继承Runnable接口实现
public class TicketSaling implements Runnable{
private int ticket=100;
private Object obj= new Object();
public void run(){
while(ticket>0){
synchronized(obj){
System.out.println(Thread.currentThread().getName()+
"售出一张票,剩余"+--ticket+"张票");
}
try{
Thread.sleep(10);
}
catch(InterruptedException e){
e.printStackTrace();
}
}
}
}
售票窗口的测试类
public class TicketSalingTest {
public static void main(String[] args){
TicketSaling t=new TicketSaling();
Thread t1=new Thread(t,"售票窗口1");
Thread t2=new Thread(t,"售票窗口2");
Thread t3=new Thread(t,"售票窗口3");
Thread t4=new Thread(t,"售票窗口4");
t1.start();
t2.start();
t3.start();
t4.start();
}
}
存钱 类 采用继承Thread的子类创建线程,采用同步方法实现线程同步,注意互斥资源使用静态资源,同步方法也要定义为静态方法,使得所有对象都是用同一个资源和同一个方法(没有采用静态方法前,会才生线程互斥操作)
public class SaveMoney extends Thread{
private static int count=0;
public SaveMoney(String str){
super(str);
}
public synchronized static void saveMoney(){
System.out.println(Thread.currentThread().getName()+
"存入100元,银行总资金为"+(count+=100));
}
public void run(){
for(int i=0;i<4;i++){
saveMoney();
try{
Thread.sleep(100);
}
catch(Exception e){
e.printStackTrace();
}
}
}
}
测试类
public class SaveMoneyTest {
public static void main(String[] args){
SaveMoney t1= new SaveMoney("老张");
SaveMoney t2= new SaveMoney("老王");
t1.start();
t2.start();
}
}
测试结果
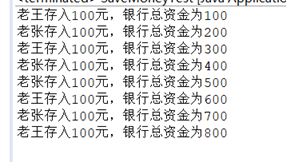
最后一问不仅要实现线程同步,还有考虑线程间的通信,这样才能让读写操作能够交替进行。主要使用wait方法和notify方法,也就线程阻塞和线程唤醒。
互斥资源类
public class Data{
private TreeMap<String,String> data= new TreeMap<String,String>();
boolean isWrited=false;//是否为写入状态
public synchronized void readData(){
if(!isWrited){
try{
wait();
}catch(InterruptedException e){
e.printStackTrace();
}
}
System.out.println("读取数据: "+data);
isWrited=false;
notify();
}
public synchronized void writeData(String name,String sex){
if(isWrited){
try{
wait();
}
catch(InterruptedException e){
e.printStackTrace();
}
}
data.put(name, sex);
System.out.println("写入数据: "+name+" "+sex);
isWrited=true;
notify();
}
}
写线程
public class WriteThread extends Thread{
Data data=new Data();
public WriteThread(Data data){
this.data=data;
}
public void run(){
data.writeData("小明", "男");
data.writeData("小红", "女");
data.writeData("小冉", "女");
data.writeData("小烨", "男");
}
}
读线程
public class ReadThread extends Thread{
Data data=new Data();
public ReadThread(Data data){
this.data=data;
}
public void run(){
while(true){
data.readData();
}
}
}
测试类
public class TestThread {
public static void main(String[] args){
Data data=new Data();
WriteThread w=new WriteThread(data);
ReadThread r=new ReadThread(data);
w.start();
r.start();
}
}
注意线程唤醒和阻塞的条件,不然会产生线程死锁。
测试结果
Java多线程与线程同步
最新推荐文章于 2024-07-22 14:15:06 发布