model
import java.math.BigDecimal;
import com.alibaba.excel.annotation.ExcelProperty;
import com.alibaba.excel.metadata.BaseRowModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.ToString;
@Data
@ToString
@EqualsAndHashCode
public class StudentExcel extends BaseRowModel {
@ApiModelProperty("姓名")
@ExcelProperty(value = { "姓名", "姓名"}, index = 0)
private String studentName;
@ApiModelProperty("班级")
@ExcelProperty(value = { "班级", "班级"}, index = 1)
private String studentClass;
@ApiModelProperty("数学")
@ExcelProperty(value = { "成绩", "数学"}, index = 2)
private BigDecimal math;
@ApiModelProperty("语文")
@ExcelProperty(value = { "成绩", "语文"}, index = 3)
private BigDecimal language;
@ApiModelProperty("英语")
@ExcelProperty(value = { "成绩", "英语"}, index = 4)
private BigDecimal english;
}
Controller
import java.math.BigDecimal;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletResponse;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.alibaba.excel.ExcelWriter;
import com.alibaba.excel.metadata.Sheet;
import com.alibaba.excel.metadata.Table;
import com.alibaba.excel.support.ExcelTypeEnum;
import com.alibaba.fastjson.JSON;
import com.gemantic.cnooc.model.StudentExcel;
import com.google.common.collect.Lists;
import io.swagger.annotations.ApiOperation;
import lombok.extern.slf4j.Slf4j;
@RestController
@RequestMapping(path = "/student")
@Slf4j
public class StudentExcelController {
private static final String FILE_HEADER_KEY = "Content-Disposition";
private static final String FILE_CONTENT_TYPE = "application/vnd.ms-excel;charset=utf-8";
@PostMapping(value = "exportExcel", headers = "Accept=application/octet-stream")
@ApiOperation(value = "导出excel", notes = "导出excel")
public void exportExcel(HttpServletResponse response) throws Exception {
Map<String, List<StudentExcel>> collect = getList();
try (ServletOutputStream out = response.getOutputStream();) {
ExcelWriter writer = new ExcelWriter(out, ExcelTypeEnum.XLSX);
Sheet sheet1 = new Sheet(1, 0);
sheet1.setSheetName("sheet1");
response.setContentType(FILE_CONTENT_TYPE);
String fileName = URLEncoder.encode("学生成绩", "UTF-8");
response.setHeader(FILE_HEADER_KEY, "attachment;filename=" + fileName + ".xls");
int tableCount = 0;
for (String string : collect.keySet()) {
List<StudentExcel> excelList =
JSON.parseArray(JSON.toJSONString(collect.get(string)), StudentExcel.class);
tableCount = tableCount + 1;
Table table = new Table(tableCount);
table.setClazz(StudentExcel.class);
List<List<String>> list2 = new ArrayList<List<String>>() {
{
add(Arrays.asList(string));
}
};
writer.write(excelList, sheet1, table);
writer.write0(list2, sheet1);
}
writer.finish();
} catch (Exception e) {
log.error("导出详情excel 出现错误", e);
}
}
public Map<String, List<StudentExcel>> getList() {
List<StudentExcel> list = Lists.newArrayList();
StudentExcel a = new StudentExcel();
a.setStudentName("张三");
a.setStudentClass("五年级");
a.setMath(new BigDecimal(99.5));
a.setLanguage(new BigDecimal(99.4));
a.setEnglish(new BigDecimal(99.3));
StudentExcel b = new StudentExcel();
b.setStudentName("李四");
b.setStudentClass("五年级");
b.setMath(new BigDecimal(96.5));
b.setLanguage(new BigDecimal(96.4));
b.setEnglish(new BigDecimal(96.3));
StudentExcel c = new StudentExcel();
c.setStudentName("王五");
c.setStudentClass("四年级");
c.setMath(new BigDecimal(96.5));
c.setLanguage(new BigDecimal(96.4));
c.setEnglish(new BigDecimal(96.3));
list.add(a);
list.add(b);
list.add(c);
Map<String, List<StudentExcel>> map =
list.stream().collect(Collectors.groupingBy(StudentExcel::getStudentClass));
Map<String, List<StudentExcel>> sortMap = new LinkedHashMap(map.size());
map.entrySet().stream().sorted(Map.Entry.<String, List<StudentExcel>>comparingByKey().reversed())
.forEachOrdered(e -> sortMap.put(e.getKey(), e.getValue()));
return sortMap;
}
}
导出结果
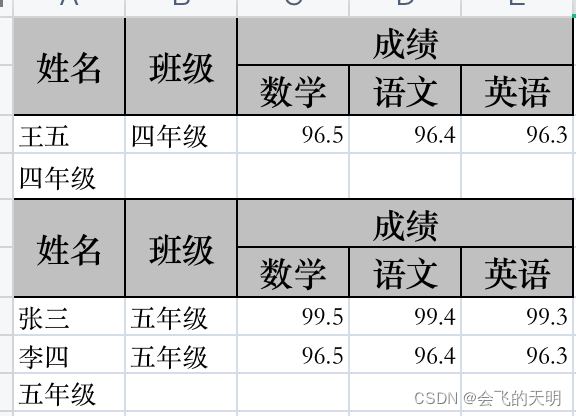