1 代码
#include<bits/stdc++.h>
#define max 1000
#define num 100
using namespace std;
typedef struct TBNode
{
int data;
int ltag, rtag;
struct TBNode *lchild, *rchild;
}*TBLink, TBNode;
void creat();
void creat0(TBLink &L);
void creatTree(TBLink &L, int &n, int length);
void creat1(TBLink &L);
void precreat(TBLink &L, TBLink &pre);
void preorder(TBLink L);
void preorder3(TBLink L);
void creat2(TBLink &L);
void increat(TBLink &L, TBLink &pre);
void inorder(TBLink L);
void inorder3(TBLink L);
TBLink next(TBLink L);
TBLink first(TBLink L);
void creat3(TBLink &L);
void postcreat(TBLink &L, TBLink &pre);
void postorder(TBLink L);
void postorder3(TBLink L);
int main()
{
srand(time(NULL));
creat();
system("pause");
return 0;
}
void creat()
{
TBLink L;
L = (TBNode *)calloc(1, sizeof(TBNode));
creat0(L);
creat1(L);
cout << "前序遍历 - 递归:" << endl;
preorder(L);
cout << endl << endl;
cout << "中序遍历 - 递归:" << endl;
inorder(L);
cout << endl << endl;
cout << "后序遍历 - 递归:" << endl;
postorder(L);
cout << endl << endl;
cout << "前序遍历:" << endl;
preorder3(L);
cout << endl << endl;
L = (TBNode *)calloc(1, sizeof(TBNode));
creat0(L);
creat2(L);
cout << "前序遍历 - 递归:" << endl;
preorder(L);
cout << endl << endl;
cout << "中序遍历 - 递归:" << endl;
inorder(L);
cout << endl << endl;
cout << "后序遍历 - 递归:" << endl;
postorder(L);
cout << endl << endl;
cout << "中序遍历:" << endl;
inorder3(L);
L = (TBNode *)calloc(1, sizeof(TBNode));
creat0(L);
creat3(L);
cout << "前序遍历 - 递归:" << endl;
preorder(L);
cout << endl << endl;
cout << "中序遍历 - 递归:" << endl;
inorder(L);
cout << endl << endl;
cout << "后序遍历 - 递归:" << endl;
postorder(L);
cout << endl << endl;
cout << "后序遍历:" << endl;
postorder3(L);
}
void creat0(TBLink &L)
{
int t,length = rand()%num;
cout << "随机生成 " << length << "个元素" << endl;
if(length > 0)
{
L = (TBNode *)calloc(1, sizeof(TBNode));
L->data = rand()%max;
t = 0;
creatTree(L->lchild, t, (length-1)/2);
t = 0;
creatTree(L->rchild, t, length-1-(length-1)/2);
cout << "创建成功!" << endl << endl;
}
else
{
L = NULL;
cout << "树为空!" << endl << endl;
}
}
void creatTree(TBLink &L, int &n, int length)
{
if(n < length)
{
TBLink p;
p = (TBNode *)calloc(1, sizeof(TBNode));
p->data = rand()%max;
L = p;
n++;
creatTree(L->lchild, n, length);
creatTree(L->rchild, n, length);
}
}
void creat1(TBLink &L)
{
cout << "尝试前序线索化..." << endl ;
if(L != NULL)
{
TBLink pre = NULL;
precreat(L, pre);
pre->rchild = NULL;
pre->rtag = 1;
cout << "成功!" << endl << endl;
}
else
cout << "树为空!" << endl << endl;
}
void precreat(TBLink &L, TBLink &pre)
{
if(L != NULL)
{
if(L->lchild == NULL)
{
L->lchild = pre;
L->ltag = 1;
}
if(pre != NULL && pre->rchild == NULL)
{
pre->rchild = L;
pre->rtag = 1;
}
pre = L;
if(L->ltag == 0)
precreat(L->lchild, pre);
if(L->rtag == 0)
precreat(L->rchild, pre);
}
}
void preorder(TBLink L)
{
if(L != NULL)
{
cout << L->data << " ";
if(L->ltag == 0)
preorder(L->lchild);
if(L->rtag == 0 )
preorder(L->rchild);
}
}
void preorder3(TBLink L)
{
if(L != NULL)
{
TBLink p;
p = L;
while(p != NULL)
{
while(p->ltag == 0)
{
cout << p->data << " ";
p = p->lchild;
}
cout << p->data << " ";
p = p->rchild;
}
}
}
void creat2(TBLink &L)
{
cout << "尝试中序线索化..." << endl ;
if(L != NULL)
{
TBLink pre = NULL;
increat(L, pre);
pre->rchild = NULL;
pre->rtag = 1;
cout << "成功!" << endl << endl;
}
else
cout << "树为空!" << endl << endl;
}
void increat(TBLink &L, TBLink &pre)
{
if(L != NULL)
{
increat(L->lchild, pre);
if(L->lchild == NULL)
{
L->lchild = pre;
L->ltag = 1;
}
if(pre != NULL && pre->rchild == NULL)
{
pre->rchild = L;
pre->rtag = 1;
}
pre = L;
increat(L->rchild, pre);
}
}
void inorder(TBLink L)
{
if(L != NULL)
{
if(L->ltag==0)
inorder(L->lchild);
cout << L->data << " " ;
if(L->rtag==0)
inorder(L->rchild);
}
}
TBLink first(TBLink L)
{
while(L->ltag == 0)
L = L->lchild;
return L;
}
TBLink next(TBLink L)
{
if(L->rtag == 1)
return L->rchild;
else
return first(L->rchild);
}
void inorder3(TBLink L)
{
TBLink p;
for(p = first(L); p != NULL; p = next(p))
cout << p->data << " " ;
cout << endl << endl;
}
void creat3(TBLink &L)
{
cout << "尝试后序线索化..." << endl ;
if(L != NULL)
{
TBLink pre = NULL;
postcreat(L, pre);
if(L->rchild == NULL)
{
L->rtag = 1;
}
cout << "成功!" << endl << endl;
}
else
cout << "树为空!" << endl << endl;
}
void postcreat(TBLink &L, TBLink &pre)
{
if(L != NULL)
{
postcreat(L->lchild,pre);
postcreat(L->rchild,pre);
if(L->lchild == NULL)
{
L->lchild = pre;
L->ltag = 1;
}
if(pre != NULL && pre->rchild == NULL)
{
pre->rchild = L;
pre->rtag = 1;
}
pre = L;
}
}
void postorder(TBLink L)
{
if(L != NULL)
{
if(L->ltag == 0)
postorder(L->lchild);
if(L->rtag == 0)
postorder(L->rchild);
cout << L->data << " ";
}
}
void postorder3(TBLink L)
{
if(L != NULL )
{
cout << "待续..." << endl << endl;
}
}
2 运行结果
2.1 前序线索二叉树
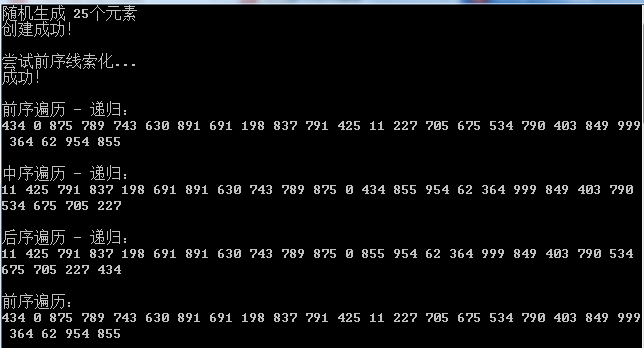
2.2 中序线索二叉树
2.3 后序线索二叉树