一、Object类的介绍
Object类是所有Java类的根基类,也就意味着所有的Java对象都拥有Object类的属性和方法。如果在类的声明中未使用extends关键字指明其父类,则默认继承Object类。Object类中定义了一些JAVA所有的类都必须具有的一些方法
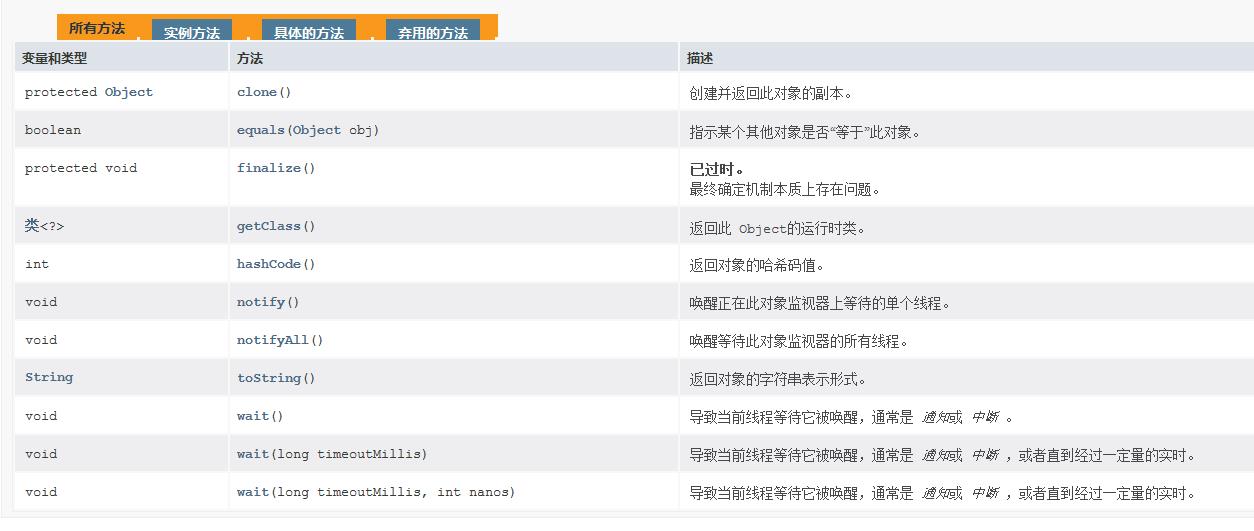
1.==和equals方法
“==”代表比较双方是否相同。如果是基本类型则表示值相等,如果是引用类型则表示地址相等即是同一个对象。
Object 的 equals 方法默认就是比较两个对象的hashcode,是同一个对象的引用时返回 true 否则返回 false。显然,这无法满足子类的要求,可根据要求重写equals方法。
/*
* ==
* 两端如果是基本数据类型,那么就是单纯判断值是否相同
* 两端是引用类型,那么判断的引用的地址是否相同,判断是否指向内存上的同一个数据
* */
int a=10;
int b=10;
System.out.println(a==b);
String s =new String("pptv");
String s2=new String("pptv");
System.out.println(s);
System.out.println(s2);
System.out.println(s==s2);//false
String s3="abc";
String s4="abc";
System.out.println(s3==s4);//true
System.out.println(s.equals(s2));//true
System.out.println(s3.equals(s4));//true
}
重写equals方法
/**
* 判断自定义类的两个对象属性值是否全部相同
* 重写equals方法即可 alt+insert
* 使用equals方法去判断
*
*/
Dog dog = new Dog("rous","red",11);
Dog dog1 = new Dog("pans","green",11);
boolean equals = dog.equals(dog1);
System.out.println(equals);
public class Dog {
private String type;
private String color;
private int month;
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Dog dog = (Dog) o;
//通过month属性来判断是否是同一个dog,month值相等则是
return month == dog.month;
}
}
2. hashcode方法
哈希码:hash码,散列码,是一种无序不重复的一串十六进制数据,Object类中定义的方法作用就是为每一个对象生成一个独立的哈希码,用以区分每个对象,我们可以通过重写的方式自定义哈希码的声明规则,让其和属性值相关联.一般在重写equals方法时,都会重写hashcode方法
/**
* 判断自定义类的两个对象属性值是否全部相同
* 重写equals方法即可 alt+insert
* 使用equals方法去判断
*
*/
Dog dog = new Dog("哈士奇","green",11);
Dog dog1 = new Dog("哈士奇","green",11);
boolean equals = dog.equals(dog1);
System.out.println(equals);//true
System.out.println(dog.hashCode());//-1968384693
System.out.println(dog1.hashCode());//-1968384693
public class Dog {
private String type;
private String color;
private int month;
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Dog dog = (Dog) o;
return month == dog.month &&
Objects.equals(type, dog.type) &&
Objects.equals(color, dog.color);
}
@Override
public int hashCode() {
return Objects.hash(type, color, month);
}
}
3.toString方法
Object 类中定义类一个方便我们快捷查看对象属性信息的一个方法,toString的目的是返回一个对象的字符串表达形式,Object类中定义的方法规则为类的全路径名+哈希码,我们可以通过重写的方式重新定义该方法
@Override
public String toString() {
return "Dog{" +
"type='" + type + '\'' +
", color='" + color + '\'' +
", month=" + month +
'}';
}
二、包装类
Java提供了两个类型系统,基本类型与引用类型,使用基本类型在于效率,然后很多情况,会创建对象使用,因为对象可以做更多的功能,如果想要我们的基本类型像对象一样操作,就可以使用基本类型对应的包装类.
byte–>Byte
short–>Short
int–>Integer
long–>Long
char–>Character
float–>Float
double–>Double
boolean–>Boolean
装箱与拆箱
基本类型与对应的包装类对象之间,来回转换的过程称为"装箱"与"拆箱"。
装箱
:从基本类型转换成对应的包装对象
Integer i = new Integer(5);//使用构造函数
Integer i2 = Integer.valueOf(77);//使用包装类中的valueOf方法
拆箱
:从包装类对象转换为对应的基本类型
int num = i2.intValue();
自动装箱与自动拆箱
由于我们经常要做基本类型与包装类之间的转换,从JDK1.5开始,基本类型与包装类的装箱、拆箱动作可以自动完成
Integer integer1=6;//自动装箱
int i = integer1 + 1;//自动拆箱
基本类型与字符串之间的装换
基本类型转字符串
int a=26;
String aStr=a+"";
字符串转基本类型
int i = Integer.parseInt("100");
**注意:**
1. JDK1.5以后,增加了自动装箱与拆箱功能,如:Integer i = 100; int j = new Integer(100);
2. 自动装箱调用的是valueOf()方法,而不是new Integer()方法。
3. 自动拆箱调用的xxxValue()方法。
4. 包装类在自动装箱时为了提高效率,对于-128~127之间的值会进行缓存处理。超过范围后,对象之间不能再使用==进行数值的比较,而是使用equals方法。
三、String类
字符串对象,程序中定义""都是字符串对象,这个对象的使用频率最高.
字符串类 java.lang.String类,继承Object类,实现了三个接口.
程序中只要你写 “里面任意” 都是String类的对象.
字符串对象是常量,一旦创建不能修改.
字符串的实现原理
字符串这个数据类,在Java中是不存在的,字符串的实现原理是用char[]数组表示.
“abc”,使用数组char[] ch = {‘a’,‘b’,‘c’} ;来表示.
JDK9版本之后,节约内存,char数组改变为了byte数组
JDK8版本以前都是char数组
private final char value[]; //JDK中String类的源码
数组的前面的修饰符final, 最终的数组,数组一旦建立,数组的地址就被锁死(常量)使用常量的原因,为了线程安全
2.1 构建字符串方式
//1.构造函数
String str = "hello";
String helloStr = new String("hello");
//2.字符数组构建字符串
char [] chars={'h','e','l','l','o'};
String s = new String(chars);
System.out.println(s);
//3.字节数组构建字符串
byte [] bytes={97,98,99,100};
String s1 = new String(bytes); //abcd;
String s2 = new String(bytes, 0, 3);//abc;
2.2 String常用方法
charAt(int index)
:返回指定索引的 char的值
String str="abc123456";
char charAt = str.charAt(4);//2;
concat(String str)
:将指定字符串连接到该字符串的末尾(创建一个新的字符数组,存放原来字符数组和新加入的字符数组内容,然后以该新数组创建一个新的字符串。)
String str="abc123456";
String helloStr = str.concat("hello");//abc123456hello;
contains(CharSequence s)
:当此字符串包含指定char值序列返回true
String str="abc123456";
boolean con = str.contains("abc");//true
endsWith(String suffix)
:测试此字符串是否以指定的后缀结尾
String str="hello.txt";
boolean b = str.endsWith(".txt");//true
startsWith(String prefix)
:测试此字符串是否以指定的开头
String str="hello.txt";
boolean b = str.startsWith("hello");//true
equals(Object anObject)
:将字符串与指定对象比较
String str="abc";
boolean abc = str.equals("abc"); //true
equalsIgnoreCase(String anotherString)
:将字符串与指定对象比较,忽略大小写
String str="abc";
boolean abc = str.equalsIgnoreCase("aBc"); //true
toCharArray()
:将此字符串转换为一个新的字符数组。
String str="abc";
char[] chars = str.toCharArray();
System.out.println(Arrays.toString(chars));//[a, b, c]
indexOf(int ch)
:返回在指定字符的第一个发生的字符串中的索引。
String str="abc123a456";
int a = str.indexOf("a");//0;
indexOf(int ch, int fromIndex)
:返回在指定字符的第一个发生的字符串中的索引,在指定的索引处开始搜索。
String str="abc123a456";
int a = str.indexOf("a",3);//6
isEmpty()
:判断_字符串_是否为空。
String str="";//true
String str1=" ";//false
boolean empty = str.isEmpty();
replace(char oldChar, char newChar)
:返回一个新的字符串,它是通过用newChar
替换此字符串中出现的所有oldChar
得到的。
String str="www123www";
String s = str.replace("www", "123"); //123123123;
split(String regex)
:按照切符,将此字符串分割为数组(分隔符放在首位,中间起作用,放在末尾不起作用)
String str="--www--123--www--";
String[] split = str.split("--");
System.out.println(Arrays.toString(split));//[, www, 123, www]
substring(int beginIndex)
:返回一个字符串,这个字符串的子串
String str="abc12345";
String substring = str.substring(3);//12345;
String substring1 = str.substring(0, 3);//abc;包含前面索引不包含后面索引
toLowerCase()
转小写、toUpperCase()
转大写
String str="aBc";
String s = str.toUpperCase();//ABC
String s1 = str.toLowerCase();//abc
trim()
:返回一个字符串,其值为此字符串,并删除任何前导和尾随空格,不会删除中间空格
String str=" abc de ";
System.out.println("使用trim后字符长度"+str.trim().length());//使用trim后字符长度6;
System.out.println("使用前长度"+str.length());//使用前长度8
2.3 字符串内存分析
String str1 = "abcd";
String str2=new String("abcd");
System.out.println(str1==str2);//false;
String str3="ab";
String str4="cd";
String newStr=str3+str4;//此处调用了new StringBuffer().append(str3).append(str4).toString();
System.out.println(str1==newStr);//false;
String str5="ab"+"cd";
System.out.println(str1==str5);//true
final String str="cd";
String str6="ab"+str;
System.out.println(str1==str6);//true
2.4 StringBuilder
一个可变的字符序列,字符序列就是字符数组
String 类中 : private final char[] value;
StringBuilder 类中 : char[] value;
字符序列是数组,Java数组的是定长的,一旦创建,长度固定!
创建对象的时候,StringBuilder中的数组的初始化长度为16个字符
StringBuilder自动的进行数组的扩容,新数组实现,原来数组的中元素复制到新的数组.(扩容的默认策略时候增加到原来长度的2倍再加2)
结论 : 无论怎么做字符串的操作,StringBuilder内部永远只有一个数组
StringBuilder类是线程不安全的类,运行速度快 , 推荐使用StringBuilder
StringBuffer是线程安全的类,运行速度慢,多线程的程序,使用
两个类的构造方法,和其他的方法,一模一样.
StringBuffer sb = new StringBuffer();
//sb.append()追加操作;
sb.append("abc").append("123").append("111");
System.out.println(sb.toString());//abc123111;
//sb.insert(6,"abc");在指定位置插入;
sb.insert(6,"abc");
System.out.println(sb.toString());//abc123abc11 ;
sb.delete(6,9);//包含前面下标,不包含后面下标;
System.out.println(sb.toString());//abc123111;
//字符串长度;
System.out.println(sb.length());
StringBuffer sb = new StringBuffer("ppt123");
sb.reverse();//倒排321tpp
//替换指定位置的内容
StringBuffer sb1 = sb.replace(1, 3, "999");//p999123
四 、日期类
4.1 Date
- Date表示特定的瞬间,精确到毫秒。
- Date类的大部分方法都已经被Calender类中的方法所取代
Date now = new Date();
//输出当前的时间
System.out.println(now.toString());//Tue Oct 25 14:18:41 CST 2022
System.out.println(now.toLocaleString());//2022-10-25 14:18:41
System.out.println(now.getYear()); // 结果122从1900开始+122=2022
System.out.println(now.getMonth());// 0---11
System.out.println(now.getDate());//日
//星期几 星期日是0,一周是从星期日开始
System.out.println(now.getDay());
System.out.println(now.getHours());
System.out.println(now.getMinutes());
System.out.println(now.getSeconds());
4.2 Calender
- Calender提供了获取设置各种日历字段的方法。
- protected Calender()构造方法为protected修饰,无法直接创建该对象。
常用方法:
- 使用默认时区和区域获取日历
Calendar now = Calendar.getInstance();
- 获取年月日时分秒
System.out.println("年:"+now.get(Calendar.YEAR));
System.out.println("月:"+now.get(Calendar.MONTH));//(0-11)
System.out.println("日:"+now.get(Calendar.DAY_OF_MONTH));
System.out.println("时:"+now.get(Calendar.HOUR_OF_DAY));
System.out.println("分:"+now.get(Calendar.MINUTE));
System.out.println("秒:"+now.get(Calendar.SECOND));
- 设置年月日时分秒
now.set(2011,11,11,11,11,11);
//单独设置
now.set(Calendar.YEAR,2033);
- Date转Calender
now.setTime(new Date());
- Calender转Date
Date time = now.getTime();//Tue Oct 25 14:24:00 CST 2022
- 按照日历规则,给指定字段添加或减少时间量
now.add(Calendar.MONTH,2);
4.3 simpleDateFormat
SimpleDateFormat
是以语言环境有关的方式来格式化和解析日期的类。
String 转Date
String str = "2011-11-11 12:11:11";
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date parse = simpleDateFormat.parse(str);//Fri Nov 11 12:11:11 CST 2011
Date转String
Date date = new Date();
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy年MM月dd日");
String time = simpleDateFormat.format(date);
System.out.println(time);//2021年08月01日
4.4 JDK8新的时间日期对象
1.LocalDate 本地日期
- 获取该类的对象,静态方法
- static LocalDate now() 获取LocalDate的对象,跟随操作系统
- static LocalDate of() 获取LocalDate的对象,自己设置日期
- of方法中传递年月日 of(int year,int month,int day)
/**
* LocalDate的静态方法获取对象
*/
public static void getInstance(){
//静态方法now()
LocalDate localDate = LocalDate.now();
System.out.println("localDate = " + localDate);
//静态方法of()设置日期
LocalDate of = LocalDate.of(2022,5,10);
System.out.println("of = " + of);
}
- 获取日期字段的方法 : 名字是get开头
- int getYear() 获取年份
- int getDayOfMonth()返回月中的天数
- int getMonthValue() 返回月份
/**
* LocalDate类的方法 getXXX()获取日期字段
*/
public static void get(){
LocalDate localDate = LocalDate.now();
//获取年份
int year = localDate.getYear();
//获取月份
int monthValue = localDate.getMonthValue();
//获取天数
int dayOfMonth = localDate.getDayOfMonth();
System.out.println("year = " + year);
System.out.println("monthValue = " + monthValue);
System.out.println("dayOfMonth = " + dayOfMonth);
}
- 设置日期字段的方法 : 名字是with开头
- LocalDate withYear(int year)设置年份
- LocalDate withMonth(int month)设置月份
- LocalDate withDayOfMonth(int day)设置月中的天数
- LocalDate对象是不可比对象,设置方法with开头,返回新的LocalDate对象
/**
* LocalDate类的方法 withXXX()设置日期字段
*/
public static void with(){
LocalDate localDate = LocalDate.now();
System.out.println("localDate = " + localDate);
//设置年,月,日
//方法调用链
LocalDate newLocal = localDate.withYear(2025).withMonth(10).withDayOfMonth(25);
System.out.println("newLocal = " + newLocal);
}
- 设置日期字段的偏移量, 方法名plus开头,向后偏移
- 设置日期字段的偏移量, 方法名minus开头,向前偏移
/**
* LocalDate类的方法 minusXXX()设置日期字段的偏移量,向前
*/
public static void minus() {
LocalDate localDate = LocalDate.now();
//月份偏移10个月
LocalDate minusMonths = localDate.minusMonths(10);
System.out.println("minusMonths = " + minusMonths);
}
/**
* LocalDate类的方法 plusXXX()设置日期字段的偏移量,向后
*/
public static void plus(){
LocalDate localDate = LocalDate.now();
//月份偏移10个月
LocalDate plusMonths = localDate.plusMonths(10);
System.out.println("plusMonths = " + plusMonths);
}
2. Period和Duration类
2.1 Period 计算日期之间的偏差
- static Period between(LocalDate d1,LocalDate d2)计算两个日期之间的差值.
- 计算出两个日期相差的天数,月数,年数
public static void between(){
//获取2个对象,LocalDate
LocalDate d1 = LocalDate.now(); // 2021-4-13
LocalDate d2 = LocalDate.of(2022,4,13); // 2022-6-15
//Period静态方法计算
Period period = Period.between(d1, d2);
//period非静态方法,获取计算的结果
int years = period.getYears();
System.out.println("相差的年:"+years);
int months = period.getMonths();
System.out.println("相差的月:"+months);
int days = period.getDays();
System.out.println("相差的天:"+days);
}
2.2 Duration计算时间之间的偏差
- static Period between(Temporal d1,Temporal d2)计算两个日期之间的差值.
public static void between(){
LocalDateTime d1 = LocalDateTime.now();
LocalDateTime d2 = LocalDateTime.of(2021,5,13,15,32,20);
// Duration静态方法进行计算对比
Duration duration = Duration.between(d1, d2);
// Duration类的对象,获取计算的结果
long minutes = duration.toMinutes();
System.out.println("相差分钟:" + minutes);
long days = duration.toDays();
System.out.println("相差天数:"+days);
long millis = duration.toMillis();
System.out.println("相差秒:" + millis);
long hours = duration.toHours();
System.out.println("相差小时:"+hours);
}
2.3 DateTimeFormatter
JDK8中的日期格式化对象 : java.time.format包
- static DateTimeFormatter ofPattern(String str)自定义的格式
- String format(TemporalAccessor t)日期或者时间的格式化
- TemporalAccessor parse(String s)字符串解析为日期对象
/**
* 方法parse,字符串转日期
*/
public static void parse(){
//静态方法,传递日期格式,返回本类的对象
DateTimeFormatter dateTimeFormatter =
DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String str = "2021-04-13 15:55:55";
//dateTimeFormatter调用方法parse转换
//返回接口类型,接口是LocalDate,LocalDateTime 都实现了该接口
TemporalAccessor temporalAccessor = dateTimeFormatter.parse(str);
//System.out.println(temporalAccessor);
LocalDateTime localDateTime = LocalDateTime.from(temporalAccessor);
System.out.println(localDateTime);
}
/**
* 方法format格式化
*
*/
public static void format(){
//静态方法,传递日期格式,返回本类的对象
DateTimeFormatter dateTimeFormatter =
DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
//dateTimeFormatter对象调用方法format
String format = dateTimeFormatter.format(LocalDateTime.now());
System.out.println(format);
}
五、 BigDecimal
很多实际应用中需要精确计算,而double是近似值存储,不再符合要求,需要借助BigDecimal
。
double d1=1.0;
double d2=0.9;
System.out.println(d1-d2);//0.09999999999999998
BigDecimal
:计算浮点数
浮点数的加减乘除
BigDecimal b1 = new BigDecimal("1.0");
BigDecimal b2 = new BigDecimal("0.9");
//加减乘
BigDecimal add = b1.add(b2);
System.out.println(add);//1.9
BigDecimal subtract = b1.subtract(b2);
System.out.println(subtract);//0.1;
BigDecimal multiply = b1.multiply(b2);
System.out.println(multiply);//0.90;
//除
BigDecimal b3 = new BigDecimal("0.3");
BigDecimal divide = b1.divide(b3,4,BigDecimal.ROUND_HALF_UP);//BigDecimal.ROUND_HALF_UP四舍五入
System.out.println(divide);//3.3333;
六、System系统方法
System系统类,主要用于获取系统的属性数据和其他操作。
常用方法:
- 复制数组
System.arraycopy(源数组,从哪个位置开始复制,目标数组,目标数组的位置,复制的长度);
int[] arr={3,11,31,21,55};
int[] newArr=new int[5];
//
System.arraycopy(arr,1,newArr,1,3);
System.out.println(Arrays.toString(newArr));//[0, 11, 31, 21, 0]
- 获取当前系统时间返回是毫秒值
System.currentTimeMillis();
- 建议jvm赶快启动垃圾回收器回收垃圾
System.gc();
- 退出jvm,如果参数是0表示正常退出jvm,非0表示异常退出jvm。
System.exit(0);
七、 Math类
- static double PI 圆周率
- static double E 自然数的底数
- static int abs(int a) 返回参数的绝对值
- static double ceil(double d)返回大于或者等于参数的最小整数
- static double floor(double d)返回小于或者等于参数的最大整数
- static long round(double d)对参数四舍五入
- static double pow(double a,double b ) a的b次幂
- static double random() 返回随机数 0.0-1.0之间
- static double sqrt(double d)参数的平方根
public static void main(String[] args) {
// System.out.println("Math.PI = " + Math.PI);
// System.out.println("Math.E = " + Math.E);
//static int abs(int a) 返回参数的绝对值
System.out.println(Math.abs(-6));
//static double ceil(double d)返回大于或者等于参数的最小整数
System.out.println(Math.ceil(12.3)); //向上取整数
//static double floor(double d)返回小于或者等于参数的最大整数
System.out.println("Math.floor(5.5) = " + Math.floor(5.5));//向下取整数
//static long round(double d)对参数四舍五入
long round = Math.round(5.5); //取整数部分 参数+0.5
System.out.println("round = " + round);
//static double pow(double a,double b ) a的b次幂
System.out.println("Math.pow(2,3) = " + Math.pow(2, 3));
//static double sqrt(double d)参数的平方根
System.out.println("Math.sqrt(4) = " + Math.sqrt(3));
// static double random() 返回随机数 0.0-1.0之间
for(int x = 0 ; x < 10 ; x++){
System.out.println(Math.random()); //伪随机数
}
}
八、枚举
枚举是一种特殊的数据类型,它既是一种类却又比类多了些特殊的约束,但这些约束也造就了枚举简洁性、安全性以及便捷性。
枚举通常用来表示颜色、方式、类别、状态等等数目有限、形式离散、表达又极为明确的量。
//1.Java中是单继承,枚举已经隐含集成java.lang.Enum;但是可以实现接口
public enum Sex {
MAN(1),WOMAN(2);
private int value;
//2.枚举的构造函数,不能用public修饰
Sex() {
}
Sex(int value) {
this.value=value;
}
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
//成员方法
public void test(){}
}
public class User {
private String name;
private Sex sex;
}
Sex.WOMAN.getValue()
获取枚举的值