js小练习–日历
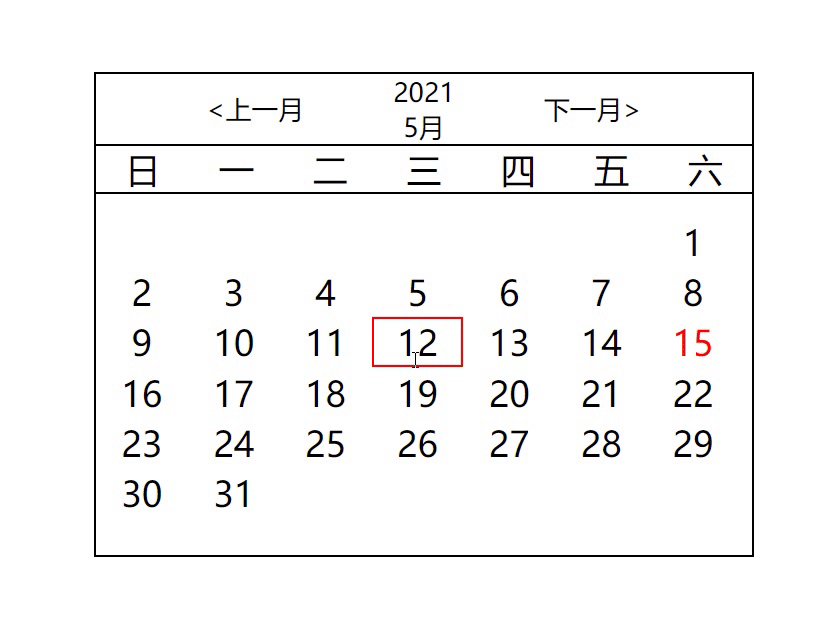
## css模块
<style type="text/css">
*{
margin: 0;
padding: 0;
}
.date{
width: 300px;
height: 220px;
border: 1px solid #000;
margin: 100px auto;
}
.title{
width: 200px;
display: flex;
font-size: 12px;
margin: auto;
text-align: center;
justify-content: space-around;
align-items: center;
}
.year{
margin: 0 40px;
display: flex;
flex-direction: column;
}
#week{
border-top: 1px solid #000;
border-bottom: 1px solid #000;
margin: auto;
list-style-type: none;
display: flex;
}
#week li{
display: inline-block;
text-align: center;
flex:1;
}
#ul{
list-style-type: none;
margin-top: 5px;
}
#ul li {
display: inline-block;
width: 40px;
height: 21px;
text-align: center;
border: 1px solid #fff;
}
.current{
color:red;
}
#ul li:hover{
border: 1px solid red;
}
#prev,#next{
cursor: pointer;
}
</style>
## html
<div class="date">
<div class="title">
<span id="prev"><上一月</span>
<div class="year">
<span id="year">2021</span>
<span id="month">5月</span>
</div>
<span id="next">下一月></span>
</div>
<ul id="week">
<li>日</li>
<li>一</li>
<li>二</li>
<li>三</li>
<li>四</li>
<li>五</li>
<li>六</li>
</ul>
<ul id="ul">
</ul>
</div>
## js代码
<script type="text/javascript">
let date = new Date();
document.getElementById('prev').addEventListener('click',function(){
date.setMonth(date.getMonth()-1);
add();
})
document.getElementById('next').addEventListener('click',function(){
date.setMonth(date.getMonth()+1);
add();
})
add();
function add(){
let cYear = date.getFullYear();
let cMonth = date.getMonth()+1;
let cDay = date.getDate();
document.getElementById('year').innerHTML = cYear;
document.getElementById('month').innerHTML = cMonth+'月';
let days = new Date(cYear,cMonth,-1);
let n = days.getDate()+1;
let week = new Date(cYear,cMonth-1,1).getDay();
let html = '';
for(let i=0;i<week;i++){
html+=`<li></li>`
}
for(let i=1;i<=n;i++){
if(i==cDay){
html+=`<li class="current">${i}</li>`
}else{
html+=`<li>${i}</li>`
}
}
document.getElementById('ul').innerHTML = html
}
</script>