public class DownPOIUtils {
public static void downPoi(HttpServletResponse response,Map<Student, String> map) throws Exception {
String fname = "detial" + getTimeStamp();
OutputStream os = response.getOutputStream();
response.reset();
response.setHeader("Content-disposition", "attachment; filename="
+ fname + ".xls");
response.setContentType("application/msexcel");
try {
new DownPOIUtils().new POIS().createFixationSheet(os, map);
} catch (Exception e) {
e.printStackTrace();
}
}
public static String getTimeStamp() {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd hh:MM:ss");
Date date = new Date();
return dateFormat.format(date);
}
class POIS {
public void createFixationSheet(OutputStream os,Map<Student, String> student) throws Exception {
HSSFWorkbook wb = new HSSFWorkbook();
HSSFSheet sheet = wb.createSheet();
HSSFRow row = sheet.createRow((short) 0);
sheet.createFreezePane(0, 1);
cteateCell(wb, row, (short) 0, "学号");
cteateCell(wb, row, (short) 1, "姓名");
cteateCell(wb, row, (short) 2, "性别");
cteateCell(wb, row, (short) 3, "班级");
cteateCell(wb, row, (short) 4, "分数");
int i = 0;
Set<Student> keySet = student.keySet();
Iterator<Student> iterator = keySet.iterator();
while (iterator.hasNext()) {
HSSFRow rowi = sheet.createRow((short) (++i));
Student student2 = iterator.next();
for (int j = 0; j < 4; j++) {
cteateCell(wb, rowi, (short) 0, student2.getId());
cteateCell(wb, rowi, (short) 1, student2.getName());
cteateCell(wb, rowi, (short) 2, student2.getSex());
cteateCell(wb, rowi, (short) 3, student2.getGrade());
cteateCell(wb, rowi, (short) 4, student.get(student2));
}
}
wb.write(os);
os.flush();
os.close();
System.out.println("文件生成");
}
@SuppressWarnings("deprecation")
private void cteateCell(HSSFWorkbook wb, HSSFRow row, short col,String val) {
HSSFCell cell = row.createCell(col);
cell.setCellValue(val);
HSSFCellStyle cellstyle = wb.createCellStyle();
cellstyle.setAlignment(HSSFCellStyle.ALIGN_CENTER_SELECTION);
cell.setCellStyle(cellstyle);
}
}
}
<li onclick="printMonthOrder()" style="background-color: #db9907;"><span class="btn_insert">导出Excel</span></li>
function printMonthOrder(){
var type=$("#type").textbox('getValue');
var orgId=$("#orgId").textbox('getValue');
var beginDT=$("#beginDT").textbox('getValue')==""?null:$("#beginDT").textbox('getValue');
var endDT=$("#endDT").textbox('getValue')==""?null:$("#endDT").textbox('getValue');
var userId=$("#userId").textbox('getValue')==""?null:$("#userId").textbox('getValue');
window.location.href = _ctx+"/rest/checkorder/DownloadExcel/"+type+"/"+orgId+"/"+beginDT+"/"+endDT+"/"+userId;
}
@Controller
@RequestMapping("checkorder")
public class CheckOrderController extends BaseController {
@RequestMapping(value="DownloadExcel/{type}/{orgId}/{beginDT}/{endDT}/{userId}")
@ResponseBody
public void DownloadExcel(HttpServletRequest request,HttpServletResponse response,@PathVariable() Integer type,@PathVariable() String orgId,
@PathVariable() String beginDT,@PathVariable() String endDT,@PathVariable() String userId) throws Exception{
List<Maxterms> titleList = manager.findForColumns(type);
List<MonthSort> sorts = manager.getByMonthAndUser(beginDT.equals("null")?null:beginDT,endDT.equals("null")?null:endDT,
orgId, type,userId.equals("null")?null:userId);
DownPOIUtils.downPoi(response, titleList,sorts);
}
}
public class DownPOIUtils {
public static void downPoi(HttpServletResponse response,List<Maxterms> titleList,List<MonthSort> sorts) throws Exception {
String fname = "MonthOrder" + getTimeStamp();
OutputStream os = response.getOutputStream();
response.reset();
response.setHeader("Content-disposition", "attachment; filename="
+ fname + ".xls");
response.setContentType("application/msexcel");
try {
new DownPOIUtils().new POIS().createFixationSheet(os, titleList,sorts);
} catch (Exception e) {
e.printStackTrace();
}
}
public static String getTimeStamp() {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date date = new Date();
return dateFormat.format(date);
}
class POIS {
public void createFixationSheet(OutputStream os,List<Maxterms> titleList,List<MonthSort> sorts) throws Exception {
HSSFWorkbook wb = new HSSFWorkbook();
HSSFSheet sheet = wb.createSheet();
for(int i = 3;i<titleList.size()+3;i++){
sheet.setColumnWidth(i, 4000);
}
HSSFRow row = sheet.createRow((short) 0);
sheet.createFreezePane(0, 1);
cteateCell(wb, row, (short) 0, "姓名");
cteateCell(wb, row, (short) 1, "职务");
cteateCell(wb, row, (short) 2, "日期");
for(int i = 3;i<titleList.size()+3;i++){
cteateCell(wb, row, (short)i, titleList.get(i-3).getCheckMaxterm().getName()+titleList.get(i-3).getType());
}
cteateCell(wb, row, (short)(titleList.size()+3), "得分");
for(int i=0;i<sorts.size();i++){
if(sorts.get(i)!=null){
MonthSort monthSort = sorts.get(i);
HSSFRow rowi = sheet.createRow((short)i+1);
cteateCell(wb, rowi, (short) 0, monthSort.getUser().getUsername());
cteateCell(wb, rowi, (short) 1, monthSort.getUser().getDescription());
cteateCell(wb, rowi, (short) 2, monthSort.getDate());
List<UserSortForMaxterm> item = monthSort.getUserSorts();
for(int j =0;j<item.size();j++){
if(item.get(j).getOrders()==null){
cteateCell(wb, rowi, (short)(j+3),"/");
}else{
List<CheckOrderVo> vo = item.get(j).getOrders();
String s = "";
for(CheckOrderVo voi:vo){
s += voi.getCheckItem().getItem()+"("+voi.getCheckItem().getSort()+")分。";
}
cteateCell(wb, rowi, (short)(j+3),s);
}
}
cteateCell(wb, rowi, (short)(item.size()+3), monthSort.getSort());
}
}
wb.write(os);
os.flush();
os.close();
System.out.println("文件生成");
}
@SuppressWarnings("deprecation")
private void cteateCell(HSSFWorkbook wb, HSSFRow row, short col,String val) {
HSSFCell cell = row.createCell(col);
cell.setCellValue(val);
}
}
}
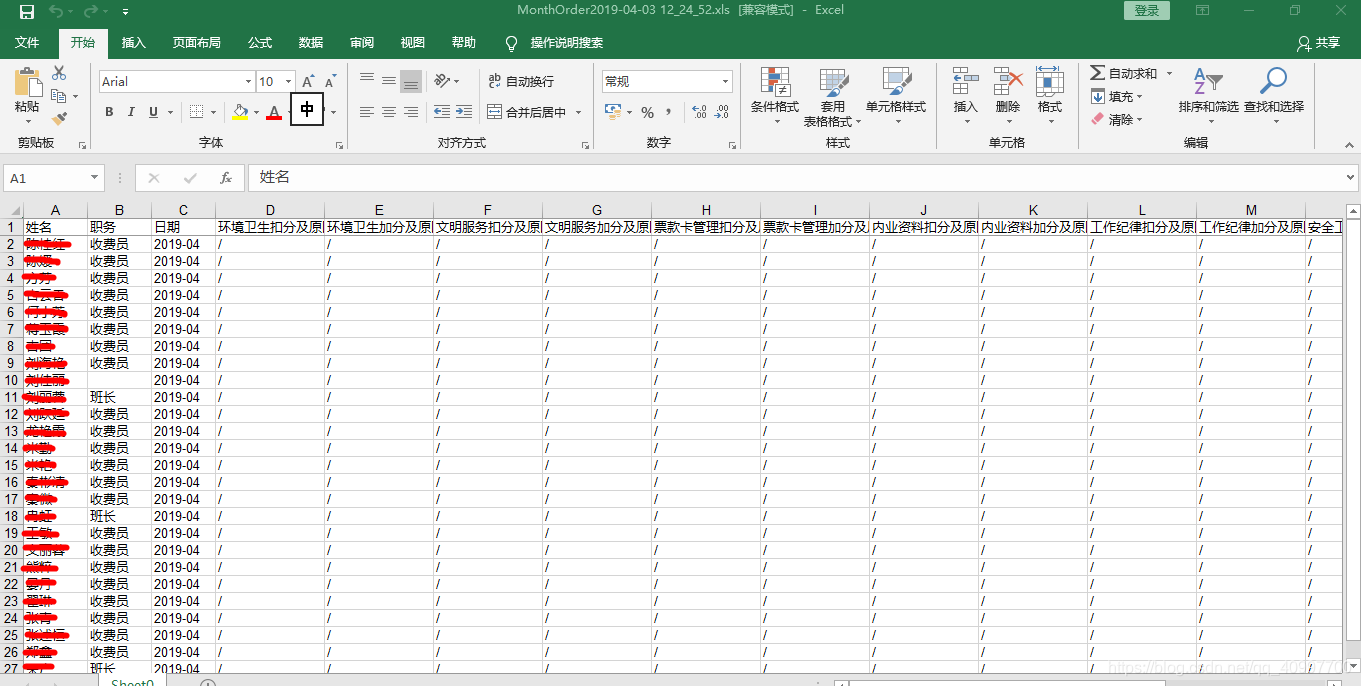