目录
一、软件的结构
C/S (Client - Server 客户端-服务器端)
典型应用:QQ软件 ,飞秋,印象笔记。
特点:
1)必须下载特定的客户端程序。
2)服务器端升级,客户端升级。
B/S (Broswer -Server 浏览器端- 服务器端)
典型应用: 腾讯官方(www.qq.com) 163新闻网站(俗称:网站)
特点:
1)不需要安装特定的客户端(只需要安装浏览器即可!!)
2)服务器端升级,浏览器不需要升级!!!!
javaweb的程序就是b/s软件结构!!!
1.服务器
从物理上来说,服务器就是一台PC机器。8核,8G以上,T来计算,带宽100M
web服务器:PC机器安装一个具有web服务的软件,称之为web服务器
数据库服务器:PC机器安装一个具有数据管理件服务的软件,称之为数据库服务器。
邮件服务器:PC机器安装一个具有发送邮件服务的软件,称之为邮件服务器。
2.web 服务软件
常见的市面上 web 服务软件
WebLogic: BEA 公司的产品。 收费的。支持 JavaEE规范。
WebSphere : IBM公司的产品。收费的。支持 JavaEE 规范
JBoss: Redhat 公司的产品。收费的。支持 JavaEE 规范
Tomcat : 开源组织 Apache 的产品。免费的。支持部分的 JavaEE 规范。(servlet 、 jsp。 jdbc ,但 ejb , rmi不支持)
Web服务器作用:把服务器端的资源共享给外部访问。
B/S工作结构图:
使用JSP页面来代替Html页面展示数据,Html是写死的数据,在Html页面中没法写逻辑控制语句,
而JSP页面中即可以展示Html标签又可以写逻辑控制
二、Servlet:
静态网页:
1、无论谁看内容都是一样的。
2、百度百科、新闻、login.html登陆界面。
3、服务器直接保存html,直接返回html即可。
动态网页:
1、不同的人看到的内容是有差异的。
2、微博、登陆思途管理系统看到的内容。
3、服务器保存一个组件,动态的给每个用户拼接一个网页。
4、这个组件就是servlet。
什么是Servlet:
就是不同的用户拼接动态网页的组件。
Servlet运行在服务端的Java小程序,是sun公司提供的一套规范(接口),用来处理客户端的请求、响应动态资源给浏览器的。
JSP本质是Servlet
实现步骤:
1、创建类继承HttpServlet
2、覆盖未实现的方法--service方法
package com.servlet;
import com.entity.Banji;
import com.entity.Student;
import com.util.JDBCUtil;
import com.util.Pageinfo;
import com.vo.StudentBanji;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
// url-pattern
// http://localhost:8080/JavaWeb/selectAll
@WebServlet("/student")
public class StudentServlet extends HttpServlet {
@Override
//service 服务器
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// 解决post请求乱码问题
req.setCharacterEncoding("UTF-8");
// 把所有和学生相关的增删改查放到一个Servlet
// http://localhost:8080/JavaWeb/student?method=selectAll
// http://localhost:8080/JavaWeb/student?method=deleteById&id=2
// http://localhost:8080/JavaWeb/student?method=insert
String method = req.getParameter("method");
if (method == null || method.equals("")){
method = "selectPage";
}
switch (method){
// case "selectALL":
// //查询表的全部数据并显示出来
// selectALL(req,resp);
// break;
case "selectPage":
selectPage(req,resp);
break;
case "deleteById":
//通过id删除数据库的数据
deleteById(req,resp);
break;
case "insert":
//增加数据
insert(req,resp);
break;
case "selectById":
//修改一行数据首先通过id查询出来并显示出来
//然后通过修改上传后台保存到数据库中
//最后重定向到查询表
selectById(req,resp);
break;
case "update":
//将修改的数据提交后台
update(req,resp);
break;
case "getStudentInsertPage":
getStudentInsertPage(req, resp);
break;
}
}
private void getStudentInsertPage(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("StudentServlet.getStudentInsertPage");
Connection connection = null;
PreparedStatement statement = null;
ResultSet resultSet = null;
List<Banji> list = new ArrayList<>();
try {
connection = JDBCUtil.getConnection();//建立连接
String sql = "select id,name from banji";
statement = connection.prepareStatement(sql);
System.out.println(statement);
resultSet = statement.executeQuery();//将查询的结果数据进行存储
while (resultSet.next()) {//resultSet不能自己滚动,所以只能用next一条条遍历
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
Banji banji = new Banji(id, name);
list.add(banji);//加入到ArrayLIst中
}
for (Banji banji : list) {
System.out.println(banji);
}
} catch (SQLException throwables) {
throwables.printStackTrace();
}
req.setAttribute("banjiList", list);
req.getRequestDispatcher("/student_insert.jsp").forward(req, resp);
}
private void selectPage(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("StudentServlet.selectPage");
String pageNostr = req.getParameter("PageNo");//网址上的固定为string类型
if (pageNostr == null || pageNostr.equals("")){
pageNostr = "1";
}
String pageSizestr = req.getParameter("PageSize");
if (pageSizestr == null || pageSizestr.equals("")){
pageSizestr = "5";
}
int PageNo = Integer.parseInt(pageNostr);
int PageSize = Integer.parseInt(pageSizestr);
//select id,name,age from teacher limit offset,PageSIze
//offset从第几行开始往下数,PageSIze是每一页的行数,
// 每一页数PageSIze行就是输出一页
int offset = (PageNo - 1 ) * PageSize;
//获得所有数据组成的集合
ArrayList<StudentBanji> list = getCurrentPageList(offset,PageSize);
//得到所有数据的数量
int totalCount =getTotalCount();
//计算医用能分成多少页
int totalPage = (int)Math.ceil((double) totalCount/PageSize);
//构建分页对象,参数为数组集合,页号,总页数,每页大小
Pageinfo pageinfo = new Pageinfo(list,PageNo,totalPage,PageSize);
//将pageinfo加入到内存中
req.setAttribute("pageinfo",pageinfo);
// 跳转到student_list.jsp展示数据
// Dispatcher:分发 forward:转发
//转发到student_list.jsp
req.getRequestDispatcher("/student_list.jsp").forward(req,resp);
}
private int getTotalCount() {
Connection connection = null;
PreparedStatement statement = null;
ResultSet resultSet = null;
int totalCount = 0;
try {
connection = JDBCUtil.getConnection();
String sql = "select count(*) from student0";
statement = connection.prepareStatement(sql);
System.out.println(statement);
resultSet = statement.executeQuery();
if (resultSet.next()) {
totalCount = resultSet.getInt(1);
}
} catch (SQLException throwables) {
throwables.printStackTrace();
}
return totalCount;
}
private ArrayList<StudentBanji> getCurrentPageList(int offset, int PageSize) {
Connection connection = null;
PreparedStatement statement = null;
ResultSet resultSet = null;
ArrayList<StudentBanji> list = new ArrayList<>();/*new ArrayList后<>可加可不加*/
try {
connection = JDBCUtil.getConnection();
String sql = "SELECT s.id AS studentId,s.name AS studentName,s.age AS studentAge,s.gender AS studentGender,b.name AS studentBanji\n" +
"FROM student0 AS s INNER JOIN banji AS b\n" +
"ON s.banji_id=b.id LIMIT ?,?";
statement = connection.prepareStatement(sql);
statement.setInt(1,offset);
statement.setInt(2,PageSize);
resultSet = statement.executeQuery();
System.out.println(statement);
while (resultSet.next()) {
int studentId = resultSet.getInt("studentId");
String studentName = resultSet.getString("studentName");
int studentAge = resultSet.getInt("studentAge");
String studentGender = resultSet.getString("studentGender");
String studentBanji = resultSet.getString("studentBanji");
StudentBanji studentbanji = new StudentBanji(studentId, studentName, studentAge, studentGender,studentBanji);
list.add(studentbanji);
}
for (StudentBanji studentbanji : list) {
System.out.println(studentbanji);
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtil.close(connection, statement, resultSet);
}
return list;
}
private void update(HttpServletRequest req, HttpServletResponse resp) throws IOException {
System.out.println("StudentServlet.update");
// 这些也是传递的参数
// id:23
// name: 张三
// age: 23
// gender: 男
String id = req.getParameter("id");
String name = req.getParameter("name");
String age = req.getParameter("age");
String gender = req.getParameter("gender");
String banji = req.getParameter("banji");
Connection connection = null;/*创建连接对象*/
PreparedStatement statement = null;/*创建船*/
try {
connection = JDBCUtil.getConnection();/*建立连接*/
String sql = "update student0 set name=?,age=?,gender=? ,banji_id=? where id=?";/*写sql语句*/
statement = connection.prepareStatement(sql);/*将语句放入connetion的statement船中*/
statement.setString(1,name);/*给sql中的?将进行赋值*/
statement.setInt(2,Integer.parseInt(age));
statement.setString(3,gender);
statement.setString(4,banji);
statement.setInt(5,Integer.parseInt(id));
System.out.println(statement);/*delete from student0 where id=5*/
int count = statement.executeUpdate();/*修改了几行*/
System.out.println("count :"+count);
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally {
JDBCUtil.close(connection,statement,null);/*关闭连接*/
}
// 删除完成之后再去查找所有,展示最新的数据
// 重定向 :让浏览器发送这个请求 /JavaWeb/selectAll
resp.sendRedirect(req.getContextPath() + "/student?method=selectPage");
}
private void selectById(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("StudentServlet.selectById");
String id =req.getParameter("id");
Connection connection = null;
PreparedStatement statement = null;
ResultSet resultSet = null;
ArrayList<Student> list = new ArrayList<>();/*new ArrayList后<>可加可不加*/
Student student=null;
try {
connection = JDBCUtil.getConnection();
String sql = "select id,name,age,gender from student0 where id=?";
statement = connection.prepareStatement(sql);
statement.setInt(1,Integer.parseInt(id));
resultSet = statement.executeQuery();
System.out.println(statement);
while (resultSet.next()) {
String name = resultSet.getString("name");
int age = resultSet.getInt("age");
String gender = resultSet.getString("gender");
student = new Student(Integer.parseInt(id), name, age, gender);
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtil.close(connection, statement, resultSet);
}
// 把list数据放到一块内存区域里面
req.setAttribute("student", student);
// 跳转到student_list.jsp展示数据
// Dispatcher:分发 forward:转发
req.getRequestDispatcher("/student_update.jsp").forward(req,resp);
}
private void insert(HttpServletRequest req, HttpServletResponse resp) throws IOException {
System.out.println("StudentServlet.insert");
String name = req.getParameter("name");
String age = req.getParameter("age");
String gender = req.getParameter("gender");
String banji = req.getParameter("banji");
Connection connection = null;/*创建连接对象*/
PreparedStatement statement = null;/*创建船*/
try {
connection = JDBCUtil.getConnection();/*建立连接*/
String sql = "insert into student0(name,age,gender,banji_id) values(?,?,?,?)";/*写sql语句*/
statement = connection.prepareStatement(sql);/*将语句放入connetion的statement船中*/
statement.setString(1,name);/*给sql中的?将进行赋值*/
statement.setInt(2,Integer.parseInt(age));
statement.setString(3,gender);
statement.setInt(4,Integer.parseInt(banji));
System.out.println(statement);/*delete from student0 where id=5*/
int count = statement.executeUpdate();/*修改了几行*/
System.out.println("count :"+count);
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally {
JDBCUtil.close(connection,statement,null);/*关闭连接*/
}
// 删除完成之后再去查找所有,展示最新的数据
// 重定向 :让浏览器发送这个请求 /JavaWeb/selectAll
resp.sendRedirect(req.getContextPath() + "/student?method=selectPage");
}
private void deleteById(HttpServletRequest req, HttpServletResponse resp) throws IOException {
System.out.println("StudentServlet.deleteById");
// http://localhost:8080/JavaWeb/deleteById?id=1
/*收到请求req网址的id ,然后根据id删除响应数据库数据*/
String id = req.getParameter("id");
Connection connection = null;/*创建连接对象*/
PreparedStatement statement = null;/*创建船*/
try {
connection = JDBCUtil.getConnection();/*建立连接*/
String sql = "delete from student0 where id=?";/*写sql语句*/
statement = connection.prepareStatement(sql);/*将语句放入connetion的statement船中*/
statement.setInt(1,Integer.parseInt(id));/*给sql中的?将进行赋值*/
System.out.println(statement);/*delete from student0 where id=5*/
int count = statement.executeUpdate();/*修改了几行*/
System.out.println("count :"+count);
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally {
JDBCUtil.close(connection,statement,null);/*关闭连接*/
}
// 删除完成之后再去查找所有,展示最新的数据
// 重定向 :让浏览器发送这个请求 /JavaWeb/selectAll
resp.sendRedirect(req.getContextPath() + "/student?method=selectPage");
}
// private void selectALL(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// System.out.println("StudentServlet.selectByPage");
// Connection connection = null;
// PreparedStatement statement = null;
// ResultSet resultSet = null;
// ArrayList<StudentBanji> list = new ArrayList<>();/*new ArrayList后<>可加可不加*/
// try {
// connection = JDBCUtil.getConnection();
// String sql = "SELECT s.id AS studentId,s.name AS studentName,s.age AS studentAge,s.gender AS studentGender,b.name AS studentBanji\n" +
// "FROM student0 AS s INNER JOIN banji AS b\n" +
// "ON s.banji_id=b.id";
// statement = connection.prepareStatement(sql);
// resultSet = statement.executeQuery();
// System.out.println(statement);
// while (resultSet.next()) {
// int studentId = resultSet.getInt("studentId");
// String studentName = resultSet.getString("studentName");
// int studentAge = resultSet.getInt("studentAge");
// String studentGender = resultSet.getString("studentGender");
// String studentBanji = resultSet.getString("studentBanji");
// StudentBanji studentbanji = new StudentBanji(studentId, studentName, studentAge, studentGender,studentBanji);
// list.add(studentbanji);
// }
// for (StudentBanji studentbanji : list) {
// System.out.println(studentbanji);
// }
// } catch (SQLException throwables) {
// throwables.printStackTrace();
// } finally {
// JDBCUtil.close(connection, statement, resultSet);
// }
// // 把list数据放到一块内存区域里面
// req.setAttribute("list", list);
// // 跳转到student_list.jsp展示数据
// // Dispatcher:分发 forward:转发
// req.getRequestDispatcher("/student_list.jsp").forward(req,resp);
// }
}
三、JSP
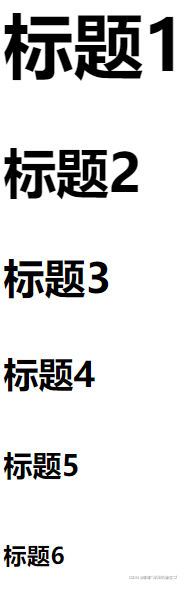
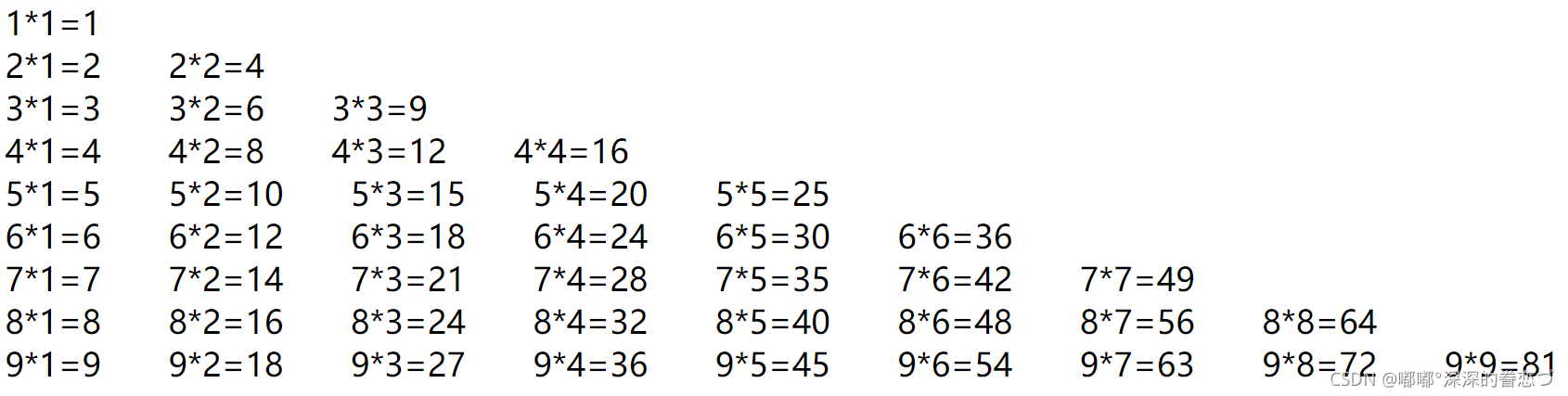
<%@ page import="java.util.ArrayList" %>
<%@ page import="com.entity.Student" %>
<%@ page import="com.vo.StudentBanji" %>
<%@ page import="com.util.Pageinfo" %><%--
Created by IntelliJ IDEA.
User: gaoweijie
Date: 2021/8/10
Time: 19:39
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
<%--关联bootstrap,能够直接使用该框架内容 --%>
<link rel="stylesheet" href="<%=request.getContextPath()%>/static/bootstrap-3.4.1-dist/css/bootstrap.css">
</head>
<body>
<%-- 将写的insert浏览器页面在查询页面设置超链接,能够从该页面访问增加数据页面 --%>
<%--<a class="btn btn-success btn-lg" href="<%=request.getContextPath()%>/student?method=getStudentInsertPage">添加</a>--%>
<%-- ${list}--%><%--能够将list中的数据在网页上进行显示--%>
<a class="btn btn-primary" href="<%=request.getContextPath()%>/student_insert.jsp">添加</a>
<%--视同table创建表格; table table-bordered table-hover table-striped table-condensed 都是bootstrapo中的框架--%>
<table class="table table-bordered table-hover table-striped table-condensed">
<tr class="info">
<td>id</td>
<td>名字</td>
<td>年龄</td>
<td>性别</td>
<td>班级名字</td>
<td>删除/编辑</td>
</tr>
<%-- 表格的标题--%>
<%-- <%%> 符号能够使jsp文件在符号中书写Java代码--%>
<%
Pageinfo pageinfo =(Pageinfo) request.getAttribute("pageinfo");
ArrayList<StudentBanji> list =pageinfo.getList();
// List取自selectall的键值对的存储
for (StudentBanji studentBanji : list) {
%>
<tr>
<td><%=studentBanji.getStudentId()%></td>
<td><%=studentBanji.getStudentName()%></td>
<td><%=studentBanji.getStudentAge()%></td>
<td><%=studentBanji.getStudentGender()%></td>
<td><%=studentBanji.getStudentBanji()%></td>
<td>
<a class="btn btn-danger btn-lg" href="javascript:void(0)" onclick="deleteById(<%=studentBanji.getStudentId()%>)">删除</a>
<a class="btn btn-warning btn-lg" href="<%=request.getContextPath()%>/student?method=selectById&id=<%=studentBanji.getStudentId()%>">编辑</a>
</td>
<%-- <td><a href="<%=request.getContextPath()%>/student?method=deleteById&id=<%=student.getId()%>">删除</a></td>--%>
<%-- ? 后边是参数--%>
<%-- <td><a href="javascript:void(0)" onclick="deleteById(<%=student.getId()%>)">删除</a></td>--%>
</tr>
<%
}
%>
</table>
<nav aria-label="Page navigation">
<ul class="pagination">
<%
if (pageinfo.getPageNo() > 1) {
%>
<li>
<a href="<%=request.getContextPath()%>/student?method=selectPage&PageNo=<%=pageinfo.getPageNo()-1%>" aria-label="Previous">
<span aria-hidden="true">«</span>
</a>
</li>
<%
} else {
%>
<li class="disabled">
<a aria-label="Previous">
<span aria-hidden="true">«</span>
</a>
</li>
<%
}
%>
<%
for (Integer i = 1; i <=pageinfo.getTotalpage() ; i++) {
%>
<li><a href="<%=request.getContextPath()%>/student?method=selectPage&PageNo=<%=i%>&PageSize=5"><%=i%></a></li>
<%
}
%>
<%
if (pageinfo.getPageNo() <pageinfo.getTotalpage()) {
%>
<li>
<a href="<%=request.getContextPath()%>/student?method=selectPage&PageNo=<%=pageinfo.getPageNo()+1%>" aria-label="Previous">
<span aria-hidden="true">»</span>
</a>
</li>
<%
} else {
%>
<li class="disabled">
<a aria-label="Previous">
<span aria-hidden="true">»</span>
</a>
</li>
<%
}
%>
</ul>
</nav>
<%--弹窗,按下删除键后会提示是否确认删除防止误删--%>
<script type="text/javascript">
function deleteById(id){
var isDelete = confirm('您确认要删除么?');
if (isDelete){
location.href='<%=request.getContextPath()%>/student?method=deleteById&id='+id;
}
}
</script>
</body>
</html>
四、Tomcat 的目录结构
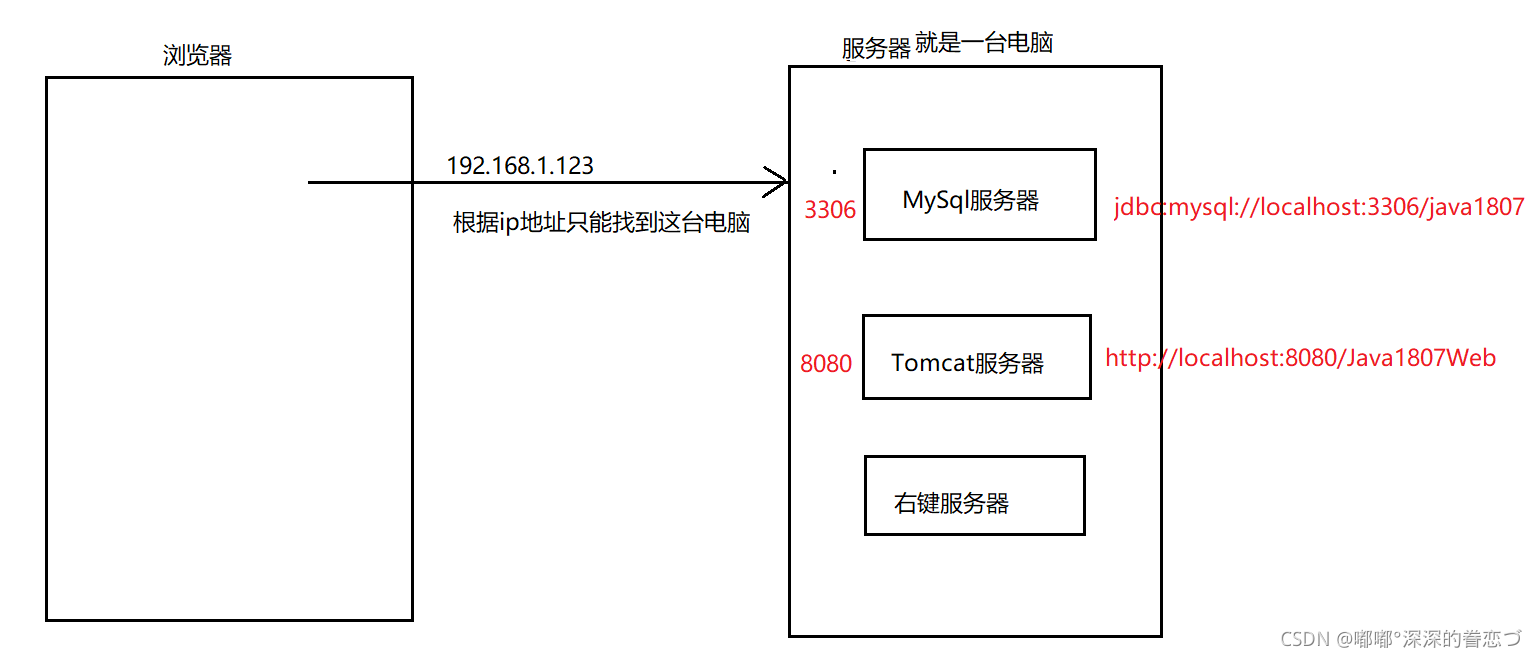
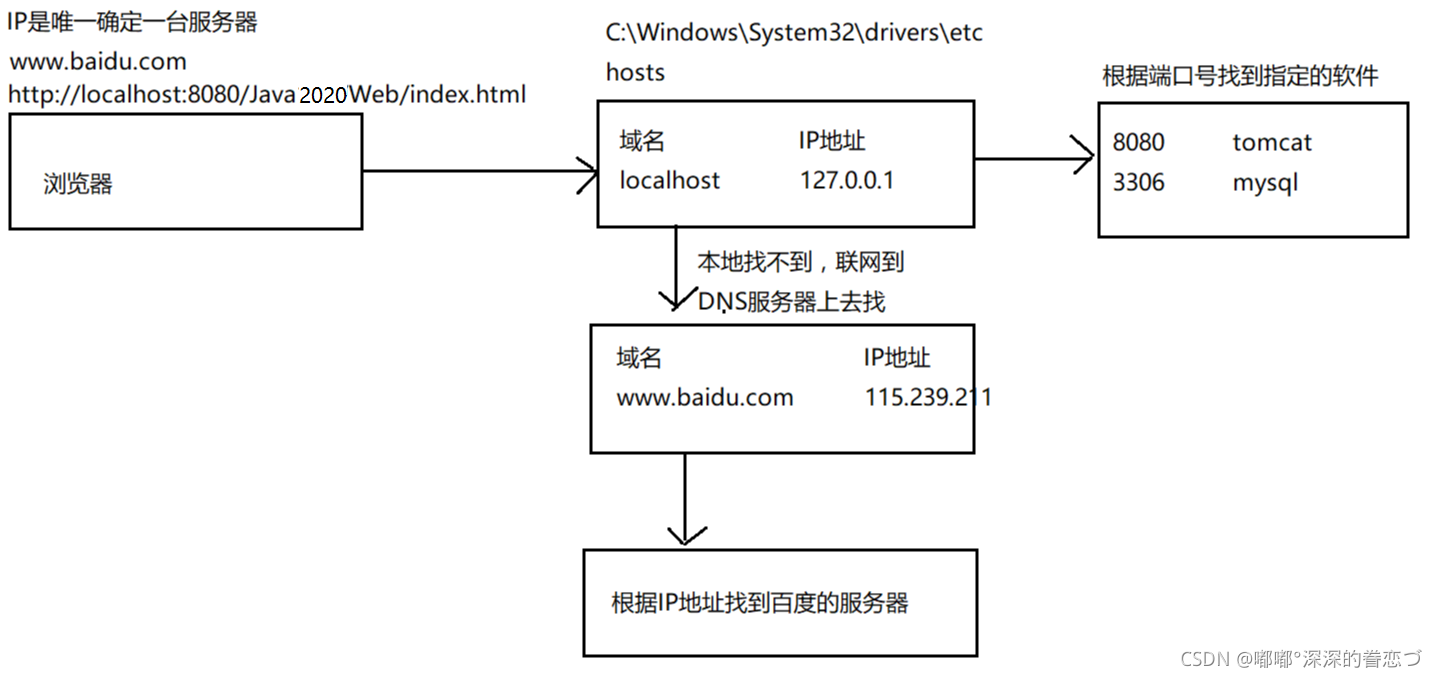
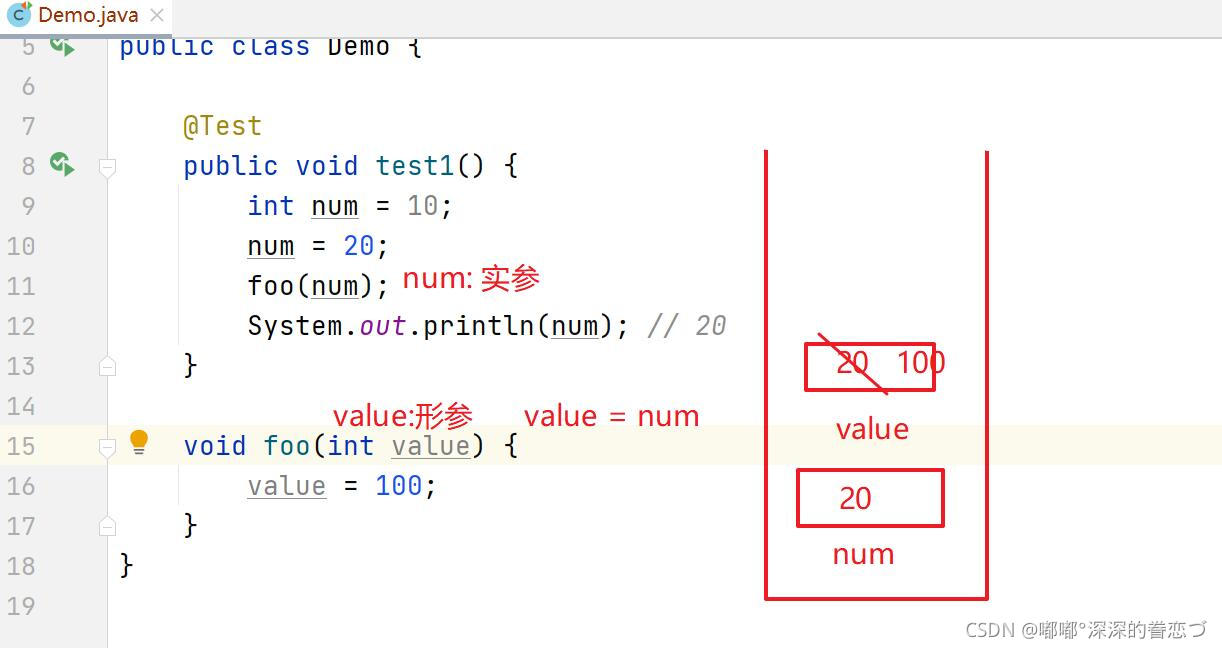
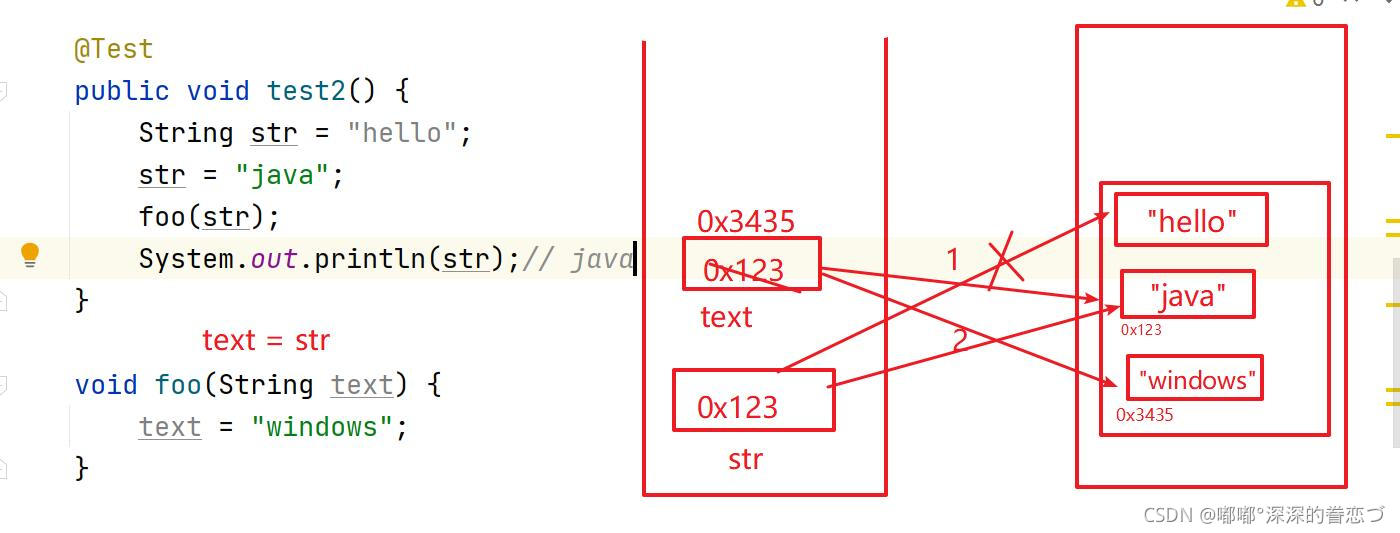