import static java.lang.Math.*;
public class MathDemo {
public static void main(String[] args) {
double pow = pow(2, 8);
System.out.println("pow(2,8) = " + pow);
double x = ceil(-2.3);
double y = ceil(0);
double z = ceil(+2.3);
System.out.println("ceil(-2.3) = " + x);
System.out.println("ceil(0) = " + y);
System.out.println("ceil(+2.3) = " + z);
int totalRecords = 107;
final int PAGE_SIZE = 10;
int pageCount = (int)Math.ceil(totalRecords * 1.0 / PAGE_SIZE);
System.out.println("总共107条记录,每页显示10条记录,共" + pageCount + "页");
x = floor(-2.3);
y = floor(0);
z = floor(+2.3);
System.out.println("floor(-2.3) = " + x);
System.out.println("floor(0) = " + y);
System.out.println("floor(+2.3) = " + z);
double random = Math.random();
System.out.println("Math.random() = " + random);
int count = 0;
for (int i = 0; i < 50; i++) {
int number = (int)((Math.random() * (100- 50 + 1)) + 50);
System.out.print(number + "\t");
if((i+1) % 10 == 0) {
System.out.println();
}
}
int min = (int)Math.min(10.0, 20.0);
System.out.println("Math.min(10.0, 20.0) = " + min);
int max = (int)Math.max(10.0, 20.0);
System.out.println("Math.max(10.0, 20.0) = " + max);
double sqrt = sqrt(9.0);
System.out.println("sqrt(9.0) = " + sqrt);
double cbrt = cbrt(8.0);
System.out.println("cbrt(8.0) = " + cbrt);
}
}
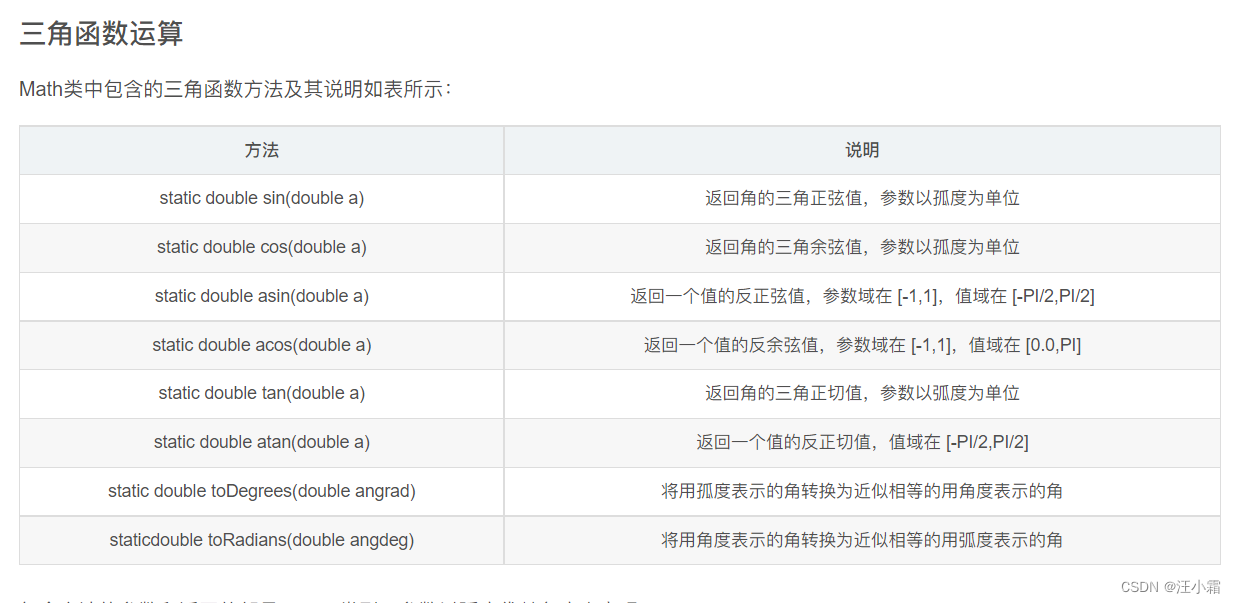