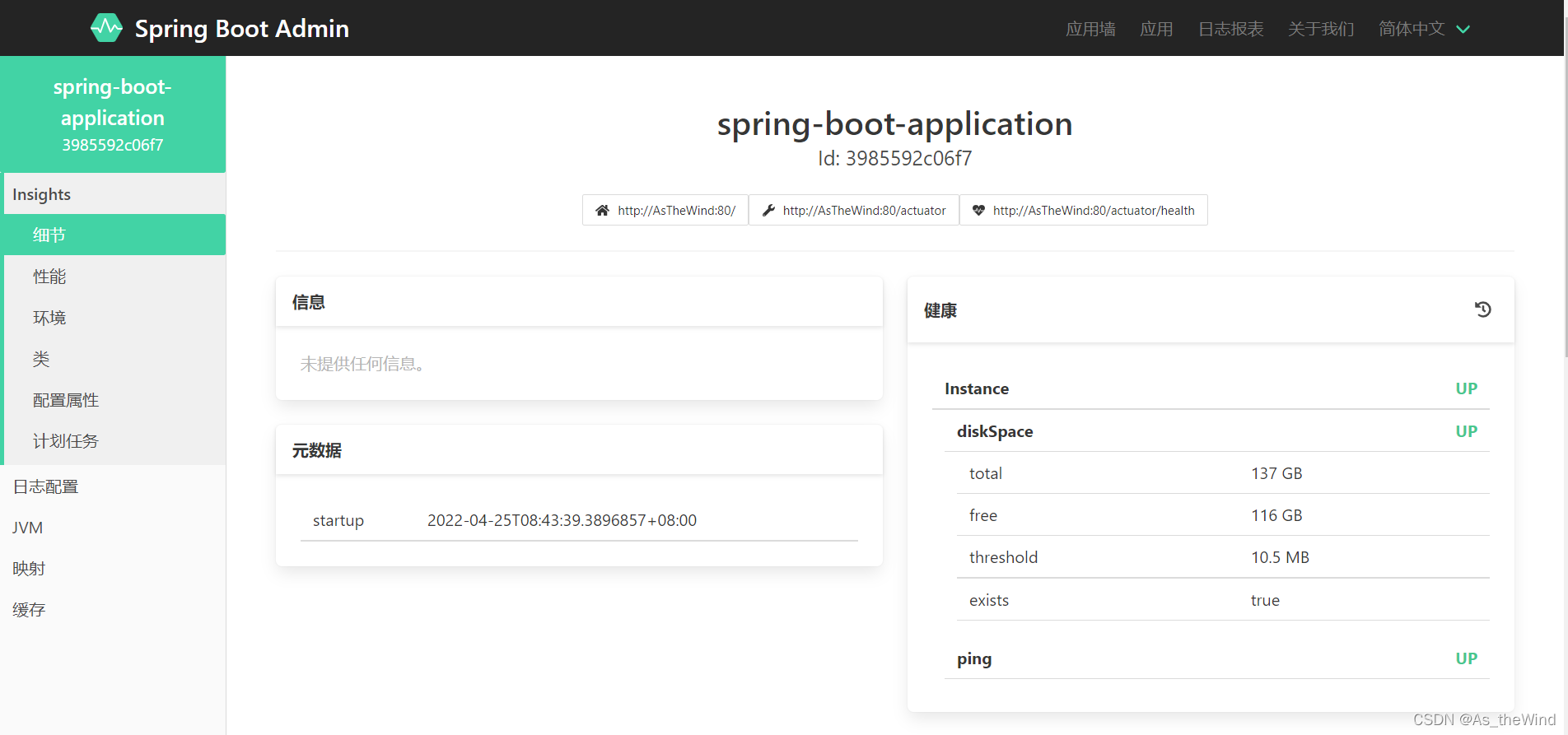
监控原理
Actuator
- Actuator提供了SpringBoot生产就绪功能,通过端点的配置与访问,获取端点信
- 端点描述了一组监控信息,SpringBoot提供了多个内置端点,也可以根据需要自定义端点信息
- 访问当前应用所有端点信息:
/actuator
- 访问端点详细信息:/actuator/
端点名称
端点功能开启与关闭
ID | 描述 | 默认启动 |
---|
auditevents | 暴露当前程序的审计事件信息 | 是 |
beans | 显示应用程序中所有Spring bean的完整列表 | 是 |
caches | 暴露可用的缓存 | 是 |
conditions | 显示在匹配和自动配置类上评估的条件以及它们匹配或不匹配的原因 | 是 |
configprops | 显示所有@ConfigurationProperties的校对清单 | 是 |
env | 暴露Spring ConfigurableEnvironment中的属性 | 是 |
flyway | 显示已应用的Flyway数据库迁移 | 是 |
health | 显示应用程序健康信息 | 是 |
httptrace | 显示HTTP追踪信息(默认情况下,最后100个HTTP请求/响应交换) | 是 |
info | 显示应用程序信息 | 是 |
integrationgraph | 显示Spring Integration图 | 是 |
loggers | 显示和修改应用程序中日志记录器的配置 | 是 |
liquibase | 显示已应用Liquibase数据库迁移 | 是 |
metrics | 显示当前应用程序的指标量信息 | 是 |
mappings | 显示所有@RequestMapping路径的整理清单 | 是 |
sessions | 允许从Spring Session支持会话存储中检索和删除用户会话。当使用Spring Seesion的响应式Web应用程序支持时不可用 | 是 |
shutdown | 正常关闭应用程序 | 否 |
threaddump | 执行线程dump | 是 |
heapdump | 返回一个hprof堆dump文件 | 是 |
jolokia | 通过HTTP暴露JMX bean(当Jolokia在classpath上时,不适用于WebFlux) | 是 |
logfile | 返回日志文件的内容(如果已设置logging.file或logging.path属性)。支持使用HTTP Range头来检索部分日志文件的内容 | 是 |
prometheus | 以可以由Prometheus服务器抓取的格式暴露指标 | 是 |
management:
endpoint:
health:
enabled: true
show-details: always
beans:
enabled: true
management:
endpoint:
enabled-by-default: true
端点功能暴露
- 暴露端点功能
♦ 端点中包含的信息存在敏感信息,需要对外暴露端点功能时手动设定指定端点信息
属性 | 默认 |
---|
management.endpoints.jmx.exposure.exclude | |
management.endpoints.jmx.exposure.include | * |
management.endpoints.web.exposure.exclude | |
management.endpoints.web.exposure.include | info,health |
ID | JMX | Web |
---|
auditevents | 是 | 否 |
beans | 是 | 否 |
caches | 是 | 否 |
conditions | 是 | 否 |
configprops | 是 | 否 |
env | 是 | 否 |
flyway | 是 | 否 |
health | 是 | 是 |
heapdump | N/A | 否 |
httptrace | 是 | 否 |
info | 是 | 是 |
integrationgraph | 是 | 否 |
jolokia | N/A | 否 |
logfile | N/A | 否 |
liquibase | 是 | 否 |
metrics | 是 | 否 |
mappings | 是 | 否 |
prometheus | N/A | 否 |
scheduledtasks | 是 | 否 |
sessions | 是 | 否 |
shutdown | 是 | 否 |
threaddump | 是 | 否 |
自定义监控指标
info:
appName: @project.artifactId@
version: @project.version@
author: itheima
@Component
public class infoConfig implements InfoContributor {
@Override
public void contribute(Info.Builder builder) {
builder.withDetail("runtime", System.currentTimeMillis())
.withDetail("company", "xxxx");
Map infoMap = new HashMap();
infoMap.put("buildTime", "2006");
builder.withDetails(infoMap);
}
}
@Component
public class HealthConfig extends AbstractHealthIndicator {
@Override
protected void doHealthCheck(Health.Builder builder) throws Exception {
boolean condition = true;
if (true) {
builder.withDetail("runtime", System.currentTimeMillis());
Map infoMap = new HashMap();
infoMap.put("buildTime", "2006");
builder.withDetails(infoMap);
} else {
builder.status(Status.DOWN);
builder.withDetail("上线了吗?", "你做梦");
builder.status(Status.UP);
}
}
}
@Service
public class BookServerImpl extends ServiceImpl<BookDao,Book> implements IBookService{
private Counter counter;
public BookServiceImpl(MeterRegistry meterRegistry){
counter = meterRegistry.counter("用户付费操作次数:");
}
@Override
public boolean delete(Integer id){
counter.increment();
return bookDao.deleteById(id) > 0;
}
}
@Component
@Endpoint(id = "pay", enableByDefault = true)
public class payEndpoint {
@ReadOperation
public Object getPay() {
Map payMap = new HashMap();
payMap.put("level 1", "300");
payMap.put("level 2", "291");
payMap.put("level 3", "666");
return payMap;
}
}