目录
1:默认就是这个几个HandlerExceptionResolver
ExceptionHandlerExceptionResolver
ResponseStatusExceptionResolver
DefaultHandlerExceptionResolver
2:ExceptionHandlerExceptionResolver:对标注@ExceptionHandler的方法进行解析
1:在当前Controller中标注@ExceptionHandler
2:利用@ControllerAdvice和@ExceptionHandler标注公共处理异常的类
3:ResponseStatusExceptionResolver处理自定义异常类
4:异常处理_DefaultHandlerExceptionResolver-Spring自己的异常:
5:SimpleMappingExceptionResolver:通过配置的方式进行异常处理
1:默认就是这个几个HandlerExceptionResolver
在页面渲染之前会提前处理异常
遍历异常,找到对应的那个 ;所有的异常解析器尝试解析,解析完成进行后续,解析失败下一个解析器
默认的异常:
ExceptionHandlerExceptionResolver
ResponseStatusExceptionResolver
DefaultHandlerExceptionResolver
如果异常解析器都不能处理就直接抛出去;
遍历执行的顺序为:
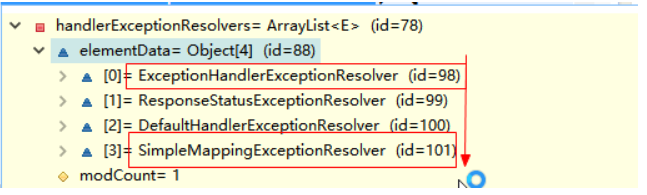
2:ExceptionHandlerExceptionResolver:对标注@ExceptionHandler的方法进行解析
1:在当前Controller中标注@ExceptionHandler
package com.wkl.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
/**
* Description:
* Date: 2020/8/18 - 下午 7:54
* author: wangkanglu
* version: V1.0
*/
@Controller
public class ExceptionController {
@RequestMapping("/handle01")
public String handle01(@RequestParam("i")Integer i){
System.out.println("handle01----");
int y = 10/i;
return "success";
}
@ExceptionHandler(value = {ArithmeticException.class,NullPointerException.class})
public String handleException01(){
System.out.println("error....");
return "error";
}
@ExceptionHandler(value = {Exception.class})
public String handleException02(){
System.out.println("error2....");
return "error";
}
}
2:利用@ControllerAdvice和@ExceptionHandler标注公共处理异常的类
package com.wkl.exception;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.servlet.ModelAndView;
/**
* Description:
* Date: 2020/8/19 - 下午 12:16
* author: wangkanglu
* version: V1.0
*/
@ControllerAdvice
public class GenalException {
/**
* 给方法上接收Exception参数,用来接收发生的异常
* @param ex
* @return
*/
@ExceptionHandler(value = {ArithmeticException.class,NullPointerException.class})
public ModelAndView handleException01(Exception ex){
System.out.println("整体的error....");
ModelAndView modelAndView = new ModelAndView("error");
modelAndView.addObject("exception",ex);
System.out.println("ex:"+ex);
return modelAndView;
}
}
3:如何将异常对象从控制器携带给页面,做异常信息的获取
/**
* 给方法上接收Exception参数,用来接收发生的异常
* @param ex
* @return
*/
@ExceptionHandler(value = {ArithmeticException.class,NullPointerException.class})
public ModelAndView handleException01(Exception ex){
System.out.println("error....");
ModelAndView modelAndView = new ModelAndView("error");
modelAndView.addObject("exception",ex);
System.out.println("ex:"+ex);
return modelAndView;
}
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ page isELIgnored="false" %>
<html>
<head>
<title>错误</title>
</head>
<body>
<h1>错误</h1>
<h3>错误信息:${exception}</h3>
</body>
</html>
4:各个@ExceptionHandler的执行顺序
- @ExceptionHandler 注解定义的方法优先级问题:例如发生的是NullPointerException,但是声明的异常有 RuntimeException 和 Exception,此候会根据异常的最近继承关系找到继承深度最浅的那个 @ExceptionHandler 注解方法,即标记了 RuntimeException 的方法
- ExceptionHandlerMethodResolver 内部若找不到@ExceptionHandler 注解的话,会找 @ControllerAdvice 中的@ExceptionHandler 注解方法
3:ResponseStatusExceptionResolver处理自定义异常类
1:原理:
- 若在处理器方法中抛出了自定义异常:若ExceptionHandlerExceptionResolver 不解析自定义异常。由于触发的异常 自定义异常 带有@ResponseStatus 注解。因此会被ResponseStatusExceptionResolver 解析到。
2:实验代码
自定义异常:
package com.wkl.exception;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ResponseStatus;
/**
* Description:
* Date: 2020/8/19 - 下午 12:36
* author: wangkanglu
* version: V1.0
*/
@ResponseStatus(reason = "就是错了!",value = HttpStatus.NO_CONTENT)
public class MyException extends RuntimeException{
}
抛出异常的类:
@RequestMapping("/handle01")
public String handle01(@RequestParam("i")Integer i){
System.out.println("handle01----"+i);
if(i==10){
System.out.println("xxxxx");
throw new MyException();
}
System.out.println("yyyyy");
return "success";
}
4:异常处理_DefaultHandlerExceptionResolver-Spring自己的异常:
- NoSuchRequestHandlingMethodException、
- HttpRequestMethodNotSupportedException、
- HttpMediaTypeNotSupportedException、
- HttpMediaTypeNotAcceptableException等。
try {
if (ex instanceof NoSuchRequestHandlingMethodException) {
return handleNoSuchRequestHandlingMethod((NoSuchRequestHandlingMethodException) ex, request, response,
handler);
}
else if (ex instanceof HttpRequestMethodNotSupportedException) {
return handleHttpRequestMethodNotSupported((HttpRequestMethodNotSupportedException) ex, request,
response, handler);
}
else if (ex instanceof HttpMediaTypeNotSupportedException) {
return handleHttpMediaTypeNotSupported((HttpMediaTypeNotSupportedException) ex, request, response,
handler);
}
else if (ex instanceof HttpMediaTypeNotAcceptableException) {
return handleHttpMediaTypeNotAcceptable((HttpMediaTypeNotAcceptableException) ex, request, response,
handler);
}
else if (ex instanceof MissingServletRequestParameterException) {
return handleMissingServletRequestParameter((MissingServletRequestParameterException) ex, request,
response, handler);
}
else if (ex instanceof ServletRequestBindingException) {
return handleServletRequestBindingException((ServletRequestBindingException) ex, request, response,
handler);
}
else if (ex instanceof ConversionNotSupportedException) {
return handleConversionNotSupported((ConversionNotSupportedException) ex, request, response, handler);
}
else if (ex instanceof TypeMismatchException) {
return handleTypeMismatch((TypeMismatchException) ex, request, response, handler);
}
else if (ex instanceof HttpMessageNotReadableException) {
return handleHttpMessageNotReadable((HttpMessageNotReadableException) ex, request, response, handler);
}
else if (ex instanceof HttpMessageNotWritableException) {
return handleHttpMessageNotWritable((HttpMessageNotWritableException) ex, request, response, handler);
}
else if (ex instanceof MethodArgumentNotValidException) {
return handleMethodArgumentNotValidException((MethodArgumentNotValidException) ex, request, response, handler);
}
else if (ex instanceof MissingServletRequestPartException) {
return handleMissingServletRequestPartException((MissingServletRequestPartException) ex, request, response, handler);
}
else if (ex instanceof BindException) {
return handleBindException((BindException) ex, request, response, handler);
}
else if (ex instanceof NoHandlerFoundException) {
return handleNoHandlerFoundException((NoHandlerFoundException) ex, request, response, handler);
}
}
catch (Exception handlerException) {
logger.warn("Handling of [" + ex.getClass().getName() + "] resulted in Exception", handlerException);
}
return null;
}
5:SimpleMappingExceptionResolver:通过配置的方式进行异常处理
当上述异常配置都没生效的时候,可以通过我们配置的特定错误,映射的页面,直接来到页面
在dispatcher-servlet.xml中配置
<bean class="org.springframework.web.servlet.handler.SimpleMappingExceptionResolver"><!-- exceptionMappings:配置哪些异常去哪些页面 --><property name="exceptionMappings"><props><!-- key:异常全类名;value:要去的页面视图名; --><prop key="java.lang.NullPointerException">myerror</prop></props></property><!--指定错误信息取出时使用的key --><property name="exceptionAttribute" value="ex"></property></bean>