效果图如下:
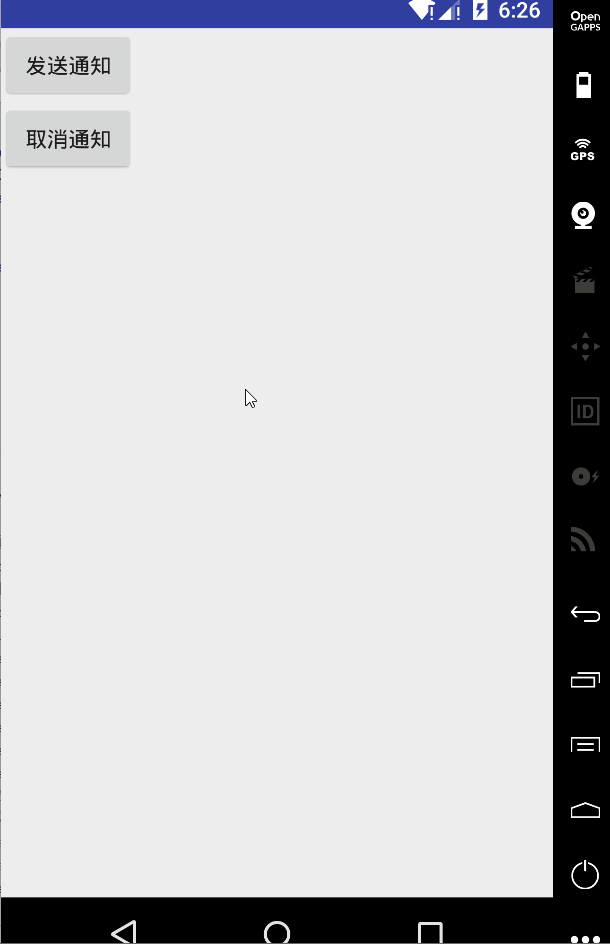
一、实验环境
- 开发软件:Android Studio 3.1.4
- 模拟器:Genymotion
二、实现
- 界面布局文件————activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:orientation="vertical">
<Button
android:id="@+id/bt_send"
android:layout_width="wrap_content"
android:layout_height&