shiro
1.shiro介绍
apache shiro是一款简单且功能强大的能够完成身份认证,用户授权,会话管理的安全框架;
2.shiro跟spring security的区别:
* shiro是一款轻量级的安全框架,使用简单,不需要依赖spring,但是功能不如spring security(如oahtn2)
* spring security是一款重量级的安全框架,功能全面,但是必须整合spring使用
3.shiro的组件:
* subject: 主体,即用户
* security manager:安全管理器,shiro的核心部分;包括authenticator(认证器),authrizor(授权器),realm(安全数据源)
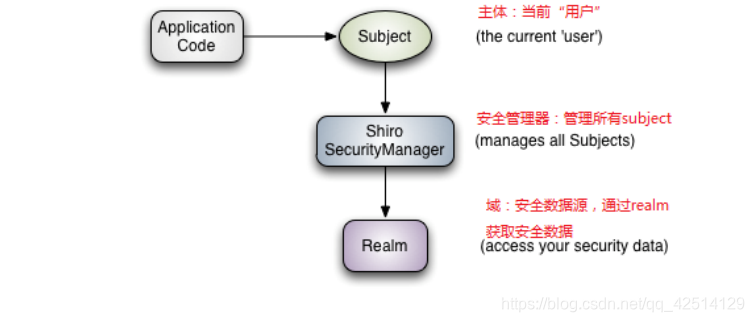
shiro入门测试
<!-----------身份认证------------>
1.配置安全数据源realm
* 在resources目录下,创建shiro-auth1.ini
[users] #模拟在数据库中定义的username,password
zhangsan=123
lisi=456
2.编写测试程序
public void testLogin() {
IniSecurityManagerFactory factory = new IniSecurityManagerFactory("classpath:shiro-auth1.ini");
SecurityManager manager = factory.getInstance();
String username = "zhangsan";
String password = "123";
UsernamePasswordToken token = new UsernamePasswordToken(username, password);
SecurityUtils.setSecurityManager(manager);
Subject subject = SecurityUtils.getSubject();
subject.login(token);
System.out.println("身份认证成功..." + subject.isAuthenticated());
}
<!-----------用户授权------------>
1.配置安全数据源realm
* 在resources目录下,创建shiro-auth2.ini
[users] #模拟在数据库中定义的username,password;password后面跟的就是用户拥有的角色
zhangsan=123,role1,role2
lisi=456,role1
[roles] #角色所拥有的权限
role1=save,delete,update
role2=query
2.编写测试程序
public void testRole() {
IniSecurityManagerFactory factory = new IniSecurityManagerFactory("classpath:shiro-2.ini");
SecurityManager securityManager = factory.getInstance();
String username = "zhangsan";
String password = "123";
UsernamePasswordToken token = new UsernamePasswordToken(username, password);
SecurityUtils.setSecurityManager(securityManager);
Subject subject = SecurityUtils.getSubject();
subject.login(token);
System.out.println(subject.hasRole("role1") ? "当前主体有role1角色" : "没有..");
}
<!-----------自定义realm,从数据库查询数据------------>
1.配置安全数据源realm
* 在resources目录下,创建shiro-auth3.ini
[main]
#声明realm,指定自定义realm的全类名
permRealm=com.baidu.shiro2.PermisssionRealm
#将realm注册到SecurityManager中,注意$符不能掉
securityManager.realms=$permRealm
2.编写测试程序,同上
3.自定义realm,继承AuthorizingRealm
public class PermisssionRealm extends AuthorizingRealm {
@Autowired
private AuthorizingDao authorizingDao;
@Override
public void setName(String name) {
super.setName("permisssionRealm");
}
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken authenticationToken) throws AuthenticationException {
UsernamePasswordToken token = (UsernamePasswordToken) authenticationToken;
String username = token.getUsername();
String password = new String(token.getPassword());
int count = authorizingDao.login(username,password);
if(count!=1){
throws new RuntimeException("账号密码错误...");
}
SimpleAuthenticationInfo info = new SimpleAuthenticationInfo(username, password, getName());
return info;
}
protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principalCollection) {
String username = (String) principalCollection.getPrimaryPrincipal();
Set<String> roleSet = new HashSet<String>();
Set<String> permissionSet = new HashSet<String>();
authorizingDao.getRoleAndPermission(roleSet,permissionSet,username);
SimpleAuthorizationInfo info = new SimpleAuthorizationInfo();
info.setRoles(roleSet);
info.setStringPermissions(permissionSet);
return info;
}
}
shiro坐标
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-core</artifactId>
<version>1.3.2</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<version>1.1.3</version>
</dependency>