程序清单3.1,platinum.c:
/* platinum.c -- your weight in platinum */
#include <stdio.h>
int main(void)
{
float weight; //你的体重
float value; // 相等重量的白金价值
printf("Are you worth your weight in platinum?\n");
printf("Let's check it out.\n");
printf("Please enter your weight in pounds:");
/* 获取用户的输入 */
scanf("%f", &weight);
/* 假设白金的价值是每蛊司 $1700 */
/* 14.5833 用于把英镑常衡蛊司转换为金衡蛊司 */
value = 1700.0 * weight * 14.5833;
printf("Your weight in platinum is worth $%.2f.\n", value);
printf("You are easily worth that!If platinum prices drop,\n");
printf("eat more to maintain your value.\n");
return 0;
}
输出结果:

程序清单3.2,print1.c:
/* print1.c -- 演示 printf() 的一些特性 */
#include <stdio.h>
int main(void)
{
int ten = 10;
int two = 2;
printf("Doing it right:");
printf("%d minus %d is %d\n", ten, 2, ten - two);
printf("Doing is wrong:");
printf("%d minus %d is %d\n", ten); // 遗漏 2 个参数
return 0;
}
输出结果:

程序清单3.3,bases.c:
/* bases.c -- 以十进制、八进制、十六进制打印十进制数 100 */
#include <stdio.h>
int main(void)
{
int x = 100;
printf("dec = %d; octal = %o; hex = %x\n", x, x, x);
printf("dec = %d; octal = %#o; hex = %#x\n", x, x, x);
return 0;
}
输出结果:

程序清单3.4,print2.c:
/* print2.c -- 更多 printf() 的特性 */
#include <stdio.h>
#include <windows.h>
int main(void)
{
unsigned int un = 3000000000; /* int 为 32 位和 short 为 16 位的系统 */
short end = 200;
long big = 65537;
long long verybig = 12345678908642;
printf("un = %u and not %d\n", un, un);
printf("end = %hd and %d\n", end, end);
printf("big = %ld and not %hd\n", big, big);
printf("verybig = %lld and not %ld\n", verybig, verybig);
printf("\007");
Sleep(1000);
printf("\a");
return 0;
}
输出结果:

程序清单3.4_2,test.c:
#include <stdio.h>
int main(void)
{
int i = 2147483647;
unsigned int j = 4294967295;
printf("%d %d %d\n", i, i+1, i+2);
printf("%u %u %u\n", j, j+1, j+2);
return 0;
}
输出结果:

程序清单3.5,charcode.c:
/* charcode.c -- 显示字符的代码编号 */
#include <stdio.h>
int main(void)
{
char ch;
printf("Please enter a character.\n");
scanf("%c", &ch); /* 用户输入字符 */
printf("The code for %c is %d.\n", ch, ch);
return 0;
}
输出结果:

程序清单3.6,altnames.c:
/* altnames.c -- 可移植整数类型名 */
#include <stdio.h>
#include <inttypes.h> // 支持可移植类型
int main(void)
{
int32_t me32; // me32 是一个 32 位有符号整型变量
me32 = 45933945;
printf("First, assume int32_t is int: ");
printf("me32 = %d\n", me32);
printf("Next, let's not make any assumptions.\n");
printf("Instead, use a \"macro\" from inttypes.h: ");
printf("me32 = %" PRId32 "\n", me32);
return 0;
}
输出结果:

程序清单3.7,showf_pt.c:
/* showf_pt.c -- 以两种方式显示 float 类型的值 */
#include <stdio.h>
int main(void)
{
float aboat = 32000.0;
double abet = 2.14e9;
long double dip = 5.32e-5;
printf("%f can be written %e\n", aboat, aboat);
printf("And it's %a in hexadecimal, powers of 2 notation\n", aboat);
printf("%f can be written %e\n", abet, abet);
printf("%Lf can be written %Le\n", dip, dip);
return 0;
}
输出结果(结果部分错误是由于编译器原因):

程序清单3.8,typesize.c:
/* typesize.c -- 打印类型大小 */
#include <stdio.h>
int main(void)
{
printf("Type int has a size of %zd bytes.\n", sizeof(int));
printf("Type char has a size of %zd bytes.\n", sizeof(char));
printf("Type long has a size of %zd bytes.\n", sizeof(long));
printf("Type long long has a size of %zd bytes.\n", sizeof(long long));
printf("Type double has a size of %zd bytes.\n", sizeof(double));
printf("Type long double has a size of %zd bytes.\n", sizeof(long double));
return 0;
}
输出结果:

程序清单3.9,badcount.c:
/* badcount.c -- 参数错误的情况 */
#include <stdio.h>
int main(void)
{
int n = 4;
int m = 5;
float f = 7.0f;
float g = 8.0f;
printf("%d\n", n, m); /* 参数太多 */
printf("%d %d %d\n", n); /* 参数太少 */
printf("%d %d\n", f, g); /* 值的类型不匹配 */
return 0;
}
输出结果:

程序清单3.10,escape.c:
/* escape.c -- 使用转移序列 */
#include <stdio.h>
int main(void)
{
float salary;
printf("\aEnter your desired monthly salary:"); /* 1 */
printf(" $_______\b\b\b\b\b\b\b"); /* 2 */
scanf("%f", &salary);
printf("\n\t$%.2f a month is $%.2f a year.", salary,
salary * 12); /* 3 */
printf("\rGee!\n"); /* 4 */
return 0;
}

编程练习
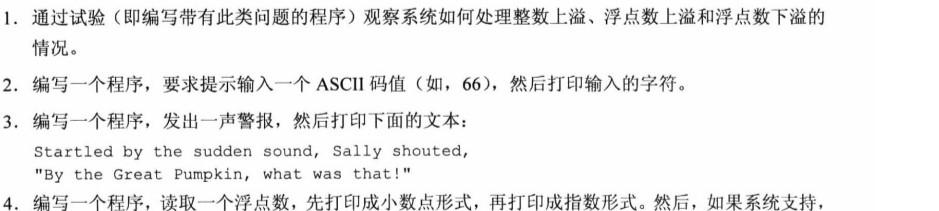
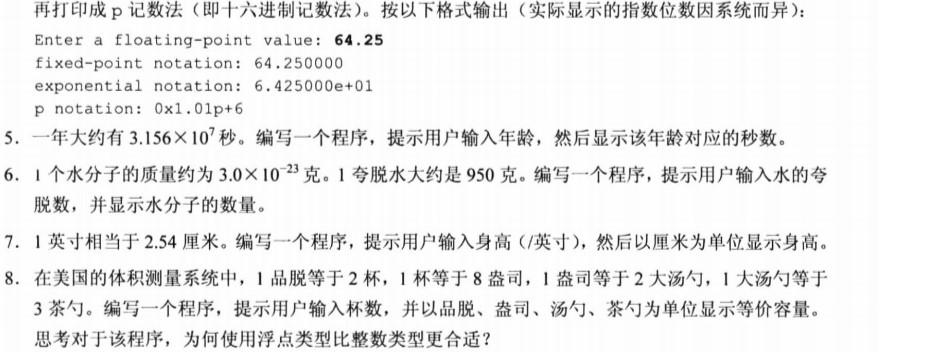
题目1,方法:测试浮点数溢出。示例代码3_1.c:
#include <stdio.h>
int main(void)
{
int int_max = 2147483647;
float float_max = 3.40e38;
float float_min = 3.40e-38;
printf("%d %d\n", int_max, int_max + 1);
printf("%f %f\n", float_max, float_max + 1);
printf("%f %f\n", float_min, float_min - 1);
return 0;
}
输出结果:

题目2,方法:输入ASCII码并打印字符。示例代码3_2.c:
#include <stdio.h>
int main(void)
{
int ASCII;
printf("Please input a ASCII code:__\b\b");
scanf("%d", &ASCII);
printf("The ASCII %d is %c\n", ASCII, ASCII);
return 0;
}
输出结果:

题目3,方法:打印语句。示例代码3_3.c:
#include <stdio.h>
int main(void)
{
printf("\aStarted by the sudden sound, Sally shouted,\n");
printf("\"By the great Pumpkin, what was that!\"");
return 0;
}
输出结果:

题目4,方法:以指定形式打印浮点数。示例代码3_4.c:
#include <stdio.h>
int main(void)
{
float n;
printf("Enter a floating-value: ");
scanf("%f", &n);
printf("fixed-point notation: %f\n", n);
printf("exponential notation: %e\n", n);
printf("p notation: %#a\n", n);
return 0;
}
输出结果:

题目5,方法:计算并打印数值。示例代码3_5.c:
#include <stdio.h>
int main(void)
{
int age;
float second = 3.156e7;
printf("Please input your age: ");
scanf("%d", &age);
printf("It has been %f seconds since you come to the world.\n", age * second);
return 0;
}
输出结果:

题目6,计算并输出数值。示例代码3_6.c:
#include <stdio.h>
int main(void)
{
int quart;
double weight = 3.0e-23;
printf("Please input the number of quart of the water: ");
scanf("%d", &quart);
printf("%d quarts of water include %f water molecules.",
quart, quart * 950 / weight);
return 0;
}
输出结果:

题目7,方法:计算并输出数值。示例代码3_7.c:
#include <stdio.h>
int main(void)
{
int height;
printf("Please intput your height with inch: ") ;
scanf("%d", &height);
printf("Your height is %f centimetres.", height * 2.54);
return 0;
}
输出结果:

题目8,计算方法:计算并输出数值。示例代码3_8.c:
#include <stdio.h>
int main(void)
{
float cups;
printf("Please input the number of the cups: ");
scanf("%f", &cups);
printf("%.2f cups equals to %.2f pints.\n", cups, cups / 2);
printf("%.2f cups equals to %.2f ounces.\n", cups, cups * 8);
printf("%.2f cups equals to %.2f soup ladles.\n", cups, cups * 8 * 2);
printf("%.2f cups equals to %.2f tea ladles.\n", cups, cups * 8 * 2 * 3);
return 0 ;
}
输出结果:
