HashSet
package com.wdzl.demo01;
import java.util.HashSet;
import java.util.Iterator;
public class TestHashSet {
public static void main(String[] args) {
HashSet<String> hs = new HashSet<String>();
hs.add("RRR");
hs.add("DDD");
hs.add("SSS");
hs.add("AAA");
hs.add(null);
System.out.println(hs);
System.out.println(hs.size());
System.out.println(hs.isEmpty());
System.out.println(hs.contains("DDD"));
System.out.println("==="+hs);
for (String string : hs) {
System.out.println(string);
}
Iterator<String> it = hs.iterator();
while(it.hasNext()) {
System.out.println(it.next());
}
System.out.println("------------------------");
hs.forEach(s -> System.out.println(s));
}
}
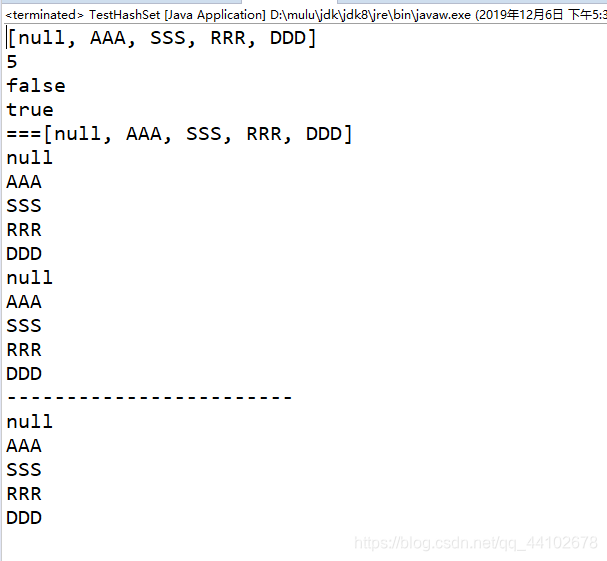
package com.wdzl.demo01;
import java.util.HashMap;
import java.util.HashSet;
public class TestHashSet2 {
public static void main(String[] args) {
HashSet<Student> hs = new HashSet<Student>();
Student st1 = new Student("zhang", 22);
Student st2 = new Student("zhang", 22);
Student st3 = new Student("zhang", 22);
hs.add(st1);
hs.add(st2);
hs.add(st3);
System.out.println(hs.size());
}
}
class Student{
private String name;
private int age;
public Student(String name,int age) {
this.age = age;
this.name = name;
}
@Override
public String toString() {
return "Student [name=" + name + ", age=" + age + "]";
}
@Override
public int hashCode() {
System.out.println("------hashCode()-----");
return 2;
}
@Override
public boolean equals(Object obj) {
System.out.println("=========equals======");
if(obj.getClass()!=this.getClass()) {
return false;
}
Student student = (Student)obj;
boolean is = (this.age==student.age)&&(this.name.equals(student.name));
return is;
}
}
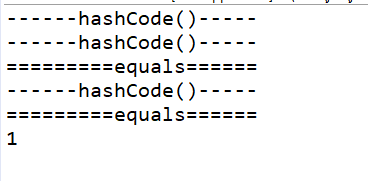
Map
HashMap
package com.wdzl.demo02;
import java.util.HashMap;
import java.util.Map.Entry;
import java.util.Set;
public class TestHashMap {
public static void main(String[] args) {
HashMap<String, String> map = new HashMap<String, String>();
map.put("sky", "天空");
map.put("book", "书");
System.out.println(map.size());
System.out.println(map.isEmpty());
map.remove("sky", "天空");
map.clear();
System.out.println(map);
map.keySet();
map.values();
Set<Entry<String, String>> entrys = map.entrySet();
for (Entry<String, String> entry : entrys) {
System.out.println(entry.getKey()+"=="+entry.getValue());
}
HashMap<Student, School> hh = new HashMap<Student, School>();
}
}
class Student{}
class School{}
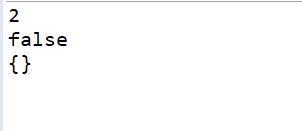
TreeMap
package com.wdzl.demo02;
import java.util.Set;
public class TestTreeMap {
public static void main(String[] args) {
My my = new My();
TreeMap<MyKey, String> tm = new TreeMap<MyKey, String>(my);
tm.put(new MyKey(3), "叁");
tm.put(new MyKey(5), "伍");
tm.put(new MyKey(6), "陆");
tm.put(new MyKey(7), "柒");
System.out.println(tm);
}
static class My implements Comparator<MyKey> {
@Override
public int compare(MyKey o1, MyKey o2) {
return o1.id-o2.id;
}
}
}
class MyKey{
int id;
public MyKey(int id) {
this.id = id;
}
@Override
public String toString() {
return "MyKey [id=" + id + "]";
}
}

HashTable
package com.wdzl.demo02;
import java.util.Hashtable;
import java.util.Properties;
public class TestHashtable {
public static void main(String[] args) {
Hashtable<String, String> ht = new Hashtable<String, String>();
ht.put("name", "zhang");
System.out.println(ht);
}
}
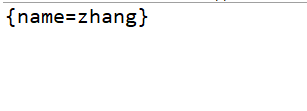
Properties
package com.wdzl.demo02;
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
public class TestProperties {
public static void main(String[] args) throws IOException {
InputStream is = TestProperties.class.getResourceAsStream("/config.properties");
Properties p = new Properties();
p.load(is);
String ip = p.getProperty("ip");
String name = p.getProperty("username");
String pass = p.getProperty("password");
System.out.println(ip+"=="+name+"=="+pass);
is.close();
}
public static void test(String[] args) {
Properties p = new Properties();
p.put("a", "AAA");
p.setProperty("b", "BBBB");
p.setProperty("c", "CCC");
String a = p.getProperty("a");
String b = (String)p.get("b");
System.out.println(a+"==="+b);
System.out.println(p);
}
}
