代码1(不支持https链接)
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.UUID;
public class Test {
public static void main(String[] args) {
String imgUrl = "https://bkimg.cdn.bcebos.com/pic/55e736d12f2eb938e8824ef3da628535e4dd6fc7?x-bce-process=image/watermark,image_d2F0ZXIvYmFpa2UxMTY=,g_7,xp_5,yp_5/format,f_auto";
File file = null;
FileOutputStream out = null;
HttpURLConnection conn = null;
InputStream inputStream = null;
File dir = new File("C:/test/img/" + new SimpleDateFormat("yyyy/MM-dd/HH").format(new Date()));
if (!dir.exists()) {
dir.mkdirs();
}
try {
file = new File(dir, UUID.randomUUID().toString().replace("-", "") + ".jpg");
URL url = new URL(imgUrl);
conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setReadTimeout(3000);
conn.setConnectTimeout(3000);
inputStream = conn.getInputStream();
out = new FileOutputStream(file);
byte[] arr = new byte[1024];
int len = 0;
while ((len = inputStream.read(arr)) != -1) {
out.write(arr, 0, len);
}
out.flush();
System.out.println("提醒:图片下载成功!!!\n图片保存地址:" + file.getAbsolutePath());
} catch (Exception e) {
e.printStackTrace();
} finally {
if (out != null) {
try {
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (conn != null) {
conn.disconnect();
}
if (inputStream != null) {
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
代码2(支持https链接)
import com.leadal.framework.util.UUIDUtil;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.conn.ClientConnectionManager;
import org.apache.http.conn.scheme.Scheme;
import org.apache.http.conn.scheme.SchemeRegistry;
import org.apache.http.conn.ssl.SSLSocketFactory;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.DefaultHttpClient;
import javax.net.ssl.SSLContext;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
import java.io.*;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
public class ImgUtil {
public static void main(String[] args) throws Exception {
String imgUrl = "https://bkimg.cdn.bcebos.com/pic/55e736d12f2eb938e8824ef3da628535e4dd6fc7";
File file = getImgFile(imgUrl);
System.out.println("提醒:图片下载成功!!!\n图片保存地址:" + file.getAbsolutePath());
}
public static File getImgFile(String imgUrl) {
File imgFile = FileUtil.createTempFile(UUIDUtil.create() + ".jpg");
CloseableHttpClient client = null;
try {
client = new DefaultSSLUtils();
} catch (Exception e) {
e.printStackTrace();
}
CloseableHttpResponse response = null;
InputStream inputStream = null;
FileOutputStream out = null;
try {
URIBuilder uriBuilder = new URIBuilder(imgUrl);
HttpGet httpGet = new HttpGet(uriBuilder.build());
RequestConfig requestConfig = RequestConfig.custom().setConnectTimeout(10000).setConnectionRequestTimeout(10000).setSocketTimeout(10000).build();
httpGet.setConfig(requestConfig);
response = client.execute(httpGet);
inputStream = response.getEntity().getContent();
out = new FileOutputStream(imgFile);
byte[] arr = new byte[1024];
int len = 0;
while ((len = inputStream.read(arr)) != -1) {
out.write(arr, 0, len);
}
out.flush();
} catch (Exception e) {
e.printStackTrace();
} finally {
close(client, response, inputStream, out);
}
return imgFile;
}
private static void close(Closeable... closeables) {
for (Closeable closeable : closeables) {
if (closeable != null) {
try {
closeable.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
class DefaultSSLUtils extends DefaultHttpClient {
public DefaultSSLUtils() throws Exception {
super();
SSLContext ctx = SSLContext.getInstance("TLS");
X509TrustManager tm = new X509TrustManager() {
@Override
public void checkClientTrusted(X509Certificate[] chain,
String authType) throws CertificateException {
}
@Override
public void checkServerTrusted(X509Certificate[] chain,
String authType) throws CertificateException {
}
@Override
public X509Certificate[] getAcceptedIssuers() {
return null;
}
};
ctx.init(null, new TrustManager[]{tm}, null);
SSLSocketFactory ssf = new SSLSocketFactory(ctx, SSLSocketFactory.ALLOW_ALL_HOSTNAME_VERIFIER);
ClientConnectionManager ccm = this.getConnectionManager();
SchemeRegistry sr = ccm.getSchemeRegistry();
sr.register(new Scheme("https", 443, ssf));
}
}
下载效果
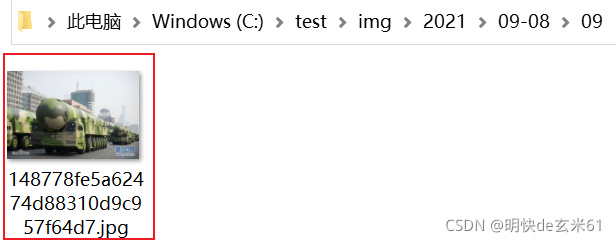