Libreoffice Online实现文件在线预览
1.Docker中安装配置Libreoffice Online
1. 拉取docker镜像:
a) docker search libreoffice/online
b) docker pull libreoffice/online:master
2. 创建并启动LibreOffice Online 服务
a) docker run -e TZ="Asia/Shanghai" --restart always --name libreoffice -d -p 9980:9980 -e "username=root" -e "password=123456" -e "extra_params=--o:ssl.enable=false --o:net.post_allow.host[0]=.\{1,99\}" libreoffice/online:master修改容器中loolwsd配置文件
b) 进去docker : docker exec -it --user root id /bin/bash
c) 禁用SSL 加密传输:(其默认是True,开启)
修改配置文件 /etc/loolwsd/loolwsd.xml
3. 宿主机防火墙放行对应端口
a) firewall-cmd --add-port=9980/tcp –permanent
b) systemctl restart firewalld
c) 查看放行的端口: firewall-cmd --zone=public --list-ports
4. 验证Libre Office Online 安装:
a) 访问: http://IP:(docker映射出的端口) ,返回OK,即为安装成功;
b) 直接访问:http://IP: (docker映射出的端口) /loleaflet/dist/admin/admin.html, 出现xml内容,证明软件安装成功
5. Libreoffice容器内使用curl http://IP: (docker映射出的端口) 连接成功即可
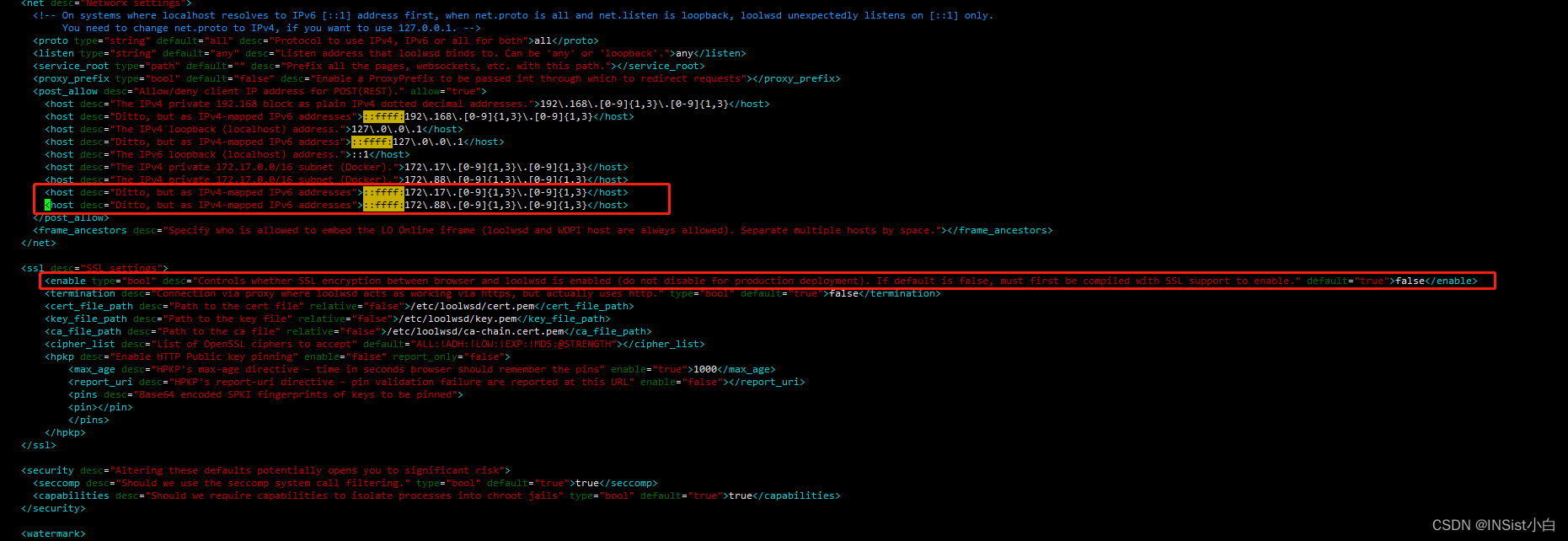
2.Maven中添加
<properties>
<libreoffice.version>5.4.2</libreoffice.version>
</properties>
<dependency>
<groupId>org.libreoffice</groupId>
<artifactId>juh</artifactId>
<version>${libreoffice.version}</version>
</dependency>
<dependency>
<groupId>org.libreoffice</groupId>
<artifactId>jurt</artifactId>
<version>${libreoffice.version}</version>
</dependency>
<dependency>
<groupId>org.libreoffice</groupId>
<artifactId>ridl</artifactId>
<version>${libreoffice.version}</version>
</dependency>
<dependency>
<groupId>org.libreoffice</groupId>
<artifactId>unoil</artifactId>
<version>${libreoffice.version}</version>
</dependency>
<dependency>
<groupId>org.jodconverter</groupId>
<artifactId>jodconverter-online</artifactId>
<version>4.2.4</version>
</dependency>
3.业务处理
FileAttribute fileAttribute = new FileAttribute();
File inputFile = new File(url);
if (!inputFile.exists()){
throw new BaseException("预览文件不存在");
}
String url2OutFilePath = StringUtils.replaceOnce(url, "upload-dir", "temp-file");
String outFilePathStr = StringUtils.substringBeforeLast(url2OutFilePath,".")+".pdf";
fileAttribute.setUrl(outFilePathStr);
File outputFilePath = new File(outFilePathStr);
if (outputFilePath.exists()) {
result.setResult(fileAttribute);
return result;
} else {
if (!outputFilePath.getParentFile().exists() && !outputFilePath.getParentFile().mkdirs()) {
log.error("创建目录【{}】失败,请检查目录权限!",url2OutFilePath);
}
final OnlineOfficeManager onlineOfficeManager = OnlineOfficeManager.make(libreofficeServer);
try {
onlineOfficeManager.start();
OnlineConverter.builder().officeManager(onlineOfficeManager).build()
.convert(inputFile).to(outputFilePath).execute();
onlineOfficeManager.stop();
}catch (Exception e){
log.error("文件转换异常信息:"+e);
throw new BaseException("文件转换失败,请下载后查看");
}finally {
if (onlineOfficeManager!=null){
try {
onlineOfficeManager.stop();
} catch (OfficeException e) {
e.printStackTrace();
}
}
}
}
result.setResult(fileAttribute);
return result;