本人使用 android 10
效果展示
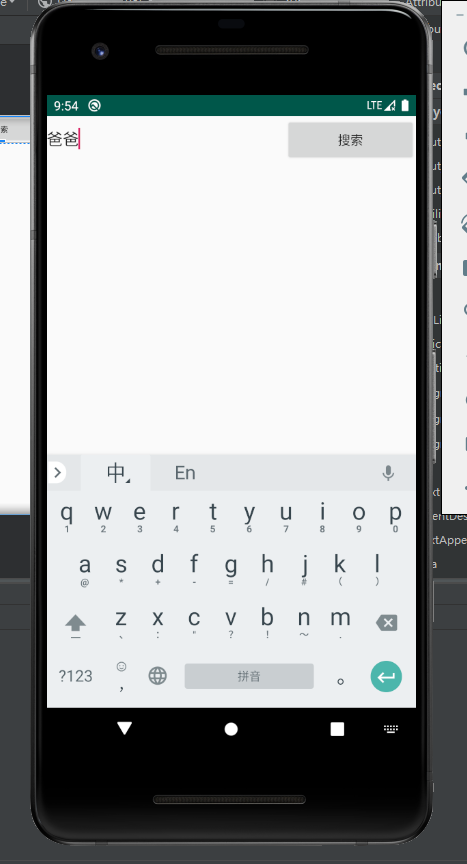
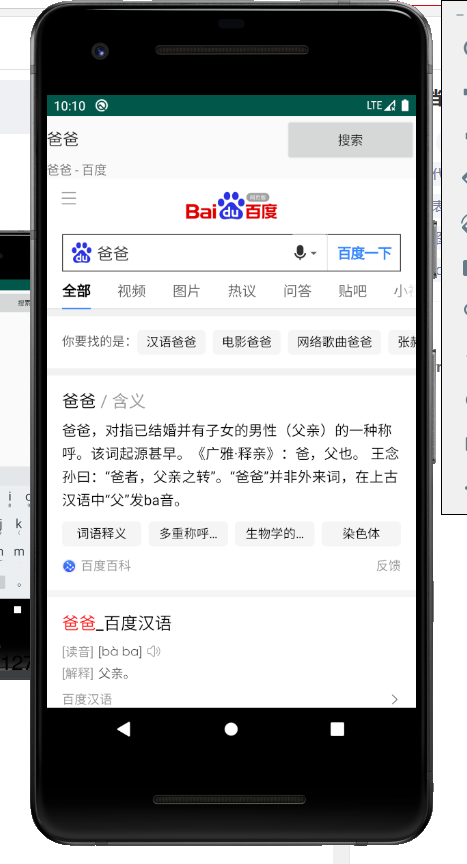
显示标题,进度条。
代码部分
布局文件
- activity_main
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<EditText
android:id="@+id/editText"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:layout_weight="4"
android:background="@null" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:layout_weight="1"
android:text="搜索"
/>
</LinearLayout>
</LinearLayout>
- activity_web
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".web.WebActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<EditText
android:id="@+id/editText"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:layout_weight="4"
android:background="@null" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:layout_weight="1"
android:text="搜索" />
</LinearLayout>
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyleHorizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="TextView" />
<WebView
android:id="@+id/webView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
关键代码
- MainActivity
package com.example.webview;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.graphics.Color;
import android.graphics.drawable.GradientDrawable;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import com.example.webview.web.WebActivity;
public class MainActivity extends AppCompatActivity{
public EditText editText;
public Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText=findViewById(R.id.editText);
button= findViewById(R.id.button);
GradientDrawable drawable = new GradientDrawable();
drawable.setCornerRadius(15);
drawable.setColor(Color.parseColor("#ffffff"));
drawable.setStroke(10,Color.parseColor("#cccccc"));
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String keyWord=""+editText.getText().toString().trim();
Intent i=new Intent(MainActivity.this, WebActivity.class);
i.putExtra("keyWord",keyWord);
startActivity(i);
}
});
}
}
- WebActivity
package com.example.webview.web;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.KeyEvent;
import android.view.View;
import android.webkit.WebView;
import android.widget.EditText;
import android.widget.ProgressBar;
import android.widget.TextView;
import android.widget.Toast;
import com.example.webview.R;
public class WebActivity extends AppCompatActivity implements MyWebView {
public WebView webView;
private TextView textView;
private ProgressBar progressBar;
private EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_web);
webView=findViewById(R.id.webView);
progressBar=findViewById(R.id.progressBar);
textView=findViewById(R.id.textView);
WebSetting webSetting=new WebSetting(webView);
webView.setWebViewClient(new MyWebClient(this));
MyWebChromeClient myWebChromeClient=new MyWebChromeClient(this);
webView.setWebChromeClient(myWebChromeClient);
Intent intent=getIntent();
String keyWord=intent.getStringExtra("keyWord");
editText=findViewById(R.id.editText);
editText.setText(keyWord);
String url="https://www.baidu.com/s?wd="+keyWord;
webView.loadUrl(url);
}
//点击返回上一页面,而不是退出webView
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
if (keyCode == KeyEvent.KEYCODE_BACK && webView.canGoBack()) {
webView.goBack();
return true;
}
return super.onKeyDown(keyCode, event);
}
//设置标题
@Override
public void setTitle(String title) {
textView.setText(title);
}
//设置进度条的进度
@Override
public void setMyProgress(int newProgress){
progressBar.setProgress(newProgress);
}
//页面开始的时候显示
@Override
public void StartProgress() {
progressBar.setVisibility(View.VISIBLE);
}
//页面结束的时候显示
@Override
public void FinishProgress() {
progressBar.setVisibility(View.GONE);
}
//打印链接
@Override
public void printUrl(String url) {
Toast.makeText(this,url,Toast.LENGTH_SHORT).show();
}
}
重要代码
- WebSetting部分
package com.example.webview.web;
import android.os.Build;
import android.webkit.WebSettings;
import android.webkit.WebView;
public class WebSetting {
public WebSetting(WebView webView){
//声明WebSettings子类
WebSettings mWebSettings =webView.getSettings();
mWebSettings.setJavaScriptCanOpenWindowsAutomatically(true);//设置js可以直接打开窗口,如window.open(),默认为false
mWebSettings.setJavaScriptEnabled(true);//是否允许JavaScript脚本运行,默认为false。设置true时,会提醒可能造成XSS漏洞
mWebSettings.setSupportZoom(true);//是否可以缩放,默认true
mWebSettings.setBuiltInZoomControls(true);//是否显示缩放按钮,默认false
mWebSettings.setUseWideViewPort(true);//设置此属性,可任意比例缩放。大视图模式
mWebSettings.setLoadWithOverviewMode(true);//和setUseWideViewPort(true)一起解决网页自适应问题
mWebSettings.setAppCacheEnabled(true);//是否使用缓存
mWebSettings.setDomStorageEnabled(true);//开启本地DOM存储
mWebSettings.setLoadsImagesAutomatically(true); // 加载图片
mWebSettings.setMediaPlaybackRequiresUserGesture(false);//播放音频,多媒体需要用户手动?设置为false为可自动播放
//从Android5.0开始,WebView默认不支持同时加载Https和Http混合模式。
if (Build.VERSION.SDK_INT >= 21) {
mWebSettings.setMixedContentMode(WebSettings.MIXED_CONTENT_ALWAYS_ALLOW);
}
}
}
- 关键数据,获取接口
package com.example.webview.web;
public interface MyWebView {
//ChromeWebClient
public void setTitle(String title);
public void setMyProgress(int newProgress);
//WebClient
public void StartProgress();
public void FinishProgress();
public void printUrl(String url);
}
- WebViewClient设置
package com.example.webview.web;
import android.annotation.TargetApi;
import android.graphics.Bitmap;
import android.net.http.SslError;
import android.os.Build;
import android.webkit.SslErrorHandler;
import android.webkit.WebResourceError;
import android.webkit.WebResourceRequest;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import android.widget.Toast;
public class MyWebClient extends WebViewClient {
private MyWebView mywebview;
public MyWebClient(MyWebView mywebview){
this.mywebview= mywebview;
}
//例如对网址进行拦截
//For example, block the URL
@SuppressWarnings("deprecation")
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
return false; //false 在WebView加载网页
//Load web pages in WebView
}
@TargetApi(Build.VERSION_CODES.N)
@Override
public boolean shouldOverrideUrlLoading(WebView view, WebResourceRequest request) {
return false; //false 在WebView加载网页
//Load web pages in WebView
}
//For example, operate on the progress bar
@Override
public void onPageStarted(WebView view, String url, Bitmap favicon) {
super.onPageStarted(view, url, favicon);
mywebview.StartProgress();
//页面加载之前
//Before the page loads
}
@Override
public void onPageFinished(WebView view, String url) {
super.onPageFinished(view, url);
mywebview.FinishProgress();
//页面加载之后
//After the page loads
}
//图片,资源,链接,加载
//Every time you click on the picture, resource,link, load
@Override
public void onLoadResource(WebView view, String url) {
super.onLoadResource(view, url);
}
//更改网络错误 服务器错误界面
// 旧版本,会在新版本中也可能被调用,所以加上一个判断,防止重复显示
@Override
public void onReceivedError(WebView view, int errorCode, String description, String failingUrl) {
super.onReceivedError(view, errorCode, description, failingUrl);
if(Build.VERSION.SDK_INT >= Build.VERSION_CODES.M){
return;
}
if (errorCode == 404) {
//用javascript隐藏系统定义的404页面信息
String data = "Page NO FOUND!";
view.loadUrl("javascript:document.body.innerHTML=\"" + data + "\"");
}
}
// 新版本,只会在Android6及以上调用
@TargetApi(Build.VERSION_CODES.M)
@Override
public void onReceivedError(WebView view, WebResourceRequest request, WebResourceError error) {
super.onReceivedError(view, request, error);
if (request.isForMainFrame()){ // 或者: if(request.getUrl().toString() .equals(getUrl()))
// 在这里显示自定义错误页
int errorCode=error.getErrorCode();
if (errorCode == 404) {
//用javascript隐藏系统定义的404页面信息
String data = "Page NO FOUND!";
view.loadUrl("javascript:document.body.innerHTML=\"" + data + "\"");
}
}
}
// SSL Error. Failed to validate the certificate chain,error: java.security.cert.CertPathValidatorExcept
@Override
public void onReceivedSslError(WebView view, SslErrorHandler handler, SslError error) {
handler.proceed(); //解决方案, 不要调用super.xxxx
}
// 视频全屏播放按返回页面被放大的问题
@Override
public void onScaleChanged(WebView view, float oldScale, float newScale) {
super.onScaleChanged(view, oldScale, newScale);
if (newScale - oldScale > 7) {
view.setInitialScale((int) (oldScale / newScale * 100)); //异常放大,缩回去。
}
}
}
- WebChromeClient设置
package com.example.webview.web;
import android.webkit.WebChromeClient;
import android.webkit.WebView;
public class MyWebChromeClient extends WebChromeClient {
private MyWebView mywebview;
public MyWebChromeClient(MyWebView mywebview) {
this.mywebview =mywebview;
}
@Override
public void onProgressChanged(WebView view, int newProgress) {
mywebview.setMyProgress(newProgress);
super.onProgressChanged(view, newProgress);
}
@Override
public void onReceivedTitle(WebView view, String title) {
super.onReceivedTitle(view, title);
mywebview.setTitle(title);
}
}
清单文件
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.webview">
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme"
android:usesCleartextTraffic="true">
<activity android:name=".web.WebActivity"></activity>
<activity
android:name=".MainActivity"
>
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<uses-library
android:name="org.apache.http.legacy"
android:required="false" />
</application>
</manifest>