1、MagCode,错误码枚举类
package com.mgx.common.enums;
import lombok.*;
import lombok.extern.slf4j.Slf4j;
/**
* 错误码
* @author mgx
*/
@Slf4j
@NoArgsConstructor
@AllArgsConstructor
public enum MsgCode {
/**
* 枚举标识,根据业务类型进行添加
*/
Code_200("操作成功",200)
,Code_400("错误请求", 400)
,Code_401("未授权", 401)
,Code_402("权限不足,请联系管理员", 402)
,Code_403("禁止请求", 403)
,Code_404("找不到相关内容", 404)
,Code_408("请求超时", 408)
,Code_410("该资源已删除", 410)
,Code_413("请求体过大", 413)
,Code_414("请求URI过长", 414)
,Code_415("不支持的媒体类型", 415)
,Code_500("系统错误", 500)
,Code_501("用户未登录", 501)
,Code_502("错误网关", 502)
,Code_503("服务不可用", 503)
,Code_504("网关超时", 504)
,Code_505("HTTP版本暂不支持", 505)
,Code_506("时间格式错误", 506)
//自定义提示
,Code_2001("参数错误", 2001)
,Code_2002("传入参数不符合条件", 2002)
,Code_2003("缺少请求参数", 2003)
,Code_2004("请求方式错误", 2004)
,Code_2005("sql语法错误", 2005)
,Code_2006("数据不存在", 2006)
,Code_2007("同步失败", 2007)
,Code_2008("添加失败", 2008)
,Code_2009("修改失败", 2009)
,Code_2010("删除失败", 2010)
,Code_2011("查询失败", 2011)
,Code_2012("评估失败", 2012)
,Code_2013("移出黑名单失败",2013)
,Code_2014("该车辆已在黑名单中",2014)
,Code_2015("请输入正确的手机号",2015)
,Code_2016("不支持的编码格式", 2016)
,Code_2017("不支持的解码格式", 2017)
,Code_2018("保存失败", 2018)
;
@Getter
@Setter
private String msg;
@Getter
@Setter
private Integer code;
public static String getMessage(Integer code){
MsgCode msgCode;
try {
msgCode = Enum.valueOf(MsgCode.class, "Code_" + code);
} catch (IllegalArgumentException e) {
log.error("传入枚举code错误!code:{}",code);
return null;
}
return msgCode.getMsg();
}
}
2、统一返回结果类
package com.mgx.common.dto;
import com.mgx.common.enums.MsgCode;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import java.io.Serializable;
/**
* @author mgx
*/
@AllArgsConstructor
@NoArgsConstructor
public class Result<T> implements Serializable {
private static final long serialVersionUID = 1L;
/** 成功与否 */
@Getter protected boolean success;
/** 结果代码 */
@Getter protected Integer code;
/** 消息 */
@Getter protected String message;
/** 标识(方便接口调试) */
@Getter @Setter
protected String tag;
/** 版本(方便接口调试) */
@Getter @Setter
protected String version;
/** 结果数据 */
@Getter protected T data;
/**
* 不需指定code和message
* @return SuccessBuilder
*/
public static SuccessBuilder success() {
return new SuccessBuilder(Boolean.TRUE, MsgCode.Code_200.getCode(), MsgCode.Code_200.getMsg());
}
/**
* 同时指定code和message
* @return FailBuilder
*/
public static FailBuilder failure() {
return new FailBuilder(Boolean.FALSE);
}
public static class SuccessBuilder {
protected boolean success;
protected Integer code;
protected String message;
protected String tag = "mgx";
protected String version = "1.0";
protected Object data;
protected SuccessBuilder(boolean success, Integer code, String message) {
this.success = success;
this.code = code;
this.message = message;
}
public SuccessBuilder data(Object data) {
this.data = data;
return this;
}
@SuppressWarnings("unchecked")
public <T> Result<T> build() {
return new Result<>(success, code, message, tag, version, (T) data);
}
}
public static class FailBuilder {
protected boolean success;
protected Integer code;
protected String message;
protected String tag = "mgx";
protected String version = "1.0";
protected Object data;
protected FailBuilder(boolean success) {
this.success = success;
}
public FailBuilder code(Integer code) {
this.code = code;
String message = MsgCode.getMessage(code);
if(message != null){
this.message = message;
}
return this;
}
//如果调用code再调用此message方法,此message方法会覆盖code方法中set的message
public FailBuilder message(String message) {
this.message = message;
return this;
}
public FailBuilder data(Object data) {
this.data = data;
return this;
}
@SuppressWarnings("unchecked")
public <T> Result<T> build() {
return new Result<>(success, code, message, tag, version, (T) data);
}
}
}
3、BooleanResult封装
package com.mgx.common.dto;
import lombok.Data;
import java.io.Serializable;
/**
* @author mgx
*/
@Data
public class BooleanResult implements Serializable {
private Boolean result;
private String reason;
public static BooleanResult success(){
BooleanResult booleanResult = new BooleanResult();
booleanResult.setResult(true);
return booleanResult;
}
public static BooleanResult fail(){
return fail(null);
}
public static BooleanResult fail(String reason){
BooleanResult booleanResult = new BooleanResult();
booleanResult.setResult(false);
booleanResult.setReason(reason);
return booleanResult;
}
}
4、结构展示
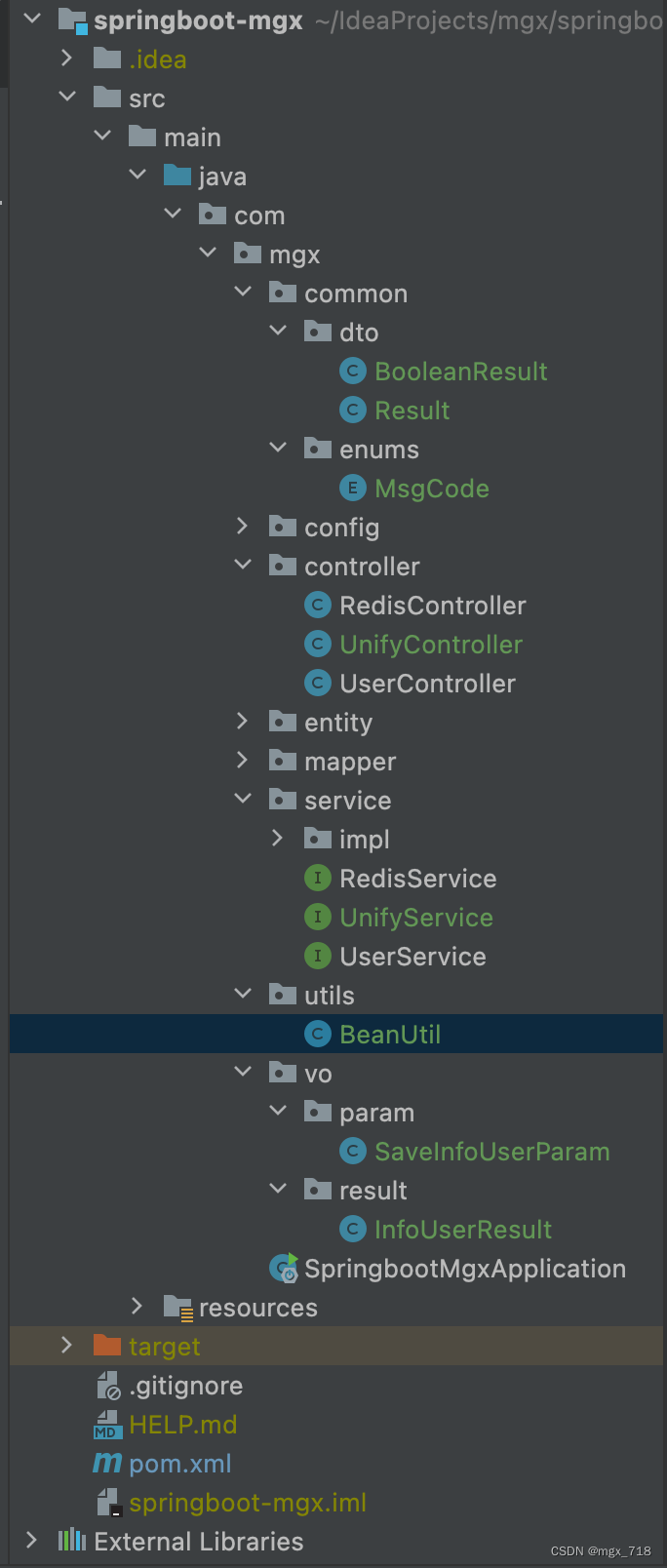
5、类
package com.mgx.controller;
import com.mgx.common.dto.BooleanResult;
import com.mgx.common.dto.Result;
import com.mgx.service.UnifyService;
import com.mgx.vo.param.SaveInfoUserParam;
import com.mgx.vo.result.InfoUserResult;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.apache.ibatis.annotations.Param;
import org.springframework.web.bind.annotation.*;
import javax.annotation.Resource;
/**
* @author mgx
* @date 2023/9/18 3:36 PM
*/
@Api(tags = "springboot整合 统一类型结果集")
@RestController
@RequestMapping("/Unify")
public class UnifyController {
@Resource
private UnifyService unifyService;
@ApiOperation("新增")
@PostMapping("/add")
public Result<BooleanResult> add(@RequestBody SaveInfoUserParam saveInfoUserParam) {
return Result.success().data(unifyService.add(saveInfoUserParam)).build();
}
@ApiOperation("详情")
@GetMapping("/detail")
public Result<InfoUserResult> detail(@ApiParam("用户信息的ID") @Param("id") Long id) {
return Result.success().data(unifyService.detail(id)).build();
}
@ApiOperation("删除")
@DeleteMapping("/delete")
public Result<BooleanResult> delete(@ApiParam("用户信息的ID") @Param(value = "id") Long id){
return Result.success().data(unifyService.delete(id)).build();
}
@ApiOperation("更新")
@PutMapping("/update")
public Result<BooleanResult> update(@RequestBody SaveInfoUserParam saveInfoUserParam){
return Result.success().data(unifyService.update(saveInfoUserParam)).build();
}
}
package com.mgx.service;
import com.mgx.common.dto.BooleanResult;
import com.mgx.vo.param.SaveInfoUserParam;
import com.mgx.vo.result.InfoUserResult;
/**
* @author mgx
* @date 2023/9/18 3:38 PM
*/
public interface UnifyService {
BooleanResult add(SaveInfoUserParam saveInfoUserParam);
InfoUserResult detail(Long id);
BooleanResult delete(Long id);
BooleanResult update(SaveInfoUserParam saveInfoUserParam);
}
package com.mgx.service.impl;
import com.mgx.common.dto.BooleanResult;
import com.mgx.entity.InfoUser;
import com.mgx.mapper.InfoUserMapper;
import com.mgx.service.UnifyService;
import com.mgx.utils.BeanUtil;
import com.mgx.vo.param.SaveInfoUserParam;
import com.mgx.vo.result.InfoUserResult;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.Objects;
/**
* @author mgx
* @date 2023/9/18 3:38 PM
*/
@Service
public class UnifyServiceImpl implements UnifyService {
@Resource
private InfoUserMapper infoUserMapper;
@Override
public BooleanResult add(SaveInfoUserParam saveInfoUserParam) {
InfoUser infoUser = BeanUtil.map(saveInfoUserParam,InfoUser.class);
int addRow = infoUserMapper.insert(infoUser);
if (addRow < 1){
return BooleanResult.fail();
}
return BooleanResult.success();
}
@Override
public InfoUserResult detail(Long id) {
InfoUser infoUser = infoUserMapper.selectByPrimaryKey(id);
if (Objects.isNull(infoUser)){
throw new RuntimeException("数据不存在");
}
return BeanUtil.map(infoUser,InfoUserResult.class);
}
@Override
public BooleanResult delete(Long id) {
InfoUser infoUser = infoUserMapper.selectByPrimaryKey(id);
if (Objects.isNull(infoUser)){
throw new RuntimeException("数据不存在");
}
int deleteRow = infoUserMapper.deleteByPrimaryKey(id);
if (deleteRow<1){
return BooleanResult.fail();
}
return BooleanResult.success();
}
@Override
public BooleanResult update(SaveInfoUserParam saveInfoUserParam) {
InfoUser queryInfoUser = infoUserMapper.selectByPrimaryKey(saveInfoUserParam.getId());
if (Objects.isNull(queryInfoUser)){
throw new RuntimeException("数据不存在");
}
InfoUser infoUser = BeanUtil.map(saveInfoUserParam,InfoUser.class);
int updateRow = infoUserMapper.updateByPrimaryKeySelective(infoUser);
if (updateRow<1){
return BooleanResult.fail();
}
return BooleanResult.success();
}
}
package com.mgx.utils;
import org.apache.commons.collections4.MapUtils;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.BeansException;
import java.beans.BeanInfo;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Method;
import java.util.*;
/**
* @author mgx
*/
public class BeanUtil {
public BeanUtil() {}
public static <T> T map(Object source, Class<T> target) {
if (null == source) {
return null;
} else {
T t = BeanUtils.instantiateClass(target);
BeanUtils.copyProperties(source, t);
return t;
}
}
public static <T> List<T> mapList(Collection<?> sourceList, Class<T> target) {
if (sourceList == null) {
return null;
} else {
List<T> destinationList = new ArrayList<>();
for (Object sourceObject : sourceList) {
T newObj = map(sourceObject, target);
destinationList.add(newObj);
}
return destinationList;
}
}
public static void copyProperties(Object source, Object target, String... ignoreProperties) {
if (null != source && null != target) {
BeanUtils.copyProperties(source, target, ignoreProperties);
}
}
public static <T> T convert(Object source, Class<T> targetClass) {
if (source == null) {
return null;
} else {
try {
T result = targetClass.newInstance();
copyProperties(source, result);
return result;
} catch (IllegalAccessException | BeansException | InstantiationException var3) {
throw new RuntimeException(var3);
}
}
}
public static void copyProperties(Object source, Object target) throws BeansException {
BeanUtils.copyProperties(source, target);
if (target instanceof ConversionCustomizble) {
((ConversionCustomizble) target).convertOthers(source);
}
}
public interface ConversionCustomizble {
void convertOthers(Object var1);
}
public static Map<String, Object> beanToMap(Object beanObj) {
if (Objects.isNull(beanObj)) {
return null;
}
Map<String, Object> map = new HashMap<>();
try {
BeanInfo beanInfo = Introspector.getBeanInfo(beanObj.getClass());
PropertyDescriptor[] propertyDescriptors = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor property : propertyDescriptors) {
String key = property.getName();
if (key.compareToIgnoreCase("class") == 0) {
continue;
}
Method getter = property.getReadMethod();
Object value = Objects.isNull(getter) ? null : getter.invoke(beanObj);
map.put(key, value);
}
return map;
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
public static <T> T mapToBean(Map<String, Object> map, Class<T> beanClass) {
if (MapUtils.isEmpty(map)) {
return null;
}
try {
T t = beanClass.newInstance();
BeanInfo beanInfo = Introspector.getBeanInfo(t.getClass());
PropertyDescriptor[] propertyDescriptors = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor property : propertyDescriptors) {
Method setter = property.getWriteMethod();
if (Objects.nonNull(setter)) {
setter.invoke(t, map.get(property.getName()));
}
}
return t;
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
}
6、测试
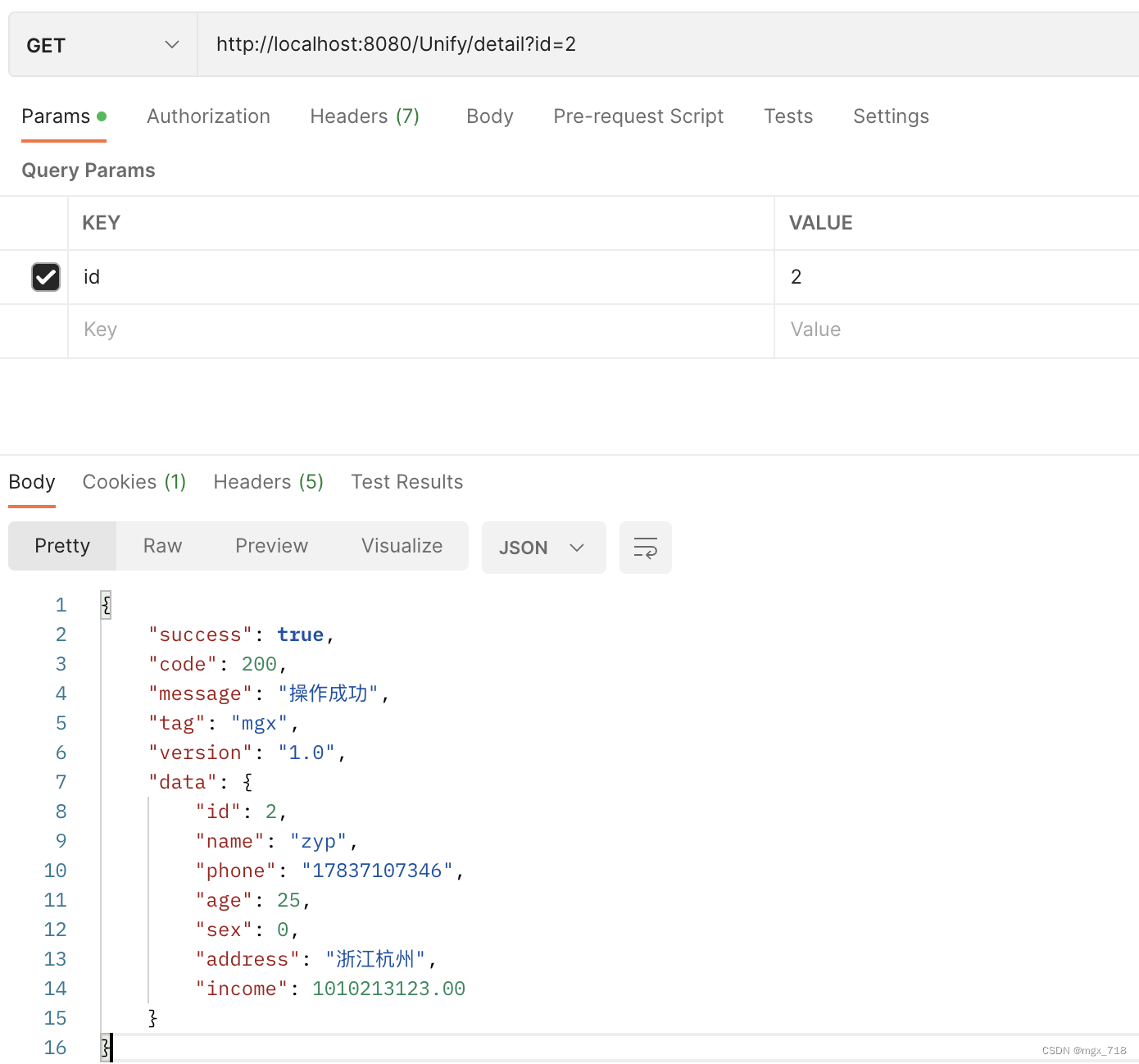
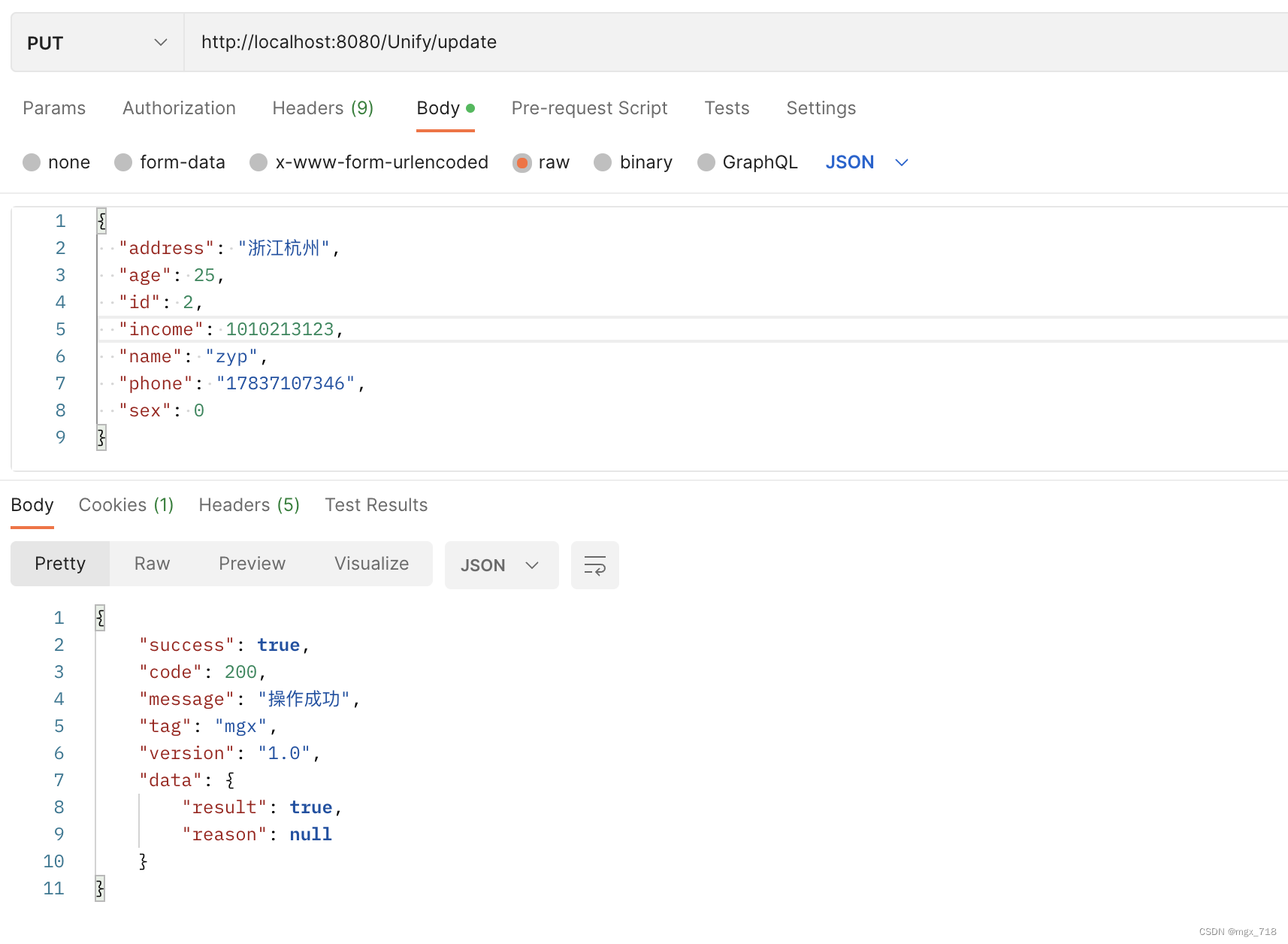