一:我们先来看接口里面的数据怎么样的
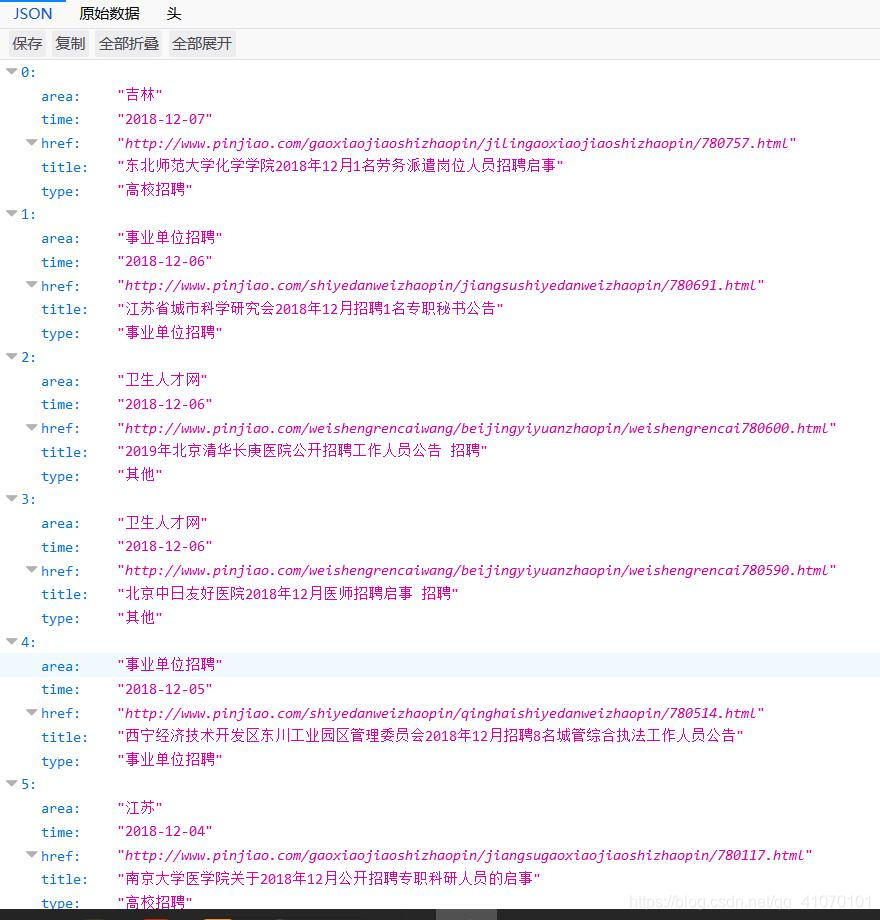
在FireFox浏览器上能很简明的显示接口数据
2.xml 布局文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="刷新"
android:gravity="center"
android:layout_gravity="center"
android:onClick="getJson"
/>
<ListView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:orientation="vertical"
android:id="@+id/Lv_main_list"
>
</ListView>
</LinearLayout>
3. 安卓的Http的GET请求将获取的对象数组,并解析,存到一个实体类里面
3.1 我们先来生成一个实体类
AndroidStudio 可以用GsonFormat轻松生成一个实体类
File>Settings>Plugins>Browse repositories
然后重启下AS 新建一个Class 用ALT+S弹出
AS就会自动生成一个实体类
3.2 MainActivity 代码
package com.example.administrator.bijian;
import android.app.ProgressDialog;
import android.os.AsyncTask;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.LinearLayout;
import android.widget.ListView;
import android.widget.TextView;
import com.alibaba.fastjson.JSON;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private ListView Lv_main_list;
private List<JsonTeacherEntity> TeacherList = new ArrayList<JsonTeacherEntity>();
private ProgressDialog progressDialog;
private MyAdapter myAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Lv_main_list =(ListView) findViewById(R.id.Lv_main_list);
//实例化进度条对话框
progressDialog = new ProgressDialog(this);
progressDialog.setMessage("亲,正在玩命加载中哦!");
实例化适配器
myAdapter = new MyAdapter();
Lv_main_list.setAdapter(myAdapter);
// Utility.setListViewHeightBasedOnChildren(Lv_main_list);
}
//适配器
class MyAdapter extends BaseAdapter{
@Override
public int getCount() {
return TeacherList.size();
}
@Override
public Object getItem(int i) {
return TeacherList.get(i);
}
@Override
public long getItemId(int i) {
return i;
}
@Override
public View getView(int i, View view, ViewGroup viewGroup) {
LinearLayout layout = new LinearLayout(MainActivity.this);
// layout.setOrientation(LinearLayout.HORIZONTAL);
layout.setOrientation(LinearLayout.VERTICAL);
TextView textViewArea = new TextView(MainActivity.this);
textViewArea.setText(TeacherList.get(i).getArea()+"\t"+" ");
TextView textViewTime = new TextView(MainActivity.this);
textViewTime.setText(TeacherList.get(i).getTime()+"\t");
// textViewArea.setTextSize(20);
TextView textViewHref = new TextView(MainActivity.this);
textViewHref.setText(TeacherList.get(i).getHref()+"\t");
TextView textViewTitle = new TextView(MainActivity.this);
textViewTitle.setText(TeacherList.get(i).getTitle()+"\t");
TextView textViewType = new TextView(MainActivity.this);
textViewType.setText(TeacherList.get(i).getType()+"\t");
layout.addView(textViewArea);
layout.addView(textViewTime);
layout.addView(textViewHref);
layout.addView(textViewTitle);
layout.addView(textViewType);
return layout;
}
}
//获取xml数据
public void getJson(View view){
new MyTask().execute();
}
//通过异步任务类获取数据
class MyTask extends AsyncTask{
private JsonTeacherEntity jsonTeacherEntity;
//准备执行
@Override
protected void onPreExecute() {
super.onPreExecute();
progressDialog.show();
}
@Override
protected Object doInBackground(Object[] objects) {
String path="接口URL";
try {
URL url = new URL(path);
//获取连接对象
HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection();
//设置请求方式
httpURLConnection.setRequestMethod("GET");
//设置连接超时
httpURLConnection.setConnectTimeout(5000);
//获取响应码
int code = httpURLConnection.getResponseCode();
if (code==200)
{
//响应成功,获取服务器返回过来的数据
final InputStream is = httpURLConnection.getInputStream();
//测试数据
StringBuffer stringBuffer = new StringBuffer();
String str=null;
BufferedReader br = new BufferedReader(new InputStreamReader(is));
while ((str=br.readLine())!=null){
stringBuffer.append(str);
}
//03.使用fastJson解析Json、
List<JsonTeacherEntity> Teachers = JSON.parseArray(stringBuffer.toString(), JsonTeacherEntity.class);
for (JsonTeacherEntity TT:Teachers)
{
jsonTeacherEntity = new JsonTeacherEntity();
String area = TT.getArea();
String href = TT.getHref();
String time = TT.getTime();
String title = TT.getTitle();
String type = TT.getType();
jsonTeacherEntity.setArea(area);
jsonTeacherEntity.setHref(href);
jsonTeacherEntity.setTime(time);
jsonTeacherEntity.setTitle(title);
jsonTeacherEntity.setType(type);
TeacherList.add(jsonTeacherEntity);
}
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
@Override
protected void onPostExecute(Object o) {
super.onPostExecute(o);
//通知适配器发生改变
myAdapter.notifyDataSetChanged();
//取消进度条对话框
progressDialog.cancel();
}
}
}
显示的效果