上一节对“我”模块(一)进行了综述(可参见 “我”模块(一) 进行了解),接下来将从“我”模块(二)开始详细介绍:
知识点
- 掌握“日历”界面的开发,使用日历展示当前年份
- 掌握“星座”界面的开发,选择不同的星座展示不同的运势
- 掌握“涂鸦”界面的开发,实现图画的绘制功能
- 掌握“地图”界面的开发,可以定位一个指定地点
星座
任务综述:
点击“星座”界面右上角的“切换”按钮会弹出“星座选择”界面,该界面主要用于展示十二星座的图标、名称、阳历日期,点击“星座选择”界面上的任意一个星座,会显示对应星座的详细信息。
7. “星座选择”界面
任务分析:
“星座选择”界面主要用于展示十二星座的相关信息,界面效果如图所示。
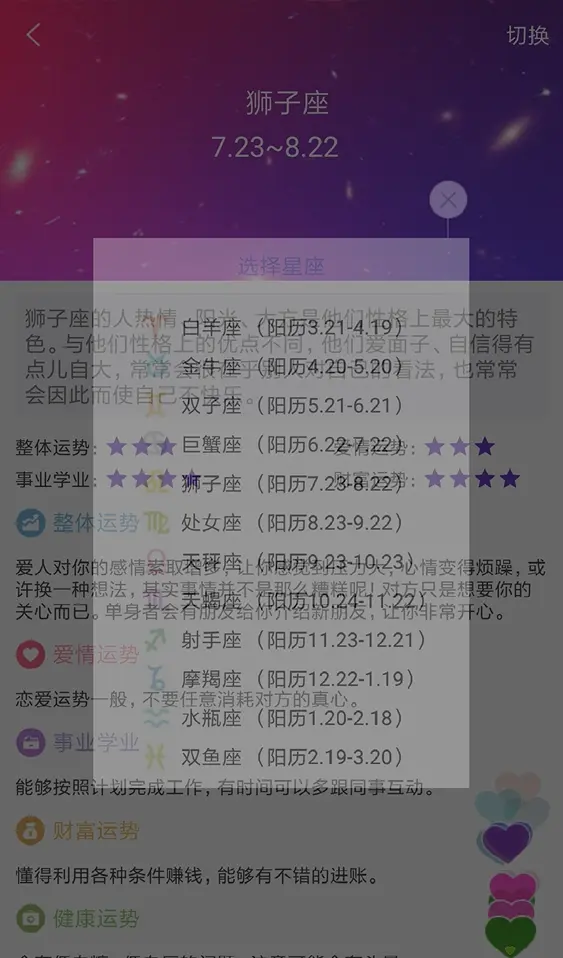
任务实施:
(1)创建“星座选择”界面:ChooseConstellationActivity & activity_choose_constellation。
(2)导入界面图片(choose_constella_close_icon)。
(3)放置界面控件。
一个ImageView控件用于显示关闭按钮;
一个TextView控件用于显示“选择星座”文字;
一个View控件用于显示灰色分割线;
一个RecyclerView控件用于显示十二星座的列表信息。
activity_choose_constellation.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/transparent"
android:orientation="vertical">
<ImageView
android:id="@+id/iv_close"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="right"
android:src="@drawable/choose_constella_close_icon" />
<LinearLayout
android:layout_width="240dp"
android:layout_height="match_parent"
android:background="@android:color/white"
android:orientation="vertical">
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="8dp"
android:text="选择星座"
android:textColor="@color/choose_constellation_title_color"
android:textSize="14sp" />
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_marginLeft="15dp"
android:layout_marginRight="15dp"
android:background="@color/divider_line_color" />
<android.support.v7.widget.RecyclerView
android:id="@+id/rv_list"
android:layout_width="fill_parent"
android:layout_height="match_parent"
android:divider="@null"
android:dividerHeight="0dp"
android:fadingEdge="none"
android:paddingBottom="8dp"
android:paddingTop="8dp" />
</LinearLayout>
</LinearLayout>
(4)修改colors.xml,添加一个名为choose_constellation_title_color的颜色值用于设置选择星座文本的颜色。
<color name="choose_constellation_title_color">#8bdcea</color>
8. “星座选择”界面Item
任务分析:
“星座选择”界面是使用RecyclerView控件展示十二星座列表的,因此需要创建一个该列表的Item界面。在Item界面中需要展示星座图标、名称以及阳历日期,界面如图所示。

任务实施:
(1)创建“星座选择”界面Item:choose_constella_item.xml。
(2)放置界面控件。
一个ImageView控件用于显示星座图片;
两个TextView控件分别用于显示星座名称与星座阳历日期。
choose_constella_item.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="240dp"
android:layout_height="25dp"
android:layout_marginLeft="30dp"
android:background="@android:color/white"
android:gravity="center_vertical">
<ImageView
android:id="@+id/iv_constella_img"
android:layout_width="20dp"
android:layout_height="20dp"
android:scaleType="fitXY" />
<TextView
android:id="@+id/tv_contella_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="6dp"
android:textColor="@android:color/black"
android:textSize="13sp" />
<TextView
android:id="@+id/tv_date"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="6dp"
android:textColor="@android:color/black"
android:textSize="13sp" />
</LinearLayout>
9. “星座选择”界面Adapter
任务分析:
“星座选择”列表界面是使用RecyclerView控件战士星座信息,因此需要创建一个数据适配器ChooseConstellationListAdapter对RecyelerView控件进行数据适配。
任务实施:
(1)创建ChooseConstellationListAdapter类。在adapter包中创建一个ChooseConstellaListAdapter类继承RecyclerView.Adapter<RecyclerView.ViewHolder>类,并重写onCreateViewHolder()、onBindViewHolder()、getItemCount()方法。
(2)创建ViewHolder类。在ChooseConstellaListAdapter类中创建一个ViewHolder类用于获取Item界面上的控件。
ChooseConstellationListAdapter.java
public class ChooseConstellaListAdapter extends RecyclerView.Adapter<RecyclerView.ViewHolder> implements View.OnClickListener {
private List<ConstellationBean> cbList;
private Context context;
private OnItemClickListener mOnItemClickListener = null;
public ChooseConstellaListAdapter(Context context) {
this.context = context;
}
public void setOnItemClickListener(OnItemClickListener listener) {
this.mOnItemClickListener = listener;
}
public void setData(List<ConstellationBean> cbList) {
this.cbList = cbList;
notifyDataSetChanged();
}
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup viewGroup, int viewType)
{
View view = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.
choose_constella_item, viewGroup, false);
ViewHolder viewHolder = new ViewHolder(view);
view.setOnClickListener(this);
return viewHolder;
}
@Override
public void onBindViewHolder(final RecyclerView.ViewHolder holder, int i) {
ConstellationBean bean = cbList.get(i);
((ViewHolder) holder).tv_contella_name.setText(bean.getName());
((ViewHolder) holder).tv_date.setText(bean.getDate());
Glide
.with(context)
.load(bean.getImg())
.error(R.mipmap.ic_launcher)
.into(((ViewHolder) holder).iv_img);
//将i保存在itemView的Tag中,以便点击时进行获取
holder.itemView.setTag(i);
}
@Override
public int getItemCount() {
return cbList == null ? 0 : cbList.size();
}
@Override
public void onClick(View v) {
if (mOnItemClickListener != null) {
//注意这里使用getTag方法获取position
mOnItemClickListener.onItemClick(v, (int) v.getTag());
}
}
public class ViewHolder extends RecyclerView.ViewHolder {
public TextView tv_contella_name, tv_date;
public ImageView iv_img;
public ViewHolder(View itemView) {
super(itemView);
tv_contella_name = (TextView) itemView.findViewById(R.id.tv_contella_name);
tv_date = (TextView) itemView.findViewById(R.id.tv_date);
iv_img = (ImageView) itemView.findViewById(R.id.iv_constella_img);
}
}
public interface OnItemClickListener {
void onItemClick(View view, int position);
}
}
10.“星座选择”界面数据
任务分析:
“星座选择”界面的图片存放在Tomcat的ROOT文件夹中的constellation文件夹中数据是通过在ROOT文件夹中创建一个choose_constellation_list_data.json文件存放的。
任务实施:
(1)存放“星座选择”界面的图片。将“星座选择”界面需要的图片放入Tomcat的ROOT/newsdemo/topline/img/constellation文件夹中。
(2)创建“星座选择”界面数据文件。在Tomcat的ROOT/nesdemo目录中创建一个choose_constellation_list_data.json文件,该文件用于存放“星座选择”界面需要加载的数据。
choose_constellation_list_data.json
[
{
"id":1,
"name":"白羊座",
"img":"http://172.25.32.1:8080/newsdemo/img/constellation/baiyang_icon.png",
"date":"(阳历3.21-4.19)",
},
……
{
"id":12,
"name":"双鱼座",
"img":"http://172.25.32.1:8080/newsdemo/img/constellation/shuangyu_icon.png",
"date":"(阳历2.19-3.20)",
}
]
(3)修改Constant.java文件。在utils包中的Constant类中添加一个名为REQUEST_CHOOSE_CONSTELLATION_LIST_URL的“星座选择”界面接口地址。
//星座选择界面接口
public static final String REQUEST_CHOOSE_CONSTELLATION_LIST_URL = "/choose_constellation_list_data.json";
11.“星座选择”界面逻辑代码
任务分析:
“星座选择”界面主要显示星座的图标、名称以及阳历日期。点击每个星座会关闭界面并显示“星座选择”界面,同时会把选择的星座Id回传给“星座”界面。
任务实施:
(1)获取界面控件。在ChooseConstellationActivity中创建界面控件的初始化方法init(),获取“星座选择”界面所要用到的控件。
(2)获取数据。在ConstellationActivity中创建getData()方法,用于获取服务器中的十二星座信息数据。
ChooseConstellationActivity.java
public class ChooseConstellationActivity extends AppCompatActivity {
private ImageView iv_close;
private ChooseConstellaListAdapter adapter;
private OkHttpClient okHttpClient;
public static final int MSG_CHOOSE_CONSTELLATION_OK = 1;//获取星座数据
private MHandler mHandler;
private RecyclerView recyclerView;
private List<ConstellationBean> list;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_choose_constellation);
mHandler = new MHandler();
okHttpClient = new OkHttpClient();
getData();
init();
}
private void init() {
iv_close = (ImageView) findViewById(R.id.iv_close);
recyclerView = (RecyclerView) findViewById(R.id.rv_list);
//这里用线性显示 类似于 ListView
recyclerView.setLayoutManager(new LinearLayoutManager(this));
adapter = new ChooseConstellaListAdapter(ChooseConstellationActivity.this);
recyclerView.setAdapter(adapter);
iv_close.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
ChooseConstellationActivity.this.finish();
}
});
adapter.setOnItemClickListener(new ChooseConstellaListAdapter.
OnItemClickListener() {
@Override
public void onItemClick(View view, int position) {
Intent intent = new Intent();
intent.putExtra("id", list.get(position).getId());
setResult(RESULT_OK, intent);
ChooseConstellationActivity.this.finish();
}
});
}
/**
* 事件捕获
*/
class MHandler extends Handler {
@Override
public void dispatchMessage(Message msg) {
super.dispatchMessage(msg);
switch (msg.what) {
case MSG_CHOOSE_CONSTELLATION_OK:
if (msg.obj != null) {
String result = (String) msg.obj;
list = JsonParse.getInstance().getConstellaList(result);
if (list != null) {
if (list.size() > 0) {
adapter.setData(list);
}
}
}
break;
}
}
}
private void getData() {
Request request = new Request.Builder().url(Constant.WEB_SITE +
Constant.REQUEST_CHOOSE_CONSTELLATION_LIST_URL).build();
Call call = okHttpClient.newCall(request);
//开启异步线程访问网络
call.enqueue(new Callback() {
@Override
public void onResponse(Response response) throws IOException {
String res = response.body().string();
Message msg = new Message();
msg.what = MSG_CHOOSE_CONSTELLATION_OK;
msg.obj = res;
mHandler.sendMessage(msg);
}
@Override
public void onFailure(Request arg0, IOException arg1) {
}
});
}
}
(3)修改清单文件。由于“星座选择”界面是对话框的样式,在清单文件的ChooseConstellationActivity对应的activity标签中添加如下代码:
<activity
android:name=".activity.ChooseConstellationActivity"
android:theme="@style/AppTheme.NoTitle.Dialog" />
(4)修改“星座”界面逻辑代码。由于点击“星座”界面右上角的“切换”按钮会跳转到“星座选择”界面,因此需要在ConstellationActivity的init()方法,在该方法找找到tv_switch控件的点击事件,并在该控件的点击事件中添加如下代码:
Intent intent = new Intent(ConstellationActivity.this,ChooseConstellationActivity.class);
startActivityForResult(intent, 1);