package com.prophesy.util;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.prophesy.bean.common.exception.BizException;
import lombok.extern.slf4j.Slf4j;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLSession;
import java.io.*;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
@Slf4j
public class IPFSHttpTool {
/**
* 下载文件
*/
public static void downFile(InputStream is, String savePath, String fileName) throws IOException {
BufferedOutputStream bos = null;
is = new BufferedInputStream(is);
try {
bos = new BufferedOutputStream(new FileOutputStream(savePath + "/" + fileName));
byte[] bytes = new byte[1024 * 8];
int len = 0;
while ((len = is.read(bytes)) != -1) {
bos.write(bytes);
}
} catch (FileNotFoundException e) {
log.error(e.getMessage());
} catch (IOException e) {
log.error(e.getMessage());
} finally {
is.close();
bos.close();
}
}
/**
* 获取Md5码文本
*/
public static String getMd5Code(String httpUrl, String path, String fileName, String reqType, String netType) {
StringBuffer result = new StringBuffer();
InputStream is;
try {
if (!"".equals(httpUrl) && !"".equals(path) && !"".equals(fileName) && !"".equals(reqType)) {
if ("0".equals(netType.trim())) {
is = httpDownFile(httpUrl, path, fileName, reqType);
} else if ("1".equals(netType.trim())) {
is = httpsDownFile(httpUrl, path, fileName, reqType);
} else {
throw new BizException("不支持的协议类型");
}
String data;
BufferedReader reader = new BufferedReader(new InputStreamReader(is, "UTF-8"));
while ((data = reader.readLine()) != null) {
result.append(data);
}
log.info(result.toString());
JSONObject jsonObject = JSON.parseObject(result.toString());
return replaceAllBlank(jsonObject.get("result").toString());
}
return null;
} catch (IOException e) {
throw new BizException(e.getMessage());
} catch (NullPointerException e) {
throw new BizException("md5码获取失败");
}
}
public static InputStream httpDownFile(String httpUrl, String path, String fileName, String type) {
DataOutputStream dataout = null;
InputStream is = null;
HttpURLConnection connection = null;
try {
URL url = new URL(httpUrl);
connection = (HttpURLConnection) url.openConnection();// 根据URL生成HttpURLConnection
connection.setDoOutput(true);// 设置是否向connection输出,因为这个是post请求,参数要放在http正文内,因此需要设为true,默认情况下是false
connection.setDoInput(true); // 设置是否从connection读入,默认情况下是true;
connection.setRequestMethod("1".equals(type) ? "POST" : "GET");// 设置请求方式为post,默认GET请求
if ("1".equals(type)) {
connection.setUseCaches(false);// post请求不能使用缓存设为false
}
connection.setConnectTimeout(3000);// 连接主机的超时时间
connection.setReadTimeout(3000);// 从主机读取数据的超时时间
connection.setInstanceFollowRedirects(true);// 设置该HttpURLConnection实例是否自动执行重定向
connection.setRequestProperty("connection", "Keep-Alive");// 连接复用
connection.setRequestProperty("charset", "utf-8");
connection.setRequestProperty("Content-Type", "application/json");
connection.connect();// 建立TCP连接,getOutputStream会隐含的进行connect,所以此处可以不要
dataout = new DataOutputStream(connection.getOutputStream());// 创建输入输出流,用于往连接里面输出携带的参数
JSONObject body = new JSONObject();
body.put("path", path);
body.put("fileName", fileName);
dataout.write(body.toString().getBytes("UTF-8"));
dataout.flush();
dataout.close();
if (connection.getResponseCode() == HttpURLConnection.HTTP_OK) {
is = connection.getInputStream();
}
} catch (MalformedURLException e) {
throw new BizException("URL地址异常");
} catch (IOException e) {
throw new BizException(e.getMessage());
}
if (is == null) {
throw new BizException("文件下载异常");
}
return is;
}
public static String replaceAllBlank(String str) {
String s = "";
if (str != null) {
Pattern p = Pattern.compile("\\s*|\t|\r|\n");
/*\n 回车(\u000a)
\t 水平制表符(\u0009)
\s 空格(\u0008)
\r 换行(\u000d)*/
Matcher m = p.matcher(str);
s = m.replaceAll("");
}
return s;
}
public static InputStream httpsDownFile(String httpUrl, String path, String fileName, String type) {
DataOutputStream dataout = null;
InputStream is = null;
HttpsURLConnection connection = null;
try {
URL url = new URL(httpUrl);
if ("1".equals(type)) {
HostnameVerifier hv = new HostnameVerifier() {
public boolean verify(String urlHostName, SSLSession session) {
return true;
}
};
trustAllHttpsCertificates();
HttpsURLConnection.setDefaultHostnameVerifier(hv);
}
connection = (HttpsURLConnection) url.openConnection();// 根据URL生成HttpURLConnection
connection.setDoOutput(true);// 设置是否向connection输出,因为这个是post请求,参数要放在http正文内,因此需要设为true,默认情况下是false
connection.setDoInput(true); // 设置是否从connection读入,默认情况下是true;
connection.setRequestProperty("accept", "*/*");
connection.setRequestProperty("connection", "Keep-Alive");
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("user-agent", "Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; SV1)");
connection.setRequestProperty("charset", "utf-8");
connection.setRequestMethod(type == "1" ? "POST" : "GET");// 设置请求方式为post,默认GET请求
if ("1".equals(type)) {
connection.setUseCaches(false);// post请求不能使用缓存设为false
}
connection.setConnectTimeout(30000);// 连接主机的超时时间
connection.setReadTimeout(30000);// 从主机读取数据的超时时间
connection.setInstanceFollowRedirects(true);// 设置该HttpURLConnection实例是否自动执行重定向
connection.connect();// 建立TCP连接,getOutputStream会隐含的进行connect,所以此处可以不要
dataout = new DataOutputStream(connection.getOutputStream());// 创建输入输出流,用于往连接里面输出携带的参数
JSONObject json = new JSONObject();
json.put("path", path);
json.put("fileName", fileName);
dataout.write(json.toJSONString().getBytes("UTF-8"));
dataout.flush();
dataout.close();
if (connection.getResponseCode() == HttpsURLConnection.HTTP_OK) {
is = connection.getInputStream();
}
} catch (Exception e) {
throw new BizException(e.getMessage());
}
if (is == null) {
throw new BizException("文件下载异常");
}
return is;
}
private static void trustAllHttpsCertificates() throws Exception {
javax.net.ssl.TrustManager[] trustAllCerts = new javax.net.ssl.TrustManager[1];
javax.net.ssl.TrustManager tm = new miTM();
trustAllCerts[0] = tm;
javax.net.ssl.SSLContext sc = javax.net.ssl.SSLContext
.getInstance("SSL");
sc.init(null, trustAllCerts, null);
javax.net.ssl.HttpsURLConnection.setDefaultSSLSocketFactory(sc
.getSocketFactory());
}
static class miTM implements javax.net.ssl.TrustManager,
javax.net.ssl.X509TrustManager {
public java.security.cert.X509Certificate[] getAcceptedIssuers() {
return null;
}
public boolean isServerTrusted(
java.security.cert.X509Certificate[] certs) {
return true;
}
public boolean isClientTrusted(
java.security.cert.X509Certificate[] certs) {
return true;
}
public void checkServerTrusted(
java.security.cert.X509Certificate[] certs, String authType)
throws java.security.cert.CertificateException {
return;
}
public void checkClientTrusted(
java.security.cert.X509Certificate[] certs, String authType)
throws java.security.cert.CertificateException {
return;
}
}
}
IPFSHttpUtils
于 2022-04-28 11:54:06 首次发布
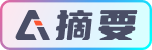