1.1 Collection
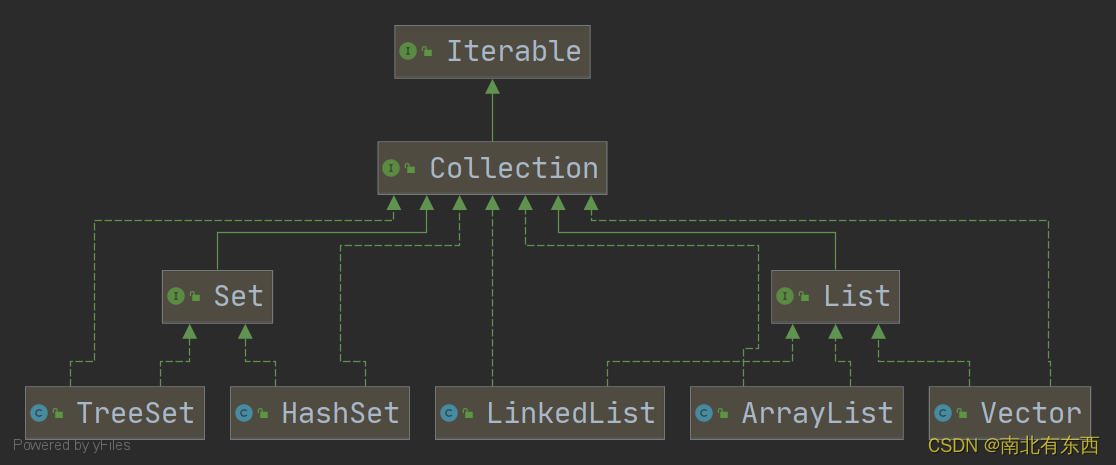
1.1常用的方法
public class CollectionMethod {
@SuppressWarnings({"all"})
public static void main(String[] args) {
List list = new ArrayList();
list.add("jack");
list.add(10);
list.add(true);
System.out.println("list=" + list);
list.remove(true);
System.out.println("list=" + list);
System.out.println(list.contains("jack"));
System.out.println(list.size());
System.out.println(list.isEmpty());
list.clear();
System.out.println("list=" + list);
ArrayList list2 = new ArrayList();
list2.add("红楼梦");
list2.add("三国演义");
list.addAll(list2);
System.out.println("list=" + list);
System.out.println(list.containsAll(list2));
list.add("聊斋");
list.removeAll(list2);
System.out.println("list=" + list);
}
1.2 迭代器(Iterator)
public class CollectionIterator {
@SuppressWarnings({"all"})
public static void main(String[] args) {
Collection col = new ArrayList();
col.add(new Book("三国演义", "罗贯中", 10.1));
col.add(new Book("小李飞刀", "古龙", 5.1));
col.add(new Book("红楼梦", "曹雪芹", 34.6));
Iterator iterator = col.iterator();
while (iterator.hasNext()) {
Object obj = iterator.next();
System.out.println("obj=" + obj);
}
迭代器
iterator = col.iterator();
System.out.println("===第二次遍历===");
while (iterator.hasNext()) {
Object obj = iterator.next();
System.out.println("obj=" + obj);
}
}
}
class Book {
private String name;
private String author;
private double price;
public Book(String name, String author, double price) {
this.name = name;
this.author = author;
this.price = price;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", author='" + author + '\'' +
", price=" + price +
'}';
}
2 List接口
- List集合类中的元素是有序的,可重复
- List集合中每个元素都有对应的索引,可用get()得到
List常用方法
public class ListMethod {
@SuppressWarnings({"all"})
public static void main(String[] args) {
List list = new ArrayList();
list.add("张三丰");
list.add("贾宝玉");
list.add(1, "Curry");
System.out.println("list=" + list);
List list2 = new ArrayList();
list2.add("jack");
list2.add("tom");
list.addAll(1, list2);
System.out.println("list=" + list);
System.out.println(list.indexOf("tom"));
list.add("Curry");
System.out.println("list=" + list);
System.out.println(list.lastIndexOf("Curry"));
list.remove(0);
System.out.println("list=" + list);
list.set(1, "玛丽");
System.out.println("list=" + list);
List returnlist = list.subList(0, 2);
System.out.println("returnlist=" + returnlist);
}
}
3 ArrayList
- ArrayList: 维护了一个Object[]数组。
- 扩容机制:
1.如果使用无参构造器,则数组初始容量为0,第一次添加后容量为10,再次扩容就会变成1.5倍
2.如果使用指定大小的构造器,则初始为指定大小,扩容就变成1.5倍。
4 Vector
- Vector底层也是一个Object[]数组,但Vector是线程安全的,效率没有ArrayListg高
- 扩容机制:
1.如果使用无参构造器,则数组初始容量为10,再次扩容就会变成2倍
2.如果使用指定大小的构造器,则初始为指定大小,扩容就变成2倍。
5 LinkedList
6 Set接口
7 HashSet
- 实现了Set接口
- 底层是HashMap(数组+链表)
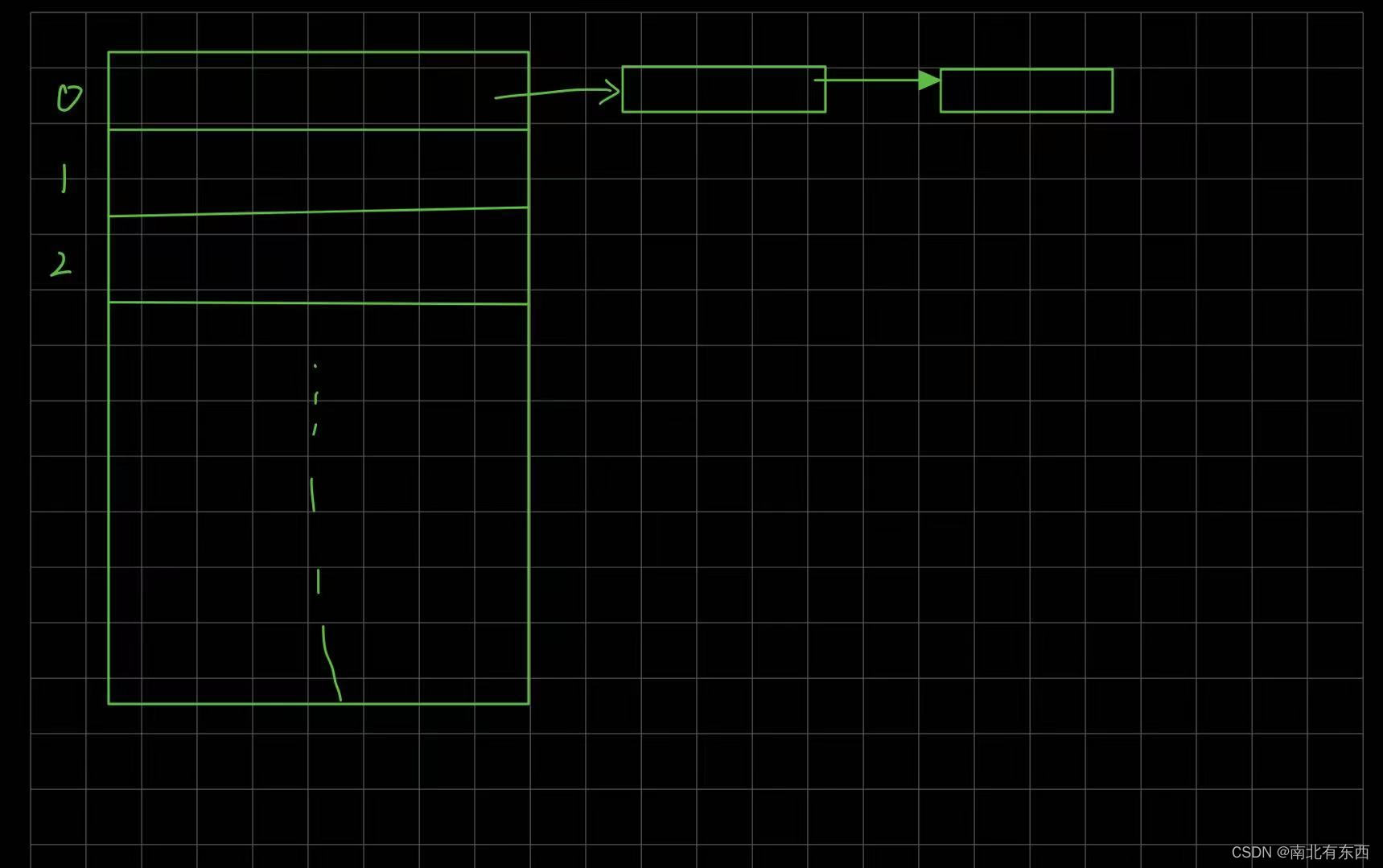
7.1 HashSet添加元素的步骤
- 添加一个元素时,会先得到Hash值,然后转成索引值
- 在存储数据表中的索引上调用equals方法比较,如果有相同元素,就放弃添加,没有就添加的链表尾部
- jdk8中,如果链表元素超过阈值(16),且索引表的大小>=设定的值(64),就会进行树化(红黑树)
8 LinkedHashSet
- 底层是LinkedHashMap(数组+双向链表)
9 Collections工具类
public class Collections_ {
public static void main(String[] args) {
List list = new ArrayList();
list.add("tom");
list.add("smith");
list.add("king");
list.add("milan");
list.add("tom");
Collections.reverse(list);
System.out.println("list=" + list);
Collections.sort(list);
System.out.println("自然排序后");
System.out.println("list=" + list);
Collections.sort(list, new Comparator() {
@Override
public int compare(Object o1, Object o2) {
return ((String) o2).length() - ((String) o1).length();
}
});
System.out.println("字符串长度大小排序=" + list);
Collections.swap(list, 0, 1);
System.out.println("交换后的情况");
System.out.println("list=" + list);
System.out.println("自然顺序最大元素=" + Collections.max(list));
Object maxObject = Collections.max(list, new Comparator() {
@Override
public int compare(Object o1, Object o2) {
return ((String)o1).length() - ((String)o2).length();
}
});
System.out.println("长度最大的元素=" + maxObject);
System.out.println("tom出现的次数=" + Collections.frequency(list, "tom"));
ArrayList dest = new ArrayList();
for(int i = 0; i < list.size(); i++) {
dest.add("");
}
Collections.copy(dest, list);
System.out.println("dest=" + dest);
Collections.replaceAll(list, "tom", "汤姆");
System.out.println("list替换后=" + list);
}