HTML代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>聊天机器人</title>
<link rel="stylesheet" type="text/css" href="css1.css"/>
<script src="https://cdn.staticfile.org/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(function(){
$('.sendButton').click(function(){
//生成人的话
var $f_r = $('<div class="self clearfix" ><a href="#" class="f_r">我</a><p class="f_r"></p></div>');
$('.message').append($f_r);
$('p.f_r:last').text($('.inputBox').val());
$('.inputBox').val('');
//1.创建异步对象
var xhr=new XMLHttpRequest;
//2.设置请求行 (get请求数据写在url后面)
xhr.open('POST','chat.php');
//3.设置请求头(get请求可以忽略,post请求不发送数据可以省略)
//xhr.setRequestHeader('Content-Type','application/x-www-form-urlencoded');
//4.请求主体发送(参数为空或者写NULL,post请求如果有数据提交就写在这里,格式'key=value&key2=value2')
xhr.send(null);
//5.回调函数处理
xhr.onreadystatechange=function()
{
if(xhr.readyState==4&&xhr.status==200)
{
//生成机器人话
var $f_l=$('<div class="robot clearfix"><a href="#" class="f_l">小爱</a><p class="f_l">你好,很高兴认识你!</p></div>');
$('.message').append($f_l);
$('p.f_l:last').text(xhr.responseText);
var sh = $(".message").prop("scrollHeight");
$('.message').animate({scrollTop:sh},600);
}
}
});
$(document).keydown(function(e){
if(e.keyCode==13){
$('.sendButton').trigger('click')
}
})
});
</script>
</head>
<body>
<h2 style="text-align:center">在线机器人</h2>
<div class="container">
<div class="message">
<div class="self clearfix" >
<a href="#" class="f_r">我</a>
<p class="f_r">你好!小爱同学</p>
</div>
<div class="robot clearfix">
<a href="#" class="f_l">小爱</a>
<p class="f_l">你好,很高兴认识你!</p>
</div>
</div>
<div class="control">
<input type="text" class='inputBox f_l'>
<input type="button" class='sendButton f_r' value='发 送'>
</div>
</div>
</body>
</html>
css代码:
@charset "utf-8";
/* CSS Document */
<style>
body {
margin: 0;
padding: 20px;
}
.clearfix::before,
.clearfix::after {
content: '';
visibility: hidden;
clear: both;
line-height: 0;
height: 0;
display: block;
}
.clearfix {
zoom: 1;
}
.f_l {
float: left;
}
.f_r {
float: right;
}
.container {
margin: 50px auto 0;
width: 800px;
height: 500px;
border: 1px solid skyblue;
border-radius: 10px;
}
.message {
border-bottom: 1px solid skyblue;
height: 400px;
overflow-y: scroll;
padding: 20px;
box-sizing: border-box;
transition: all 2s;
}
.control {
height: 100px;
padding-left: 50px;
padding-right: 50px;
}
.inputBox {
height: 60px;
margin-top: 20px;
border-radius: 6px;
outline: none;
padding: 0;
box-sizing: border-box;
width: 500px;
font-size: 30px;
box-shadow: 0 0 3px gray;
border: 1px solid #ccc;
transition: all .2s;
padding-left: 10px;
}
.inputBox:focus {
border-color: skyblue;
box-shadow: 0 0 3px skyblue;
}
.sendButton {
height: 70px;
margin-top: 15px;
background-color: yellowgreen;
width: 150px;
border: none;
outline: none;
border-radius: 10px;
color: white;
font-size: 40px;
font-family: '微软雅黑';
cursor: pointer;
}
.message>div>a {
text-decoration: none;
width: 50px;
height: 50px;
border-radius: 50%;
background-color: skyblue;
text-align: center;
line-height: 50px;
color: white;
font-size: 25px;
font-weight: 700;
font-family: '微软雅黑';
}
.message>.robot>a {
background-color: hotpink;
}
.message>.self>a {
background-color: yellowgreen;
}
.message>div {
padding: 10px 0;
}
.message>div>p {
max-width: 300px;
min-height: 28px;
margin: 0 10px;
padding: 10px 10px;
background-color: #ccc;
border-radius: 10px;
word-break: break-all;
position: relative;
line-height: 28px;
font-weight: 400;
font-family: '微软雅黑';
color: white;
font-size: 20px;
}
.message>.robot>p {
background-color: skyblue;
}
.message>.robot>p::before {
content: '';
position: absolute;
border-top: 6px solid transparent;
border-bottom: 6px solid transparent;
border-right: 6px solid skyblue;
left: -5px;
top: 15px;
}
.message>.self>p {
color: black;
}
.message>.self>p::before {
content: '';
position: absolute;
border-top: 6px solid transparent;
border-bottom: 6px solid transparent;
border-left: 6px solid #ccc;
right: -6px;
top: 15px;
}
</style>
PHP文件代码:
<?php
// 休息一会
sleep(1);
// 获取 用户发送的 消息 (可选)
// 后端 对于 用户发过来的 时候 是否 使用 (可选)
// 根据 发送 过来的 消息 返回 不同的内容
$messageList =array(
'床前明月光,有饼没包装。',
'举头望明月,低头闻饼香',
'细草微风岸,今年送礼多。',
'何物能摆阔?月饼最出色!',
'无权鸟飞绝,没钱人踪灭。',
'品饼衰笠翁,独过中秋节。',
'明月几时有,举饼问青天。',
'不知天上宫阙,月饼卖多钱?!',
'悄地我收了,正如你悄悄地送,',
'我挥一挥衣袖,来年你还送不送?',
'我有一块月饼,不知与谁能共?',
'多少秘密在其中,送饼之人能懂。'
);
// 从 数组中 每次 随机 取出
// array_rand 返回的 是一个 随机的 下标
$randomKey = array_rand($messageList,1);
// 使用 随机 下标 返回 随机的 值
echo $messageList[$randomKey];
?>
效果图:
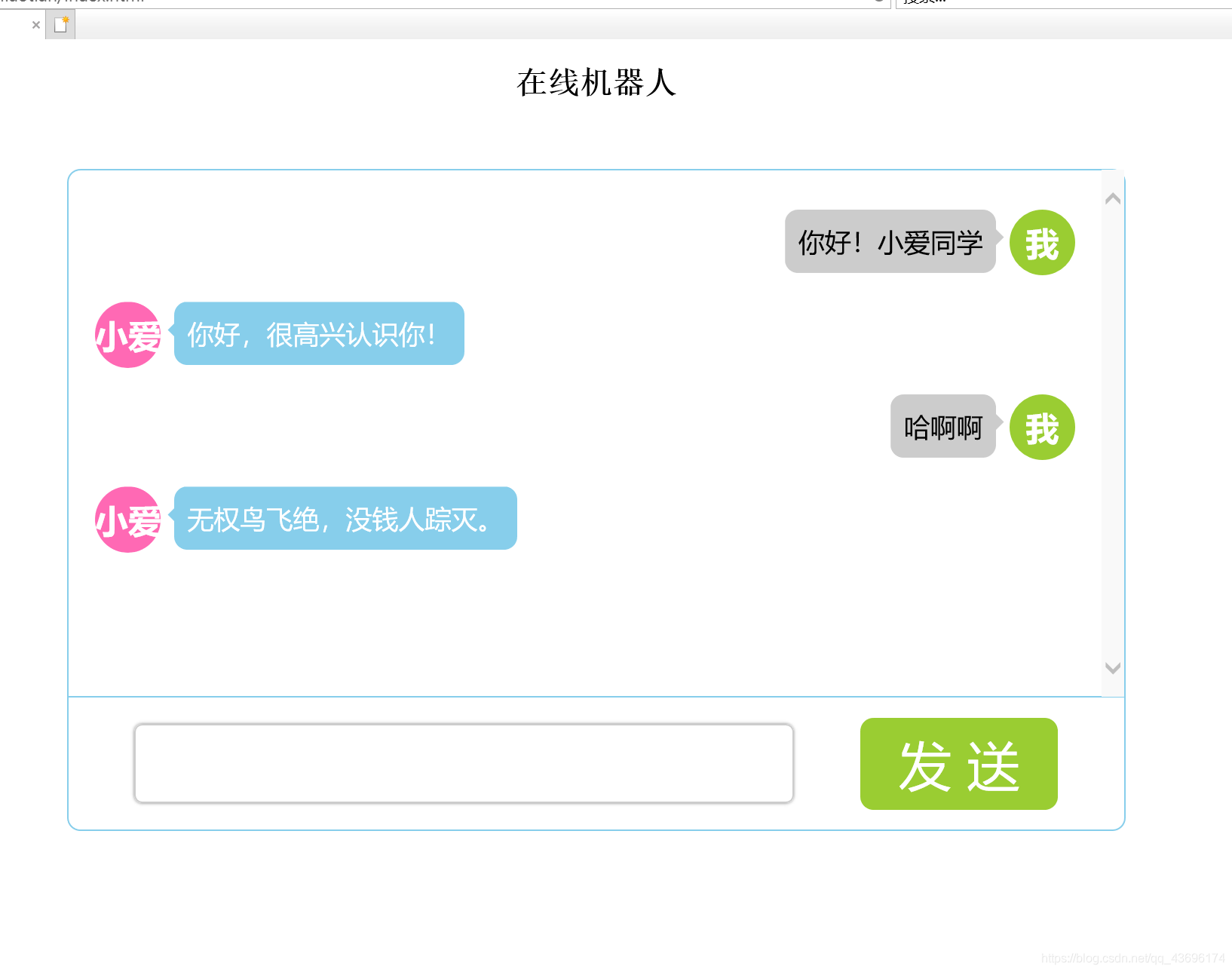