首先是要求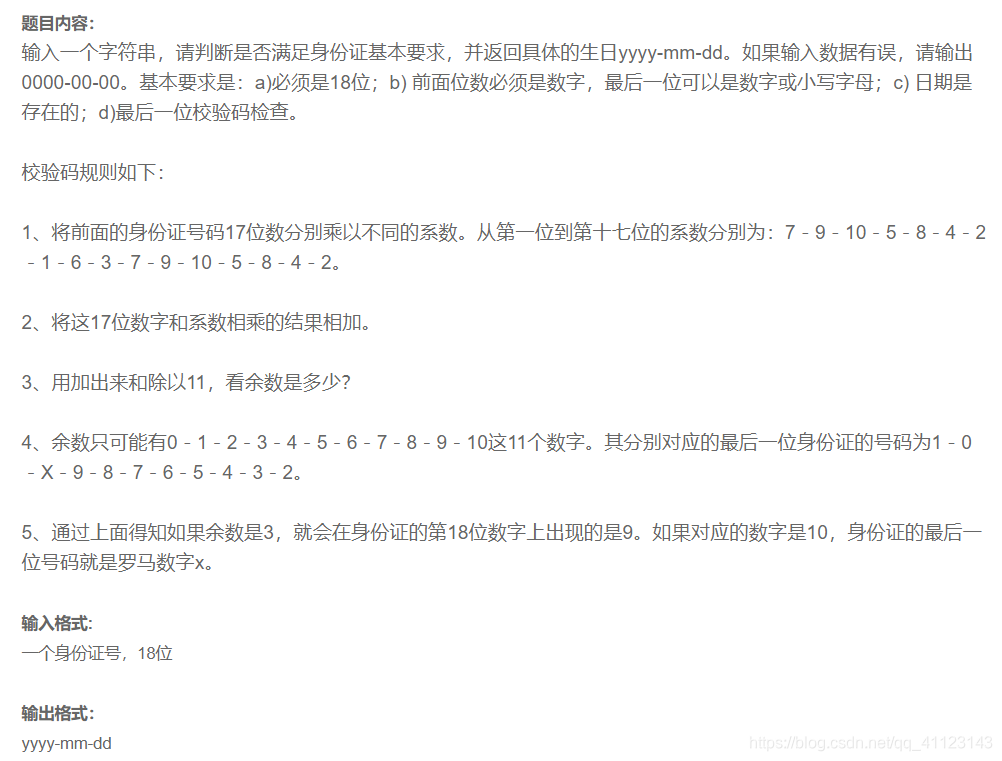
然后进行开发
- 首先验证格式是否正确,这里我采用正则表达式进行格式规范
String formatID = "[0-9]{17}[a-z0-9]{1}";
- 再进行验证日期存在
1.日期是否在今天之前
if ( year1 > gc.get(Calendar.YEAR)) {
return false;
}
2.还有就是月份是否正确
if ( month1 < 1 || month1 > 12 ) {
return false;
}
3.以及每个月的天数(其中二月要判断是否闰年)
switch (month1) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
if ( day1 > 0 && day1 < 32) {
bFlag = true;
}
break;
case 2://判断是否闰年
if ( ((year1 % 4 == 0) && (!(year1 % 100 == 0))) || (year1 % 400 == 0) ) {
if (day1 > 0 && day1 < 30) {
bFlag = true;
}
}else {
if (day1 > 0 && day1 < 29) {
bFlag = true;
}
}
case 4:
case 6:
case 9:
case 11:
if ( day1 > 0 && day1 < 31) {
bFlag = true;
}
break;
}
- 实现校验码验证
public static boolean isCheckCode(String str) {
String number = str.substring(0, 17);
String last = str.substring(17);
final int[] coefficient = {7, 9, 10, 5, 8, 4, 2, 1, 6, 3, 7, 9, 10, 5, 8, 4, 2};
final String[] checkCode = {"1", "0", "x", "9", "8", "7", "6", "5", "4", "3", "2"};
//先转换为int数组
int[] a = new int[str.length()-1];
for (int i = 0; i < a.length; i++) {
a[i] = Integer.parseInt(str.substring(i, i+1));
}
//进行求和
int sum = 0;
for (int j = 0; j < a.length; j++) {
sum += a[j]*coefficient[j];
}
//取余
int reste = 0;
reste = sum % 11;
//进行校验
if (checkCode[reste].equals(last)) {
return true;
}else {
return false;
}
}
最后整合在一块就可以了
public class FormatTest {
public static void main(String[] args) throws ParseException {
Scanner sc = new Scanner(System.in);
System.out.println("请输入一个18位的身份证号:");
String str = sc.nextLine(); //获取输入的字符串,仅限一行
//身份证格式
String formatID = "[0-9]{17}[a-z0-9]{1}";
if (str.matches(formatID)) {
if (isBirthday(str)) {
if (isCheckCode(str)) {
outBirth(str);
}else {
System.out.println("0000-00-00");
}
}else {
System.out.println("0000-00-00");
}
}else {
System.out.println("0000-00-00");
}
}
//按规定格式输出生日
public static void outBirth(String str) throws ParseException {
//获取的值
String birth = str.substring(6, 14);
//1、定义转换格式
SimpleDateFormat bir = new SimpleDateFormat("yyyy-MM-dd");
SimpleDateFormat bir2 = new SimpleDateFormat("yyyyMMdd");
//2、调用formatter2.parse()
Date date = bir2.parse(birth);
//3、将date类型 转化为String类型
String birthDate = bir.format(date);
System.out.println(birthDate);
}
//判断生日格式是否正确
public static boolean isBirthday(String str) {
//生日格式
String year = str.substring(6, 10);
String month = str.substring(10, 12);
String day = str.substring(12, 14);
int year1 = Integer.parseInt(year);
int month1 = Integer.parseInt(month);
int day1 = Integer.parseInt(day);
//开始验证日期
Date today = new Date();
Calendar gc = Calendar.getInstance();
gc.setTime(today);
//验证年
if ( year1 > gc.get(Calendar.YEAR)) {
return false;
}
//判断是否为合法月份
if ( month1 < 1 || month1 > 12 ) {
return false;
}
//判断日期是否对
boolean bFlag = false;
switch (month1) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
if ( day1 > 0 && day1 < 32) {
bFlag = true;
}
break;
case 2://判断是否闰年
if ( ((year1 % 4 == 0) && (!(year1 % 100 == 0))) || (year1 % 400 == 0) ) {
if (day1 > 0 && day1 < 30) {
bFlag = true;
}
}else {
if (day1 > 0 && day1 < 29) {
bFlag = true;
}
}
case 4:
case 6:
case 9:
case 11:
if ( day1 > 0 && day1 < 31) {
bFlag = true;
}
break;
}
if (!bFlag) {
return false;
}
return true;
}
//判断校验码是否正确
public static boolean isCheckCode(String str) {
String number = str.substring(0, 17);
String last = str.substring(17);
final int[] coefficient = {7, 9, 10, 5, 8, 4, 2, 1, 6, 3, 7, 9, 10, 5, 8, 4, 2};
final String[] checkCode = {"1", "0", "x", "9", "8", "7", "6", "5", "4", "3", "2"};
//先转换为int数组
int[] a = new int[str.length()-1];
for (int i = 0; i < a.length; i++) {
a[i] = Integer.parseInt(str.substring(i, i+1));
}
//进行求和
int sum = 0;
for (int j = 0; j < a.length; j++) {
sum += a[j]*coefficient[j];
}
//取余
int reste = 0;
reste = sum % 11;
//进行校验
if (checkCode[reste].equals(last)) {
return true;
}else {
return false;
}
}
}