一、String
1、创建字符串
string s = new String("新字符串")
2、字符串的不变性
String 对象创建后则不能被修改,是不可变的,所谓的修改其实是创建了新的对象,所指向的内存空间不同。
public class Demo {
public static void main(String[] args) {
String str1 = "数据结构";
String str2 = "数据结构";
String str3 = new String("数据结构");
String str4 = new String("数据结构");
//多次出现的字符常量,Java编译器只能创建一个,所以返回true
System.out.println(str1==str2);
//str1与str3是不同的对象,所以返回false
System.out.println(str1==str3);
//str3与str4是不同的对象,所以返回false
System.out.println(str3==str4);
}
}
3、连接多个字符串
public class Demo {
public static void main(String[] args) {
String s1 = new String("字");
String s2 = new String("符");
String s3 = new String("串");
String s = s1+s2+s3;
System.out.println(s);
}
}
4、连接其他数据类型
package DemoClass;
public class Demo {
public static void main(String[] args) {
String s1 = new String("每天 ");
int t = 24;
String s2 = new String(" 个小时");
System.out.println(s1+t+s2);
}
}
5、获取字符串长度
package DemoClass;
public class Demo {
public static void main(String[] args) {
String str = "异常就是从一个异常类创建的对象";
int size = str.length();
System.out.println(size);
}
}
二、字符串查找
1、indexOf()和lastIndexOf()
indexOf()方法返回的是搜索的字符或字符串首次出现的位置
lastIndexOf()方法返回的是搜索的字符或字符串最后一次出现的位置,如果lastIndexOf()方法中的参数是空字符串“”(没有空格),则返回的结果与调用该字符串length()方法的返回结果相同
语法
str.indexOf("substr")
str.lastIndexOf("substr")
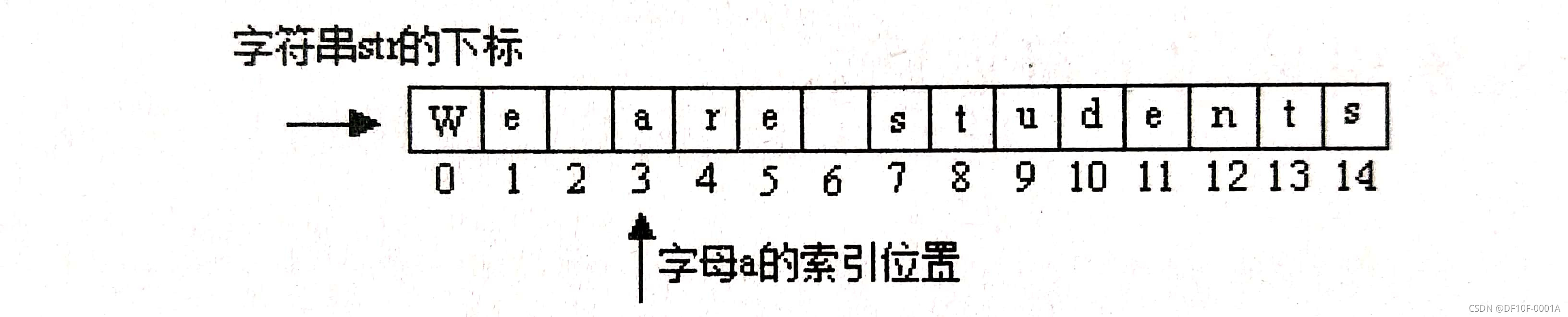
代码:
package DemoClass;
public class Demo {
public static void main(String[] args) {
String str = "异常就是从一个异常类创建的对象";
int size = str.indexOf("是");
System.out.println(size);
}
}
public class Demo {
public static void main(String[] args) {
String str = "异常就是从一个异常类创建的对象";
System.out.println(str.lastIndexOf("一"));
}
}
2、获取指定索引位置的字符
语法
str.charA(int index)
代码:
public class Demo {
public static void main(String[] args) {
String str = "异常就是从一个异常类创建的对象";
char maychar = str.charAt(6);
System.out.println(maychar);
}
}
3、获取字符串
substring(int beginIndex),该方法返回的是从指定索引位置开始截取直到该字符串结尾的子串。
语法
str.substring(int beginIndex)
代码:
public class Demo {
public static void main(String[] args) {
String str = "异常就是从一个异常类创建的对象";
String substr = str.substring(5);
//截取从第五个字符之后的字段
System.out.println(substr);
}
}
substring(int beginIndex,int endIndex),该方法返回的是从字符串某一索引位置开始至某一索引位置结束的字符串
语法
str.substring(int beginIndex,int endIndex)
代码:
public class Demo {
public static void main(String[] args) {
String str = "异常就是从一个异常类创建的对象";
String substr = str.substring(3,10);
//截取从第五个字符之后的字段
System.out.println(substr);
}
}
4、去除空格
语法
str.trim()
只能忽略前导空格和尾部空格,不能忽略字符串中的空格
public class Demo {
public static void main(String[] args) {
String str = " 异常 类 ";
System.out.println("字符串原长:"+str.length());
System.out.println("去除空格后的字符串长:"+str.trim().length());
}
}
5、字符串替换
语法
str.replace(char oldChar,char newChar)
代码:
public class Demo {
public static void main(String[] args) {
String str = "异常就是从一个异常类创建的对象";
String newstr = str.replace("一","二");
System.out.println(newstr);
}
}
6、判断字符串的开始与结尾
startsWith(),该方法用于判断当前字符串对象的前缀是否为参数指定的字符串
语法
str.startsWith(String prefix)
代码:
public class Demo {
public static void main(String[] args) {
String num = "123456789";
boolean n1 = num.startsWith("1");
System.out.println("字符串num是以1开头的吗?"+n1);
}
}
endWith(),该方法用于判断当前字符串是否以给定的子字符串结束
语法:
str.endsWith(String suffix)
代码:
public class Demo {
public static void main(String[] args) {
String num = "123456789";
boolean n1 = num.startsWith("1");
System.out.println("字符串num是以9结尾的吗?"+n1);
}
}
7、判断字符串是否相等
比较运算符”==“,比较两个字符串的地址是否相同
public class Demo {
public static void main(String[] args) {
String name1 = "Mr. Wang";
String name2 = "Ms. Wang";
boolean n =(name1==name2);
System.out.println("name1与name2是否相同? "+n);
}
}
euqals()方法,如果两个字符串有相同的字符和长度,则使用此方法比较内容,且此方法区分大小写
语法:
str.equals(String otherstr)
代码:
public class Demo {
public static void main(String[] args) {
String name1 = "Mr. Wang";
String name2 = "Mr. Wang";
boolean n =name1.equals(name2);
System.out.println("name1与name2内容是否相同? "+n);
}
}
equalsgnoreCase(String otherstr)方法,和euqals()方法一样,只不过不区分大小写
语法:
str.equalsgnoreCase(String otherstr)
代码:
public class Demo {
public static void main(String[] args) {
String name1 = "Mr. Wang";
String name2 = "Mr. Wang";
boolean n =name1.equalsIgnoreCase(name2);
System.out.println("name1与name2内容是否相同? "+n);
}
}
8、按字典顺序比较两个字符
compareT0()方法,该比较基于字符串中各个字符的Unicode值,按字典顺序将此String对象表示的字符序列与参数字符串所表示的字符序列进行比较。如果按字典顺序此String对象位于参数字符之前则比较结果为一个负整数,如果按字典顺序此String对象位于参数字符串之后则比较结果为一个正整数,如果这两个字符串相等,则结果为0。只有在equals(Object)方法返回true时才返回0。
语法:
str. compareT0(String otherstr)
代码:
public class Demo {
public static void main(String[] args) {
String str1 = "a";
String str2 = "b";
System.out.println(str1+"compareTo"+str2+":"+ str1.compareTo(str2));
//将str1与str2比较的结果输出
System.out.println(str2+"compareTo"+str1+":"+ str2.compareTo(str1));
//将str2与str1比较的结果输出
}
}
三、StringBuilder
1、StringBuilder 类的常用方法
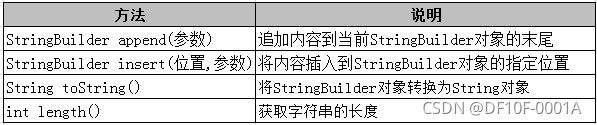
代码:
public static void main(String[] args) {
// 创建一个空的StringBuilder对象
StringBuilder str = new StringBuilder();
// 追加字符串
str.append("qwertyuiopasdfghjklzxcvbnm");
// 从后往前每隔三位插入逗号
for(int i = str.length()-3; i>=0 ;i=i-3){
str.insert(i,",");
}
// 将StringBuilder对象转换为String对象并输出
System.out.print(str.toString());
}
2、格式化字符串
String类的静态format()方法用于创建格式化的字符串。format()有两种重载形式
(1)、format(String format,Object...args)
语法
str.format(String format,Object...args)
(2)、format(Local I,String format,Object...args)
3、时间和日期字符串格式化
(1)、日期格式化
常用的日期格式化转换符
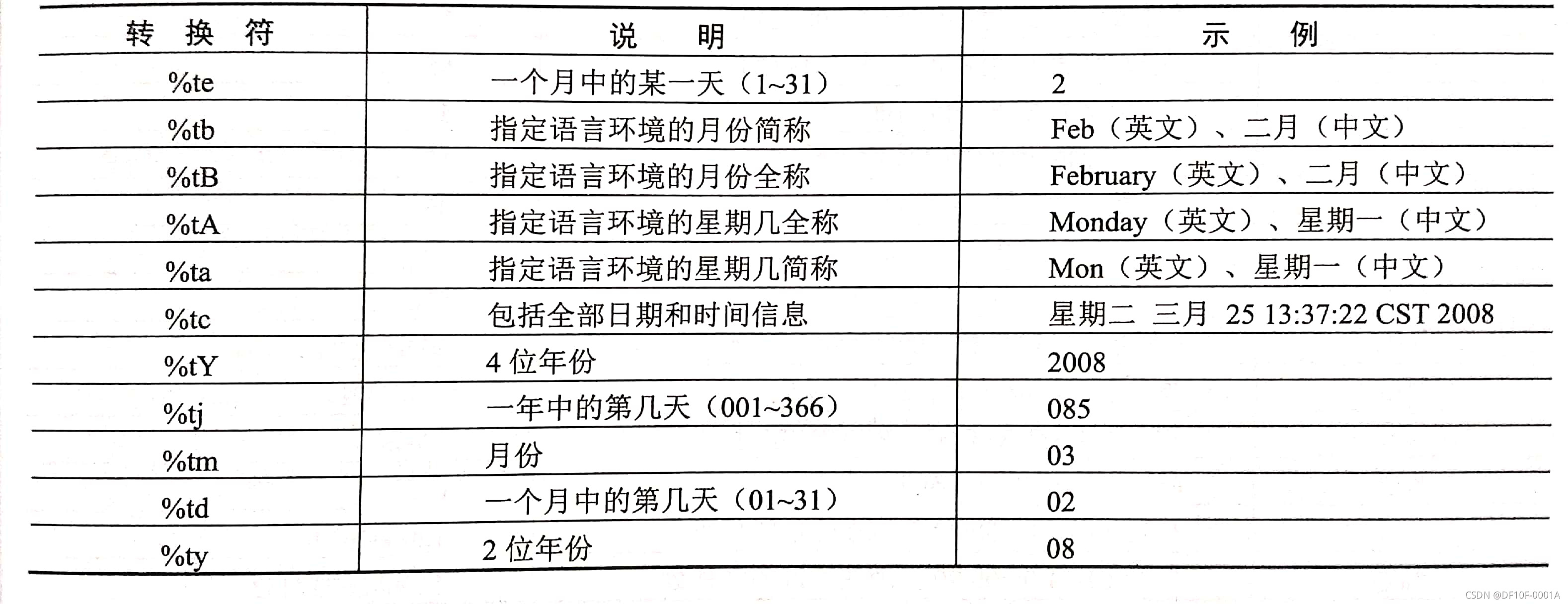
代码:
import java.util.Date;
//导入java.util.Date类
public class Demo {
public static void main(String[] args) {
Date date = new Date();
//将date进行格式化
String years = String.format("%tY",date);
String month = String.format("%tB",date);
String day = String.format("%td",date);
System.out.println("年:"+years);
System.out.println("月:"+month);
System.out.println("日:"+day);
}
}
(2)、时间格式化
常用的时间格式化转换符
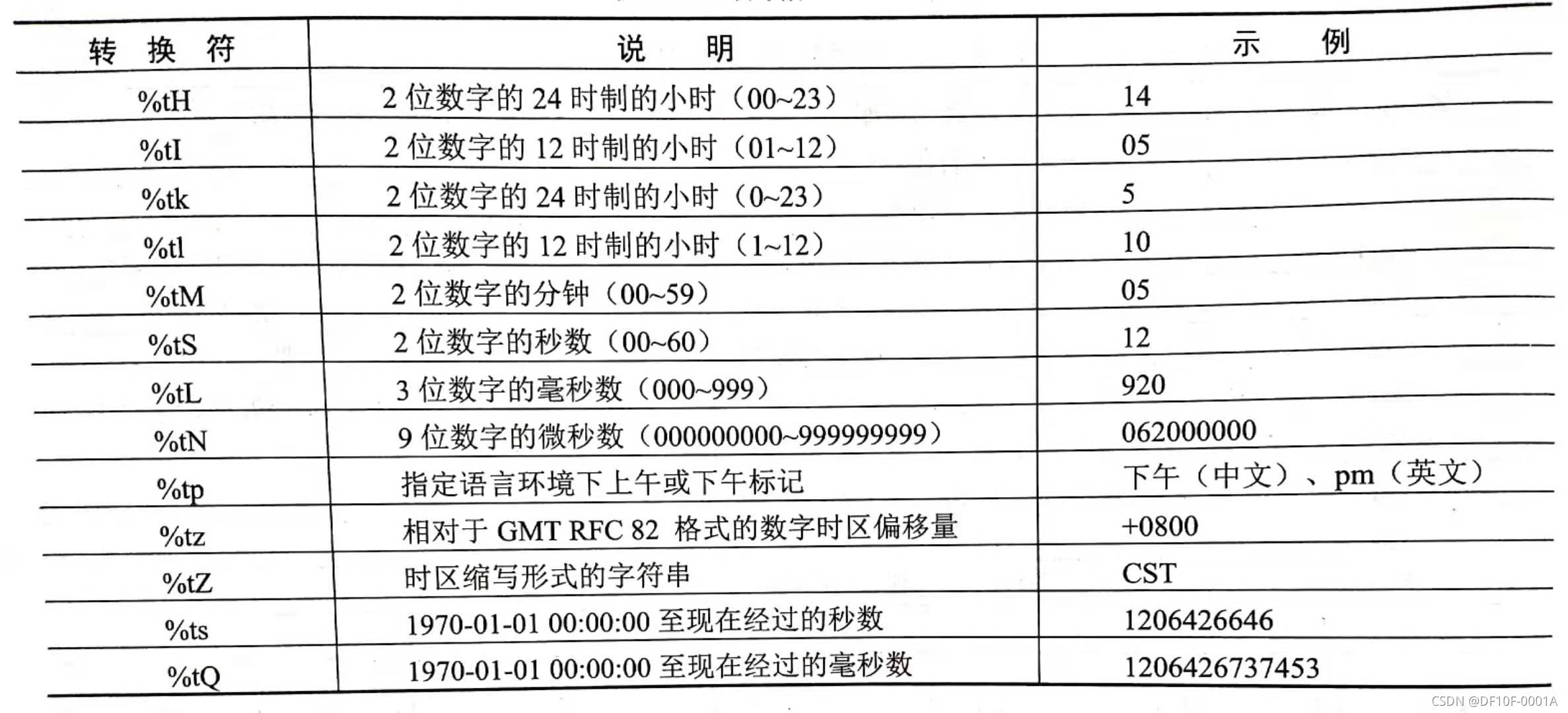
代码:
import java.util.Date;
//导入java.util.Date类
public class Demo {
public static void main(String[] args) {
Date date = new Date();
//将date进行格式化
String hour = String.format("%tH",date);
String minute = String.format("%tM",date);
String second = String.format("%tS",date);
System.out.println("时:"+hour);
System.out.println("分:"+minute);
System.out.println("秒:"+second);
}
}
(3)、格式化常见的日期时间组合
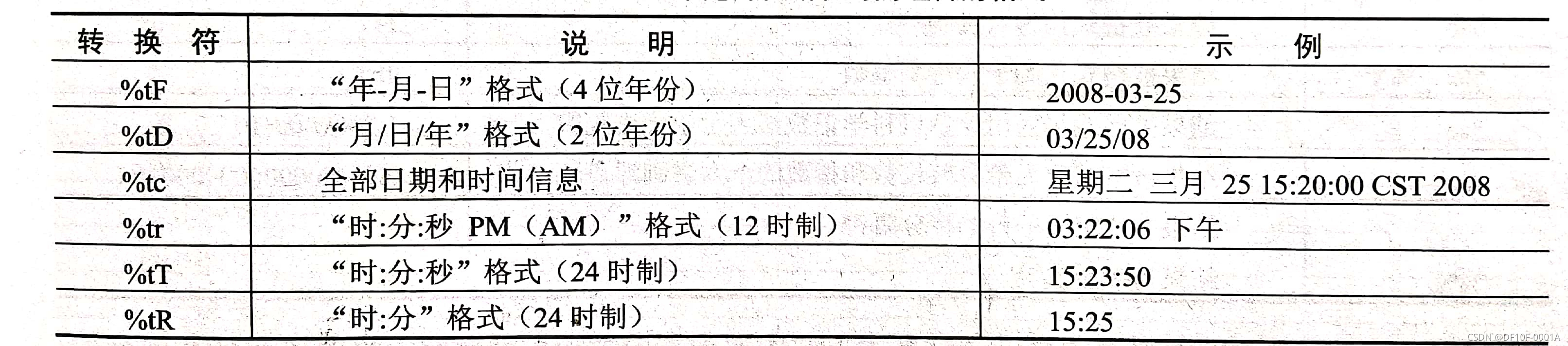
代码:
import java.util.Date;
//导入java.util.Date类
public class Demo {
public static void main(String[] args) {
Date date = new Date();
//将date进行格式化
String time = String.format("%tc",date);
String form = String.format("%tF",date);
System.out.println("全部日期和时间信息:"+time);
System.out.println("年-月-日:"+form);
}
}
(4)、常规类型格式转化
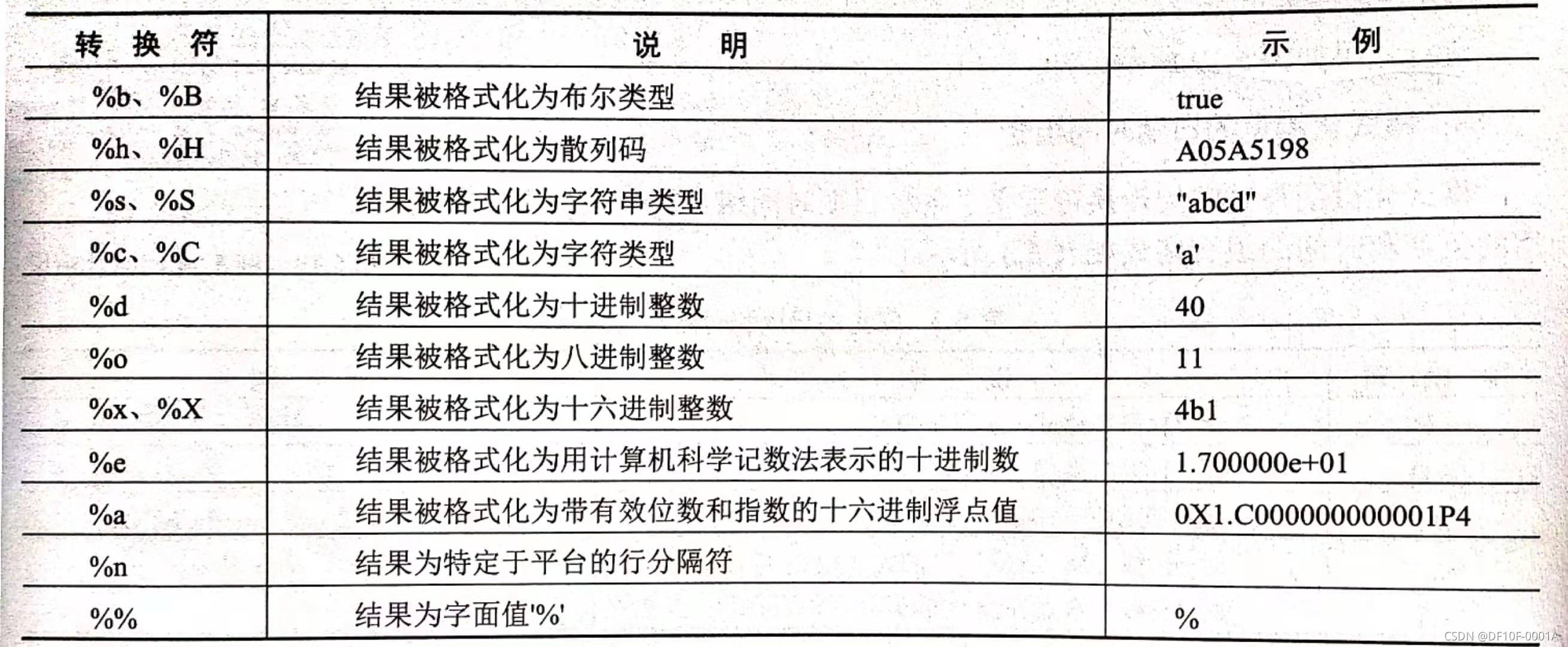