1.为什么需要划分
1.1 引入
像我们之前的这个项目,很常见的Spring+SpringMVC+Mybatis整合开发的web应用,在这个应用中,我们习惯性的把项目分了这么几层:
(1) Web层,负责与客户端交互,主要是一些Controller类
(2) Service层,处理业务逻辑,放一些Service接口和实现类
(3) Mapper层负责与数据库的交互
(4) Domain层,存放封装数据的实体类
对应的结构图如下:
但随着项目的进行,我们可能遇到下面一系列的问题:
(1) 大部分的domain或者一些service以及mapper在多个项目中是通用的
(2) Pom.xml中的依赖越来越长
(3) Build整个项目的时间越来越长,尽管你只是一直在web层工作,但是不得不build整个项目
(4) 某个模块,比如mapper,你只想让一些经验丰富的人来维护,但是现在每个开发者都能修改这个模块,这导致关键模块的代码质量达不到你的要求
所以需要把一个大项目拆分为多个小项目(maven模块)组成,而且它们是有依赖关系的
划分如下
还要注意配置文件拆分:
web.xml配置
mvc:web配置
service :service配置
mapper: db,数据源配置,mapper配置
1.2 basic-common
BaseService:
package cn.itsource.common.base;
import java.util.List;
import com.github.pagehelper.PageInfo;
/**
* 代号: 隐无为
* 用途:基类接口
*/
public interface BaseService<T> {
/**
* @功能: 分页查询
* @代号: 隐无为
*/
public PageInfo<T> queryPageList(Integer pageNum, Integer pageSize);
/**
* @功能:分页查询(默认pageSize)
* @代号:隐无为
*/
public PageInfo<T> queryPageList(Integer pageNum);
/**
* @功能:条件参数分页查询
* @代号:隐无为
*/
public PageInfo<T> queryPageListByParam(Integer pageNum, Integer pageSize, T model);
/**
* @功能:模糊条件参数分页查询
* @代号:隐无为
*/
public PageInfo<T> queryPageListByLike(Integer pageNum, Integer pageSize, T model);
/**
* @return
* @功能:条件参数分页查询(默认pageSize)
* @代号:隐无为
*/
public PageInfo<T> queryPageListByParam(Integer pageNum, T model);
/**
* @功能:查询所有
* @代号:隐无为
*/
public List<T> queryAllList();
/**
* @param model
* @功能:带有条件参数的查询
* @代号:隐无为
*/
public List<T> queryParamList(T model);
/**
* @功能:查询单个实体类
* @代号:隐无为
*/
public T queryById(Object id);
/**
* @return
* @功能:带有条件参数单个实体类的查询
* @代号:隐无为
*/
public T queryByParam(T model);
/**
* @param model
* @功能:根据实体中的属性查询总数
* @代号:隐无为
*/
public Integer queryCount(T model);
/**
* @param model
* @功能:保存一个实体 null值的属性也会保存,不会使用数据库默认值
* @代号:隐无为
*/
public Integer insert(T model);
/**
* @param model
* @功能:保存一个实体,null值的属性不会保存,会使用数据库默认值
* @代号:隐无为
*/
public Integer insertNoNull(T model);
/**
* @param model
* @return
* @功能:根据实体属性作为条件进行删除
* @代号:隐无为
*/
public Integer deleteByParam(T model);
/**
* @param
* @功能:根据主键字段进行删除
* @代号:隐无为
*/
public Integer delete(Object id);
/**
* @param model
* @return
* @功能:根据主键更新实体全部字段,null值会被更新
* @代号:隐无为
*/
public Integer update(T model);
/**
* @param model
* @功能:根据主键更新属性不为null的值
* @代号:隐无为
*/
public Integer updateNoNull(T model);
///
//
// 批量
//
///
/**
* @param list
* @功能:批量添加
* @代号:隐无为
*/
public Integer insertList(List<T> list);
/**
* @param ids
* @功能:根据主键字符串进行批量删除,类中要有一个带有@Id注解的字段
* @代号:隐无为
*/
public Integer deleteByIds(String ids);
/**
* @param ids
* @功能:ids批量查询,类中要有一个带有@Id注解的字段
* @代号:隐无为
*/
public List<T> queryByIds(String ids);
/**
* @param list
* @功能:批量更新
* @代号:隐无为
*/
public Integer updateList(List<T> list);
///
//
// 扩展
//
///
/**
* @param model
* @功能:逻辑删除
* @代号:隐无为
*/
public Integer updateDelete(T model);
/**
* @param ids
* @功能:批量逻辑删除
* @代号:隐无为
*/
public Integer updateDeletes(String ids);
}
BaseController:
package cn.itsource.common.base.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import cn.itsource.common.ResponseAPI;
import cn.itsource.utils.JsonReturnData;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class BaseController {
@Autowired
protected ResponseAPI responseAPI;
public static final String SUCCESS = "success";
public static final String ERROR = "error";
public static final String ERRORCODE = "500";
/**
* 功能: 异常统一捕获
* 作者: 柯栋 @代号:隐无为
*/
@ExceptionHandler
@ResponseBody
public String exceptionProcess(HttpServletRequest request, HttpServletResponse response, RuntimeException ex) {
JsonReturnData<String> jsonReturnData = new JsonReturnData<String>(500, "error");
ex.printStackTrace();
return responseAPI.getJsonString(jsonReturnData);
}
}
ResponseAPI:
package cn.itsource.common;
import java.io.IOException;
import java.text.SimpleDateFormat;
import org.springframework.stereotype.Component;
import com.fasterxml.jackson.core.JsonGenerationException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
/**
* 响应单例类 spring Component
*/
@Component
public class ResponseAPI {
//实例化jackson mapperX
public ObjectMapper mapper = new ObjectMapper();
public SimpleDateFormat sdf=new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
/**
* jackson 的 json 序列化
*/
public String getJsonString(Object object){
String result =null;
try {
mapper.setDateFormat(sdf);
result= mapper.writeValueAsString(object);
} catch (JsonGenerationException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
// /**
// * jackson 的 json 序列化 (null 忽略)
// */
// public String getJsonStringNoNull(Object object){
// String result =null;
// try {
// ObjectMapper mapperNoNull1 = new ObjectMapper();
// System.out.println(mapperNoNull1.getSerializerProvider());
// System.out.println(mapperX.getSerializerProvider());
// mapperNoNull1.setDateFormat(sdf);
// mapperNoNull1.setSerializationInclusion(Inclusion.NON_NULL);
// result= mapperX.writeValueAsString(object);
// } catch (JsonGenerationException e) {
// e.printStackTrace();
// } catch (JsonMappingException e) {
// e.printStackTrace();
// } catch (IOException e) {
// e.printStackTrace();
// }
// return result;
// }
/**@功能: json转List集合
* @代号:隐无为
* @param jsonStr
* @param targetClass 目标类
* @return list<targetClass> 返回list集合
*//*
public <T> T getJsonToList(String jsonStr, Class<?>targetClass) {
JavaType javaType = mapperX.getTypeFactory().constructParametricType(List.class, targetClass);
try {
return mapperX.readValue(jsonStr, javaType);
} catch (JsonParseException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
*//**@功能: json 转换 bean 对象
* @代号:隐无为
* @param jsonStr
* @return
*//*
public <T> T getJsonToBean(String jsonStr,Class<T> c){
try {
return mapperX.readValue(jsonStr, c);
} catch (JsonParseException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (JsonMappingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
*//**@功能: 集合类转换
* @代号:隐无为
* @param jsonStr
* @param collectionClass 集合类class
* @param targetClass 目标类
* @return
*//*
public <T> T readJson(String jsonStr, Class<?> collectionClass, Class<?>... targetClass) {
JavaType javaType = mapperX.getTypeFactory().constructParametricType(collectionClass, targetClass);
try {
return mapperX.readValue(jsonStr, javaType);
} catch (JsonParseException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
*/
//测试
// public static void main(String[] args) throws JsonParseException, JsonMappingException, IOException {
// ResponseAPI api=new ResponseAPI();
// Test t=new Test();
// t.setId(null);//int 类型
// t.setName(null);//字符串
// t.setDate(new Date());//时间
String json1= api.getJsonString(t);
Test tt=api.getJsonToBean(json1, Test.class);
System.out.println(tt.getDate());
// Test t2=new Test();
// t2.setId(1);//int 类型
// t2.setName("T");//字符串
// t2.setDate(new Date());//时间
// List<Test>list=new ArrayList<Test>();
// list.add(t2);
// list.add(t);
//
// String json3= api.getJsonStringNoNull(list);
// System.out.println(json3);
// String json2= api.getJsonString(list);
// System.out.println(json2);
List<Test> list2 = api.getJsonToList(json2, Test.class);
System.out.println(list2.size());
// }
}
crm-web-mavenweb项目
crm-service本模块作为一个项目表现层,依赖crm-service
Controller
而现在我们的整体架构为前后端分离架构,所有的静态资源全部在前端项目中
2、接口规范swagger
这是使用swagger所需要的配置文件
<springfox.version>2.7.0</springfox.version>
</properties>
<dependencies>
<dependency>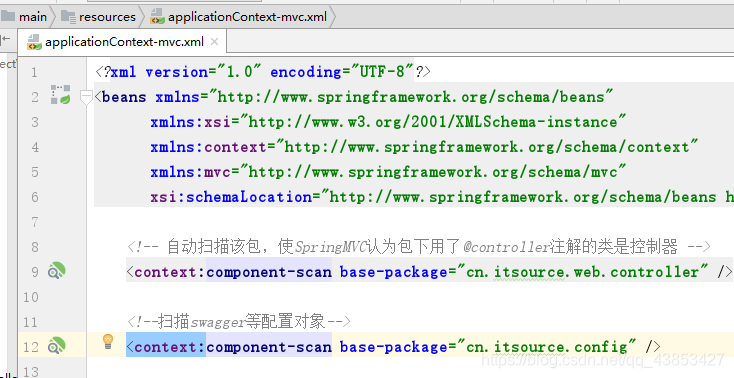
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>${springfox.version}</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>${springfox.version}</version>
</dependency>
不要忘记扫描包
运行:
http://localhost/swagger-ui.html
就可以进入
可以在此测试,无需使用浏览器