AIDL连接池
创建AIDL接口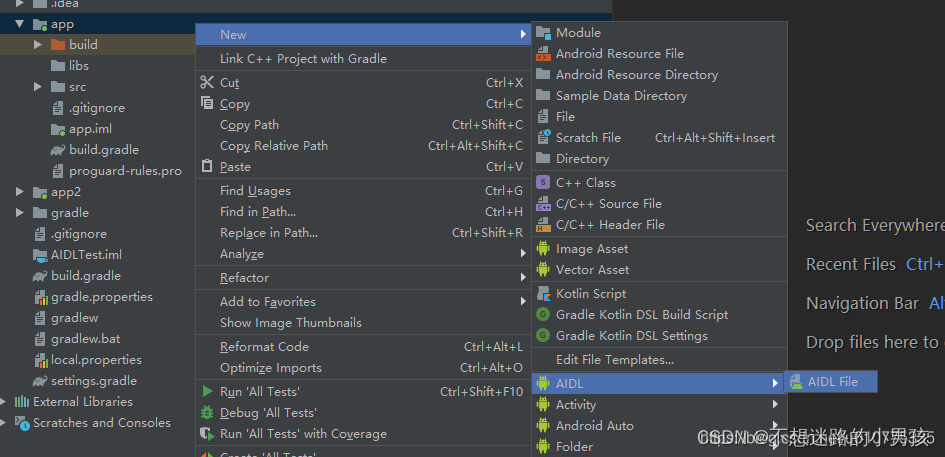
ISecurityCenter.aidl
interface ISecurityCenter {
String encrypt(String content);
String decrypt(String password);
}
ICompute.aidl
interface ICompute {
int add(int a, int b);
}
IBinderPool.aidl
interface IBinderPool {
IBinder queryBinder(int binderCode);
}
注意:创建好接口后记得Rebuild一下,让AIDL自动生成相应代码
实现AIDL接口:
public class SecurityCenterImpl extends ISecurityCenter.Stub{
private static final char SECRET_CODE = '^';
@Override
public String encrypt(String content) throws RemoteException {
char[] chars = content.toCharArray();
for (int i = 0; i < chars.length; i++) {
//^运算符,参加运算的两个对象,如果两个相应位为“异”(值不同),则该位结果为1,否则为0。
chars[i] ^= SECRET_CODE;
}
return new String(chars);
}
@Override
public String decrypt(String password) throws RemoteException {
return encrypt(password);
}
}
public class ComputeImpl extends ICompute.Stub {
@Override
public int add(int a, int b) throws RemoteException {
return a + b;
}
}
public class BinderPoolImpl extends IBinderPool.Stub {
public static final int BINDER_COMPUTE = 0;
public static final int BINDER_SECURITY_CENTER = 1;
public BinderPoolImpl() {
super();
}
@Override
public IBinder queryBinder(int binderCode) throws RemoteException {
IBinder binder = null;
switch (binderCode){
case BINDER_SECURITY_CENTER:{
binder = new SecurityCenterImpl();
break;
}
case BINDER_COMPUTE:
binder = new ComputeImpl();
break;
default:
break;
}
return binder;
}
}
创建远程Service
public class BinderPoolService extends Service {
public static final String TAG = BinderPoolService.class.getSimpleName();
private Binder mBinderPool = new BinderPoolImpl();
public BinderPoolService() {
}
@Override
public IBinder onBind(Intent intent) {
Log.d(TAG, "onBind: ");
return mBinderPool;
}
@Override
public void onCreate() {
Log.d(TAG, "onCreate: ");
super.onCreate();
}
@Override
public void onDestroy() {
Log.d(TAG, "onDestroy: ");
super.onDestroy();
}
}
注:别忘了在服务中注册服务
实现Binder连接池
在它的内部首先要去绑定远程服务,绑定成功后,客户端可以通过它的queryBinder方法去获取各自对应的Binder
public class BinderPool {
public static final String TAG = "BinderPool";
public static final int BINDER_NONE = -1;
public static final int BINDER_COMPUTE = 0;
public static final int BINDER_SECURITY_CENTER = 1;
private static Context mContext;
private IBinderPool mBinderPool;
private static BinderPool instance;
private CountDownLatch mConnectBinderPoolCountDownLatch;
private BinderPool(Context context){
mContext = context;
connectBinderPoolService();
}
public static BinderPool getInstance(Context context){
if(instance == null){
synchronized (BinderPool.class){
if(instance == null){
instance = new BinderPool(context);
}
}
}
return instance;
}
private synchronized void connectBinderPoolService(){
mConnectBinderPoolCountDownLatch = new CountDownLatch(1);
Intent service = new Intent(mContext, BinderPoolService.class);
mContext.bindService(service, mBinderPoolConnection, Context.BIND_AUTO_CREATE);
}
public IBinder queryBinder(int binderCode){
IBinder binder = null;
try {
if(mBinderPool != null){
binder = mBinderPool.queryBinder(binderCode);
}
} catch (RemoteException e) {
e.printStackTrace();
}
return binder;
}
private ServiceConnection mBinderPoolConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
Log.d(TAG, "onServiceConnected: 服务连接成功");
mBinderPool = IBinderPool.Stub.asInterface(service);
try {
mBinderPool.asBinder().linkToDeath(mBinderPoolDeathRecipient, 0);
} catch (RemoteException e) {
e.printStackTrace();
}
mConnectBinderPoolCountDownLatch.countDown();
}
@Override
public void onServiceDisconnected(ComponentName name) {
}
};
private IBinder.DeathRecipient mBinderPoolDeathRecipient = new IBinder.DeathRecipient() {
@Override
public void binderDied() {
mBinderPool.asBinder().unlinkToDeath(mBinderPoolDeathRecipient, 0);
mBinderPool = null;
connectBinderPoolService();
}
};
}
调用代码:
BinderPool binderPool = BinderPool.getInstance(MainActivity.this);
new Handler().postDelayed(() -> {
IBinder securityBinder = binderPool.queryBinder(BinderPool.BINDER_SECURITY_CENTER);
mSecurityCenter = SecurityCenterImpl.asInterface(securityBinder);
Log.d(TAG, "doWork: visit ISecurityCenter");
String msg = "helloWorld-安卓";
System.out.println("content:"+msg);
try {
String password = mSecurityCenter.encrypt(msg);
System.out.println("encrypt :"+password);
System.out.println("decrypt:"+mSecurityCenter.decrypt(password));
} catch (RemoteException e) {
e.printStackTrace();
}
Log.d(TAG, "doWork: visit ICompute");
IBinder computeBinder = binderPool.queryBinder(BinderPool.BINDER_COMPUTE);
mCompute = ComputeImpl.asInterface(computeBinder);
try {
System.out.println("3 + 5 = "+mCompute.add(3, 5));
} catch (RemoteException e) {
e.printStackTrace();
}
}, 2000);
最后附上效果图: