函数目录
思维导图(自定义)
函数具体用法
方法名:++
使用规范:
</font>def ++[B >: A, That](that : scala.collection.GenTraversableOnce[B])(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述: 合并两个序列,若类型不同合并之后一般为Array[Any]
val a = Array(1,2,3)
val b = Array(4,5,6)
val c = List(7,8,9)
val d = a ++ b
val d = a ++ c
val d = c ++ a
方法名:++:
使用规范:
def ++:[B >: A, That](that: collection.Traversable[B])(implicit bf: CanBuildFrom[Array[T], B, That]): That
具体描述:将两个集合中的元素合并到一个新的集合中,新集合的类型由“:”后面的集合类型决定
val a = List(1, 2)
val b = scala.collection.mutable.LinkedList(3, 4)
val c = a ++: b
println(c.getClass().getName()) // c 的类型: scala.collection.mutable.LinkedList
方法名:/:
使用规范: def /:[B](z: B)(op: (B, T) => B): B
具体描述:对数组中所有的元素从左向右遍历,进行相同的迭代操作,foldLeft 的简写
val a = List(1, 2, 3, 4)
val b = (10 /: a) (_ + _) // (((10+1)+2)+3)+4
val c = (10 /: a) (_ * _) // (((10*1)*2)*3)*4
println("b: " + b) // b: 20
println("c: " + c) // c: 240
方法名::+
使用规范:def :+(elem: A): Array[A]
具体描述:在数组后面添加一个元素,并返回新的数组对象
val a = List(1, 2)
val b = a :+ 3
println(b.mkString(",")) // 1,2,3
方法名::\
使用规范: def :[B](z: B)(op: (T, B) ⇒ B): B
具体描述:对数组中所有的元素从右向左遍历,进行相同的迭代操作,foldRight 的简写,返回计算结果
val a = List(1, 2, 3, 4)
val b = (a :\ 10) (_ - _) // 1-(2-(3-(4-10)))
val c = (a :\ 10) (_ * _) // 1*(2*(3*(4*10)))
方法名:+:
使用规范:
def +:[B >: Int](elem: B)(implicit evidence$3: scala.reflect.ClassTag[B]): Array[B]
具体描述:在数组前面添加一个元素,并返回新的数组对象
val a = List(1, 2)
val b = 3 +: a
println(b.mkString(",")) //3,,2
方法名:addString
使用规范: 三种运用方法
def addString(b : scala.StringBuilder, start : scala.Predef.String, sep : scala.Predef.String, end : scala.Predef.String) : scala.StringBuilder = { /* compiled code */ }
def addString(b : scala.StringBuilder, sep : scala.Predef.String) : scala.StringBuilder = { /* compiled code */ }
def addString(b : scala.StringBuilder) : scala.StringBuilder = { /* compiled code */ }
具体描述:将数组中的元素逐个添加到StringBuilder中
val a = List(1, 2, 3, 4)
val b = new StringBuilder()
a.addString(b)
println(b) // 1234
val a = List(1, 2, 3, 4)
val b = new StringBuilder()
a.addString(b, ",")
println(b) // 1,2,3,4
val a = List(1, 2, 3, 4)
val b = new StringBuilder()
a.addString(b, "[",",","]")
println(b) // [1,2,3,4]
方法名:aggregate
使用规范: def aggregate[B](z: ⇒ B)(seqop: (B, T) ⇒ B, combop: (B, B) ⇒ B): B
具体描述:聚合计算,aggregate 是柯里化方法,参数是两个方法
为了方便理解,把 aggregate 的两个参数分别封装成两个方法,并把分区和不分区的计算过程分别打印出来
def seqno(m: Int, n: Int): Int = {
val s = "seq_exp = %d + %d"
println(s.format(m, n))
m + n
}
def combine(m: Int, n: Int): Int = {
val s = "com_exp = %d + %d"
println(s.format(m, n))
m + n
}
def main(args: Array[String]) {
val a = List(1, 2, 3, 4)
val b = a.aggregate(5)(seqno, combine) // 不分区
println("b = " + b)
/**
* seq_exp = 5 + 1
* seq_exp = 6 + 2
* seq_exp = 8 + 3
* seq_exp = 11 + 4
* b = 15
*/
val c = a.par.aggregate(5)(seqno, combine) // 分区
println("c = " + c)
/**
* seq_exp = 5 + 3
* seq_exp = 5 + 2
* seq_exp = 5 + 4
* seq_exp = 5 + 1
* com_exp = 6 + 7
* com_exp = 8 + 9
* com_exp = 13 +17
* c = 30
*/
}
方法名:andThen
使用规范:
override def andThen[C](k : scala.Function1[B, C]) : scala.PartialFunction[A, C] = { /* compiled code */ }
具体描述:表示方法的连续调用,相当于嵌套函数 g(f(x)) (需满足第一个函数的返回值类型是第二个函数的输入值类型),与 compose 相反
def f(a:Int) = {
println("2*"+a)
2*a
}
def g(b:Int) = {
println("3*"+b)
3*b
}
def result1 = f _ andThen g _
println(result1(1))
/*
2*1
2*3
6
*/
def result2 = f _ compose g _
println(result2(1))
/*
3*1
3*2
6
*/
方法名:apply
使用规范:def apply(i : scala.Int) : T = { /* compiled code */ }
具体描述: 按下标取数组数据
val a = Array(1,2,3,4)
a.apply(2) //等同于直接写a(2)
a(2)
方法名:applyOrElse
使用规范:
def applyOrElse[A1 <: A, B1 >: B](x : A1, default : scala.Function1[A1, B1]) : B1 = { /* compiled code */ }
具体描述:接收2个参数,第一个是调用的参数,第二个是个回调函数。如果第一个调用的参数匹配,返回匹配的值,否则调用回调函数。(回调函数的输入类型需与调用参数类型一致)
val pf1:PartialFunction[Int,String] = { //定义一个偏函数,输入 1 返回 "one"
case i if i == 1 => "One"
}
pf1.applyOrElse(1,{num:Int=>"two"}) //输入1匹配到"one"
pf1.applyOrElse(2,{num:Int=>"two"}) //输入2匹配失败则触发回调函数,返回 "Two" 字符串
pf1.applyOrElse(3,{num:Int=>"two"}) //输入3匹配失败则触发回调函数,返回 "Two" 字符串
方法名:array
使用规范: 同其他语言数组的定义没什么区别
具体描述:Array是scala中数组是用来存储同类型的元素结构,数组中本身size不可变,但是数组中元素可变,可重新赋值
val arr = Array(1, 2, 3, 4) // 声明一个数组对象
val first = arr(0) // 读取第一个元素
arr(3) = 100 // 替换第四个元素为 100
val newarr = arr.map(_ * 2) // 所有元素乘 2
println(newarr.mkString(",")) // 打印数组,结果为:2,4,6,200
方法名:canEqual
使用规范:def canEqual(that: Any): Boolean
具体描述:判断两个对象是否可以进行比较
这个方法基本可以忽略
val a = List(1, 2, 3, 4)
val b = Array('a', 'b', 'c')
println(a.canEqual(b)) // true
方法名:clone
使用规范:def clone(): Array[T]
具体描述:创建一个集合的副本
注意:clone是浅拷贝,拷贝的数组中的引用,在引用指向的地址中的值发生变化时,副本的值也会发生变化
val a = Array(1,2,3,4)
val b = a.clone()
方法名:collect
使用规范:
def collect[B, That](pf : scala.PartialFunction[A, B])(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:输入参数类型为偏函数,对序列中的元素进行转换
(scala里的偏函数定义:对给的集合中的部分值进行特定的操作的函数,就时偏函数)
val fun: PartialFunction[Char, Char] = {
case 'a' => 'A'
case x => x
}
val a = Array('a', 'b', 'c')
val b = a.collect(fun)
println(b.mkString(",")) // A,b,c
方法名:collectFirst
使用规范: def collectFirst[B](pf: PartialFunction[T, B]): Option[B]
具体描述:在序列中查找第一个符合偏函数定义的元素,并执行偏函数计算
val fun: PartialFunction[Any, Int] = {
case x: Int => x + 1
}
val a = Array('a',1,3, "A")
val b = a.collectFirst(fun)
println(b) // Some(2)
方法名:combinations
使用规范:
def combinations(n : scala.Int) : scala.collection.Iterator[Repr] = { /* compiled code */ }
具体描述:combinations 表示组合,这个排列组合会选出所有包含字符不一样的组合,但不考虑顺序,对于 “abc”、“cba”,视为相同组合,参数 n 表示序列长度,就是几个字符为一组
val a = Array("a", "b", "c")
val b = a.combinations(2)
b.foreach(x => println(x.mkString(",")))
/*
* a,b
* a,c
* b,c
*/
方法名:companion
使用规范:
override def companion : scala.collection.generic.GenericCompanion[scala.collection.mutable.IndexedSeq] = { /* compiled code */ }
具体描述:暂时不知道怎么使用
方法名:compose
使用规范:
def compose[A](g : scala.Function1[A, T1]) : scala.Function1[A, R] = { /* compiled code */ }
具体描述:表示方法的连续调用,与 andThen 相反,不同的是需满足第二个函数的返回值类型是第一个函数的输入值类型
def f(a:Int) = {
println("2*"+a)
2*a
}
def g(b:Int) = {
println("3*"+b)
3*b
}
def result1 = f _ andThen g _
println(result1(1))
/*
2*1
2*3
6
*/
def result2 = f _ compose g _
println(result2(1))
/*
3*1
3*2
6
*/
方法名:contains
使用规范:
override def contains[A1 >: A](elem : A1) : scala.Boolean = { /* compiled code */ }
具体描述:判断序列中是否包含指定对象
val a = List(1, 2, 3, 4)
println(a.contains(1)) // true
方法名:containsSlice
使用规范:
def containsSlice[B](that : scala.collection.GenSeq[B]) : scala.Boolean = { /* compiled code */ }
具体描述:判断当前序列中是否包含另一个序列
val a = List(1,2,3,4)
val b = List(2,3)
val c = List(2,3,5)
println(a.containsSlice(b)) // true
println(a.containsSlice(c)) //false
方法名:copyToArray
使用规范:
def copyToArray[B >: A](xs : scala.Array[B], start : scala.Int) : scala.Unit = { /* compiled code */ }
def copyToArray[B >: A](xs : scala.Array[B]) : scala.Unit = { /* compiled code */ }
具体描述:将当前数组元素复制到另一个数组中(可以指定起始下标引入,选择引入几个元素)
val a = Array(1, 2, 3)
val b: Array[Int] = new Array(10)
a.copyToArray(b)
println(b.mkString(",")) // 1,2,3,0,0,0,0,0,0,0
a.copyToArray(b, 4, 2) //从5位引入2个数字
println(b.mkString(",")) // 1,2,3,0,1,2,0,0,0,0
方法名:copyToBuffer
使用规范:
def copyToBuffer[B >: A](dest : scala.collection.mutable.Buffer[B]) : scala.Unit = { /* compiled code */ }
具体描述:将数组中的元素复制到 Buffer 中
(这里的Buffer就相当于java中ArrayList)
//需先引入ArrayBuffer jar包
import scala.collection.mutable.ArrayBuffer
val a = Array(1, 2, 3, 4)
val b: ArrayBuffer[Int] = ArrayBuffer()
a.copyToBuffer(b)
println(b.mkString(",")) // 1,2,3,4
方法名:corresponds
使用规范:
def corresponds[B](that : scala.collection.GenSeq[B])(p : scala.Function2[A, B, scala.Boolean]) : scala.Boolean = { /* compiled code */ }
具体描述: 判断两个序列的长度以及对应位置元素是否符合某个条件,满足返回true,不满足返回false
val a = Array(1, 2, 3, 4)
val b = Array(5, 6, 7, 8)
val c = Array(2, 1, 4, 5)
println(a.corresponds(b)(_ < _)) // true
println(a.corresponds(c)(_ < _)) // 第二个元素不满足,false
方法名:count
使用规范:
def count(p : scala.Function1[A, scala.Boolean]) : scala.Int = { /* compiled code */ }
具体描述:与java的count不同的是,这里的count是对满足条件的元素计数
val a = Array(1, 2, 3, 4)
println(a.count(x => x > 2)) // 2
方法名:deep
使用规范:
def deep : scala.collection.IndexedSeq[scala.Any] = { /* compiled code */ }
具体描述:暂时不知道如何使用
方法名:diff
使用规范:
def diff[B >: A](that : scala.collection.GenSeq[B]) : Repr = { /* compiled code */ }
具体描述:计算当前数组与另一个数组的差集,即返回另外一个数组中没有的元素(即减去与另一个集合的交集)
val a = Array(1, 2, 3, 4)
val b = Array(3, 4, 5, 6)
val c = a.diff(b)
println(c.mkString(",")) // 1,2
方法名:distinct
使用规范:def distinct : Repr = { /* compiled code */ }
具体描述:去掉集合中重复的元素,保留一个
val a = Array(1, 2, 2, 3, 3,4, 4)
val b = a.distinct
println(b.mkString(",")) // 1,2,3,4
方法名:drop
使用规范:override def drop(n : scala.Int) : Repr = { /* compiled code */ }
具体描述:删除集合中前n个元素
val a = Array(1, 2, 3, 4)
val b = a.drop(2) //删除前两个元素
println(b.mkString(",")) // 3,4
方法名:dropRight
使用规范: override def dropRight(n : scala.Int) : Repr = { /* compiled code */ }
具体描述: 删除集合中尾部的 n 个元素
val a = Array(1, 2, 3, 4)
val b = a.dropRight(2)
println(b.mkString(",")) // 1,2
方法名:dropWhile
使用规范:
override def dropWhile(p : scala.Function1[A, scala.Boolean]) : Repr = { /* compiled code */ }
具体描述: 删除数组中符合条件的元素,从第一个元素起,直到碰到第一个不满足条件的元素结束(即使后面还有符合条件的元素),若第一个条件就不满足就返回整个数组
val a = Array(1,2,3,4,3,2,1)
val b = a.dropWhile(x => x < 3) //删除小于3的元素
println(b.mkString(",")) // 3,4,3,2,1 遇到4截止
val b = a.dropWhile(x => x > 2)
println(b.mkString(",")) // 1,2,3,4,3,2,1 第一个条件就不满足,返回整个数组
方法名:elemManifest
使用规范:
具体描述:暂时不知道使用方法
方法名:elemTag
使用规范:
具体描述:暂时不知道使用方法
方法名:endsWith
使用规范:
override def endsWith[B](that : scala.collection.GenSeq[B]) : scala.Boolean = { /* compiled code */ }
具体描述:判断当前序列是否以某个序列结尾
val a = Array(1, 2, 3, 4)
val b = Array(3, 4)
println(a.endsWith(b)) // true
方法名:exists
使用规范:
override def exists(p : scala.Function1[A, scala.Boolean]) : scala.Boolean = { /* compiled code */ }
具体描述:判断当前数组是否包含符合条件的元素
val a = Array(1, 2, 3, 4)
println(a.exists(x => x == 3)) // true
println(a.exists(x => x == 5)) // false
方法名:filter
使用规范:
def filter(p : scala.Function1[A, scala.Boolean]) : Repr = { /* compiled code */ }
具体描述:筛选数组中符合条件的元素
val a = Array(1, 2, 3, 4)
val b = a.filter(x => x > 2)
println(b.mkString(",")) // 3,4
方法名:filterNot
使用规范:
def filterNot(p : scala.Function1[A, scala.Boolean]) : Repr = { /* compiled code */ }
具体描述:与filter相反,将不满足条件的元素筛选出来
val a = Array(1, 2, 3, 4,0)
val b = a.filterNot(x => x > 2)
println(b.mkString(",")) // 1,2,0
方法名:find
使用规范:
override def find(p : scala.Function1[A, scala.Boolean]) : scala.Option[A] = { /* compiled code */ }
具体描述:查找第一个符合条件的元素
val a = Array(1, 2, 3, 4)
val b = a.find(x => x > 2)
println(b) // Some(3)
方法名:flatMap
使用规范:
def flatMap[B, That](f : scala.Function1[A, scala.collection.GenTraversableOnce[B]])(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:flatMap = map + flatten 即先对集合中的每个元素进行map,再对map后的每个元素(map后的每个元素必须还是集合)中的每个元素进行flatten
val a = Array(1, 2, 3, 4)
val b = a.flatMap(x => 1 to x)
println(b.mkString(",")) //展开返回一个数组 1,1,2,1,2,3,1,2,3,4
/*
1
1,2
1,2,3
1,2,3,4
*/
//与map的区别
val c = a.map(x => 1 to x)
println(c.mkString(",")) //分别返回4个数组 Range(1),Range(1, 2),Range(1, 2, 3),Range(1, 2, 3, 4)
//展开一个Array[Array]类型的数组
val arr = Array(Array(1, 2, 3), Array(4, 5, 6), Array(7, 8, 9))
val ints = arr.flatMap(x => x)
println(ints.mkString(",")) //展开arr中每一个数组元素 1,2,3,4,5,6,7,8,9
方法名:flatten
使用规范:
def flatten[U](implicit asTrav: (T) ⇒ collection.Traversable[U], m: ClassTag[U]): Array[U]
具体描述:扁平化,将二维数组的所有元素组合在一起,形成一个一维数组返回
val a = Array(Array(1, 2, 3), Array(4, 5, 6))
val b = a.flatten
println(b.mkString(",")) // 1,2,3,4,5,6
方法名:fold
使用规范:
def fold[A1 >: A](z: A1)(op: (A1, A1) ⇒ A1): A1ClassTag[U]): Array[U]
具体描述:对序列中的每个元素进行二元运算,和 aggregate 有类似的语义,但执行过程有所不同
def seqno(m: Int, n: Int): Int = {
val s = "seq_exp = %d + %d"
println(s.format(m, n))
m + n
}
def main(args: Array[String]) {
val a = Array(1, 2, 3, 4)
val b = a.fold(5)(seqno) // 不分区
println("b = " + b)
/*
* seq_exp = 5 + 1
* seq_exp = 6 + 2
* seq_exp = 8 + 3
* seq_exp = 11 + 4
* b = 15
*/
val c = a.par.fold(5)(seqno) // 分区
println("c = " + c)
/*
* seq_exp = 5 + 3
* seq_exp = 5 + 2
* seq_exp = 5 + 4
* seq_exp = 5 + 1
* com_exp = 6 + 7
* com_exp = 8 + 9
* com_exp = 13 + 17
* c = 30
*/
}
方法名:foldLeft
使用规范: def foldLeft[B](z: B)(op: (B, T) ⇒ B): BClassTag[U]): Array[U]
具体描述:从左到右计算,简写方式:def /:[B](z: B)(op: (B, T) ⇒ B): B
def seqno(m: Int, n: Int): Int = {
val s = "seq_exp = %d + %d"
println(s.format(m, n))
m + n
}
def main(args: Array[String]): Unit = {
val a = Array(1, 2, 3, 4)
val b = a.foldLeft(5)(seqno) // 简写: (5 /: a)(_ + _)
println("b = " + b)
/**
* seq_exp = 5 + 1
* seq_exp = 6 + 2
* seq_exp = 8 + 3
* seq_exp = 11 + 4
* b = 15
*/
}
方法名:foldRight
使用规范: foldRight[B](z: B)(op: (B, T) ⇒ B): B
具体描述:从右到左计算,简写方式:def :[B](z: B)(op: (T, B) ⇒ B): B
def seqno(m: Int, n: Int): Int = {
val s = "seq_exp = %d + %d"
println(s.format(m, n))
m + n
}
def main(args: Array[String]): Unit = {
val a = Array(1, 2, 3, 4)
val b = a.foldRight(5)(seqno) // 简写: (a :\ 5)(_ + _)
println("b = " + b)
/**
* seq_exp = 4 + 5
* seq_exp = 3 + 9
* seq_exp = 2 + 12
* seq_exp = 1 + 14
* b = 15
*/
}
方法名:forall
使用规范:def forall(p: (T) ⇒ Boolean): Boolean
具体描述:检测序列中的元素是否都满足条件 p,如果序列为空,则返回 true
val a = Array(1, 2, 3, 4)
println(a.forall(x => x > 0)) // true
println(a.forall(x => x > 2)) // false
方法名:foreach
使用规范: def foreach(f: (A) ⇒ Unit): Unit
具体描述:遍历序列中的元素,进行 f 操作
val a = Array(1, 2, 3, 4)
a.foreach(x => println(x * 10))
/**
* 10
* 20
* 30
* 40
*/
方法名:genericBuilder
使用规范:
def genericBuilder[B] : scala.collection.mutable.Builder[B, CC[B]] = { /* compiled code */ }
具体描述:暂时不知道具体用法
方法名:groupBy
使用规范:
def groupBy[K](f : scala.Function1[A, K]) : scala.collection.immutable.Map[K, Repr] = { /* compiled code */ }
具体描述: 按条件分组,条件由 f 匹配,返回值是 Map 类型,每个 key 对应一个数组
var a = Array(1,2,3,4)
a.groupBy(x => x>2)
Map[Boolean,Array[Int]] = Map(false -> Array(1, 2), true -> Array(3, 4, 5))
//生成一个新的map集合
方法名:grouped
使用规范:
def grouped(size : scala.Int) : scala.collection.Iterator[Repr] = { /* compiled code */ }
具体描述: 按指定数量分组,每组有 size 个元素
val a = Array(1,2,3,4,5)
a.grouped(3)
Iterator[Array[Int]] = non-empty iterator
//生成的结果是一个迭代器
a.grouped(3).foreach(x => println(x.toList))
//将迭代器转成List输出
List(1, 2, 3)
List(4, 5)
方法名:hasDefiniteSize
使用规范:def hasDefiniteSize : scala.Boolean = { /* compiled code */ }
具体描述:检测序列是否存在有限的长度,对应 Stream 类型的流数据则返回 false
val a = Array(1,2,3,4,5)
a.hasDefiniteSize
Boolean = true
a.grouped(3).hasDefiniteSize//例如迭代器
Boolean = false
方法名:head
使用规范: override def head : A = { /* compiled code */ }
具体描述:返回序列的第一个元素,如果序列为空会报错
val a = Array(1, 2, 3, 4)
a.head
Int = 1
方法名:headOption
使用规范: def headOption : scala.Option[A] = { /* compiled code */ }
具体描述: 返回序列的第一个元素的 Option 类型对象,如果序列为空,则返回 None
val a = Array(1, 2, 3, 4)
a.headOption
//为了防止head一个空序列时报错影响程序,一般采用headOption比较好
Some(1)
//
val b:Array[Int] =Array()
b.head
//java.util.NoSuchElementException: next on empty iterator
b.headOption
Option[Int] = None
方法名:indexOf
使用规范: def indexOf[B >: A](elem : B) : scala.Int = { /* compiled code */ }
具体描述: 返回元素 elem 在序列中第一次出现的索引,也可以指定从索引 from 开始查找
val a = Array(1,2,3,4,2,3)
a.indexOf(3)
Int = 2
a.indexOf(3,4)
Int = 5
方法名:indexOfSlice
使用规范:
def indexOfSlice[B >: A](that : scala.collection.GenSeq[B]) : scala.Int = { /* compiled code */ }
def indexOfSlice[B >: A](that : scala.collection.GenSeq[B], from : scala.Int) : scala.Int = { /* compiled code */ }
具体描述:检测当前序列中是否包含序列 that,并返回第一次出现该序列的索引(可以指定从索引 from 开始查找,并返回第一次出现该序列的索引)
val a = Array(1,2,3,4,5,2,3,6,7,2,3)
val b = Array(2, 3)
println(a.indexOfSlice(b)) // 1
println(a.indexOfSlice(b,3)) // 5
方法名:indexWhere
使用规范:
def indexWhere(p : scala.Function1[A, scala.Boolean]) : scala.Int = { /* compiled code */ }
具体描述: 返回当前序列中第一个满足条件 p 的元素的索引,可以指定从索引 from 开始查找
val a = Array(1, 2, 3, 4)
println(a.indexWhere(x => x > 2)) // 2
println(a.indexWhere(x => x > 2, 3)) // 3
方法名:indices
使用规范:
def indices : scala.collection.immutable.Range = { /* compiled code */ }
具体描述:返回当前序列索引集合
val a = Array(1, 2, 3, 4)
val b = a.indices
println(b.mkString(",")) // 0,1,2,3
方法名:init
使用规范: override def init : Repr = { /* compiled code */ }
具体描述:返回当前序列中不包含最后一个元素的序列(即去掉序列的尾元素)
val a = Array(1, 2, 3, 4)
val b = a.init
println(b.mkString(",")) // 1,2,3
方法名:inits
使用规范: def inits : scala.collection.Iterator[Repr] = { /* compiled code */ }
具体描述:对集合中的元素进行 init 迭代操作,该操作的返回值中, 第一个值是当前序列的副本,最后一个值为空,每一步都进行 init 操作,上一步的结果作为下一步的操作对象
val a = Array(1, 2, 3, 4)
val b = a.inits.toList
for (i <- 0 until b.length) {
val s = "第 %d 个值: %s"
println(s.format(i + 1, b(i).mkString(",")))
}
/*
* 第 1 个值: 1,2,3,4
* 第 2 个值: 1,2,3
* 第 3 个值: 1,2
* 第 4 个值: 1
* 第 5 个值:
*/
方法名:intersect
使用规范:
def intersect[B >: A](that : scala.collection.GenSeq[B]) : Repr = { /* compiled code */ }
具体描述:取两个集合的交集,可以和diff结合来记忆
val a = Array(1, 2, 3, 4)
val b = Array(3, 4, 5, 6)
val c = a.intersect(b)
println(c.mkString(",")) // 3,4
方法名:isDefinedAt
使用规范:
def isDefinedAt(idx : scala.Int) : scala.Boolean = { /* compiled code */ }
具体描述:判断序列中是否存在指定索引
val a = Array(1, 2, 3, 4)
println(a.isDefinedAt(1)) // true
println(a.isDefinedAt(5)) // false
方法名:isEmpty
使用规范: override def isEmpty : scala.Boolean = { /* compiled code */ }
具体描述:判断序列是否为空
val a = Array(1, 2, 3, 4)
val b = new Array[Int](0)
println(a.isEmpty) // false
println(b.isEmpty) // true
方法名:isTraversableAgain
使用规范:final def isTraversableAgain : scala.Boolean = { /* compiled code */ }
具体描述:判断序列是否可以反复遍历,该方法是 GenTraversableOnce 中的方法,对于 Traversables 一般返回 true,对于 Iterators 返回 false,除非被复写
val a = Array(1, 2, 3, 4)
val b = a.iterator
println(a.isTraversableAgain) // true
println(b.isTraversableAgain) // false
方法名:iterator
使用规范:
override def iterator : scala.collection.Iterator[A] = { /* compiled code */ }
具体描述:生成当前序列的迭代器
val a = Array(1, 2, 3, 4)
val b = a.iterator
println(b.mkString(",")) // 1,2,3,4
方法名:last
使用规范:override def last : A = { /* compiled code */ }
具体描述:返回序列的最后一个元素,如果序列为空,将引发错误
val a = Array(1, 2, 3, 4)
println(a.last) // 4
方法名:lastIndexOf
使用规范:
def indexOfSlice[B >: A](that : scala.collection.GenSeq[B]) : scala.Int = { /* compiled code */ }
def indexOfSlice[B >: A](that : scala.collection.GenSeq[B], from : scala.Int) : scala.Int = { /* compiled code */ }
具体描述: 返回元素 elem 在序列中最后一次出现的索引 (后面加一个参数是从指定的这个参数索引开始,向前遍历,找到第一个符合的元素的索引)
val a = Array(1, 3, 2, 3, 4)
println(a.lastIndexOf(3)) // 3
println(a.lastIndexOf(3, 2)) // 1
方法名:lastIndexOfSlice
使用规范:
def lastIndexOfSlice[B >: A](that : scala.collection.GenSeq[B]) : scala.Int = { /* compiled code */ }
def lastIndexOfSlice[B >: A](that : scala.collection.GenSeq[B], end : scala.Int) : scala.Int = { /* compiled code */ }
具体描述:检测当前序列中是否包含序列 that,并返回最后一次出现该序列的索引 (可以指定索引,往前查找)
val a = Array(1, 2, 3, 2, 3, 4)
val b = Array(2, 3)
println(a.lastIndexOfSlice(b)) // 3
println(a.lastIndexOfSlice(b, 2)) // 1
方法名:lastIndexWhere
使用规范:
def lastIndexWhere(p : scala.Function1[A, scala.Boolean]) : scala.Int = { /* compiled code */ }
def lastIndexWhere(p : scala.Function1[A, scala.Boolean], end : scala.Int) : scala.Int
具体描述:返回当前序列中最后一个满足条件 p 的元素的索引 (可以指定索引,往前查找)
val a = Array(1, 2, 3, 4)
println(a.lastIndexWhere(x => x > 2)) // 3
println(a.lastIndexWhere(x => x > 2, 2)) // 2
方法名:lastOption
使用规范: def lastOption : scala.Option[A] = { /* compiled code */ }
具体描述:返回序列的最后一个元素的 Option 类型对象,如果序列为空,则返回 None
val a = Array(1, 2, 3, 4)
println(a.lastOption) // Some(4)
方法名:length
使用规范: def length : scala.Int = { /* compiled code */ }
具体描述:返回序列元素个数
val a =Array(1,2,3,4)
a.length
Int = 4
方法名:lengthCompare
使用规范:
override def lengthCompare(len : scala.Int) : scala.Int = { /* compiled code */ }
具体描述:比较序列的长度和参数 len,返回序列的长度 - len
val a = Array(1, 2, 3, 4)
//就是拿前面的数组的长度减去后面给的参数数,得到的值就是返回值,分为大于零,小于零,等于零
println(a.lengthCompare(3)) // 1
println(a.lengthCompare(4)) // 0
println(a.lengthCompare(5)) // -1
方法名:lift
使用规范: def lift: Int => Option[Int]
具体描述:给序列的索引,返回该索引处的Option类的值
val a = Array(1, 2, 3, 4)
a.lift(1)
Option[Int] = Some(2)
方法名:map
使用规范:
def map[B, That](f : scala.Function1[A, B])(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:对序列中的元素进行 f 操作,返回生成的新序列
val a = Array(1, 2, 3, 4)
val b = a.map(x => x * 10)
println(b.mkString(",")) // 10,20,30,40
方法名:max
使用规范:max[B >: A](implicit cmp : scala.Ordering[B]) : A = { /* compiled code */ }
具体描述:返回序列中最大的元素
val a = Array(1, 2, 3, 4)
println(a.max) // 4
方法名:maxBy
使用规范:
def maxBy[B](f : scala.Function1[A, B])(implicit cmp : scala.Ordering[B]) : A = { /* compiled code */ }
具体描述:返回序列中符合条件的第一个元素
val a = Array(1, 2, 3, 4)
println(a.maxBy(x => x > 2)) // 3
方法名:min
使用规范:
def min[B >: A](implicit cmp : scala.Ordering[B]) : A = { /* compiled code */ }
具体描述: 返回序列中最小的元素
val a = Array(1, 2, 3, 4)
println(a.min) // 1
方法名:minBy
使用规范:
def minBy[B](f : scala.Function1[A, B])(implicit cmp : scala.Ordering[B]) : A = { /* compiled code */ }
具体描述: 返回序列中不符合条件的第一个元素(找不到满足条件的元素返回一个元素)
val a = Array(1, 2, 3, 4)
println(a.minBy(x => x < 2)) // 2
println(a.minBy(x => x > 9)) // 1 默认第一个元素为最小,当遍历完发现没有大于5的元素,就返回第一个元素
方法名:mkString
使用规范:
def mkString(start : scala.Predef.String, sep : scala.Predef.String, end : scala.Predef.String) : scala.Predef.String = { /* compiled code */ }
具体描述:将序列中所有元素拼接成一个字符串,可以设置参数:mkString(start, sep, end) 将序列中所有元素拼接成一个字符串,以 start 开头,以 sep 作为元素间的分隔符,以 end 结尾)
val a = Array(1, 2, 3, 4)
println(a.mkString) // 1234
println(a.mkString("(", ",", ")")) // (1,2,3,4)
方法名:nonEmpty
使用规范: def nonEmpty : scala.Boolean = { /* compiled code */ }
具体描述:判断序列是否不为空
val a = Array(1, 2, 3, 4)
val b = new Array[Int](0)
println(a.nonEmpty) // true
println(b.nonEmpty) // false
方法名:orElse
使用规范:
final def orElse[B >: A](alternative : => scala.Option[B]) : scala.Option[B] = { /* compiled code */ }
具体描述:与 getOrElse(value) 类似,只不过 orElse 参数为Some, 返回Option里的Some或从参数传递进去的Some
val a = Some("Hello Option")
a.orElse(null) //返回option的Some
val b = None
b.orElse(Some("New Value")) //返回参数的Some
方法名:padTo
使用规范:
def padTo[B >: A, That](len : scala.Int, elem : B)(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:填充序列,如果当前序列长度小于 len,那么新产生的序列长度是 len,多出的几个位值填充 elem,如果当前序列长度大于等于 len ,则返回当前序列
val a = Array(1, 2, 3, 4)
val b = a.padTo(7, 8) //填充一个长度为 7 的序列,不足位补 8
println(b.mkString(",")) // 1,2,3,4,8,8,8
val c = a.padTo(3, 5) //填充序列长度3,不足位补5,长度够的情况下不做操作
println(c.mkString(",")) //1,2,3,4
方法名:par
使用规范:
override def par : scala.collection.parallel.mutable.ParArray[T] = { /* compiled code */ }
具体描述:开启分区,并行执行任务
val a = Array(1, 2, 3, 4, 5)
a.foreach(print) //12345
println()
a.par.foreach(print) //32145
//获取参与执行任务的线程
(0 to 10000).collect{case _ => Thread.currentThread.getName}.distinct
//获取并行参与执行任务的线程
(0 to 10000).par.collect{case _ => Thread.currentThread.getName}.distinct
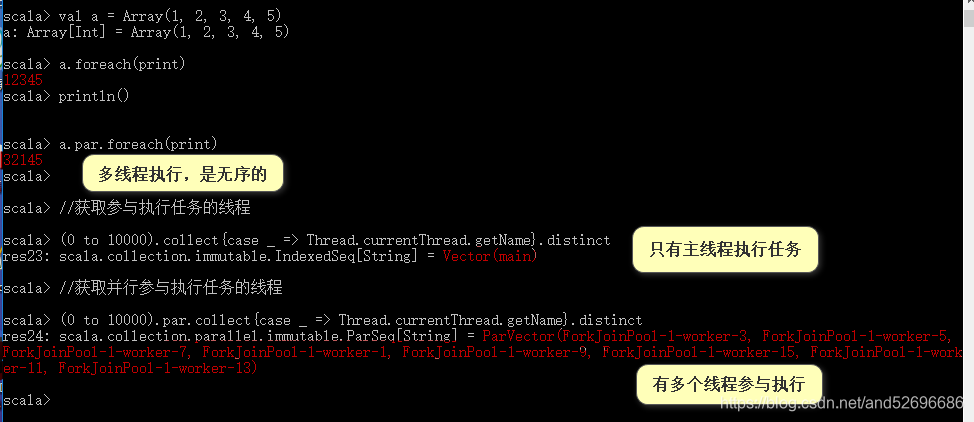
方法名:partition
使用规范:
def partition(p : scala.Function1[A, scala.Boolean]) : scala.Tuple2[Repr, Repr] = { /* compiled code */ }
具体描述:按条件将序列拆分成两个数组,满足条件的放到第一个数组,其余的放到第二个数组,返回的是包含这两个数组的元组
val a = Array(1, 2, 3, 4)
val b: (Array[Int], Array[Int]) = a.partition(x => x % 2 == 0)
println("偶数: " + b._1.mkString(",")) // 偶数: 2,4
println("奇数: " + b._2.mkString(",")) // 奇数: 1,3
方法名:patch
使用规范:
def patch[B >: A, That](from : scala.Int, patch : scala.collection.GenSeq[B], replaced : scala.Int)(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:批量替换,从原序列的 from 处开始,后面的 replaced 个元素,将被替换成序列 that
val a = Array(1, 2, 3, 4)
val b = Array(7, 8, 9)
val c = a.patch(1, b, 2)
println(c.mkString(",")) // 1,7,8,9,4
方法名:permutations
使用规范:def permutations : scala.collection.Iterator[Repr] = { /* compiled code */ }
具体描述:排列组合会选出所有排列顺序不同的字符组合,与 combinations 不同的是,对于相同的字符组合,比如 “abc”、“cba”,视为不同的组合
val a = Array("a", "b", "c")
val b = a.permutations.toList
b.foreach( x => println(x.mkString(",")))
/*
* a,b,c
* a,c,b
* b,a,c
* b,c,a
* c,a,b
* c,b,a
*/
方法名:prefixLength
使用规范:
def prefixLength(p : scala.Function1[A, scala.Boolean]) : scala.Int = { /* compiled code */ }
具体描述:给定一个条件 p,返回满足条件的元素个数
val a = Array(1, 2, 3, 4)
println(a.prefixLength(x => x < 3)) // 2
方法名:product
使用规范:
def product[B >: A](implicit num : scala.Numeric[B]) : B = { /* compiled code */ }
具体描述:返回所有元素乘积的值
val a = Array(1, 2, 3, 4)
println(a.product) // 1*2*3*4=24
方法名:reduce
使用规范:
def reduce[A1 >: A](op : scala.Function2[A1, A1, A1]) : A1 = { /* compiled code */ }
具体描述:同 fold,将序列中的元素按给定的操作依次进行。但不需要初始值
def seqno(m: Int, n: Int): Int = {
val s = "seq_exp = %d + %d"
println(s.format(m, n))
m + n
}
def main(args: Array[String]) {
val a = Array(1, 2, 3, 4)
val b = a.reduce(seqno)
println("b = " + b)
}
/*
* seq_exp = 1 + 2
* seq_exp = 3 + 3
* seq_exp = 6 + 4
* b = 10
*/
方法名:reduceLeft
使用规范:
override def reduceLeft[B >: A](op : scala.Function2[B, A, B]) : B = { /* compiled code */ }
具体描述:同 fold、foldLeft、reduce,从左向右计算,和reduce一样,也不需要初始值
def seqno(m: Int, n: Int): Int = {
val s = "seq_exp = %d + %d"
println(s.format(m, n))
m + n
}
def main(args: Array[String]) {
val a = Array(1, 2, 3, 4)
val b = a.reduce(seqno)
println("b = " + b)
}
/*
* seq_exp = 1 + 2
* seq_exp = 3 + 3
* seq_exp = 6 + 4
* b = 10
*/
方法名:reduceLeftOption
使用规范:
def reduceLeftOption[B >: A](op : scala.Function2[B, A, B]) : scala.Option[B] = { /* compiled code */ }
具体描述:同 reduceLeft,只是返回的是Option,不会因为空序列而报错
def seqno(m: Int, n: Int): Int = {
val s = "seq_exp = %d + %d"
println(s.format(m, n))
m + n
}
def main(args: Array[String]) {
val a = Array(1, 2, 3, 4)
val b = a.reduceLeftOption(seqno)
println("b = " + b)
}
/*
* seq_exp = 1 + 2
* seq_exp = 3 + 3
* seq_exp = 6 + 4
* b = Some(10)
*/
方法名:reduceOption
使用规范:
def reduceOption[A1 >: A](op : scala.Function2[A1, A1, A1]) : scala.Option[A1] = { /* compiled code */ }
具体描述:同 reduceLeftOption
def seqno(m: Int, n: Int): Int = {
val s = "seq_exp = %d + %d"
println(s.format(m, n))
m + n
}
def main(args: Array[String]) {
val a = Array(1, 2, 3, 4)
val b = a.reduceOption(seqno)
println("b = " + b)
}
/*
* seq_exp = 1 + 2
* seq_exp = 3 + 3
* seq_exp = 6 + 4
* b = Some(10)
*/
方法名:reduceRight
使用规范:
override def reduceRight[B >: A](op : scala.Function2[A, B, B]) : B = { /* compiled code */ }
具体描述:同 foldRight,从右向左计算,不需要初始值
def seqno(m: Int, n: Int): Int = {
val s = "seq_exp = %d + %d"
println(s.format(m, n))
m + n
}
def main(args: Array[String]) {
val a = Array(1, 2, 3, 4)
val b = a.reduceRight(seqno)
println("b = " + b)
}
/*
* seq_exp = 3 + 4
* seq_exp = 2 + 7
* seq_exp = 1 + 9
* b = 10
*/
方法名:reduceRightOption
使用规范:
def reduceRightOption[B >: A](op : scala.Function2[A, B, B]) : scala.Option[B] = { /* compiled code */ }
具体描述:同 reduceRight
def seqno(m: Int, n: Int): Int = {
val s = "seq_exp = %d + %d"
println(s.format(m, n))
m + n
}
def main(args: Array[String]) {
val a = Array(1, 2, 3, 4)
val b = a.reduceRightOption(seqno)
println("b = " + b)
}
/*
* seq_exp = 3 + 4
* seq_exp = 2 + 7
* seq_exp = 1 + 9
* b = 10
*/
方法名:repr
使用规范: def repr : Repr = { /* compiled code */ }
具体描述: repr() 函数将对象转化为供解释器读取的形式
val a = Map(1 -> "a",2 -> "b")
println(a.repr) //等于 println(a) Map(1 -> a, 2 -> b)
val b = Array("java","scala")
println(b.repr) // 等于 println(b) 打印地址[Ljava.lang.String;@71423665
方法名:reverse
使用规范: override def reverse : Repr = { /* compiled code */ }
具体描述:反转序列
val a = Array(1, 2, 3, 4)
val b = a.reverse
println(b.mkString(",")) // 4,3,2,1
方法名:reverseIterator
使用规范:
override def reverseIterator : scala.collection.Iterator[A] = { /* compiled code */ }
具体描述:生成反向迭代器
val a = Array(1, 2, 3, 4)
val b = a.reverseIterator
b.foreach(x => print(x + " ")) // 4 3 2 1
方法名:reverseMap
使用规范:
def reverseMap[B, That](f : scala.Function1[A, B])(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:同 map,方向相反
val a = Array(1, 2, 3, 4)
val b = a.reverseMap(x => x * 10)
println(b.mkString(",")) // 40,30,20,10
方法名:runWith
使用规范:
def runWith[U](action : scala.Function1[B, U]) : scala.Function1[A, scala.Boolean] = { /* compiled code */ }
具体描述:执行偏函数,当参数不在定义域内时,返回false,否则返回true,并执行action
val pf: PartialFunction[Int, Int] = {
case m: Int if m % 2 == 1=> m * 2
}
pf.runWith(println)(3)
// return: Boolean = true
pf.runWith(println)(2)
// return: Boolean = false
方法名:sameElements
使用规范:
override def sameElements[B >: A](that : scala.collection.GenIterable[B]) : scala.Boolean = { /* compiled code */ }
具体描述:判断两个序列是否顺序和对应位置上的元素都一样
val a = Array(1, 2, 3, 4)
val b = Array(1, 2, 3, 4)
println(a.sameElements(b)) // true
val c = Array(1, 3, 2, 4)
println(a.sameElements(c)) // false
val d = Array(1, 2, 3, 4, 5)
println(a.sameElements(d)) // false
方法名:scan
使用规范:
def scan[B >: A, That](z : B)(op : scala.Function2[B, B, B])(implicit cbf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:同 fold,scan 会把每一步的计算结果放到一个新的集合中返回,而 fold 返回的是最后的结果
val a = Array(1, 2, 3, 4)
val b = a.scan(5)(_ + _)
println(b.mkString(",")) // 5,6,8,11,15
方法名:scanLeft
使用规范:
def scanLeft[B, That](z : B)(op : scala.Function2[B, A, B])(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:同 scan,从左向右计算,每一步的计算结果放到一个新的集合中返回
val a = Array(1, 2, 3, 4)
val b = a.scanLeft(5)(_ + _)
println(b.mkString(",")) // 5,6,8,11,15
方法名:scanRight
使用规范:
def scanRight[B, That](z : B)(op : scala.Function2[A, B, B])(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:同 foldRight,从右向左计算,每一步的计算结果放到(从右向左放)一个新的集合中返回
val a = Array(1, 2, 3, 4)
val b = a.scanRight(5)(_ + _)
println(b.mkString(",")) // 15,14,12,9,5
方法名:segmentLength
使用规范:
override def segmentLength(p : scala.Function1[A, scala.Boolean], from : scala.Int) : scala.Int = { /* compiled code */ }
具体描述:从序列的 from 开始向后查找,返回满足条件 p 的连续元素的长度,只返回一次
val a = Array(1, 2, 3, 1, 1, 1, 4, 1, 2, 3)
println(a.segmentLength(x => x < 3, 3)) // 3
方法名:seq
使用规范:
def seq : scala.collection.mutable.IndexedSeq[T] = { /* compiled code */ }
具体描述:产生一个引用当前序列的 sequential 视图,就是一个特殊的集合
val a = Array(1, 2, 3, 4)
val b = a.seq
println(b.mkString(",")) // 1,2,3,4
方法名:size
使用规范: override def size : scala.Int = { /* compiled code */ }
具体描述:返回序列元素个数,同 length,几乎所有的序列都有size,这点和java不同
val a = Array(1, 2, 3, 4)
println(a.size) // 4
方法名:slice
使用规范:
override def slice(from : scala.Int, until : scala.Int) : Repr = { /* compiled code */ }
具体描述:返回当前序列中从 from 到 until 之间的序列,不包括 until 处的元素(左闭右开)
val a = Array(1, 2, 3, 4)
val b = a.slice(1, 3)
println(b.mkString(",")) // 2,3
方法名:sliding
使用规范:
def sliding(size : scala.Int) : scala.collection.Iterator[Repr] = { /* compiled code */ }
def sliding(size : scala.Int, step : scala.Int) : scala.collection.Iterator[Repr] = { /* compiled code */ }
具体描述:滑动,从第一个元素开始,每个元素和它后面的 size - 1 个元素组成一个数组,最终组成一个新的集合返回,当剩余元素个数不够 size 时,则结束 。默认步长为1, (sliding(size, step) 可以设置步长 step,每一组元素组合完后,下一组从上一组起始元素位置 + step 后的位置处开始)
val a = Array(1, 2, 3, 4, 5)
val b = a.sliding(3).toList //每次滑3步,步长为1
for (i <- 0 to b.length - 1) {
val s = "第 %d 组: %s"
println(s.format(i + 1, b(i).mkString(",")))
}
/*
* 第 1 组: 1,2,3
* 第 2 组: 2,3,4
* 第 3 组: 3,4,5
*/
val c = a.sliding(3, 2).toList //每次滑3步,步长为2
for (i <- 0 to c.length - 1) {
val s = "第 %d 组: %s"
println(s.format(i + 1, c(i).mkString(",")))
}
/*
* 第 1 组: 1,2,3
* 第 2 组: 3,4,5
*/
方法名:sortBy
使用规范:
def sortBy[B](f : scala.Function1[A, B])(implicit ord : scala.math.Ordering[B]) : Repr = { /* compiled code */ }
具体描述:按指定的排序规则对序列排序
val a = Array(3, 2, 1, 4)
val b = a.sortBy(x => x) // 按 x 从小到大,即对原序列升序排列
println("升序: " + b.mkString(",")) // 1,2,3,4
val c = a.sortBy(x => 0 - x) // 按 -x 从小到大,即对原序列降序排列
println("降序: " + c.mkString(",")) // 4,3,2,1
val d = Array('a','d','e','b')
val b = d.sortBy(x => x) // 按 x 从小到大,即对原序列升序排列
println("升序: " + b.mkString(",")) // a,b,d,e
方法名:sortWith
使用规范:
def sortWith(lt: (A, A) => Boolean): Repr = sorted(Ordering fromLessThan lt)
具体描述:sortWith需要传入一个比较函数用来比较
def func(m:Int,n:Int):Boolean={
m>n
}
val a = Array(1, 2, 3, 3, 2, 3, 3, 4)
val b = a.sortWith(func)
b.foreach(println)//4, 3, 3, 3, 3, 2, 2, 1
方法名:sorted
使用规范:
def sorted[B >: A](implicit ord : scala.math.Ordering[B]) : Repr = { /* compiled code */ }
具体描述:默认升序排列,无参数
val a = Array(3, 2, 1, 4)
val b = a.sorted
println(b.mkString(",")) // 1,2,3,4
方法名:span
使用规范:
override def span(p : scala.Function1[A, scala.Boolean]) : scala.Tuple2[Repr, Repr] = { /* compiled code */ }
具体描述:将序列按条件拆分成两个数组,从第一个元素开始,直到第一个不满足条件的元素为止,满足条件的元素放到第一个数组,其余元素放到第二个数组
返回的是包含这两个数组的元祖
val a = Array(1, 2, 3, 4, 1, 2)
val b = a.span(x => x < 3)
println(b._1.mkString(",")) // 1,2
println(b._2.mkString(",")) // 3,4
方法名:splitAt
使用规范:
override def splitAt(n : scala.Int) : scala.Tuple2[Repr, Repr] = { /* compiled code */ }
具体描述:从指定位置开始,把序列拆分成两个数组
val a = Array(5,6,7,8,9)
val b = a.splitAt(2)
println(b._1.mkString(",")) // 5,6
println(b._2.mkString(",")) // 7,8,9
方法名:startsWith
使用规范:
def startsWith[B](that : scala.collection.GenSeq[B]) : scala.Boolean = { /* compiled code */ }
def startsWith[B](that : scala.collection.GenSeq[B], offset : scala.Int) : scala.Boolean
具体描述:判断序列是否以某个序列开始。
startsWith(that, offset) :判断序列从指定偏移处是否以某个序列开始)
val a = Array(1, 2, 3, 4)
val b = Array(1, 2)
println(a.startsWith(b)) // true
val c = Array(2, 3)
println(a.startsWith(c, 1)) // true
方法名:stringPrefix
使用规范: def stringPrefix : scala.Predef.String = { /* compiled code */ }
具体描述:返回 toString 结果的前缀,一般可以用来查看序列的类型
val a = Array(1, 2, 3, 4)
println(a.toString()) // [I@16c690dc
println(a.stringPrefix) // [I
方法名:sum
使用规范:
def sum[B >: A](implicit num : scala.Numeric[B]) : B = { /* compiled code */ }
具体描述:序列求和,元素需为 Numeric[T] 类型
val a = Array(1, 2, 3, 4, 1)
println(a.sum) // 11
方法名:tail
使用规范: override def tail : Repr = { /* compiled code */ }
具体描述:返回当前序列中不包含第一个元素的序列
val a = Array(1, 2, 3, 4)
val b = a.tail
println(b.mkString(",")) // 2,3,4
方法名:tails
使用规范:def tails : scala.collection.Iterator[Repr] = { /* compiled code */ }
具体描述:同 inits,每一步都进行 tail 操作
val a = Array(1, 2, 3, 4)
val b = a.tails.toList
for (i <- 0 until b.length) {
val s = "第 %d 个值: %s"
println(s.format(i + 1, b(i).mkString(",")))
}
/*
* 第 1 个值: 1,2,3,4
* 第 2 个值: 2,3,4
* 第 3 个值: 3,4
* 第 4 个值: 4
* 第 5 个值:
*/
方法名:take
使用规范: override def take(n : scala.Int) : Repr = { /* compiled code */ }
具体描述:返回当前序列中,前 n 个元素组成的序列
val a = Array(1, 2, 3, 4)
val b = a.take(3)
println(b.mkString(",")) // 1,2,3
方法名:takeRight
使用规范: override def takeRight(n : scala.Int) : Repr = { /* compiled code */ }
具体描述:返回当前序列中,从右边开始,后 n 个元素组成的序列
val a = Array(1, 2, 3, 4)
val b = a.takeRight(3)
println(b.mkString(",")) // 2,3,4
方法名:takeWhile
使用规范:
override def takeWhile(p : scala.Function1[A, scala.Boolean]) : Repr = { /* compiled code */ }
具体描述:返回当前序列中,从第一个元素开始,满足条件的连续元素组成的序列
val a = Array(1, 2, 3, 4, 1, 2)
val b = a.takeWhile(x => x < 3)
print(b.mkString(",")) // 1,2
方法名:to
使用规范:
override def to[Col[_]](implicit cbf: scala.collection.generic.CanBuildFrom[Nothing,Int,Col[Int]]): Col[Int]
具体描述:将序列转化为scala.collection.immutable.IndexedSeq[Int] = Vector
val a = Array(1,2,3,4)
a.to
scala.collection.immutable.IndexedSeq[Int] = Vector(1, 2, 3, 4)
方法名:toArray
使用规范:def toArray: Array[A]
具体描述:将序列转换成 Array 类型
方法名:toBuffer
使用规范:def toBuffer[A1 >: A]: Buffer[A1]
具体描述:将序列转换成 Buffer 类型
方法名:toIndexedSeq
使用规范:def toIndexedSeq: collection.immutable.IndexedSeq[T]
具体描述:将序列转换成 IndexedSeq 类型
方法名:toIterable
使用规范:def toIterable: collection.Iterable[T]
具体描述:将序列转换成可迭代的类型
方法名:toIterator
使用规范: def toIterator: collection.Iterator[T]
具体描述:将序列转换成迭代器,同 iterator 方法
方法名:toList
使用规范: def toList: List[T]
具体描述:将序列转换成 List 类型
方法名:toMap
使用规范: def toMap[T, U]: Map[T, U]
具体描述:将序列转转换成 Map 类型,需要被转化序列中包含的元素是 Tuple2 类型
方法名:toSeq
使用规范:def toSeq: collection.Seq[T]
具体描述:将序列转换成 Seq 类型
方法名:toSet
使用规范: def toSet[B >: A]: Set[B]
具体描述:将序列转换成 Set 类型
方法名:toStream
使用规范:def toStream: collection.immutable.Stream[T]
具体描述:将序列转换成 Stream 类型
方法名:toTraversable
使用规范: def toTraversable: Traversable[Int]
具体描述:将序列转换成 Traversable类型
方法名:toVector
使用规范: def toVector: Vector[T]
具体描述:将序列转换成 Vector 类型
方法名:transform
使用规范:
def transform(f: Int => Int): scala.collection.mutable.WrappedArray[Int]
具体描述:将序列中的元素转换成指定的元素(同类型)
val a = Array(1,2,3,4)
a.transform(x => 6)
Array(6,6,6,6)
方法名:transpose
使用规范:
def transpose[U](implicit asArray : scala.Function1[T, scala.Array[U]]) : scala.Array[scala.Array[U]] = { /* compiled code */ }
具体描述:矩阵转置,二维数组行列转换
val a = Array(Array("a", "b"), Array("c", "d"), Array("e", "f"))
val b = a.transpose
b.foreach(x => println((x.mkString(","))))
/*
* a,c,e
* b,d,f
*/
方法名:union
使用规范:
override def union[B >: A, That](that : scala.collection.GenSeq[B])(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:合并两个序列,同操作符 ++
val a = Array(1, 2)
val b = Array(3, 4)
val c = a.union(b)
println(c.mkString(",")) // 1,2,3,4
方法名:unzip
使用规范:
def unzip[T1, T2](implicit asPair : scala.Function1[T, scala.Tuple2[T1, T2]], ct1 : scala.reflect.ClassTag[T1], ct2 : scala.reflect.ClassTag[T2]) : scala.Tuple2[scala.Array[T1], scala.Array[T2]] = { /* compiled code */ }
具体描述:将含有两个二元组的数组,每个元组的第一个元素组成一个数组,第二个元素组成一个数组,返回包含这两个数组的元组
val a = Array(("a", "b"), ("c", "d"))
val b = a.unzip
println(b._1.mkString(",")) // a,c
println(b._2.mkString(",")) // b,d
方法名:unzip3
使用规范:
def unzip3[T1, T2, T3](implicit asTriple : scala.Function1[T, scala.Tuple3[T1, T2, T3]], ct1 : scala.reflect.ClassTag[T1], ct2 : scala.reflect.ClassTag[T2], ct3 : scala.reflect.ClassTag[T3]) : scala.Tuple3[scala.Array[T1], scala.Array[T2], scala.Array[T3]] = { /* compiled code */ }
具体描述:将含有3个元祖的数组,每个元祖的第一个元素组成一个数组,第二个元素组成一个数组,第三个元素组成一个数组,返回包含这三个数组的元组
val a = Array(("a", "b", "x"), ("c", "d", "y"), ("e", "f", "z"))
val b = a.unzip3
println(b._1.mkString(",")) // a,c,e
println(b._2.mkString(",")) // b,d,f
println(b._3.mkString(",")) // x,y,z
方法名:update
使用规范: def update(i : scala.Int, x : T) : scala.Unit = { /* compiled code */ }
具体描述:将序列中 i 索引处的元素更新为 x (直接在本数组上修改)
val a = Array(1, 2, 3, 4)
a.update(1, 5)
println(a.mkString(",")) //1,5,3,4
方法名:updated
使用规范:
def updated[B >: A, That](index : scala.Int, elem : B)(implicit bf : scala.collection.generic.CanBuildFrom[Repr, B, That]) : That = { /* compiled code */ }
具体描述:将序列中 i 索引处的元素更新为 x,并返回替换后的数组(修改副本)
val a = Array(1, 2, 3, 4)
val b = a.updated(1, 5)
println(b.mkString(",")) //1,5,3,4
方法名:view
使用规范:
override def view : scala.AnyRef with scala.collection.mutable.IndexedSeqView[A, Repr] = { /* compiled code */ }
override def view(from : scala.Int, until : scala.Int) : scala.collection.mutable.IndexedSeqView[A, Repr] = { /* compiled code */ }
具体描述:返回当前序列中从 from 到 until 之间的序列,不包括 until 处的元素(左闭右开)
val a = Array(1, 2, 3, 4)
val b = a.view(1, 3)
println(b.mkString(",")) // 2,3
方法名:withFilter
使用规范:
def withFilter(p : scala.Function1[A, scala.Boolean]) : scala.collection.generic.FilterMonadic[A, Repr] = { /* compiled code */ }
具体描述:根据条件 p 过滤元素
val a = Array(1, 2, 3, 4)
val b = a.withFilter(x => x > 2).map(x => x)
println(b.mkString(",")) // 3,4
方法名:zip
使用规范:
override def zip[A1 >: A, B, That](that : scala.collection.GenIterable[B])(implicit bf : scala.collection.generic.CanBuildFrom[Repr, scala.Tuple2[A1, B], That]) : That = { /* compiled code */ }
具体描述: 将两个序列对应位置上的元素组成一个元组数组,要求两个序列长度相同
val a = Array(1, 2, 3, 4)
val b = Array(4, 3, 2, 1)
val c = a.zip(b)
println(c.mkString(",")) // (1,4),(2,3),(3,2),(4,1)
方法名:zipAll
使用规范:
def zipAll[B, A1 >: A, That](that : scala.collection.GenIterable[B], thisElem : A1, thatElem : B)(implicit bf : scala.collection.generic.CanBuildFrom[Repr, scala.Tuple2[A1, B], That]) : That = { /* compiled code */ }
具体描述:同 zip ,但是允许两个序列长度不同,不足的自动填充,如果当前序列短,不足的元素填充为 thisElem,如果 that 序列短,填充为 thatElem
val a = Array(1, 2, 3, 4, 5, 6, 7)
val b = Array(5, 4, 3, 2, 1)
val c = a.zipAll(b, 8, 9) // (1,5),(2,4),(3,3),(4,2),(5,1),(6,9),(7,9)
println(c.mkString(","))
val x = Array(1, 2, 3, 4)
val y = Array(6, 5, 4, 3, 2, 1)
val z = x.zipAll(y, 8, 9) // (1,6),(2,5),(3,4),(4,3),(8,2),(8,1)
println(z.mkString(","))
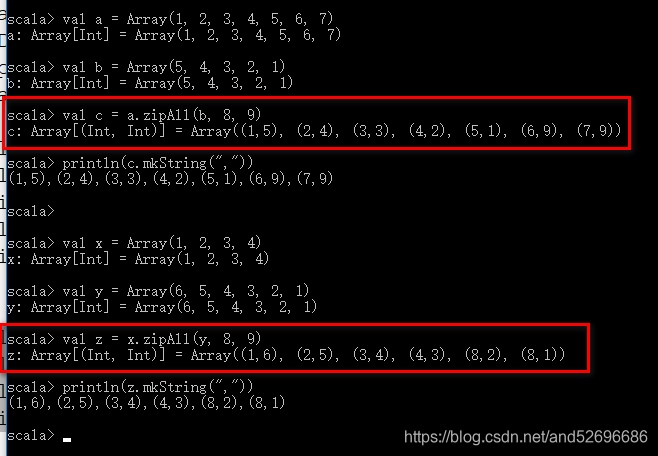
方法名:zipWithIndex
使用规范:
override def zipWithIndex[A1 >: A, That](implicit bf : scala.collection.generic.CanBuildFrom[Repr, scala.Tuple2[A1, scala.Int], That]) : That = { /* compiled code */ }
具体描述:序列中的每个元素和它的索引组成一个元组数组
val a = Array('a', 'b', 'c', 'd')
val b = a.zipWithIndex
println(b.mkString(",")) // (a,0),(b,1),(c,2),(d,3)
补充
方法名:subSequence
使用规范:
def subSequence(start : scala.Int, end : scala.Int) : java.lang.CharSequence = { /* compiled code */ }
具体描述: 返回 start 和 end 间的字符序列,不包含 end 处的元素
val a = Array('a', 'b', 'c', 'd')
val b = a.subSequence(1, 3)
println(b.toString) // bc