代码
#include <map>
#include <vector>
#include "iostream"
#include <algorithm>
using namespace std;
typedef char DataType;
typedef struct Node {
DataType data;
int weight;
struct Node* left;
struct Node* right;
}Node;
void initialHuffmanTree(vector<Node*>& vector);
bool compare(Node* a, Node* b);
void printHuffmanCode(Node *&pNode, string codeStr);
Node *createHuffmanTree(vector<Node*>& nodeVec);
int main() {
vector<Node*> nodeVec;
initialHuffmanTree(nodeVec);
Node* huffmanTree = createHuffmanTree(nodeVec);
printHuffmanCode(huffmanTree, "");
return 0;
}
Node *createHuffmanTree(vector<Node*>& nodeVec) {
int index = 0;
while (index != nodeVec.size()-1) {
sort(nodeVec.begin() + index, nodeVec.end(), compare);
Node* temp = (Node*)malloc(sizeof(Node));
temp->data = '#';
temp->weight = nodeVec[index]->weight + nodeVec[index + 1]->weight;
temp->left = nodeVec[index];
temp->right = nodeVec[index + 1];
nodeVec.push_back(temp);
index += 2;
}
return nodeVec[nodeVec.size() - 1];
}
void printHuffmanCode(Node *&pNode, string codeStr) {
if (pNode->left == nullptr || pNode->right == nullptr) {
cout<<pNode->data << " === " << codeStr << endl;
return;
}
printHuffmanCode(pNode->left, codeStr + '0');
printHuffmanCode(pNode->right, codeStr + '1');
}
bool compare(Node* a, Node* b) {
return a->weight < b->weight;
}
void initialHuffmanTree(vector<Node*>& vector) {
char c;
int weight;
while (cin >> c >> weight && c != '#') {
Node* temp = (Node*)malloc(sizeof(Node));
temp->data = c;
temp->weight = weight;
temp->left = temp->right = nullptr;
vector.push_back(temp);
}
}
结果
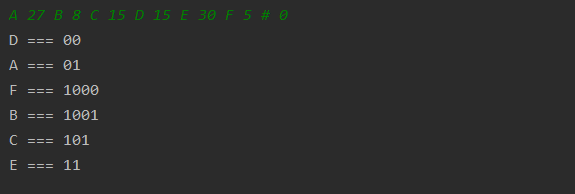