页面显示
主页面
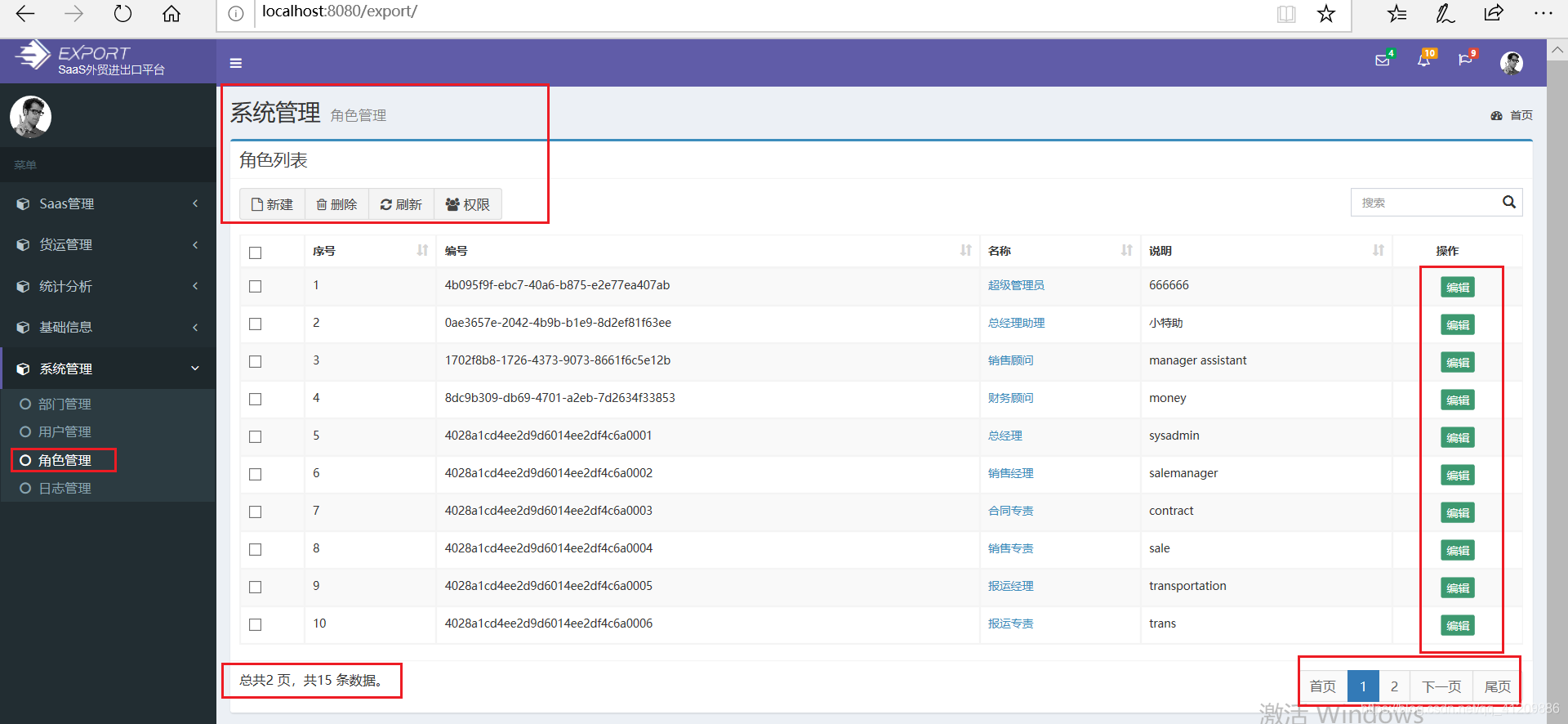
添加界面
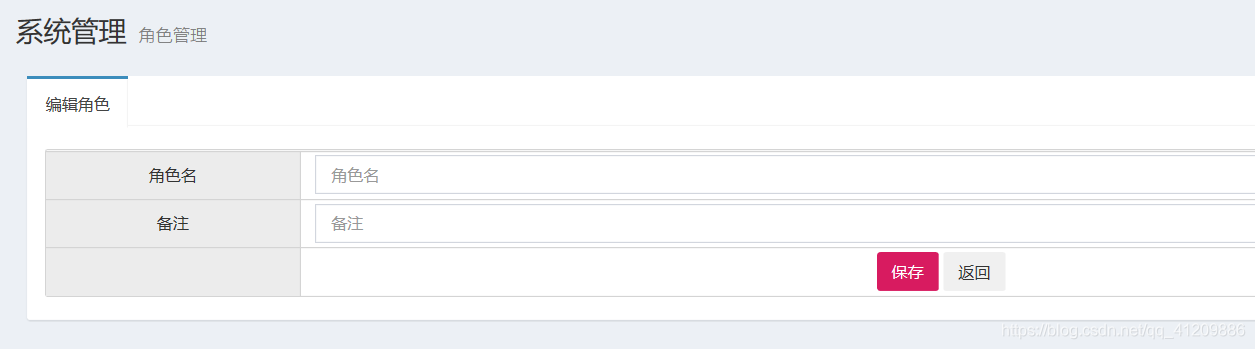
编辑界面
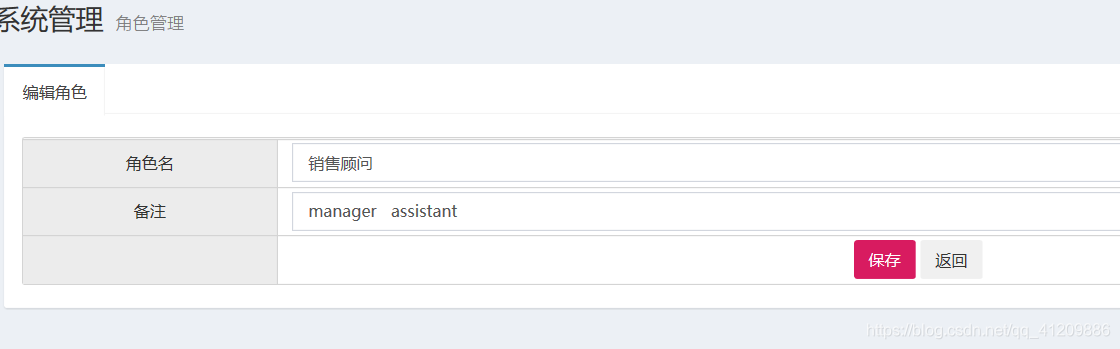
后台代码
查看页面RoleController
@Controller
@RequestMapping("/system/role")
public class RoleController {
private static final Logger l = LoggerFactory.getLogger(RoleController.class);
@RequestMapping(path="/toList",method ={ RequestMethod.GET, RequestMethod.POST})
public String toList(){
return "system/role/role-list";
}
@RequestMapping(path="/toAdd",method ={ RequestMethod.GET, RequestMethod.POST})
public String toAdd(){
return "system/role/role-add";
}
@RequestMapping(path="/toUpdate",method ={ RequestMethod.GET, RequestMethod.POST})
public String toUpdate(){
return "system/role/role-update";
}
@RequestMapping(path="/toRoleModule",method ={ RequestMethod.GET, RequestMethod.POST})
public String toRoleModule(){
return "system/role/role-module";
}
}
(1)Role.java实体类
public class Role {
private String roleId;
private String name;
private String remark;
private long orderNo;
private String createBy;
private String createDept;
private Date createTime;
private String updateBy;
private Date updateTime;
private String companyId;
private String companyName;
全参、空参(必有)、toString、getter AND setter()
(2)TestRoleService.java测试
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath*:spring/applicationContext-*.xml")
public class TestRoleService {
private static final Logger l= LoggerFactory.getLogger(TestRoleService.class);
@Autowired
IRoleService iRoleService;
@Test
public void test01(){
PageInfo<Role> pi=iRoleService.findByPage(1,3,"1");
l.info("pi="+pi);
}
@Test
public void test02(){
Role role=new Role();
role.setName("角色1");
role.setRemark("角色1的备注");
role.setCompanyId("1");
role.setCompanyName("吉首大学");
iRoleService.saveRole(role);
}
@Test
public void test03(){
String roleId ="";
Role role=iRoleService.findById(roleId);
l.info("role="+role);
role.setName("角色2");
role.setRemark("角色2备注");
iRoleService.updateRole(role);
}
@Test
public void test04(){
String roleId="33";
iRoleService.deleteRole(roleId);
}
}
(3-1)IRoleService.java
public interface IRoleService {
PageInfo<Role> findByPage(int curr, int pageSize, String companyId);
void saveRole(Role role);
Role findById(String roleId);
void updateRole(Role role);
void deleteRole(String roleId);
}
(3-2)RoleServiceImpl.java
@Service
public class RoleServiceImpl implements IRoleService {
@Autowired
IRoleDao iRoleDao;
@Override
public PageInfo<Role> findByPage(int curr, int pageSize, String companyId) {
PageHelper.startPage(curr,pageSize);
List<Role> list=iRoleDao.findAll(companyId);
PageInfo<Role> pi= new PageInfo<>(list);
return pi;
}
@Override
public void saveRole(Role role) {
String uuid= UUID.randomUUID().toString();
role.setRoleId(uuid);
iRoleDao.save(role);
}
@Override
public Role findById(String roleId) {
return iRoleDao.findById(roleId);
}
@Override
public void updateRole(Role role) {
iRoleDao.update(role);
}
@Override
public void deleteRole(String roleId) {
iRoleDao.deleteById(roleId);
}
}
(4-1)IRoleDao.java
public interface IRoleDao {
List<Role> findAll(String companyId);
void save(Role role);
Role findById(String roleId);
void update(Role role);
void deleteById(String roleId);
}
(4-2)IRoleDao.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.smp.dao.system.role.IRoleDao">
<resultMap id="roleMap" type="role">
<result column="role_id" property="roleId"/>
<result column="order_no" property="orderNo"/>
<result column="create_by" property="createBy"/>
<result column="create_dept" property="createDept"/>
<result column="create_time" property="createTime"/>
<result column="update_by" property="updateBy"/>
<result column="update_time" property="updateTime"/>
<result column="company_id" property="companyId"/>
<result column="company_name" property="companyName"/>
</resultMap>
<select id="findAll" parameterType="string" resultMap="roleMap">
select * from pe_role where company_id=#{companyId} order by order_no
</select>
<insert id="save" parameterType="role">
insert into pe_role
(
role_id ,
name ,
remark ,
order_no ,
create_by ,
create_dept ,
create_time ,
update_by ,
update_time ,
company_id ,
company_name
)
values
(
#{ roleId },
#{ name },
#{ remark },
#{ orderNo },
#{ createBy },
#{ createDept },
#{ createTime },
#{ updateBy },
#{ updateTime },
#{ companyId },
#{ companyName }
)
</insert>
<select id="findById" parameterType="string" resultMap="roleMap">
select * from pe_role where role_id=#{roleId}
</select>
<update id="update" parameterType="role">
update pe_role set
name = #{name },
remark = #{remark },
order_no = #{orderNo },
create_by = #{createBy },
create_dept = #{createDept },
create_time = #{createTime },
update_by = #{updateBy },
update_time = #{updateTime },
company_id = #{companyId },
company_name = #{companyName}
where role_id = #{roleId}
</update>
<delete id="deleteById" parameterType="string">
delete from pe_role where role_id=#{roleId}
</delete>
</mapper>
前台代码
(1)RoleController.java
- 这里继承了一个父类 —>getLoginCompanyId()
- @RequestParam(defaultValue = “1”)int curr,@RequestParam(defaultValue = “10”)int pageSize【参考:controller设置每页数据有几条】
@Controller
@RequestMapping("system/role")
public class RoleController extends BaseController {
private static final Logger l= LoggerFactory.getLogger(RoleController.class);
@Autowired
IRoleService iRoleService;
@RequestMapping(path = "/toList",method = {RequestMethod.GET,RequestMethod.POST})
public String toList(@RequestParam(defaultValue = "1")int curr,@RequestParam(defaultValue = "10")int pageSize){
PageInfo<Role> pi=iRoleService.findByPage(curr,pageSize,getLoginCompanyId());
request.setAttribute("pi",pi);
return "system/role/role-list";
}
@RequestMapping(path = "/toAdd",method = {RequestMethod.GET,RequestMethod.POST})
public String toAdd(){
return "system/role/role-add";
}
@RequestMapping(path = "/add",method = {RequestMethod.GET,RequestMethod.POST})
public String add(Role role){
l.info("add role="+role);
role.setCompanyId(getLoginCompanyId());
role.setCompanyName(getLoginCompanyName());
iRoleService.saveRole(role);
return "redirect:/system/role/toList.do";
}
@RequestMapping(path = "/toUpdate",method = {RequestMethod.GET,RequestMethod.POST})
public String toUpdate(String roleId){
Role role=iRoleService.findById(roleId);
l.info("toUpdate role="+role);
request.setAttribute("role",role);
return "system/role/role-update";
}
@RequestMapping(path = "/update",method = {RequestMethod.GET,RequestMethod.POST})
public String update(Role role){
l.info("update role="+role);
role.setCompanyId(getLoginCompanyId());
role.setCompanyName(getLoginCompanyName());
iRoleService.updateRole(role);
return "redirect:/system/role/toList.do";
}
@RequestMapping(path = "/delete",method = {RequestMethod.GET,RequestMethod.POST})
public @ResponseBody
Object delete(String roleId){
try {
iRoleService.deleteRole(roleId);
return Result.init(200,"删除成功",null);
} catch (Exception e) {
e.printStackTrace();
return Result.init(-200,"删除失败",null);
}
}
@RequestMapping(path = "/toRoleModule",method = {RequestMethod.GET,RequestMethod.POST})
public String toRoleModule(){
return "system/role/role-module";
}
}
(2)菜单栏界面地址left_menu.jsp
<li id="sys-role">
<a href="${path}/system/role/toList.do" onclick="setSidebarActive(this)" target="iframe">
<i class="fa fa-circle-o"></i>角色管理
</a>
</li>
(3)role-list.jsp列表显示界面
<script>
function deleteById() {
var id = getCheckId()
if(id) {
if(confirm("你确认要删除此条记录吗?")) {
var url='${path}/system/role/delete.do?roleId='+id
var fn=function (result) {
alert(result.msg)
window.location.reload()
}
$.get(url,fn,'json')
}
}else{
alert("请勾选待处理的记录,且每次只能勾选一个")
}
}
function findModuleByRoleId(){
var id = getCheckId();
if(id) {
location.href="/system/role/roleModule.do?roleid="+id;
}else{
alert("请勾选待处理的记录,且每次只能勾选一个")
}
}
</script>
<body>
<div id="frameContent" class="content-wrapper" style="margin-left:0px;">
<section class="content-header">
<h1>
系统管理
<small>角色管理</small>
</h1>
<ol class="breadcrumb">
<li><a href="all-admin-index.html"><i class="fa fa-dashboard"></i> 首页</a></li>
</ol>
</section>
<!-- 内容头部 /-->
<!-- 正文区域 -->
<section class="content">
<!-- .box-body -->
<div class="box box-primary">
<div class="box-header with-border">
<h3 class="box-title">角色列表</h3>
</div>
<div class="box-body">
<!-- 数据表格 -->
<div class="table-box">
<!--工具栏-->
<div class="pull-left">
<div class="form-group form-inline">
<div class="btn-group">
<button type="button" class="btn btn-default" title="新建" onclick='location.href="${path}/system/role/toAdd.do"'><i class="fa fa-file-o"></i> 新建</button>
<button type="button" class="btn btn-default" title="删除" onclick='deleteById()'><i class="fa fa-trash-o"></i> 删除</button>
<button type="button" class="btn btn-default" title="刷新" onclick="window.location.reload();"><i class="fa fa-refresh"></i> 刷新</button>
<button type="button" class="btn btn-default" title="权限" onclick="findModuleByRoleId()"><i class="fa fa-users"></i> 权限</button>
</div>
</div>
</div>
<div class="box-tools pull-right">
<div class="has-feedback">
<input type="text" class="form-control input-sm" placeholder="搜索">
<span class="glyphicon glyphicon-search form-control-feedback"></span>
</div>
</div>
<!--工具栏/-->
<!--数据列表-->
<table id="dataList" class="table table-bordered table-striped table-hover dataTable">
<thead>
<tr>
<th class="" style="padding-right:0px;">
<input type="checkbox" name="selid" onclick="checkAll('id',this)">
</th>
<th class="sorting">序号</th>
<th class="sorting">编号</th>
<th class="sorting">名称</th>
<th class="sorting">说明</th>
<th class="text-center">操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${pi.list}" var="o" varStatus="status">
<tr class="odd" onmouseover="this.className='highlight'" onmouseout="this.className='odd'" >
<td><input type="checkbox" name="id" value="${o.roleId}"/></td>
<td>${status.index+1}</td>
<td>${o.roleId}</td>
<td><a href="${path}/system/role/toUpdate.do?roleId=${o.roleId}">${o.name}</a></td>
<td>${o.remark}</td>
<th class="text-center"><button type="button" class="btn bg-olive btn-xs" onclick='location.href="${path}/system/role/toUpdate.do?roleId=${o.roleId}"'>编辑</button></th>
</tr>
</c:forEach>
</tbody>
</table>
</div>
</div>
<div class="box-footer">
<jsp:include page="../../common/page.jsp">
<jsp:param value="${path}/system/role/toList.do" name="pageUrl"/>
</jsp:include>
</div>
</div>
</section>
</div>
</body>
(4)role-add.jsp添加界面
<body>
<div id="frameContent" class="content-wrapper" style="margin-left:0px;">
<section class="content-header">
<h1>
系统管理
<small>角色管理</small>
</h1>
<ol class="breadcrumb">
<li><a href="all-admin-index.html"><i class="fa fa-dashboard"></i> 首页</a></li>
</ol>
</section>
<section class="content">
<div class="box-body">
<div class="nav-tabs-custom">
<ul class="nav nav-tabs">
<li class="active">
<a href="#tab-form" data-toggle="tab">编辑角色</a>
</li>
</ul>
<div class="tab-content">
<form id="editForm" action="${path}/system/role/add.do" method="post">
<%--<input type="hidden" name="roleId" value="${role.roleId}">--%>
<div class="tab-pane active" id="tab-form">
<div class="row data-type">
<div class="col-md-2 title">角色名</div>
<div class="col-md-10 data">
<input type="text" class="form-control" placeholder="角色名" name="name" value="${role.name}">
</div>
<div class="col-md-2 title">备注</div>
<div class="col-md-10 data line-height36">
<input type="text" class="form-control" placeholder="备注" name="remark" value="${role.remark}">
</div>
<div class="col-md-2 title"></div>
<div class="col-md-10 data text-center">
<button type="button" onclick='document.getElementById("editForm").submit()' class="btn bg-maroon">保存</button>
<button type="button" class="btn bg-default" onclick="history.back(-1);">返回</button>
</div>
</div>
</div>
</form>
</div>
</div>
</div>
</section>
</div>
</body>
(5)role-update.jsp编辑界面
<body>
<div id="frameContent" class="content-wrapper" style="margin-left:0px;">
<section class="content-header">
<h1>
系统管理
<small>角色管理</small>
</h1>
<ol class="breadcrumb">
<li><a href="all-admin-index.html"><i class="fa fa-dashboard"></i> 首页</a></li>
</ol>
</section>
<section class="content">
<div class="box-body">
<div class="nav-tabs-custom">
<ul class="nav nav-tabs">
<li class="active">
<a href="#tab-form" data-toggle="tab">编辑角色</a>
</li>
</ul>
<div class="tab-content">
<form id="editForm" action="${path}/system/role/update.do" method="post">
<input type="hidden" name="roleId" value="${role.roleId}">
<div class="tab-pane active" id="tab-form">
<div class="row data-type">
<div class="col-md-2 title">角色名</div>
<div class="col-md-10 data">
<input type="text" class="form-control" placeholder="角色名" name="name" value="${role.name}">
</div>
<div class="col-md-2 title">备注</div>
<div class="col-md-10 data line-height36">
<input type="text" class="form-control" placeholder="备注" name="remark" value="${role.remark}">
</div>
<div class="col-md-2 title"></div>
<div class="col-md-10 data text-center">
<button type="button" onclick='document.getElementById("editForm").submit()' class="btn bg-maroon">保存</button>
<button type="button" class="btn bg-default" onclick="history.back(-1);">返回</button>
</div>
</div>
</div>
</form>
</div>
</div>
</div>
</section>
</div>
</body>
(6)role-module.jsp权限界面
<head>
<base href="${path}/">
<!-- 页面meta -->
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>数据 - AdminLTE2定制版</title>
<meta name="description" content="AdminLTE2定制版">
<meta name="keywords" content="AdminLTE2定制版">
<!-- Tell the browser to be responsive to screen width -->
<meta content="width=device-width,initial-scale=1,maximum-scale=1,user-scalable=no" name="viewport">
<!-- 页面meta /-->
<link rel="stylesheet" href="plugins/ztree/css/zTreeStyle/zTreeStyle.css" type="text/css">
<script type="text/javascript" src="plugins/ztree/js/jquery-1.4.4.min.js"></script>
<script type="text/javascript" src="plugins/ztree/js/jquery.ztree.core-3.5.js"></script>
<script type="text/javascript" src="plugins/ztree/js/jquery.ztree.excheck-3.5.js"></script>
<SCRIPT type="text/javascript">
</SCRIPT>
</head>
<body style="overflow: visible;">
<div id="frameContent" class="content-wrapper" style="margin-left:0px;height: 1200px" >
<section class="content-header">
<h1>
菜单管理
<small>菜单列表</small>
</h1>
<ol class="breadcrumb">
<li><a href="all-admin-index.html"><i class="fa fa-dashboard"></i> 首页</a></li>
</ol>
</section>
<!-- 内容头部 /-->
<!-- 正文区域 -->
<section class="content">
<!-- .box-body -->
<div class="box box-primary">
<div class="box-header with-border">
<h3 class="box-title">角色 [${role.name}] 权限列表</h3>
</div>
<div class="box-body">
<!-- 数据表格 -->
<div class="table-box">
<!--工具栏-->
<div class="box-tools text-left">
<button type="button" class="btn bg-maroon" onclick="submitCheckedNodes();">保存</button>
<button type="button" class="btn bg-default" onclick="history.back(-1);">返回</button>
</div>
<!--工具栏/-->
<!-- 树菜单 -->
<form name="icform" method="post" action="${path}/system/role/toRoleModule.do">
<input type="hidden" name="roleId" value="${role.roleId}"/>
<input type="hidden" id="moduleIds" name="moduleIds" value=""/>
<div class="content_wrap">
<div class="zTreeDemoBackground left" style="overflow: visible">
<ul id="treeDemo" class="ztree"></ul>
</div>
</div>
</form>
<!-- 树菜单 /-->
</div>
<!-- /数据表格 -->
</div>
<!-- /.box-body -->
</div>
</section>
</div>
</body>