ServiceComb
1.ServiceCombo介绍:
ServiceCombo是Apache下的一款微服务框架,它的前身是华为云服务引擎(Cloud Service Engine);
ServiceCombo在微服务开发上比起SpringCloud更胜一筹(ServiceCombo提供了分布式事务解决方案);官
网:http://servicecomb.incubator.apache.org/
2.ServiceCombo设计思想:
ServiceCombo主要由编程模型,运行模型和通信模型组成;由这三者的不同组合来形成完整的ServiceCombo
3.ServiceCombo快速开发脚手架:
http://start.servicecomb.io/
4.ServiceComb运行模型:(直接使用脚手架生成)
* 负载均衡(LoadBalance): ServiceComb内置基于Ribbon的负载均衡
* 限流(FlowControl): ServiceComb基于Zuul网关实现限流
* 熔断机制(CircuitBroker): 使用熔断机制解决雪崩效应
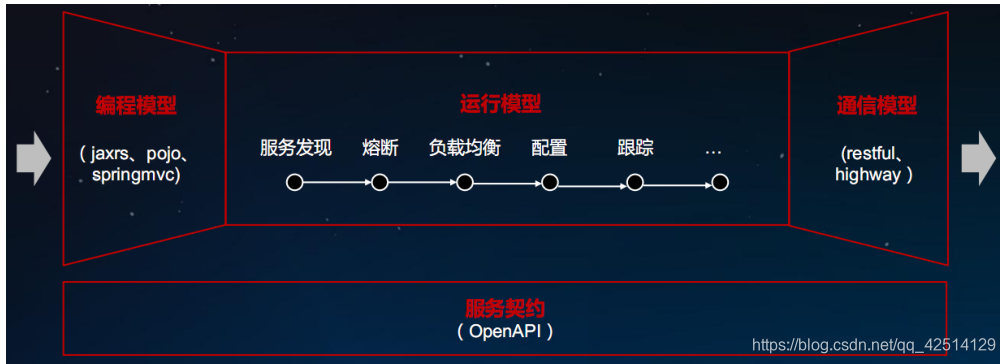
ServiceCombo入门Demo–rest通信模型
1.编写公共接口
public interface RestService{
String satHello(String name);
}
2.编写Provider
2.1编写引导类,在引导类上加@EnableServiceComb注解
@EnableServiceComb
@SpringBootApplication
public class ProviderApplication{
public static void main(String[] args){
SpringApplication.run(ProviderApplication.class);
}
}
2.2编写提供者实现类
@RestSchema(schemaId = "restServiceImpl")
@RequestMapping("/say")
public class RestServiceImpl implements RestService{
@GetMapping("/hello")
public String satHello(String name){
return "Hello " + name;
}
}
2.3编写microservice.yaml,固定名称
APPLICATION_ID: start.servicecomb.io #应用名称任意,但是provider和consumer必须保持一致
service_description:
name: myProvider #微服务名称,供consumer引用
version: 0.0.1
servicecomb:
handler:
chain:
Provider: {}
rest:
address: 0.0.0.0:8888 #consumer访问provider引用的IP和端口
service:
registry:
address: http://127.0.0.1:30100 #cse注册中心的IP和端口
autodiscovery: false
3.编写consumer
3.1编写引导类
@EnableServiceComb
@SpringBootApplication
public class ConsumerApplication{
public static void main(String[] args){
SpringApplication.run(ConsumerApplication.class);
}
@Bean
public RestTemplate restTemplate(){
return RestTemplateBuilder.create();
}
}
3.2编写provider调用者,注意跟真正Provider的区别
@Service
public class RestServiceInvoker implements RestService{
@Autowired
private RestTemplate restTemplate;
public String satHello(String name){
String providerName = "myProvider";
String url = "cse://" + providerName + "/say/hello?name=" + name;
}
}
3.3编写controller
@RestSchema( schemaId = "restController")
@RequestMapping("/test")
public class RestController{
@Autowired
private RestServiceInvoker restServiceInvoker;
@ResponseBody
@GetMapping("/testSay")
public String sayHello(String name){
return restServiceInvoker.satHello(name);
}
}
3.4编写microservice.yaml
APPLICATION_ID: start.servicecomb.io
service_description:
name: myConsumer #微服务名称
version: 0.0.1
servicecomb:
handler:
chain:
Provider: {}
rest:
address: 0.0.0.0:9999 #consumer供浏览器访问的IP端口
service:
registry:
address: http://127.0.0.1:30100 #cse注册中心的IP和端口
autodiscovery: false
测试:
先打开cse注册中心,双击service-center.exe(在官网下载);
启动provider,consumer(顺序无所谓,consumer是在真正调用provider时才去注册中心发现服务)
localhost:9999/test/testSay,在这种模式下,service也可以直接被浏览器访问
注意:
1.controller类上也必须使用@RestSchema注解
2.RestTemplate必须使用ServiceComb提供的实现
pom.xml
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.12.RELEASE</version>
<relativePath/>
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.apache.servicecomb</groupId>
<artifactId>java-chassis-dependencies</artifactId>
<version>1.0.0-m2</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
<dependency>
<groupId>org.apache.servicecomb</groupId>
<artifactId>spring-boot-starter-provider</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.baidu</groupId>
<artifactId>service-interface</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
注意:在install接口工程时,需要先将spring-boot-maven-plugin注释掉,否则install时需要依赖引导类,而接口工程是没有引导类的