1.输入一个整数,输出该数的位数
#include <stdio.h>
void main()
{ int n,k=0;
scanf("%d",&n);
while( _____1_____ )
{
k++;
_____2_____;
}
printf("%d\n",k);
}
2.

#include <stdio.h>
#include <math.h>
#define f(x) x*x-5*x+sin(x)
void main()
{ int x; float max;
______1______
for(x=2;x<=10;x++)
______2______
printf("%f\n",max);
}
3

#include <stdio.h>
void main()
{
char c;
c=getchar();
if(______1______)
c=c+5;
else
if (c>='v' && c<='z')
______2______
putchar(c);
}
4.

#include <stdio.h>
void Dec2Bin(int m)
{
int bin[32],j;
for(j=0;m!=0;j++)
{
bin[j]= ______1______;
m=m/2;
}
for(;j!=0;j--)
printf("%d", ______2______ );
void main()
{
int n;
scanf("%d",&n);
Dec2Bin(n);
}
5.

#include <stdio.h>
#include <math.h>
void main()
{
int n,s=0;
scanf("%d",&n);
______ 1 ______
while(n!=0) {
______ 2 ______
n=n/10;
}
printf("%d\n",s);
}
6

/#include <stdio.h>
void swap(______1______)
{
int temp;
temp = *pa;
*pa = *pb;
*pb = temp;
}
void main()
{
int a,b,c,temp;
scanf("%d%d%d",&a,&b,&c);
if(a>b)
swap(&a,&b);
if(b>c)
swap(&b,&c);
if(______2______)
swap(&a,&b);
printf("%d,%d,%d",a,b,c);
}
7.

#include <stdio.h>
_______1______
void main()
{
printf("%ld\n",f(30));
}
long f(int n)
{
if( ______2______ )
return 1;
else
return f(n-1)+f(n-2);
}
8

#include <stdio.h>
void main()
{
char s[80];
int i,j;
gets(s);
for(i=j=0;______1______;i++)
if(s[i] != 'c')
{
s[j]=s[i];
______2______
}
s[j]='\0';
puts(s);
}
9

#include <stdio.h>
struct STUDENT
{
char name[16];
int math;
int english;
int computer;
int average;
};
void GetAverage(struct STUDENT *pst)
{
int sum=0;
sum = ______1______;
pst->average = sum/3;
}
void main()
{
int i;
struct STUDENT st[4]={{"Jessica",98,95,90},{"Mike",80,80,90},
{"Linda",87,76,70},{"Peter",90,100,99}};
for(i=0;i<4;i++)
{
GetAverage(______2______);
}
printf("Name\tMath\tEnglish\tCompu\tAverage\n");
for(i=0;i<4;i++)
{
printf("%s\t%d\t%d\t%d\t%d\n",st[i].name,st[i].math,st[i].english,
st[i].computer,st[i].average);
} }
10
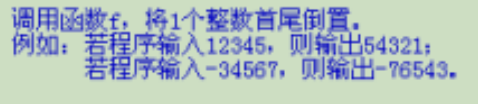
#include <stdio.h>
#include <math.h>
long f(long n)
{ long m,y=0; m=fabs(n);
while(m!=0) {
y=y*10+m%10;
____1____
}
if(n>=0) return y;
else _____2_____
}
void main()
{
printf("%ld\t",f(12345)); printf("%ld\n",f(-34567));
}
11

#include <stdio.h>
float f(float,float*,int);
void main()
{ float b[5]={1.1,2.2,3.3,4.4,5.5};
printf("%f\n",f(1.7,b,5));
}
float f( _______1________ )
{ float y=a[0],t=1; int i;
for(i=1;i<n;i++) { t=t*x; y=y+a[i]*t; }
_____2_____
}
12

#include <stdio.h>
#include <string.h>
#include <ctype.h>
void f(char *s)
{ int i=0;
while(s[i]!='\0'){
if(isdigit(s[i])) ____1____(s+i,s+i+1);
___2___ i++;}
}
void main()
{ char str[80];
gets(str); f(str); puts(str);
}
13

#include <stdio.h>
#include <ctype.h>
void main()
{ char a[80]; int n[3]={0},i; gets(a);
________1________
{if (tolower(a[i])>='a' && tolower(a[i])<='z')
n[0]++;
else if (________2________)
n[1]++;
else
n[2]++;
}
for(i=0;i<3;i++) printf("%d\n",n[i]);
}
14
#include <stdio.h>
#include<math.h>
void main()
{
int i,a,b,c;
for(i=100;i<=999;i++)
{
a=i/100;
_______1_______
c=i%10;
if (________2________)
printf("%d is a Armstrong number!\n",i);
} }
15

#include <stdio.h>
void main()
{
int a[10],b[10],i;
printf("\nInput 10 numbers: ");
for (i=0; i<10;i++)
scanf("%d", &a[i]);
for (i=1; i<10; i++)
b[i]=______1______;
for (i=1; i<10; i++)
{
printf("%3d",b[i]);
if (______2______) printf("\n");
} }