创建应用
配置应用
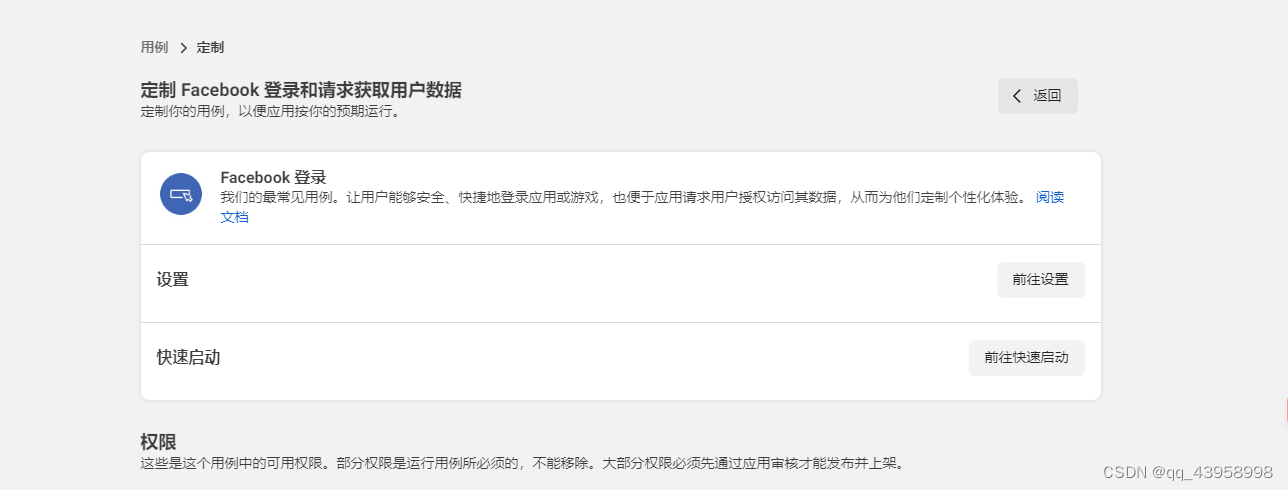
获得应用密钥
前端获得授权地址
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Facebook Login</title>
</head>
<body>
<h2>Facebook Login</h2>
<!-- Button to trigger Facebook login -->
<button id="loginBtn">Login with Facebook</button>
<!-- Set the element id for the JSON response -->
<p id="profile"></p>
<script>
// Add the Facebook SDK for Javascript
(function(d, s, id){
var js, fjs = d.getElementsByTagName(s)[0];
if (d.getElementById(id)) { return; }
js = d.createElement(s); js.id = id;
js.src = "https://connect.facebook.net/en_US/sdk.js";
fjs.parentNode.insertBefore(js, fjs);
}(document, 'script', 'facebook-jssdk'));
window.fbAsyncInit = function() {
// Initialize the SDK with your app and the Graph API version for your app
FB.init({
appId : '367426305825029',
xfbml : true,
version : 'v20.0'
});
// Check if user is logged in and if not, prompt login dialog
FB.getLoginStatus(function(response) {
if (response.status === 'connected') {
// Fetch user information if already logged in
fetchUserProfile();
}
});
};
function fetchUserProfile() {
FB.api('/me', {fields: 'name,email'}, function(response) {
document.getElementById("profile").innerHTML = "Good to see you, " + response.name + ". I see your email address is " + response.email;
});
}
// Add event listener to login button
document.getElementById('loginBtn').addEventListener('click', function() {
FB.login(function(response) {
console.log(response);
if (response.authResponse) {
fetchUserProfile();
} else {
console.log('User cancelled login or did not fully authorize.');
}
}, {scope: 'public_profile,email'});
});
</script>
</body>
</html>
登录后返回参数:
{
"authResponse": {
"accessToken": "****",
"userID": "********",
"expiresIn": *****,
"signedRequest": "********",
"graphDomain": "facebook",
"data_access_expiration_time": 1724305147
},
"status": "connected"
}
后端验证token获取用户信息
package com.example.facebooklogin;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.client.RestTemplate;
import java.io.IOException;
import java.util.Map;
@RestController
public class FacebookLoginController {
@Value("${facebook.app.id}")
private String facebookAppId;
@Value("${facebook.app.secret}")
private String facebookAppSecret;
private static final String FACEBOOK_GRAPH_API_URL = "https://graph.facebook.com/me?fields=id,name,email&access_token=";
private static final String FACEBOOK_TOKEN_DEBUG_URL = "https://graph.facebook.com/debug_token?input_token=%s&access_token=%s|%s";
@PostMapping("/verifyToken")
public ResponseEntity<?> verifyFacebookToken(@RequestBody Map<String, String> request) {
String accessToken = request.get("accessToken");
// 先验证访问令牌的有效性
if (!validateAccessToken(accessToken)) {
return ResponseEntity.status(401).body("Invalid access token");
}
String url = FACEBOOK_GRAPH_API_URL + accessToken;
RestTemplate restTemplate = new RestTemplate();
ResponseEntity<String> response = restTemplate.getForEntity(url, String.class);
if (response.getStatusCode().is2xxSuccessful()) {
try {
ObjectMapper objectMapper = new ObjectMapper();
JsonNode userInfo = objectMapper.readTree(response.getBody());
// 获取用户信息
String userId = userInfo.get("id").asText();
String userName = userInfo.get("name").asText();
String userEmail = userInfo.get("email").asText();
// 处理用户信息,例如在数据库中创建或更新用户
// ...
// 返回用户信息
return ResponseEntity.ok(userInfo);
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.status(500).body("Error parsing user info");
}
} else {
return ResponseEntity.status(response.getStatusCode()).body("Invalid access token");
}
}
private boolean validateAccessToken(String accessToken) {
String debugUrl = String.format(FACEBOOK_TOKEN_DEBUG_URL, accessToken, facebookAppId, facebookAppSecret);
RestTemplate restTemplate = new RestTemplate();
ResponseEntity<String> response = restTemplate.getForEntity(debugUrl, String.class);
if (response.getStatusCode().is2xxSuccessful()) {
try {
ObjectMapper objectMapper = new ObjectMapper();
JsonNode debugInfo = objectMapper.readTree(response.getBody());
JsonNode data = debugInfo.get("data");
return data.get("is_valid").asBoolean();
} catch (IOException e) {
e.printStackTrace();
return false;
}
} else {
return false;
}
}
}
需要代理在发送请求前添加以下代码
System.setProperty("proxyHost", "127.0.0.1"); // PROXY_HOST:代理的IP地址
System.setProperty("proxyPort", "1009"); // PROXY_PORT:代理的端口号