模块管理
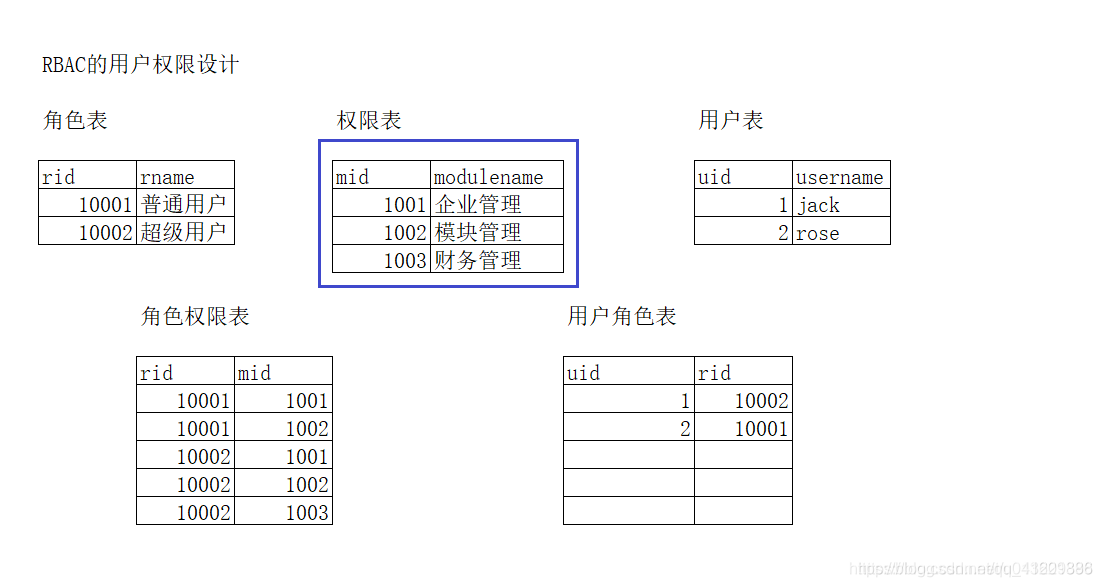
页面显示
主页面
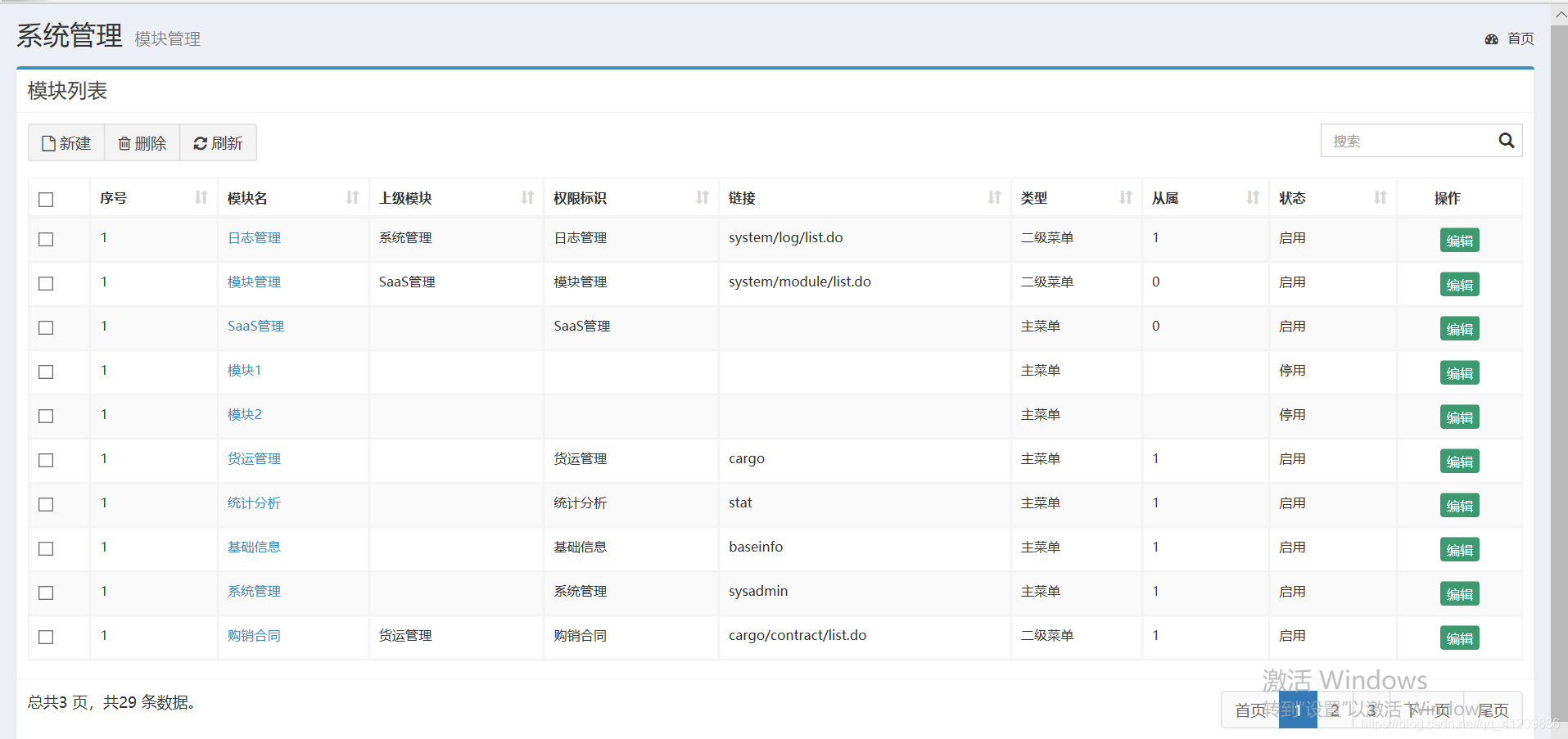
添加界面
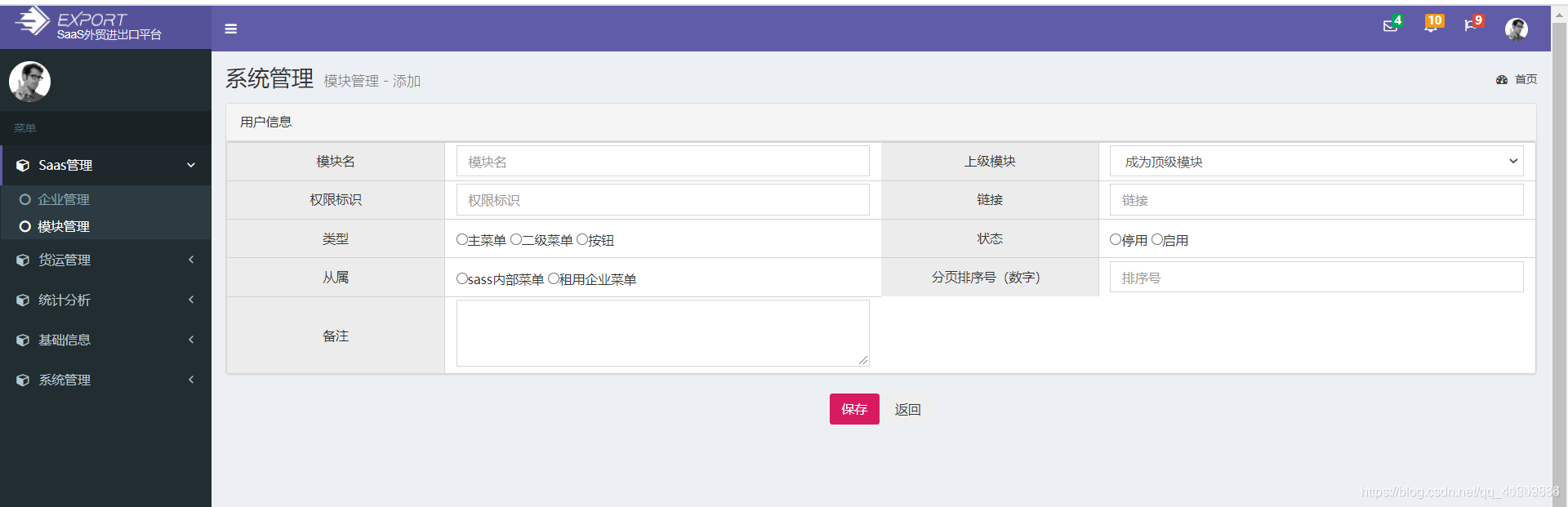
编辑界面
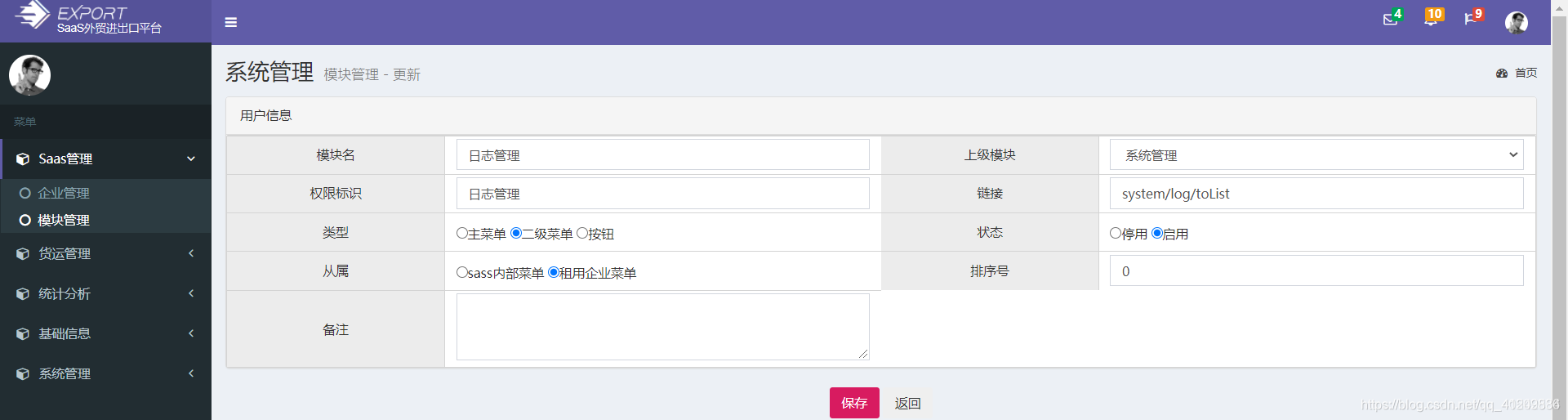
后台代码
(1)Module.java实体类
public class Module {
private String moduleId ;
private String parentId ;
private String parentName;
private String name ;
private long layerNum ;
private long isLeaf ;
private String ico ;
private String cpermission ;
private String curl ;
private long ctype ;
private long state ;
private String belong ;
private String cwhich ;
private long quoteNum ;
private String remark ;
private long orderNo ;
}
全参、空参(必有)、toString、getter AND setter()
(2)TestModuleService.java测试
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath*:spring/applicationContext-*.xml")
public class TestModuleService {
private static final Logger l= LoggerFactory.getLogger(TestModuleService.class);
@Autowired
IModuleService iModuleService;
@Test
public void test01(){
PageInfo<Module> pi=iModuleService.findByPage(1,3);
l.info("pi="+pi);
}
@Test
public void test02(){
Module module=new Module();
module.setName("模块1");
iModuleService.saveModule(module);
}
@Test
public void test03(){
String moduleId ="62c977ed-9ebd-41f4-b963-055873947f4b";
Module module=iModuleService.findModuleById(moduleId);
l.info("module="+module);
module.setName("模块2");
iModuleService.updateModule(module);
}
@Test
public void test04(){
String moduleId="9c5eb9b0-54a4-48bb-aab4-0d1d46cdfbbd";
boolean flag=iModuleService.deleteModule(moduleId);
l.info(flag+"");
}
}
(3-1)IModuleService.java
public interface IModuleService {
PageInfo<Module> findByPage(int curr, int pageSize);
void saveModule(Module module);
Module findModuleById(String moduleId);
void updateModule(Module module);
boolean deleteModule(String moduleId);
}
(3-2)ModuleServiceImpl.java
@Service
@Service
public class ModuleServiceImpl implements IModuleService {
@Autowired
IModuleDao iModuleDao;
@Override
public PageInfo<Module> findByPage(int curr, int pageSize) {
PageHelper.startPage(curr,pageSize);
List<Module> list=iModuleDao.findAll();
PageInfo<Module> pi= new PageInfo<>(list);
return pi;
}
@Override
public void saveModule(Module module) {
String uuid= UUID.randomUUID().toString();
module.setModuleId(uuid);
iModuleDao.save(module);
}
@Override
public Module findModuleById(String moduleId) {
return iModuleDao.findById(moduleId);
}
@Override
public void updateModule(Module module) {
iModuleDao.update(module);
}
@Override
public boolean deleteModule(String moduleId) {
int count = iModuleDao.findParentIdCount(moduleId);
if (count==0){
iModuleDao.deleteById(moduleId);
return true;
}else{
return false;
}
}
}
(4-1)IModuleDao.java
public interface IModuleDao {
List<Module> findAll();
void save(Module module);
void update(Module module);
Module findById(String moduleId);
int findParentIdCount(String moduleId);
void deleteById(String moduleId);
}
(4-2)IModuleDao.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.smp.dao.system.module.IModuleDao">
<resultMap id="moduleMap" type="module">
<id column="module_id" property="moduleId"/>
<result column="parent_id " property="parentId"/>
<result column="parent_name" property="parentName"/>
<result column="name" property="name"/>
<result column="layer_num" property="layerNum"/>
<result column="is_leaf" property="isLeaf"/>
<result column="ico" property="ico"/>
<result column="cpermission" property="cpermission"/>
<result column="curl" property="curl"/>
<result column="ctype" property="ctype"/>
<result column="state" property="state"/>
<result column="belong" property="belong"/>
<result column="cwhich" property="cwhich"/>
<result column="quote_num" property="quoteNum"/>
<result column="remark" property="remark"/>
<result column="order_no" property="orderNo"/>
</resultMap>
<select id="findAll" resultMap="moduleMap">
select * from ss_module order by order_no asc
</select>
<insert id="save" parameterType="module">
insert into ss_module
(
module_id ,
parent_id ,
parent_name ,
name ,
layer_num ,
is_leaf ,
ico ,
cpermission ,
curl ,
ctype ,
state ,
belong ,
cwhich ,
quote_num ,
remark ,
order_no
)
values
(
#{ moduleId },
<if test="parentId == null or parentId=='' ">
NULL,
</if>
<if test="parentId != null and parentId!='' ">
#{parentId },
</if>
#{ parentName },
#{ name },
#{ layerNum },
#{ isLeaf },
#{ ico },
#{ cpermission },
#{ curl },
#{ ctype },
#{ state },
#{ belong },
#{ cwhich },
#{ quoteNum },
#{ remark },
#{ orderNo }
)
</insert>
<select id="findById" parameterType="string" resultMap="moduleMap">
select * from ss_module where module_id=#{moduleId}
</select>
<update id="update" parameterType="module">
update ss_module set
parent_id = #{parentId },
parent_name = #{parentName },
name = #{name },
layer_num = #{layerNum },
is_leaf = #{isLeaf },
ico = #{ico },
cpermission = #{cpermission },
curl = #{curl },
ctype = #{ctype },
state = #{state },
belong = #{belong },
cwhich = #{cwhich },
quote_num = #{quoteNum },
remark = #{remark },
order_no = #{orderNo }
where module_id = #{moduleId}
</update>
<delete id="deleteById" parameterType="string">
delete from ss_module where module_id=#{moduleId}
</delete>
<select id="findParentIdCount" parameterType="string" resultType="int">
select count(*) from ss_module where parent_id=#{moduleId}
</select>
<select id="findByRoleId" parameterType="string" resultMap="moduleMap">
select m.*
from pe_role_module rm
inner join ss_module m
on rm.module_id=m.module_id
where rm.role_id=#{roleId}
</select>
<delete id="deleteRoleModule" parameterType="string">
delete from pe_role_module where role_id=#{roleId}
</delete>
</mapper>
前台代码
(1)ModuleController.java
- 这里继承了一个父类 —>getLoginCompanyId()
- @RequestParam(defaultValue = “1”)int curr,@RequestParam(defaultValue = “10”)int pageSize【参考:controller设置每页数据有几条】
@Controller
@RequestMapping("system/module")
public class ModuleController extends BaseController {
private static final Logger l= LoggerFactory.getLogger(ModuleController.class);
@Autowired
IModuleService imoduleService;
@RequestMapping(path = "/toList",method = {RequestMethod.GET,RequestMethod.POST})
public String toList(@RequestParam(defaultValue = "1") int curr, @RequestParam(defaultValue = "10")int pageSize){
PageInfo<Module> pi=imoduleService.findByPage(curr,pageSize);
request.setAttribute("pi",pi);
return "system/module/module-list";
}
@RequestMapping(path = "/toAdd",method = {RequestMethod.GET,RequestMethod.POST})
public String toAdd(){
List<Module> list= imoduleService.findAllModules();
request.setAttribute("list",list);
return "system/module/module-add";
}
@RequestMapping(path = "/add",method = {RequestMethod.GET,RequestMethod.POST})
public String add(Module module){
l.info("add module="+module);
imoduleService.saveModule(module);
return "redirect:/system/module/toList.do";
}
@RequestMapping(path = "/toUpdate",method = {RequestMethod.GET,RequestMethod.POST})
public String toUpdate(String moduleId){
l.info("toUpdate moduleId="+moduleId);
Module module=imoduleService.findModuleById(moduleId);
l.info("toUpdate module="+module);
request.setAttribute("module",module);
List<Module> modules=imoduleService.findAllModules();
request.setAttribute("modules",modules);
return "system/module/module-update";
}
@RequestMapping(path = "/update",method = {RequestMethod.GET,RequestMethod.POST})
public String update(Module module){
l.info("update module="+module);
imoduleService.updateModule(module);
return "redirect:/system/module/toList.do";
}
@RequestMapping(path = "/delete",method = {RequestMethod.GET,RequestMethod.POST})
public @ResponseBody
Object delete(String moduleId){
boolean flag=imoduleService.deleteModule(moduleId);
if(flag){
return Result.init(200,"删除成功",null);
}else {
return Result.init(-200,"不能删除当前模块,被子模块引用!!!",null);
}
}
}
(2)module-list.jsp列表显示界面
<script>
function deleteById() {
var id = getCheckId()
if(id) {
if(confirm("你确认要删除此条记录吗?")) {
var url= '${path}/system/module/delete.do?moduleId='+id;
var fn = function(result){
alert(result.msg)
window.location.reload()
}
$.get(url,fn,'json')
}
}else{
alert("请勾选待处理的记录,且每次只能勾选一个")
}
}
</script>
<body>
<div id="frameContent" class="content-wrapper" style="margin-left:0px;">
<section class="content-header">
<h1>
系统管理
<small>模块管理</small>
</h1>
<ol class="breadcrumb">
<li><a href="all-admin-index.html"><i class="fa fa-dashboard"></i> 首页</a></li>
</ol>
</section>
<!-- 内容头部 /-->
<!-- 正文区域 -->
<section class="content">
<!-- .box-body -->
<div class="box box-primary">
<div class="box-header with-border">
<h3 class="box-title">模块列表</h3>
</div>
<div class="box-body">
<!-- 数据表格 -->
<div class="table-box">
<!--工具栏-->
<div class="pull-left">
<div class="form-group form-inline">
<div class="btn-group">
<button type="button" class="btn btn-default" title="新建" onclick='location.href="${path}/system/module/toAdd.do"'><i class="fa fa-file-o"></i> 新建</button>
<button type="button" class="btn btn-default" title="删除" onclick='deleteById()'><i class="fa fa-trash-o"></i> 删除</button>
<button type="button" class="btn btn-default" title="刷新" onclick="window.location.reload();"><i class="fa fa-refresh"></i> 刷新</button>
</div>
</div>
</div>
<div class="box-tools pull-right">
<div class="has-feedback">
<input type="text" class="form-control input-sm" placeholder="搜索">
<span class="glyphicon glyphicon-search form-control-feedback"></span>
</div>
</div>
<!--工具栏/-->
<!--数据列表-->
<table id="dataList" class="table table-bordered table-striped table-hover dataTable">
<thead>
<tr>
<th class="" style="padding-right:0px;">
<input type="checkbox" name="selid" onclick="checkAll('id',this)">
</th>
<th class="sorting">序号</th>
<th class="sorting">模块名</th>
<th class="sorting">上级模块</th>
<th class="sorting">权限标识</th>
<th class="sorting">链接</th>
<th class="sorting">类型</th>
<th class="sorting">从属</th>
<th class="sorting">状态</th>
<th class="text-center">操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${pi.list}" var="o" varStatus="st">
<tr>
<td><input type="checkbox" name="moduleId" value="${o.moduleId }"/></td>
<td>${status.index+1}</td>
<td><a href="${path}/system/module/toUpdate.do?moduleId=${o.moduleId}">${o.name}</a></td>
<td>${o.parentName}</td>
<td>${o.cpermission}</td>
<td>${o.curl}</td>
<td>${o.ctype==0?'主菜单':o.ctype==1?'二级菜单':'按钮'}</td>
<td>${o.belong}</td>
<td>${o.state==0?'停用':'启用'}</td>
<th class="text-center"><button type="button" class="btn bg-olive btn-xs" onclick='location.href="${path}/system/module/toUpdate.do?moduleId=${o.moduleId}"'>编辑</button></th>
</tr>
</c:forEach>
</tbody>
</table>
</div>
</div>
<div class="box-footer">
<jsp:include page="../../common/page.jsp">
<jsp:param value="${path}/system/module/toList.do" name="pageUrl"/>
</jsp:include>
</div>
</div>
</section>
</div>
</body>
(3)module-add.jsp添加界面
<body>
<div id="frameContent" class="content-wrapper" style="margin-left:0px;">
<section class="content-header">
<h1>
系统管理
<small>部门管理</small>
</h1>
<ol class="breadcrumb">
<li><a href="all-admin-index.html"><i class="fa fa-dashboard"></i> 首页</a></li>
</ol>
</section>
<section class="content">
<div class="panel panel-default">
<div class="panel-heading">用户信息</div>
<form id="editForm" action="${path}/system/module/add.do" method="post">
<input type="hidden" id="parentName" name="parentName" value="${module.parentName}">
<div class="row data-type" style="margin: 0px">
<div class="col-md-2 title">模块名</div>
<div class="col-md-4 data">
<input type="text" class="form-control" placeholder="模块名" name="name" value="${module.name}">
</div>
<div class="col-md-2 title">上级模块</div>
<div class="col-md-4 data">
<select class="form-control" onchange="document.getElementById('parentName').value=this.options[this.selectedIndex].text" name="parentId">
<option value="">成员顶级模块</option>
<c:forEach items="${list}" var="item">
<option value="${item.moduleId}">${item.name}</option>
</c:forEach>
</select>
</div>
<div class="col-md-2 title">权限标识</div>
<div class="col-md-4 data">
<input type="text" class="form-control" placeholder="权限标识" name="cpermission" value="${module.cpermission}">
</div>
<div class="col-md-2 title">链接</div>
<div class="col-md-4 data">
<input type="text" class="form-control" placeholder="链接" name="curl" value="${module.curl}">
</div>
<div class="col-md-2 title">类型</div>
<div class="col-md-4 data">
<div class="form-group form-inline">
<div class="radio"><label><input type="radio" ${module.ctype==0?'checked':''} name="ctype" value="0">主菜单</label></div>
<div class="radio"><label><input type="radio" ${module.ctype==1?'checked':''} name="ctype" value="1">二级菜单</label></div>
<div class="radio"><label><input type="radio" ${module.ctype==2?'checked':''} name="ctype" value="2">按钮</label></div>
</div>
</div>
<div class="col-md-2 title">状态</div>
<div class="col-md-4 data">
<div class="form-group form-inline">
<div class="radio"><label><input type="radio" ${module.state==0?'checked':''} name="state" value="0">停用</label></div>
<div class="radio"><label><input type="radio" ${module.state==1?'checked':''} name="state" value="1">启用</label></div>
</div>
</div>
<div class="col-md-2 title">从属</div>
<div class="col-md-4 data">
<div class="form-group form-inline">
<div class="radio"><label><input type="radio" ${module.belong==0?'checked':''} name="belong" value="0">sass内部菜单</label></div>
<div class="radio"><label><input type="radio" ${module.belong==1?'checked':''} name="belong" value="1">租用企业菜单</label></div>
</div>
</div>
<div class="col-md-2 title">排序号</div>
<div class="col-md-4 data">
<input type="text" class="form-control" placeholder="排序号" name="orderNo" value="${module.orderNo}">
</div>
<div class="col-md-2 title rowHeight2x">备注</div>
<div class="col-md-4 data rowHeight2x">
<textarea class="form-control" rows="3" name="remark">${module.remark}</textarea>
</div>
</div>
</form>
</div>
<!--订单信息/-->
<div class="box-tools text-center">
<button type="button" onclick='document.getElementById("editForm").submit()' class="btn bg-maroon">保存</button>
<button type="button" class="btn bg-default" onclick="history.back(-1);">返回</button>
</div>
</section>
</div>
</body>
(4)module-update.jsp编辑界面
<body>
<div id="frameContent" class="content-wrapper" style="margin-left:0px;">
<section class="content-header">
<h1>
系统管理
<small>部门管理</small>
</h1>
<ol class="breadcrumb">
<li><a href="all-admin-index.html"><i class="fa fa-dashboard"></i> 首页</a></li>
</ol>
</section>
<section class="content">
<div class="panel panel-default">
<div class="panel-heading">用户信息</div>
<form id="editForm" action="${path}/system/module/update.do" method="post">
<input type="hidden" name="moduleId" value="${module.moduleId}">
<input type="hidden" id="parentName" name="parentName" value="${module.parentName}">
<div class="row data-type" style="margin: 0px">
<div class="col-md-2 title">模块名</div>
<div class="col-md-4 data">
<input type="text" class="form-control" placeholder="模块名" name="name" value="${module.name}">
</div>
<div class="col-md-2 title">上级模块</div>
<div class="col-md-4 data">
<select class="form-control" onchange="document.getElementById('parentName').value=this.options[this.selectedIndex].text" name="parentId">
<option value="">自己成为顶级模块(默认)</option>
<c:forEach items="${modules}" var="item">
<c:if test="${module.moduleId != item.moduleId }">
<%-- 当前模块不能选择自己作为上级--%>
<option
<%-- 当前模块数据有一个parentId,集合的哪个模块的moduleId跟我相同,则生成selected --%>
<c:if test="${module.parentId == item.moduleId }">
selected
</c:if>
value="${item.moduleId}">${item.name}</option>
</c:if>
</c:forEach>
</select>
</div>
<div class="col-md-2 title">权限标识</div>
<div class="col-md-4 data">
<input type="text" class="form-control" placeholder="权限标识" name="cpermission" value="${module.cpermission}">
</div>
<div class="col-md-2 title">链接</div>
<div class="col-md-4 data">
<input type="text" class="form-control" placeholder="链接" name="curl" value="${module.curl}">
</div>
<div class="col-md-2 title">类型</div>
<div class="col-md-4 data">
<div class="form-group form-inline">
<div class="radio"><label><input type="radio" ${module.ctype==0?'checked':''} name="ctype" value="0">主菜单</label></div>
<div class="radio"><label><input type="radio" ${module.ctype==1?'checked':''} name="ctype" value="1">二级菜单</label></div>
<div class="radio"><label><input type="radio" ${module.ctype==2?'checked':''} name="ctype" value="2">按钮</label></div>
</div>
</div>
<div class="col-md-2 title">状态</div>
<div class="col-md-4 data">
<div class="form-group form-inline">
<div class="radio"><label><input type="radio" ${module.state==0?'checked':''} name="state" value="0">停用</label></div>
<div class="radio"><label><input type="radio" ${module.state==1?'checked':''} name="state" value="1">启用</label></div>
</div>
</div>
<div class="col-md-2 title">从属</div>
<div class="col-md-4 data">
<div class="form-group form-inline">
<div class="radio"><label><input type="radio" ${module.belong==0?'checked':''} name="belong" value="0">sass内部菜单</label></div>
<div class="radio"><label><input type="radio" ${module.belong==1?'checked':''} name="belong" value="1">租用企业菜单</label></div>
</div>
</div>
<div class="col-md-2 title">排序号</div>
<div class="col-md-4 data">
<input type="text" class="form-control" placeholder="排序号" name="orderNo" value="${module.orderNo}">
</div>
<div class="col-md-2 title rowHeight2x">备注</div>
<div class="col-md-4 data rowHeight2x">
<textarea class="form-control" rows="3" name="remark">${module.remark}</textarea>
</div>
</div>
</form>
</div>
<!--订单信息/-->
<div class="box-tools text-center">
<button type="button" onclick='document.getElementById("editForm").submit()' class="btn bg-maroon">保存</button>
<button type="button" class="btn bg-default" onclick="history.back(-1);">返回</button>
</div>
</section>
</div>
</body>