Vue3-组件使用–路由(第二篇)
一、组件的使用
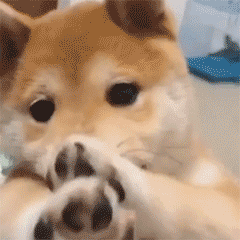
1.引用组件
1.新建一个组件命名为Header.vue
Header.vue
<template>
<h1>这是头部组件</h1>
</template>
2.在App.vue中引用
运行一下
2、将父组件的内容传递给子组件
传递变量
3.将子组件的内容传递父组件
二、声明周期函数
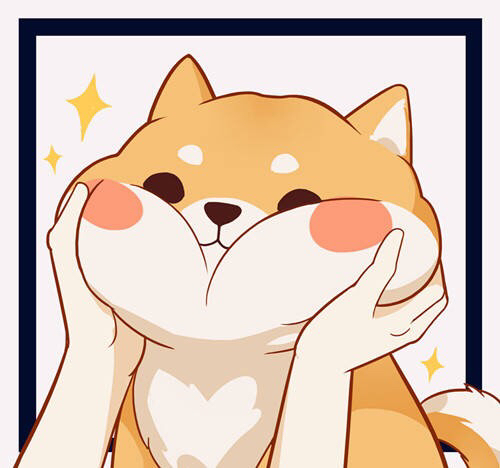
<template>
<div class="content">
</div>
</template>
<script>
//声明式
export default {
name: 'App',
data(){
return{
}
}
,
beforeCreate(){
console.log("初始化数据之前")
},
created(){
console.log("数据初始化之后")
},
beforeMount(){
console.log("挂载渲染之前")
},
mounted(){
console.log("挂载渲染之后")
},
beforeUpdate(){
console.log("更新之前")
},
updated(){
console.log("更新之后")
},
beforeUnmount(){
console.log("销毁之前")
},
unmount(){
console.log("销毁之后")
}
}
</script>
三、合成API
1.合成API初体验
setup()
这是使用composition-Api的入口;
可以接受两个参数:setup(props, context)
props:接受父组件传来的参数;
context:(执行上下文)
使用ref声明函数,才是可变的
使用函数必须从setup里return出去
<template>
<div class="content">
<h1 @click="changeNum">{{num}}</h1>
<h2>姓名:{{user.username}}</h2>
<h2>年龄:{{user.age}}</h2>
<h1>{{username}}</h1>
<h3>{{reverseType}}</h3>
</div>
</template>
<script>
//声明式
import {ref,reactive,toRefs,computed,watch,watchEffect} from 'vue'
//导入ref reacive toRef函数
export default {
name: 'App',
setup(){
//数值
//使一个值或者字符串变成响应式
const num = ref(0);
//对象
//使一个对象变成响应式
const user = reactive({
username : "可可",
age:18,
type:"爱动",
reverseType:computed(()=>{
return user.type.split('').reverse().join('')
})
})
//方法
function changeNum(){
num.value += 10;
}
function changeType(){
user.type = "超级爱动"
}
watchEffect(()=>{
//会判断里面的内容要监听的函数
console.log(user.type)
console.log("user.type改变")
})
watch([num,user],(newnum,prevNum)=>{
console.log(newnum,prevNum);
console.log("当num改变的时候,会触发执行函数")
})
//将变量方法返回
return {num,changeNum,user,...toRefs(user),changeType}
}
</script>
2.合成api详解
<template>
<div class="content">
<user :username="username" :age="age"/>
</div>
</template>
<script>
//声明式
import user from './components/user.vue'
import {ref,reactive,toRefs,computed,watch,watchEffect} from 'vue'
//导入ref reacive toRef函数
export default {
name: 'App',
components:{
user
},
setup(){
//数值
//使一个值或者字符串变成响应式
const num = ref(0);
//对象
//使一个对象变成响应式
const user = reactive({
username : "可可",
age:18,
type:"爱动",
reverseType:computed(()=>{
return user.type.split('').reverse().join('')
})
})
//方法
function changeNum(){
num.value += 10;
}
function changeType(){
user.type = "超级爱动"
}
watchEffect(()=>{
//会判断里面的内容要监听的函数
console.log(user.type)
console.log("user.type改变")
})
watch([num,user],(newnum,prevNum)=>{
console.log(newnum,prevNum);
console.log("当num改变的时候,会触发执行函数")
})
//将变量方法返回
return {num,changeNum,user,...toRefs(user),changeType}
}
</script>
user.vue
<template>
<div>
<!-- 从父组件传递给子组件参数 -->
<h1>username:{{username}}</h1>
<h1>age:{{age}}</h1>
<h3>{{description}}</h3>
</div>
</template>
<script>
import {ref,reactive,toRefs,computed,watch,watchEffect} from 'vue'
export default {
props:['age','username'],
//content可以获取从父元素传递过来的数据比如class属性值
setup(props,content){
console.log(props);
//由于setup执行在前面,this指针还未加载,所以在这不能用this传值
const description = ref(props.username+"年龄是"+props.age);
return {
description
}
}
}
</script>
3.setup中使用生命周期函数
user.vue
<template>
<div>
<!-- 从父组件传递给子组件参数 -->
<h1>username:{{username}}</h1>
<h1>age:{{age}}</h1>
<h3>{{description}}</h3>
</div>
</template>
<script>
import {ref,reactive,toRefs,computed,watch,watchEffect,onBeforeMount,onMounted,onBeforeUpdate,onBeforeUnmount,onUnmounted} from 'vue'
export default {
props:['age','username'],
//content可以获取从父元素传递过来的数据比如class属性值
setup(props,content){
console.log(props);
//由于setup执行在前面,this指针还未加载,所以在这不能用this传值
const description = ref(props.username+"年龄是"+props.age);
//可以多次触发,如果需要生命周期就把它们导入进来,前面多加一个on
onBeforeMount(()=>{
console.log("onBeforeMount")
})
onBeforeMount(()=>{
console.log("onBeforeMount")
})
return {
description
}
}
}
</script>
4、利用provide从父组件传值给子组件
<template>
<div class="content">
<student/>
</div>
</template>
<script>
//声明式
import student from './components/student.vue'
import {ref,reactive,toRefs,computed,watch,watchEffect,provide} from 'vue'
//导入ref reacive toRef函数
export default {
name: 'App',
components:{
student
},
setup(){
const student = reactive({
name:"小可",
classname:"三年二班"
})
//提供的名称,提供的内容
provide('student',student);
return {}
}
}
</script>
student.vue
<template>
<div>
<h1>学生:{{name}}</h1>
<h1>年龄:{{classname}}</h1>
</div>
</template>
<script>
import {ref,reactive,toRefs,computed,watch,watchEffect,provide,inject} from 'vue'
//获取提供的内容,需要注入函数inject
export default {
setup(props,content){
const student = inject('student')
return{
...student
}
}
}
</script>
四、路由
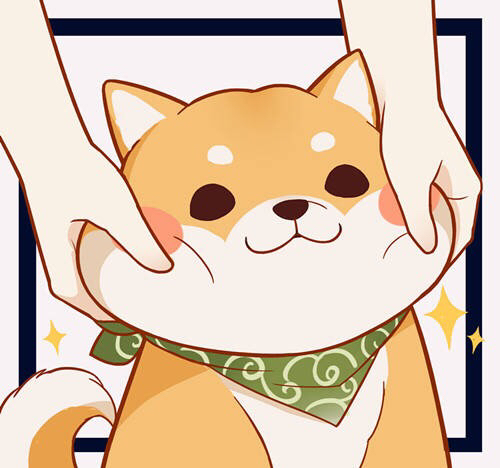
1.路由的基本概念
路由中有三个基本的概念route,routes,router.
// route 它是一条路由,就是一个路径和组件的映射关
{
path:'/',
component: Home
}
//routes
是一组路由,把每条route的路由组合起来,形成一个数组。
routes:[
{
path:'/',
component: Home
},
{
path:'/list',
component: List
}
]
/*router 是一个路由机制,相当于一个管理者,
他来管理路由。因为routes只是定义了一组路由,
也就是一组路径和组件的对应关系,当用户点击了按
钮,改变一个路径,router会更加路径处理不同组件
*/
var router = new VueRouter({
// 配置路由
routes:[...]
})
2.安装路由
终端中输入
npm install vue-router@next
router 下的index.js
// 1. 定义路由组件.
// 也可以从其他文件导入
const Home = { template: '<div>Home</div>' }
const About = { template: '<div>About</div>' }
// 2. 定义一些路由
// 每个路由都需要映射到一个组件。
// 我们后面再讨论嵌套路由。
const routes = [
{ path: '/', component: Home },
{ path: '/about', component: About },
]
// 3. 创建路由实例并传递 `routes` 配置
// 你可以在这里输入更多的配置,但我们在这里
// 暂时保持简单
const router = VueRouter.createRouter({
// 4. 内部提供了 history 模式的实现。为了简单起见,我们在这里使用 hash 模式。
history: VueRouter.createWebHashHistory(),
routes, // `routes: routes` 的缩写
})
main.js
运行一下
npm run dev
router-link组件说明:
/*
router-link是vue-router已经注册好的组件,直接使用就可以了
router-link是用来切换路由的,通过to属性来跳转到指定的路由
router-link在编译的时候会自动被编译为a标签,可以使用tag属性指定编译为你要的标签
*/
router-view组件说明:
/*
router-view用来指定当前路由所映射的组件显示的位置
router-view可以理解为占位符,为路由映射的组件占位,不同路由映射的组件通过替换显示
和动态组件的效果类似
*/
3.动态路由和404NotFound
引入:不同用户登录一个界面, 我们会发现网址中有一部分相同,但是涉及到个人id值却是不同的。这就表示,它是一个组件,假设是user组件。不同的用户(就是用户的id不同),它都会导航到同一个user 组件中。这样我们在配置路由的时候,就不能写死, 所以就引入了动态路由。
path:'/students/:id',component:students
ps:
访问`/students/101`和`/students/102`的时候都能匹配到同一个路由,走同一个路由处理函数, 咱们就得定义/students/:id的路由
router 下的index.js
import {createRouter,createWebHashHistory} from 'vue-router'
import News from '../src/components/News.vue'
import Notfound from '../src/components/Notfound.vue'
const Home = { template: '<div>Home</div>' }
const About = { template: '<div>About</div>' }
const routes = [
{ path: '/', component: Home },
{ path: '/about', component: About },
{ path:'/News/:id',component:News},
{path:'/:path(.*)',component:Notfound
//设置路径名,组件名
}
]
const router = createRouter({
history: createWebHashHistory(),
routes,
})
export default router;
Notfound.vue
<template>
<div>
<p>404 not found page</p>
</div>
</template>
import {createRouter,createWebHashHistory} from 'vue-router'
import News from '../src/components/News.vue'
import Notfound from '../src/components/Notfound.vue'
const Home = { template: '<div>Home</div>' }
const About = { template: '<div>About</div>' }
const routes = [
{ path: '/', component: Home },
{ path: '/about', component: About },
{ path:'/News/:id',component:News},
{ path:'/News/:id(//d+)',component:News},
{ path:'/News/:id+',component:News},/* +至少有一个·
?有或者没有,不可以重复
*有和没有都可以*/
{ path:'/News/:id*',component:News},
{path:'/:path(.*)',component:Notfound}
]
const router = createRouter({
history: createWebHashHistory(),
routes,
})
export default router;
4.嵌套路由
router 下的index.js
import { createRouter, createWebHashHistory } from "vue-router";
import User from "../src/components/User.vue";
import hengban from "../src/components/hengban.vue";
import shuban from "../src/components/shuban.vue";
const Home = { template: "<div>Home</div>" };
const About = { template: "<div>About</div>" };
const routes = [
{ path: "/", component: Home },
{ path: "/about", component: About },
{ path: "/user",
component: User,
children:[
{
path:'hengban',
component:hengban
},{
path:'shuban',
component:shuban
}
]
}
];
const router = createRouter({
history: createWebHashHistory(),
routes
});
export default router;
利用req.params.id
来获取路由的不同部分的数据
router 下的index.js
import { createRouter, createWebHashHistory } from "vue-router";
import User from "../src/components/User.vue";
import hengban from "../src/components/hengban.vue";
import shuban from "../src/components/shuban.vue";
const Home = { template: "<div>Home</div>" };
const About = { template: "<div>About</div>" };
const routes = [
{ path: "/", component: Home },
{ path: "/about", component: About },
{ path: "/user/:id",
component: User}
];
const router = createRouter({
history: createWebHashHistory(),
routes
});
export default router;
user.vue
<template>
<div>
<h1>User页面</h1>
<h2>{{$route.params.id}}</h2>
<router-view></router-view>
</div>
</template>
5.使用js跳转页面
$router.push()
page.vue
<template>
<div>
<button @click="toindexpage">跳转到首页</button>
</div>
</template>
<script>
export default {
methods:{
toindexpage(){
console.log("跳转到about页面")
this.$router.push({path:'/about'})
//this.$router.push({path:'/mypage/123'})
//携带参数
//this.$router.push({name:"mypage",params:{id:132}})
}
}
}
</script>
router 下的index.js
import { createRouter, createWebHashHistory } from "vue-router";
import User from "../src/components/User.vue";
import page from "../src/components/page.vue";
const Home = { template: "<div>Home</div>" };
const About = { template: "<div>About</div>" };
const routes = [
{ path: "/", component: Home },
{ path: "/about", component: About },
{path:'/page/:id',
name:'mypage',//别名
component:page}
];
const router = createRouter({
history: createWebHashHistory(),
routes
});
export default router;
也可以携带参数跳转
$router.replace()
this.$router.replace({path:'/page',query:{search:"可可"}})
$router.go(-1)返回上一个页面
$router.go(-1)
$router.go(1)前进到下一个页面
$router.go(1)
代码
<template>
<div>
<button @click="toindexpage">跳转到首页</button>
<button @click="replacePage">替换页面</button>
<button @click="$router.go(1)">前进</button>
<button @click="$router.go(-1)">后退</button>
</div>
</template>
<script>
export default {
methods:{
toindexpage(){
console.log("跳转到about页面")
this.$router.push({path:'/mypage'})
},
replacePage(){
console.log("替换页面")
//回不到刚才的页面
this.$router.replace({path:'/page',query:{search:"可可"}})
}
}
}
</script>
6.命名视图
一个页面中可以·设置多个组件进行渲染
import { createRouter, createWebHashHistory } from "vue-router";
import shop from '../src/components/shop.vue'
import shopfoot from '../src/components/shopfoot.vue'
import shopTop from '../src/components/shopTop.vue'
const Home = { template: "<div>Home</div>" };
const About = { template: "<div>About</div>" };
const routes = [
{ path: "/", component: Home },
{ path: "/about", component: About },
{path:'/shop',
components:{
default:shop,//默认值页面是shop
shopfoot:shopfoot,
shopTop:shopTop
}
}
];
const router = createRouter({
history: createWebHashHistory(),
routes
});
export default router;
<template>
<div class="content">
<router-view></router-view>
<router-view name="shopTop"></router-view>
<router-view name="shopfoot"></router-view>
<!-- 如果遇见名字相同的,会把内容更新到路由上 -->
</div>
</template>
<script>
export default {
name: "App",
components: {},
setup() {
return {};
}
};
</script>
7.重定向和别名
{path:'/mall',
redirect:(to)=>{return{path:'/shop'}}
}//重定向跳转到shop页面
//别名,取别名地址栏中的名字不会改成shop,依旧是cocoshop,可以取多个别名
alias:"/cocoshop",
8.导航守卫
如果有权限那么可以实现跳转页面
router.beforeEach((to,from)=>{
return false;//返回为false没有权限,页面无显示
})
router.beforeEach((to,from,next)=>{
console.log(to)
next();//如果想要继续访问使用next()调用起来
})
import { createRouter, createWebHashHistory } from "vue-router";
import shop from '../src/components/shop.vue'
import shopfoot from '../src/components/shopfoot.vue'
import shopTop from '../src/components/shopTop.vue'
import mypage from '../src/components/mypage.vue'
const Home = { template: "<div>Home</div>" };
const About = { template: "<div>About</div>" };
const routes = [
{ path: "/", component: Home },
{ path: "/about", component: About },
{path:'/shop',
//别名,取别名地址栏中的名字不会改成shop,依旧是cocoshop,可以取多个别名
alias:"/cocoshop",
components:{
default:shop,//默认值页面是shop
shopfoot:shopfoot,
shopTop:shopTop
},
//具体的进入某一个组件页面
beforeEnter:(to,from)=>{
console.log("beforeEnter")
//只会进入page页面才会产生经常会用到
}
},
// {path:'/mall',
// redirect:(to)=>{return{path:'/shop'}}
// },
{path:'/mypage',
component:mypage
}
];
const router = createRouter({
history: createWebHashHistory(),
routes
});
//跳转之前执行
router.beforeEach((to,from,next)=>{
console.log(to)
next();
//如果return false)有没有权利跳转到某一个页面,取消跳转
//安全导航
})
export default router;
使用选项API
beforeRouteEnter(){
console.log("路由进入")
},
beforeRouteUpdate(){
console.log("路由更新组件")
},
beforeRouteLeave(){
console.log("路由离开组件")
}
<template>
<div>
<h1>购物商城</h1>
<button @click="topage"></button>
</div>
</template>
<script>
export default {
methods:{
topage:function(){
this.$router.push({path:'/mypage'})
}
},
beforeRouteEnter(){
console.log("路由进入")
},
beforeRouteUpdate(){
console.log("路由更新组件")
},
beforeRouteLeave(){
console.log("路由离开组件")
}
}
</script>
9.路由模式
路由的实现有两种方案,一种是通过hash值,一种是通过H5 的history.但是Vue路由默认使用hash模式.
//hash路由中#看起来多多少少有点别扭.我们就像使用//history模式,那要怎么设置呢
VueRouter配置项中处理提供一个routes用来配置路由映射表,还提供了一个mode选项用来配置路由模式
import { createRouter, createWebHashHistory } from "vue-router";
const router = createRouter({
history: createWebHashHistory(),
routes
});
import { createRouter, createWebHistory } from 'vue-router'
const router = createRouter({
history: createWebHistory(process.env.BASE_URL),
routes
})